mirror of https://github.com/go-gitea/gitea
Improve queue & process & stacktrace (#24636)
Although some features are mixed together in this PR, this PR is not that large, and these features are all related. Actually there are more than 70 lines are for a toy "test queue", so this PR is quite simple. Major features: 1. Allow site admin to clear a queue (remove all items in a queue) * Because there is no transaction, the "unique queue" could be corrupted in rare cases, that's unfixable. * eg: the item is in the "set" but not in the "list", so the item would never be able to be pushed into the queue. * Now site admin could simply clear the queue, then everything becomes correct, the lost items could be re-pushed into queue by future operations. 3. Split the "admin/monitor" to separate pages 4. Allow to download diagnosis report * In history, there were many users reporting that Gitea queue gets stuck, or Gitea's CPU is 100% * With diagnosis report, maintainers could know what happens clearly The diagnosis report sample: [gitea-diagnosis-20230510-192913.zip](https://github.com/go-gitea/gitea/files/11441346/gitea-diagnosis-20230510-192913.zip) , use "go tool pprof profile.dat" to view the report. Screenshots:  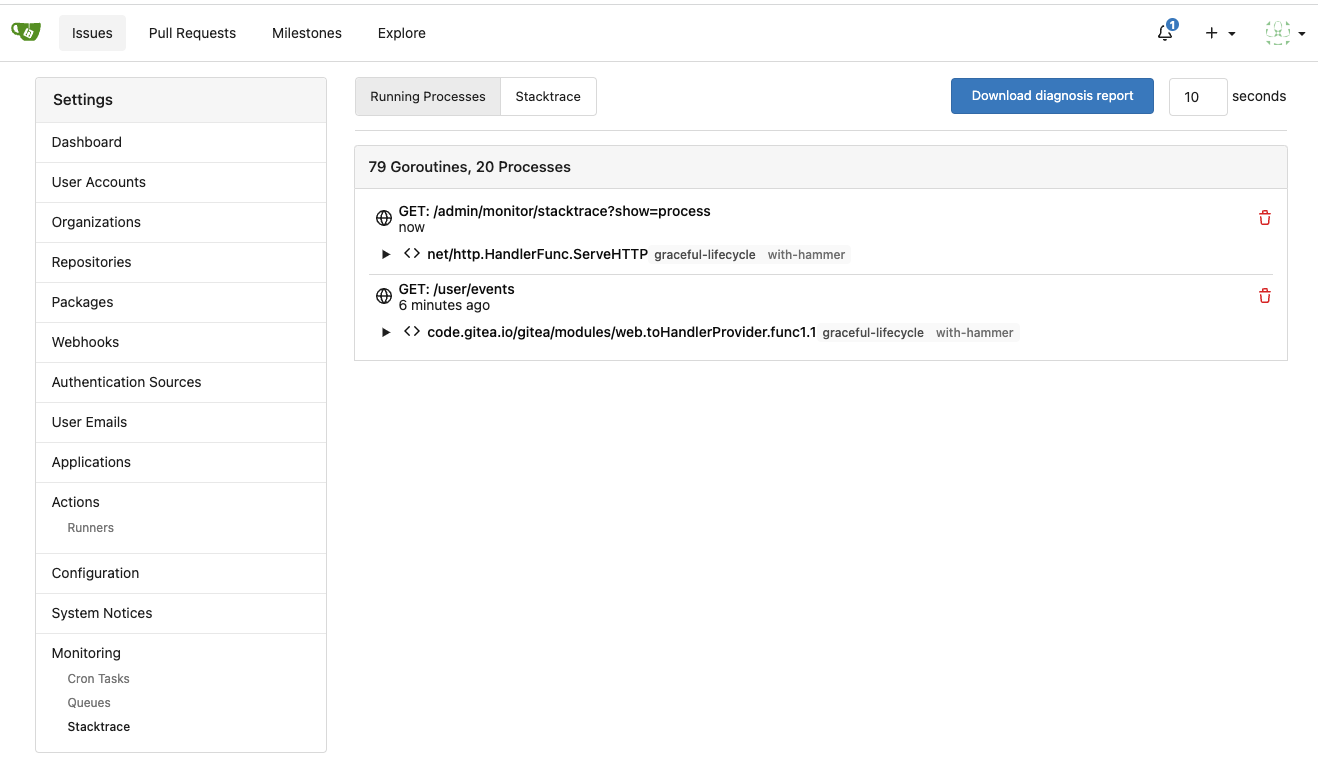  --------- Co-authored-by: Jason Song <i@wolfogre.com> Co-authored-by: Giteabot <teabot@gitea.io>pull/24605/head^2
parent
b3af7484bc
commit
58dfaf3a75
@ -0,0 +1,61 @@ |
||||
// Copyright 2023 The Gitea Authors.
|
||||
// SPDX-License-Identifier: MIT
|
||||
|
||||
package admin |
||||
|
||||
import ( |
||||
"archive/zip" |
||||
"fmt" |
||||
"runtime/pprof" |
||||
"time" |
||||
|
||||
"code.gitea.io/gitea/modules/context" |
||||
"code.gitea.io/gitea/modules/httplib" |
||||
) |
||||
|
||||
func MonitorDiagnosis(ctx *context.Context) { |
||||
seconds := ctx.FormInt64("seconds") |
||||
if seconds <= 5 { |
||||
seconds = 5 |
||||
} |
||||
if seconds > 300 { |
||||
seconds = 300 |
||||
} |
||||
|
||||
httplib.ServeSetHeaders(ctx.Resp, &httplib.ServeHeaderOptions{ |
||||
ContentType: "application/zip", |
||||
Disposition: "attachment", |
||||
Filename: fmt.Sprintf("gitea-diagnosis-%s.zip", time.Now().Format("20060102-150405")), |
||||
}) |
||||
|
||||
zipWriter := zip.NewWriter(ctx.Resp) |
||||
defer zipWriter.Close() |
||||
|
||||
f, err := zipWriter.CreateHeader(&zip.FileHeader{Name: "goroutine-before.txt", Method: zip.Deflate, Modified: time.Now()}) |
||||
if err != nil { |
||||
ctx.ServerError("Failed to create zip file", err) |
||||
return |
||||
} |
||||
_ = pprof.Lookup("goroutine").WriteTo(f, 1) |
||||
|
||||
f, err = zipWriter.CreateHeader(&zip.FileHeader{Name: "cpu-profile.dat", Method: zip.Deflate, Modified: time.Now()}) |
||||
if err != nil { |
||||
ctx.ServerError("Failed to create zip file", err) |
||||
return |
||||
} |
||||
|
||||
err = pprof.StartCPUProfile(f) |
||||
if err == nil { |
||||
time.Sleep(time.Duration(seconds) * time.Second) |
||||
pprof.StopCPUProfile() |
||||
} else { |
||||
_, _ = f.Write([]byte(err.Error())) |
||||
} |
||||
|
||||
f, err = zipWriter.CreateHeader(&zip.FileHeader{Name: "goroutine-after.txt", Method: zip.Deflate, Modified: time.Now()}) |
||||
if err != nil { |
||||
ctx.ServerError("Failed to create zip file", err) |
||||
return |
||||
} |
||||
_ = pprof.Lookup("goroutine").WriteTo(f, 1) |
||||
} |
@ -0,0 +1,72 @@ |
||||
// Copyright 2023 The Gitea Authors. All rights reserved.
|
||||
// SPDX-License-Identifier: MIT
|
||||
|
||||
package admin |
||||
|
||||
import ( |
||||
gocontext "context" |
||||
"sync" |
||||
"time" |
||||
|
||||
"code.gitea.io/gitea/modules/graceful" |
||||
"code.gitea.io/gitea/modules/log" |
||||
"code.gitea.io/gitea/modules/queue" |
||||
"code.gitea.io/gitea/modules/setting" |
||||
) |
||||
|
||||
var testQueueOnce sync.Once |
||||
|
||||
// initTestQueueOnce initializes the test queue for dev mode
|
||||
// the test queue will also be shown in the queue list
|
||||
// developers could see the queue length / worker number / items number on the admin page and try to remove the items
|
||||
func initTestQueueOnce() { |
||||
testQueueOnce.Do(func() { |
||||
qs := setting.QueueSettings{ |
||||
Name: "test-queue", |
||||
Type: "channel", |
||||
Length: 20, |
||||
BatchLength: 2, |
||||
MaxWorkers: 3, |
||||
} |
||||
testQueue, err := queue.NewWorkerPoolQueueBySetting("test-queue", qs, func(t ...int64) (unhandled []int64) { |
||||
for range t { |
||||
select { |
||||
case <-graceful.GetManager().ShutdownContext().Done(): |
||||
case <-time.After(5 * time.Second): |
||||
} |
||||
} |
||||
return nil |
||||
}, true) |
||||
if err != nil { |
||||
log.Error("unable to create test queue: %v", err) |
||||
return |
||||
} |
||||
|
||||
queue.GetManager().AddManagedQueue(testQueue) |
||||
testQueue.SetWorkerMaxNumber(5) |
||||
go graceful.GetManager().RunWithShutdownFns(testQueue.Run) |
||||
go graceful.GetManager().RunWithShutdownContext(func(ctx gocontext.Context) { |
||||
cnt := int64(0) |
||||
adding := true |
||||
for { |
||||
select { |
||||
case <-ctx.Done(): |
||||
case <-time.After(500 * time.Millisecond): |
||||
if adding { |
||||
if testQueue.GetQueueItemNumber() == qs.Length { |
||||
adding = false |
||||
} |
||||
} else { |
||||
if testQueue.GetQueueItemNumber() == 0 { |
||||
adding = true |
||||
} |
||||
} |
||||
if adding { |
||||
_ = testQueue.Push(cnt) |
||||
cnt++ |
||||
} |
||||
} |
||||
} |
||||
}) |
||||
}) |
||||
} |
@ -0,0 +1,48 @@ |
||||
// Copyright 2023 The Gitea Authors. All rights reserved.
|
||||
// SPDX-License-Identifier: MIT
|
||||
|
||||
package admin |
||||
|
||||
import ( |
||||
"net/http" |
||||
"runtime" |
||||
|
||||
"code.gitea.io/gitea/modules/context" |
||||
"code.gitea.io/gitea/modules/process" |
||||
"code.gitea.io/gitea/modules/setting" |
||||
) |
||||
|
||||
// Stacktrace show admin monitor goroutines page
|
||||
func Stacktrace(ctx *context.Context) { |
||||
ctx.Data["Title"] = ctx.Tr("admin.monitor") |
||||
ctx.Data["PageIsAdminMonitorStacktrace"] = true |
||||
|
||||
ctx.Data["GoroutineCount"] = runtime.NumGoroutine() |
||||
|
||||
show := ctx.FormString("show") |
||||
ctx.Data["ShowGoroutineList"] = show |
||||
// by default, do not do anything which might cause server errors, to avoid unnecessary 500 pages.
|
||||
// this page is the entrance of the chance to collect diagnosis report.
|
||||
if show != "" { |
||||
showNoSystem := show == "process" |
||||
processStacks, processCount, _, err := process.GetManager().ProcessStacktraces(false, showNoSystem) |
||||
if err != nil { |
||||
ctx.ServerError("GoroutineStacktrace", err) |
||||
return |
||||
} |
||||
|
||||
ctx.Data["ProcessStacks"] = processStacks |
||||
ctx.Data["ProcessCount"] = processCount |
||||
} |
||||
|
||||
ctx.HTML(http.StatusOK, tplStacktrace) |
||||
} |
||||
|
||||
// StacktraceCancel cancels a process
|
||||
func StacktraceCancel(ctx *context.Context) { |
||||
pid := ctx.Params("pid") |
||||
process.GetManager().Cancel(process.IDType(pid)) |
||||
ctx.JSON(http.StatusOK, map[string]interface{}{ |
||||
"redirect": setting.AppSubURL + "/admin/monitor/stacktrace", |
||||
}) |
||||
} |
@ -1,35 +1,39 @@ |
||||
<h4 class="ui top attached header"> |
||||
{{.locale.Tr "admin.monitor.cron"}} |
||||
</h4> |
||||
<div class="ui attached table segment"> |
||||
<form method="post" action="{{AppSubUrl}}/admin"> |
||||
<table class="ui very basic striped table unstackable"> |
||||
<thead> |
||||
<tr> |
||||
<th></th> |
||||
<th>{{.locale.Tr "admin.monitor.name"}}</th> |
||||
<th>{{.locale.Tr "admin.monitor.schedule"}}</th> |
||||
<th>{{.locale.Tr "admin.monitor.next"}}</th> |
||||
<th>{{.locale.Tr "admin.monitor.previous"}}</th> |
||||
<th>{{.locale.Tr "admin.monitor.execute_times"}}</th> |
||||
<th>{{.locale.Tr "admin.monitor.last_execution_result"}}</th> |
||||
</tr> |
||||
</thead> |
||||
<tbody> |
||||
{{range .Entries}} |
||||
{{template "admin/layout_head" (dict "ctxData" . "pageClass" "admin monitor")}} |
||||
<div class="admin-setting-content"> |
||||
<h4 class="ui top attached header"> |
||||
{{.locale.Tr "admin.monitor.cron"}} |
||||
</h4> |
||||
<div class="ui attached table segment"> |
||||
<form method="post" action="{{AppSubUrl}}/admin"> |
||||
<table class="ui very basic striped table unstackable"> |
||||
<thead> |
||||
<tr> |
||||
<td><button type="submit" class="ui green button" name="op" value="{{.Name}}" title="{{$.locale.Tr "admin.dashboard.operation_run"}}">{{svg "octicon-triangle-right"}}</button></td> |
||||
<td>{{$.locale.Tr (printf "admin.dashboard.%s" .Name)}}</td> |
||||
<td>{{.Spec}}</td> |
||||
<td>{{DateTime "full" .Next}}</td> |
||||
<td>{{if gt .Prev.Year 1}}{{DateTime "full" .Prev}}{{else}}-{{end}}</td> |
||||
<td>{{.ExecTimes}}</td> |
||||
<td {{if ne .Status ""}}data-tooltip-content="{{.FormatLastMessage $.locale}}"{{end}} >{{if eq .Status ""}}—{{else if eq .Status "finished"}}{{svg "octicon-check" 16}}{{else}}{{svg "octicon-x" 16}}{{end}}</td> |
||||
<th></th> |
||||
<th>{{.locale.Tr "admin.monitor.name"}}</th> |
||||
<th>{{.locale.Tr "admin.monitor.schedule"}}</th> |
||||
<th>{{.locale.Tr "admin.monitor.next"}}</th> |
||||
<th>{{.locale.Tr "admin.monitor.previous"}}</th> |
||||
<th>{{.locale.Tr "admin.monitor.execute_times"}}</th> |
||||
<th>{{.locale.Tr "admin.monitor.last_execution_result"}}</th> |
||||
</tr> |
||||
{{end}} |
||||
</tbody> |
||||
</table> |
||||
<input type="hidden" name="from" value="monitor"> |
||||
{{.CsrfTokenHtml}} |
||||
</form> |
||||
</thead> |
||||
<tbody> |
||||
{{range .Entries}} |
||||
<tr> |
||||
<td><button type="submit" class="ui green button" name="op" value="{{.Name}}" title="{{$.locale.Tr "admin.dashboard.operation_run"}}">{{svg "octicon-triangle-right"}}</button></td> |
||||
<td>{{$.locale.Tr (printf "admin.dashboard.%s" .Name)}}</td> |
||||
<td>{{.Spec}}</td> |
||||
<td>{{DateTime "full" .Next}}</td> |
||||
<td>{{if gt .Prev.Year 1}}{{DateTime "full" .Prev}}{{else}}-{{end}}</td> |
||||
<td>{{.ExecTimes}}</td> |
||||
<td {{if ne .Status ""}}data-tooltip-content="{{.FormatLastMessage $.locale}}"{{end}} >{{if eq .Status ""}}—{{else if eq .Status "finished"}}{{svg "octicon-check" 16}}{{else}}{{svg "octicon-x" 16}}{{end}}</td> |
||||
</tr> |
||||
{{end}} |
||||
</tbody> |
||||
</table> |
||||
<input type="hidden" name="from" value="monitor"> |
||||
{{.CsrfTokenHtml}} |
||||
</form> |
||||
</div> |
||||
</div> |
||||
{{template "admin/layout_footer" .}} |
||||
|
@ -1,19 +0,0 @@ |
||||
{{template "admin/layout_head" (dict "ctxData" . "pageClass" "admin monitor")}} |
||||
<div class="admin-setting-content"> |
||||
{{template "admin/cron" .}} |
||||
{{template "admin/queue" .}} |
||||
{{template "admin/process" .}} |
||||
</div> |
||||
|
||||
<div class="ui g-modal-confirm delete modal"> |
||||
<div class="header"> |
||||
{{.locale.Tr "admin.monitor.process.cancel"}} |
||||
</div> |
||||
<div class="content"> |
||||
<p>{{$.locale.Tr "admin.monitor.process.cancel_notices" `<span class="name"></span>` | Safe}}</p> |
||||
<p>{{$.locale.Tr "admin.monitor.process.cancel_desc"}}</p> |
||||
</div> |
||||
{{template "base/modal_actions_confirm" .}} |
||||
</div> |
||||
|
||||
{{template "admin/layout_footer" .}} |
@ -1,23 +0,0 @@ |
||||
<div class="item"> |
||||
<div class="gt-df gt-ac"> |
||||
<div class="icon gt-ml-3 gt-mr-3">{{if eq .Process.Type "request"}}{{svg "octicon-globe" 16}}{{else if eq .Process.Type "system"}}{{svg "octicon-cpu" 16}}{{else}}{{svg "octicon-terminal" 16}}{{end}}</div> |
||||
<div class="content gt-f1"> |
||||
<div class="header">{{.Process.Description}}</div> |
||||
<div class="description">{{TimeSince .Process.Start .root.locale}}</div> |
||||
</div> |
||||
<div> |
||||
{{if ne .Process.Type "system"}} |
||||
<a class="delete-button icon" href="" data-url="{{.root.Link}}/cancel/{{.Process.PID}}" data-id="{{.Process.PID}}" data-name="{{.Process.Description}}">{{svg "octicon-trash" 16 "text-red"}}</a> |
||||
{{end}} |
||||
</div> |
||||
</div> |
||||
|
||||
{{$children := .Process.Children}} |
||||
{{if $children}} |
||||
<div class="divided list"> |
||||
{{range $children}} |
||||
{{template "admin/process-row" dict "Process" . "root" $.root}} |
||||
{{end}} |
||||
</div> |
||||
{{end}} |
||||
</div> |
@ -1,13 +0,0 @@ |
||||
<h4 class="ui top attached header"> |
||||
{{.locale.Tr "admin.monitor.process"}} |
||||
<div class="ui right"> |
||||
<a class="ui primary tiny button" href="{{AppSubUrl}}/admin/monitor/stacktrace">{{.locale.Tr "admin.monitor.stacktrace"}}</a> |
||||
</div> |
||||
</h4> |
||||
<div class="ui attached segment"> |
||||
<div class="ui relaxed divided list"> |
||||
{{range .Processes}} |
||||
{{template "admin/process-row" dict "Process" . "root" $}} |
||||
{{end}} |
||||
</div> |
||||
</div> |
@ -1,29 +1,34 @@ |
||||
<h4 class="ui top attached header"> |
||||
{{.locale.Tr "admin.monitor.queues"}} |
||||
</h4> |
||||
<div class="ui attached table segment"> |
||||
<table class="ui very basic striped table unstackable"> |
||||
<thead> |
||||
<tr> |
||||
<th>{{.locale.Tr "admin.monitor.queue.name"}}</th> |
||||
<th>{{.locale.Tr "admin.monitor.queue.type"}}</th> |
||||
<th>{{.locale.Tr "admin.monitor.queue.exemplar"}}</th> |
||||
<th>{{.locale.Tr "admin.monitor.queue.numberworkers"}}</th> |
||||
<th>{{.locale.Tr "admin.monitor.queue.numberinqueue"}}</th> |
||||
<th></th> |
||||
</tr> |
||||
</thead> |
||||
<tbody> |
||||
{{range $qid, $q := .Queues}} |
||||
<tr> |
||||
<td>{{$q.GetName}}</td> |
||||
<td>{{$q.GetType}}</td> |
||||
<td>{{$q.GetItemTypeName}}</td> |
||||
<td>{{$sum := $q.GetWorkerNumber}}{{if lt $sum 0}}-{{else}}{{$sum}}{{end}}</td> |
||||
<td>{{$sum = $q.GetQueueItemNumber}}{{if lt $sum 0}}-{{else}}{{$sum}}{{end}}</td> |
||||
<td><a href="{{$.Link}}/queue/{{$qid}}" class="button">{{if lt $sum 0}}{{$.locale.Tr "admin.monitor.queue.review"}}{{else}}{{$.locale.Tr "admin.monitor.queue.review_add"}}{{end}}</a> |
||||
</tr> |
||||
{{end}} |
||||
</tbody> |
||||
</table> |
||||
{{template "admin/layout_head" (dict "ctxData" . "pageClass" "admin monitor")}} |
||||
<div class="admin-setting-content"> |
||||
<h4 class="ui top attached header"> |
||||
{{.locale.Tr "admin.monitor.queues"}} |
||||
</h4> |
||||
<div class="ui attached table segment"> |
||||
<table class="ui very basic striped table unstackable"> |
||||
<thead> |
||||
<tr> |
||||
<th>{{.locale.Tr "admin.monitor.queue.name"}}</th> |
||||
<th>{{.locale.Tr "admin.monitor.queue.type"}}</th> |
||||
<th>{{.locale.Tr "admin.monitor.queue.exemplar"}}</th> |
||||
<th>{{.locale.Tr "admin.monitor.queue.numberworkers"}}</th> |
||||
<th>{{.locale.Tr "admin.monitor.queue.numberinqueue"}}</th> |
||||
<th></th> |
||||
</tr> |
||||
</thead> |
||||
<tbody> |
||||
{{range $qid, $q := .Queues}} |
||||
<tr> |
||||
<td>{{$q.GetName}}</td> |
||||
<td>{{$q.GetType}}</td> |
||||
<td>{{$q.GetItemTypeName}}</td> |
||||
<td>{{$sum := $q.GetWorkerNumber}}{{if lt $sum 0}}-{{else}}{{$sum}}{{end}}</td> |
||||
<td>{{$sum = $q.GetQueueItemNumber}}{{if lt $sum 0}}-{{else}}{{$sum}}{{end}}</td> |
||||
<td><a href="{{$.Link}}/{{$qid}}" class="button">{{if lt $sum 0}}{{$.locale.Tr "admin.monitor.queue.review"}}{{else}}{{$.locale.Tr "admin.monitor.queue.review_add"}}{{end}}</a> |
||||
</tr> |
||||
{{end}} |
||||
</tbody> |
||||
</table> |
||||
</div> |
||||
</div> |
||||
{{template "admin/layout_footer" .}} |
||||
|
||||
|
Loading…
Reference in new issue