Show messages for users if the ROOT_URL is wrong, show JavaScript errors (#18971)
* ROOT_URL issues: some users did wrong to there app.ini config, then:
* The assets can not be loaded (AppSubUrl != "" and users try to access http://host:3000/)
*The ROOT_URL is wrong, then many URLs in Gitea are broken.
Now Gitea show enough information to users.
* JavaScript error issues, there are many users affected by JavaScript errors, some are caused by frontend bugs, some are caused by broken customized templates. If these JS errors can be found at first time, then maintainers do not need to ask about how bug occurs again and again.
* Some people like to modify the `head.tmpl`, so we separate the script part to `head_script.tmpl`, then it's much safer.
* use specialized CSS class "js-global-error", end users still have a chance to hide error messages by customized CSS styles.
3 years ago
// bootstrap module must be the first one to be imported, it handles webpack lazy-loading and global errors
import './bootstrap.ts' ;
import './htmx.ts' ;
import { initDashboardRepoList } from './features/dashboard.ts' ;
import { initGlobalCopyToClipboardListener } from './features/clipboard.ts' ;
import { initContextPopups } from './features/contextpopup.ts' ;
import { initRepoGraphGit } from './features/repo-graph.ts' ;
import { initHeatmap } from './features/heatmap.ts' ;
import { initImageDiff } from './features/imagediff.ts' ;
import { initRepoMigration } from './features/repo-migration.ts' ;
import { initRepoProject } from './features/repo-projects.ts' ;
import { initTableSort } from './features/tablesort.ts' ;
import { initAutoFocusEnd } from './features/autofocus-end.ts' ;
import { initAdminUserListSearchForm } from './features/admin/users.ts' ;
import { initAdminConfigs } from './features/admin/config.ts' ;
import { initMarkupAnchors } from './markup/anchors.ts' ;
import { initNotificationCount , initNotificationsTable } from './features/notification.ts' ;
import { initRepoIssueContentHistory } from './features/repo-issue-content.ts' ;
import { initStopwatch } from './features/stopwatch.ts' ;
import { initFindFileInRepo } from './features/repo-findfile.ts' ;
import { initCommentContent , initMarkupContent } from './markup/content.ts' ;
import { initPdfViewer } from './render/pdf.ts' ;
import { initUserAuthOauth2 , initUserCheckAppUrl } from './features/user-auth.ts' ;
import {
initRepoIssueReferenceRepositorySearch ,
initRepoIssueWipTitle ,
initRepoPullRequestMergeInstruction ,
initRepoPullRequestAllowMaintainerEdit ,
Refactor issue filter (labels, poster, assignee) (#32771)
Rewrite a lot of legacy strange code, remove duplicate code, remove
jquery, and make these filters reusable.
Let's forget the old code, new code affects:
* issue list open/close switch
* issue list filter (label, author, assignee)
* milestone list open/close switch
* milestone issue list filter (label, author, assignee)
* project view (label, assignee)
2 months ago
initRepoPullRequestReview , initRepoIssueSidebarList , initRepoIssueFilterItemLabel ,
} from './features/repo-issue.ts' ;
import { initRepoEllipsisButton , initCommitStatuses } from './features/repo-commit.ts' ;
import { initRepoTopicBar } from './features/repo-home.ts' ;
import { initAdminCommon } from './features/admin/common.ts' ;
import { initRepoCodeView } from './features/repo-code.ts' ;
import { initSshKeyFormParser } from './features/sshkey-helper.ts' ;
import { initUserSettings } from './features/user-settings.ts' ;
import { initRepoActivityTopAuthorsChart , initRepoArchiveLinks } from './features/repo-common.ts' ;
import { initRepoMigrationStatusChecker } from './features/repo-migrate.ts' ;
import { initRepoDiffView } from './features/repo-diff.ts' ;
import { initOrgTeam } from './features/org-team.ts' ;
import { initUserAuthWebAuthn , initUserAuthWebAuthnRegister } from './features/user-auth-webauthn.ts' ;
import { initRepoRelease , initRepoReleaseNew } from './features/repo-release.ts' ;
import { initRepoEditor } from './features/repo-editor.ts' ;
import { initCompSearchUserBox } from './features/comp/SearchUserBox.ts' ;
import { initInstall } from './features/install.ts' ;
import { initCompWebHookEditor } from './features/comp/WebHookEditor.ts' ;
import { initRepoBranchButton } from './features/repo-branch.ts' ;
import { initCommonOrganization } from './features/common-organization.ts' ;
import { initRepoWikiForm } from './features/repo-wiki.ts' ;
import { initRepository , initBranchSelectorTabs } from './features/repo-legacy.ts' ;
import { initCopyContent } from './features/copycontent.ts' ;
import { initCaptcha } from './features/captcha.ts' ;
import { initRepositoryActionView } from './features/repo-actions.ts' ;
import { initGlobalTooltips } from './modules/tippy.ts' ;
import { initGiteaFomantic } from './modules/fomantic.ts' ;
import { initSubmitEventPolyfill , onDomReady } from './utils/dom.ts' ;
import { initRepoIssueList } from './features/repo-issue-list.ts' ;
import { initCommonIssueListQuickGoto } from './features/common-issue-list.ts' ;
import { initRepoContributors } from './features/contributors.ts' ;
import { initRepoCodeFrequency } from './features/code-frequency.ts' ;
import { initRepoRecentCommits } from './features/recent-commits.ts' ;
import { initRepoDiffCommitBranchesAndTags } from './features/repo-diff-commit.ts' ;
import { initDirAuto } from './modules/dirauto.ts' ;
import { initRepositorySearch } from './features/repo-search.ts' ;
import { initColorPickers } from './features/colorpicker.ts' ;
import { initAdminSelfCheck } from './features/admin/selfcheck.ts' ;
import { initOAuth2SettingsDisableCheckbox } from './features/oauth2-settings.ts' ;
import { initGlobalFetchAction } from './features/common-fetch-action.ts' ;
import { initScopedAccessTokenCategories } from './features/scoped-access-token.ts' ;
import {
initFootLanguageMenu ,
initGlobalDropdown ,
initGlobalTabularMenu ,
initHeadNavbarContentToggle ,
} from './features/common-page.ts' ;
import {
initGlobalButtonClickOnEnter ,
initGlobalButtons ,
initGlobalDeleteButton ,
} from './features/common-button.ts' ;
import {
initGlobalComboMarkdownEditor ,
initGlobalEnterQuickSubmit ,
initGlobalFormDirtyLeaveConfirm ,
} from './features/common-form.ts' ;
Fine tune more downdrop settings, use SVG for labels, improve Repo Topic Edit form (#23626)
Although it seems that some different purposes are mixed in this PR,
however, they are all related, and can be tested together, so I put them
together to save everyone's time.
Diff: `+79 −84`, everything becomes much better.
### Improve the dropdown settings.
Move all fomantic-init related code into our `fomantic.js`
Fine-tune some dropdown global settings, see the comments.
Also help to fix the first problem in #23625 , cc: @yp05327
The "language" menu has been simplified, and it works with small-height
window better.
### Use SVG instead of `<i class="delete icon">`
It's also done by `$.fn.dropdown.settings.templates.label` , cc:
@silverwind
### Remove incorrect `tabable` CSS class
It doesn't have CSS styles, and it was only in Vue. So it's totally
unnecessary, remove it by the way.
### Improve the Repo Topic Edit form
* Simplify the code
* Add a "Cancel" button
* Align elements
Before:
<details>
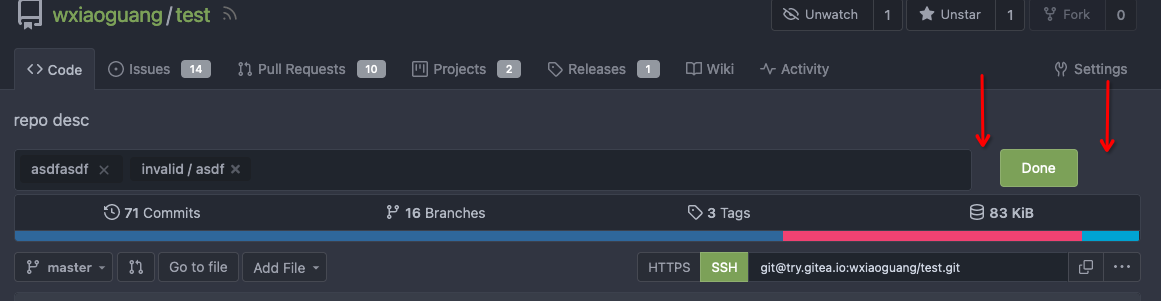
</details>
After:
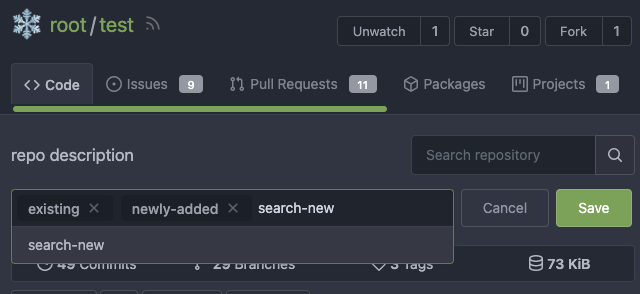
2 years ago
initGiteaFomantic ( ) ;
initDirAuto ( ) ;
initSubmitEventPolyfill ( ) ;
function callInitFunctions ( functions : ( ( ) = > any ) [ ] ) {
// Start performance trace by accessing a URL by "https://localhost/?_ui_performance_trace=1" or "https://localhost/?key=value&_ui_performance_trace=1"
// It is a quick check, no side effect so no need to do slow URL parsing.
const initStart = performance . now ( ) ;
if ( window . location . search . includes ( '_ui_performance_trace=1' ) ) {
let results : { name : string , dur : number } [ ] = [ ] ;
for ( const func of functions ) {
const start = performance . now ( ) ;
func ( ) ;
results . push ( { name : func.name , dur : performance.now ( ) - start } ) ;
}
results = results . sort ( ( a , b ) = > b . dur - a . dur ) ;
for ( let i = 0 ; i < 20 && i < results . length ; i ++ ) {
// eslint-disable-next-line no-console
console . log ( ` performance trace: ${ results [ i ] . name } ${ results [ i ] . dur . toFixed ( 3 ) } ` ) ;
}
} else {
for ( const func of functions ) {
func ( ) ;
}
}
const initDur = performance . now ( ) - initStart ;
if ( initDur > 500 ) {
console . error ( ` slow init functions took ${ initDur . toFixed ( 3 ) } ms ` ) ;
}
}
onDomReady ( ( ) = > {
callInitFunctions ( [
initGlobalDropdown ,
initGlobalTabularMenu ,
initGlobalFetchAction ,
initGlobalTooltips ,
initGlobalButtonClickOnEnter ,
initGlobalButtons ,
initGlobalCopyToClipboardListener ,
initGlobalEnterQuickSubmit ,
initGlobalFormDirtyLeaveConfirm ,
initGlobalComboMarkdownEditor ,
initGlobalDeleteButton ,
initCommonOrganization ,
initCommonIssueListQuickGoto ,
initCompSearchUserBox ,
initCompWebHookEditor ,
initInstall ,
initHeadNavbarContentToggle ,
initFootLanguageMenu ,
initCommentContent ,
initContextPopups ,
initHeatmap ,
initImageDiff ,
initMarkupAnchors ,
initMarkupContent ,
initSshKeyFormParser ,
initStopwatch ,
initTableSort ,
initAutoFocusEnd ,
initFindFileInRepo ,
initCopyContent ,
initAdminCommon ,
initAdminUserListSearchForm ,
initAdminConfigs ,
initAdminSelfCheck ,
initDashboardRepoList ,
initNotificationCount ,
initNotificationsTable ,
initOrgTeam ,
initRepoActivityTopAuthorsChart ,
initRepoArchiveLinks ,
initRepoBranchButton ,
initRepoCodeView ,
Actions support workflow dispatch event (#28163)
fix #23668
My plan:
* In the `actions.list` method, if workflow is selected and IsAdmin,
check whether the on event contains `workflow_dispatch`. If so, display
a `Run workflow` button to allow the user to manually trigger the run.
* Providing a form that allows users to select target brach or tag, and
these parameters can be configured in yaml
* Simple form validation, `required` input cannot be empty
* Add a route `/actions/run`, and an `actions.Run` method to handle
* Add `WorkflowDispatchPayload` struct to pass the Webhook event payload
to the runner when triggered, this payload carries the `inputs` values
and other fields, doc: [workflow_dispatch
payload](https://docs.github.com/en/webhooks/webhook-events-and-payloads#workflow_dispatch)
Other PRs
* the `Workflow.WorkflowDispatchConfig()` method still return non-nil
when workflow_dispatch is not defined. I submitted a PR
https://gitea.com/gitea/act/pulls/85 to fix it. Still waiting for them
to process.
Behavior should be same with github, but may cause confusion. Here's a
quick reminder.
*
[Doc](https://docs.github.com/en/actions/using-workflows/events-that-trigger-workflows#workflow_dispatch)
Said: This event will `only` trigger a workflow run if the workflow file
is `on the default branch`.
* If the workflow yaml file only exists in a non-default branch, it
cannot be triggered. (It will not even show up in the workflow list)
* If the same workflow yaml file exists in each branch at the same time,
the version of the default branch is used. Even if `Use workflow from`
selects another branch
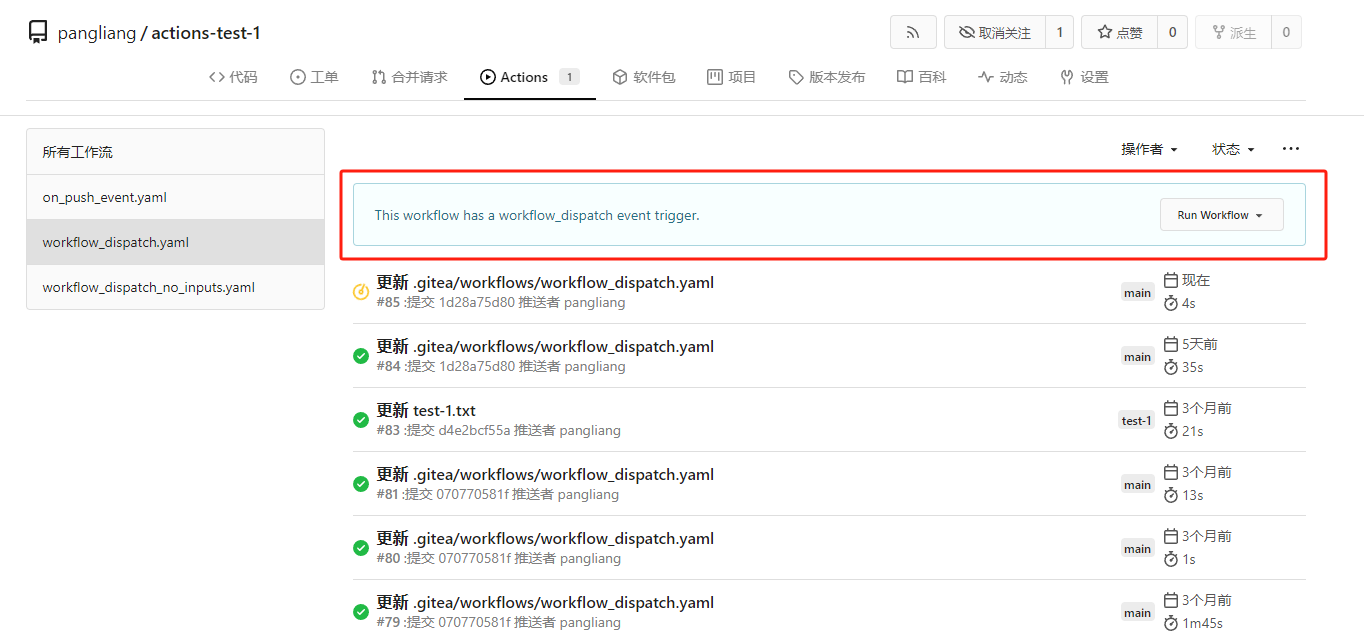
```yaml
name: Docker Image CI
on:
workflow_dispatch:
inputs:
logLevel:
description: 'Log level'
required: true
default: 'warning'
type: choice
options:
- info
- warning
- debug
tags:
description: 'Test scenario tags'
required: false
type: boolean
boolean_default_true:
description: 'Test scenario tags'
required: true
type: boolean
default: true
boolean_default_false:
description: 'Test scenario tags'
required: false
type: boolean
default: false
environment:
description: 'Environment to run tests against'
type: environment
required: true
default: 'environment values'
number_required_1:
description: 'number '
type: number
required: true
default: '100'
number_required_2:
description: 'number'
type: number
required: true
default: '100'
number_required_3:
description: 'number'
type: number
required: true
default: '100'
number_1:
description: 'number'
type: number
required: false
number_2:
description: 'number'
type: number
required: false
number_3:
description: 'number'
type: number
required: false
env:
inputs_logLevel: ${{ inputs.logLevel }}
inputs_tags: ${{ inputs.tags }}
inputs_boolean_default_true: ${{ inputs.boolean_default_true }}
inputs_boolean_default_false: ${{ inputs.boolean_default_false }}
inputs_environment: ${{ inputs.environment }}
inputs_number_1: ${{ inputs.number_1 }}
inputs_number_2: ${{ inputs.number_2 }}
inputs_number_3: ${{ inputs.number_3 }}
inputs_number_required_1: ${{ inputs.number_required_1 }}
inputs_number_required_2: ${{ inputs.number_required_2 }}
inputs_number_required_3: ${{ inputs.number_required_3 }}
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- run: ls -la
- run: env | grep inputs
- run: echo ${{ inputs.logLevel }}
- run: echo ${{ inputs.boolean_default_false }}
```

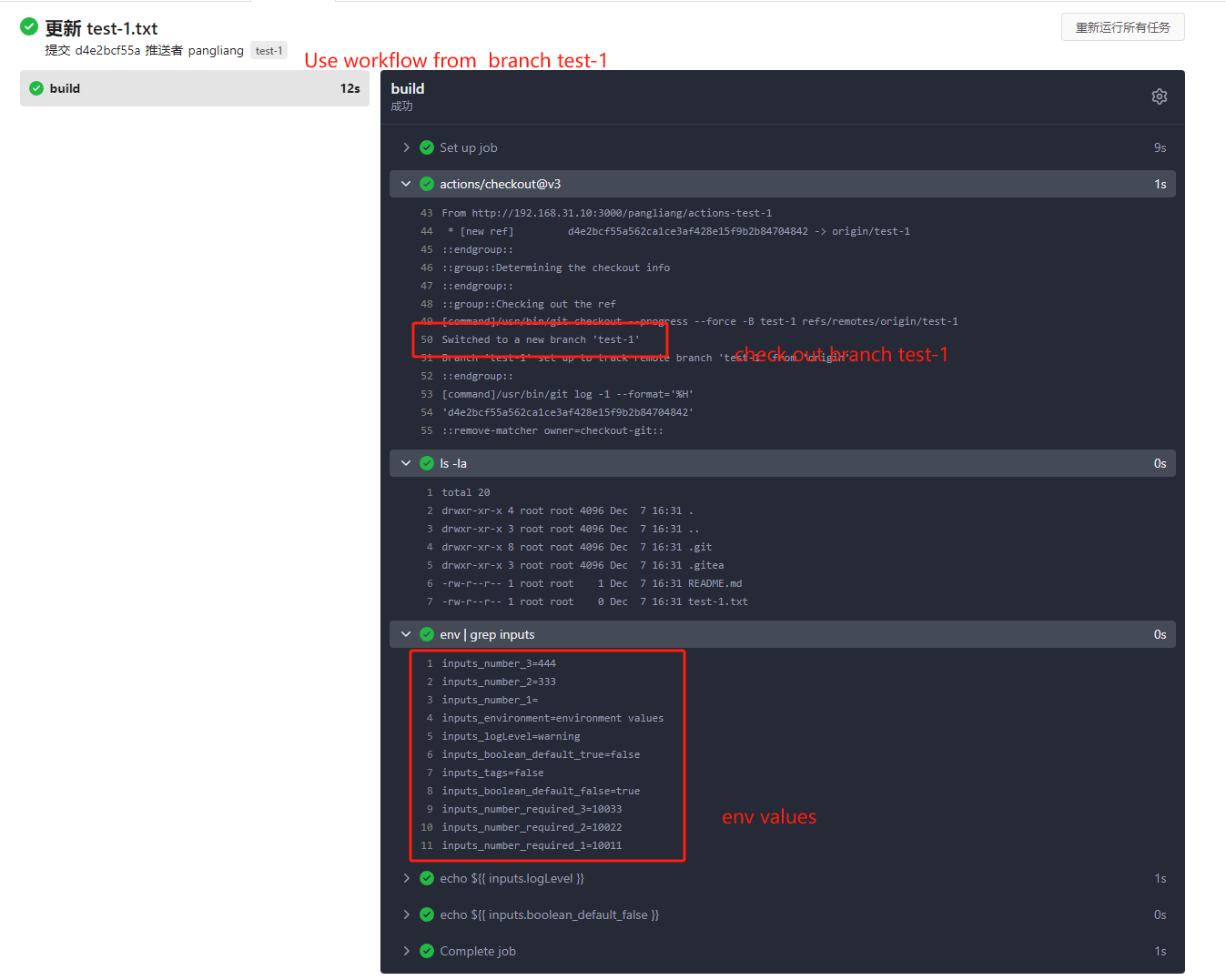
---------
Co-authored-by: TKaxv_7S <954067342@qq.com>
Co-authored-by: silverwind <me@silverwind.io>
Co-authored-by: Denys Konovalov <kontakt@denyskon.de>
Co-authored-by: Lunny Xiao <xiaolunwen@gmail.com>
6 months ago
initBranchSelectorTabs ,
initRepoEllipsisButton ,
initRepoDiffCommitBranchesAndTags ,
initRepoEditor ,
initRepoGraphGit ,
initRepoIssueContentHistory ,
initRepoIssueList ,
Refactor issue filter (labels, poster, assignee) (#32771)
Rewrite a lot of legacy strange code, remove duplicate code, remove
jquery, and make these filters reusable.
Let's forget the old code, new code affects:
* issue list open/close switch
* issue list filter (label, author, assignee)
* milestone list open/close switch
* milestone issue list filter (label, author, assignee)
* project view (label, assignee)
2 months ago
initRepoIssueFilterItemLabel ,
initRepoIssueSidebarList ,
initRepoIssueReferenceRepositorySearch ,
initRepoIssueWipTitle ,
initRepoMigration ,
initRepoMigrationStatusChecker ,
initRepoProject ,
initRepoPullRequestMergeInstruction ,
initRepoPullRequestAllowMaintainerEdit ,
initRepoPullRequestReview ,
initRepoRelease ,
initRepoReleaseNew ,
initRepoTopicBar ,
initRepoWikiForm ,
initRepository ,
initRepositoryActionView ,
initRepositorySearch ,
initRepoContributors ,
initRepoCodeFrequency ,
initRepoRecentCommits ,
initCommitStatuses ,
initCaptcha ,
initUserCheckAppUrl ,
initUserAuthOauth2 ,
initUserAuthWebAuthn ,
initUserAuthWebAuthnRegister ,
initUserSettings ,
initRepoDiffView ,
initPdfViewer ,
initScopedAccessTokenCategories ,
initColorPickers ,
initOAuth2SettingsDisableCheckbox ,
] ) ;
} ) ;