mirror of https://github.com/go-gitea/gitea
Show friendly 500 error page to users and developers (#24110)
Close #24104 This also introduces many tests to cover many complex error handling functions. ### Before The details are never shown in production. <details> 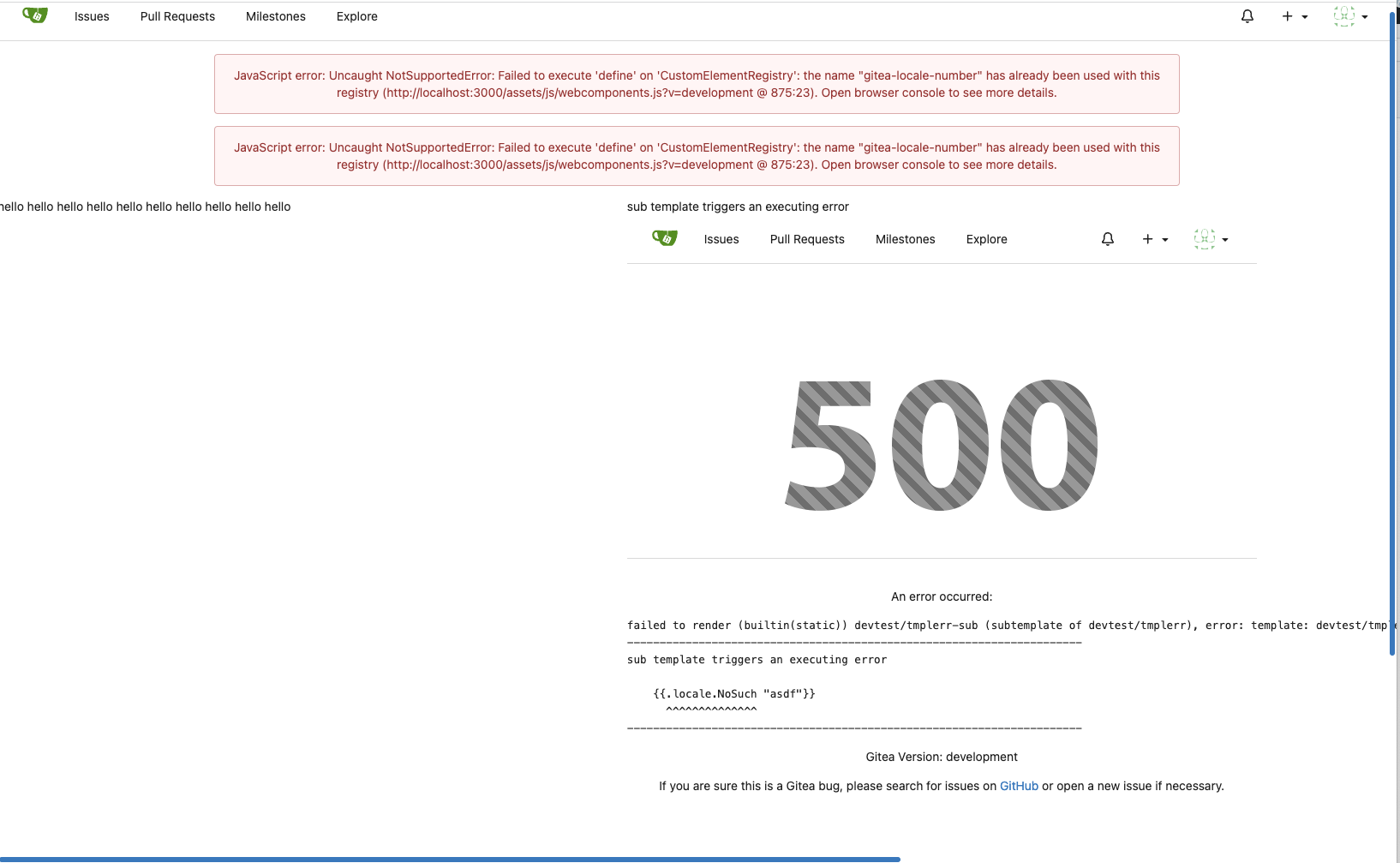 </details> ### After The details could be shown to site admin users. It is safe. 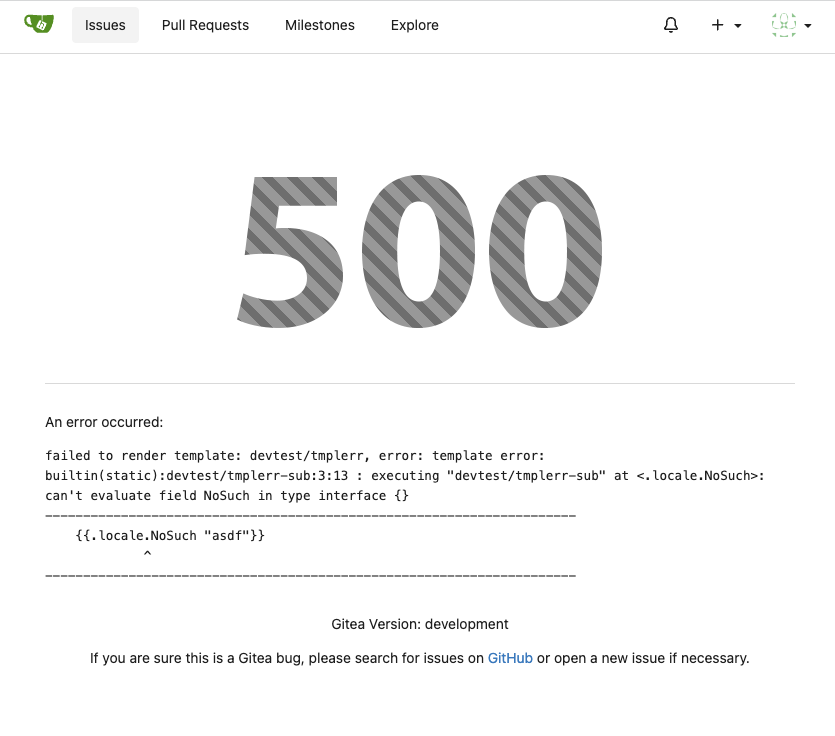pull/24109/head
parent
5768bafeb2
commit
1c8bc4081a
@ -0,0 +1,106 @@ |
||||
// Copyright 2023 The Gitea Authors. All rights reserved.
|
||||
// SPDX-License-Identifier: MIT
|
||||
|
||||
package templates |
||||
|
||||
import ( |
||||
"errors" |
||||
"html/template" |
||||
"os" |
||||
"strings" |
||||
"testing" |
||||
|
||||
"code.gitea.io/gitea/modules/assetfs" |
||||
|
||||
"github.com/stretchr/testify/assert" |
||||
) |
||||
|
||||
func TestExtractErrorLine(t *testing.T) { |
||||
cases := []struct { |
||||
code string |
||||
line int |
||||
pos int |
||||
target string |
||||
expect string |
||||
}{ |
||||
{"hello world\nfoo bar foo bar\ntest", 2, -1, "bar", ` |
||||
foo bar foo bar |
||||
^^^ ^^^ |
||||
`}, |
||||
|
||||
{"hello world\nfoo bar foo bar\ntest", 2, 4, "bar", ` |
||||
foo bar foo bar |
||||
^ |
||||
`}, |
||||
|
||||
{ |
||||
"hello world\nfoo bar foo bar\ntest", 2, 4, "", |
||||
` |
||||
foo bar foo bar |
||||
^ |
||||
`, |
||||
}, |
||||
|
||||
{ |
||||
"hello world\nfoo bar foo bar\ntest", 5, 0, "", |
||||
`unable to find target line 5`, |
||||
}, |
||||
} |
||||
|
||||
for _, c := range cases { |
||||
actual := extractErrorLine([]byte(c.code), c.line, c.pos, c.target) |
||||
assert.Equal(t, strings.TrimSpace(c.expect), strings.TrimSpace(actual)) |
||||
} |
||||
} |
||||
|
||||
func TestHandleError(t *testing.T) { |
||||
dir := t.TempDir() |
||||
|
||||
p := &templateErrorPrettier{assets: assetfs.Layered(assetfs.Local("tmp", dir))} |
||||
|
||||
test := func(s string, h func(error) string, expect string) { |
||||
err := os.WriteFile(dir+"/test.tmpl", []byte(s), 0o644) |
||||
assert.NoError(t, err) |
||||
tmpl := template.New("test") |
||||
_, err = tmpl.Parse(s) |
||||
assert.Error(t, err) |
||||
msg := h(err) |
||||
assert.EqualValues(t, strings.TrimSpace(expect), strings.TrimSpace(msg)) |
||||
} |
||||
|
||||
test("{{", p.handleGenericTemplateError, ` |
||||
template error: tmp:test:1 : unclosed action |
||||
---------------------------------------------------------------------- |
||||
{{ |
||||
---------------------------------------------------------------------- |
||||
`) |
||||
|
||||
test("{{Func}}", p.handleFuncNotDefinedError, ` |
||||
template error: tmp:test:1 : function "Func" not defined |
||||
---------------------------------------------------------------------- |
||||
{{Func}} |
||||
^^^^ |
||||
---------------------------------------------------------------------- |
||||
`) |
||||
|
||||
test("{{'x'3}}", p.handleUnexpectedOperandError, ` |
||||
template error: tmp:test:1 : unexpected "3" in operand |
||||
---------------------------------------------------------------------- |
||||
{{'x'3}} |
||||
^ |
||||
---------------------------------------------------------------------- |
||||
`) |
||||
|
||||
// no idea about how to trigger such strange error, so mock an error to test it
|
||||
err := os.WriteFile(dir+"/test.tmpl", []byte("god knows XXX"), 0o644) |
||||
assert.NoError(t, err) |
||||
expectedMsg := ` |
||||
template error: tmp:test:1 : expected end; found XXX |
||||
---------------------------------------------------------------------- |
||||
god knows XXX |
||||
^^^ |
||||
---------------------------------------------------------------------- |
||||
` |
||||
actualMsg := p.handleExpectedEndError(errors.New("template: test:1: expected end; found XXX")) |
||||
assert.EqualValues(t, strings.TrimSpace(expectedMsg), strings.TrimSpace(actualMsg)) |
||||
} |
@ -0,0 +1,3 @@ |
||||
sub template triggers an executing error |
||||
|
||||
{{.locale.NoSuch "asdf"}} |
@ -0,0 +1,12 @@ |
||||
{{template "base/head" .}} |
||||
<div class="page-content devtest"> |
||||
<div class="gt-df"> |
||||
<div style="width: 80%; "> |
||||
hello hello hello hello hello hello hello hello hello hello |
||||
</div> |
||||
<div style="width: 20%;"> |
||||
{{template "devtest/tmplerr-sub" .}} |
||||
</div> |
||||
</div> |
||||
</div> |
||||
{{template "base/footer" .}} |
@ -1,13 +1,36 @@ |
||||
{{template "base/head" .}} |
||||
<div role="main" aria-label="{{.Title}}" class="page-content ui container gt-full-screen-width center"> |
||||
<p style="margin-top: 100px"><img src="{{AssetUrlPrefix}}/img/500.png" alt="500"></p> |
||||
<div role="main" aria-label="{{.Title}}" class="page-content gt-w-screen status-page-500"> |
||||
<p class="gt-mt-5 center"><img src="{{AssetUrlPrefix}}/img/500.png" alt="Internal Server Error"></p> |
||||
<div class="ui divider"></div> |
||||
<br> |
||||
{{if .ErrorMsg}} |
||||
<p>{{.locale.Tr "error.occurred"}}:</p> |
||||
<pre style="text-align: left">{{.ErrorMsg}}</pre> |
||||
{{end}} |
||||
{{if .ShowFooterVersion}}<p>{{.locale.Tr "admin.config.app_ver"}}: {{AppVer}}</p>{{end}} |
||||
{{if .IsAdmin}}<p>{{.locale.Tr "error.report_message" | Safe}}</p>{{end}} |
||||
|
||||
<div class="ui container gt-mt-5"> |
||||
{{if .ErrorMsg}} |
||||
<p>{{.locale.Tr "error.occurred"}}:</p> |
||||
<pre class="gt-whitespace-pre-wrap">{{.ErrorMsg}}</pre> |
||||
{{end}} |
||||
|
||||
<div class="center gt-mt-5"> |
||||
{{if .ShowFooterVersion}}<p>{{.locale.Tr "admin.config.app_ver"}}: {{AppVer}}</p>{{end}} |
||||
{{if .IsAdmin}}<p>{{.locale.Tr "error.report_message" | Safe}}</p>{{end}} |
||||
</div> |
||||
</div> |
||||
</div> |
||||
{{/* when a sub-template triggers an 500 error, its parent template has been partially rendered, |
||||
then the 500 page will be rendered after that partially rendered page, the HTML/JS are totally broken. |
||||
so use this inline script to try to move it to main viewport */}} |
||||
<script type="module"> |
||||
const embedded = document.querySelector('.page-content .page-content.status-page-500'); |
||||
if (embedded) { |
||||
// move footer to main view |
||||
const footer = document.querySelector('footer'); |
||||
if (footer) document.querySelector('body').append(footer); |
||||
// move the 500 error page content to main view |
||||
const embeddedParent = embedded.parentNode; |
||||
let main = document.querySelector('.page-content'); |
||||
main = main ?? document.querySelector('body'); |
||||
main.prepend(document.createElement('hr')); |
||||
main.prepend(embedded); |
||||
embeddedParent.remove(); // remove the unrelated 500-page elements (eg: the duplicate nav bar) |
||||
} |
||||
</script> |
||||
{{template "base/footer" .}} |
||||
|
Loading…
Reference in new issue