mirror of https://github.com/ethereum/go-ethereum
parent
6b24a1e50b
commit
8285cccc7c
@ -1,180 +0,0 @@ |
||||
--- |
||||
title: Mobile API |
||||
sort_key: F |
||||
--- |
||||
|
||||
The Ethereum blockchain along with its two extension protocols Whisper and Swarm was |
||||
originally conceptualized to become the supporting pillar of web3, providing the |
||||
consensus, messaging and storage backbone for a new generation of distributed (actually, |
||||
decentralized) applications called DApps. |
||||
|
||||
The first incarnation towards this dream of web3 was a command line client providing an |
||||
RPC interface into the peer-to-peer protocols. The client was soon enough extended with a |
||||
web-browser-like graphical user interface, permitting developers to write DApps based on |
||||
the tried and proven HTML/CSS/JS technologies. |
||||
|
||||
As many DApps have more complex requirements than what a browser environment can handle, |
||||
it became apparent that providing programmatic access to the web3 pillars would open the |
||||
door towards a new class of applications. As such, the second incarnation of the web |
||||
dream is to open up all our technologies for other projects as reusable components. |
||||
|
||||
Starting with the 1.5 release family of `go-ethereum`, we transitioned away from providing |
||||
only a full blown Ethereum client and started shipping official Go packages that could be |
||||
embedded into third party desktop and server applications. It took only a small leap from |
||||
here to begin porting our code to mobile platforms. |
||||
|
||||
## Quick overview |
||||
|
||||
Similarly to our reusable Go libraries, the mobile wrappers also focus on four main usage |
||||
areas: |
||||
|
||||
- Simplified client side account management |
||||
- Remote node interfacing via different transports |
||||
- Contract interactions through auto-generated bindings |
||||
- In-process Ethereum, Whisper and Swarm peer-to-peer node |
||||
|
||||
You can watch a quick overview about these in Peter's (@karalabe) talk titled "Import |
||||
Geth: Ethereum from Go and beyond", presented at the Ethereum Devcon2 developer conference |
||||
in September, 2016 (Shanghai). Slides are [available |
||||
here](https://ethereum.karalabe.com/talks/2016-devcon.html). |
||||
|
||||
[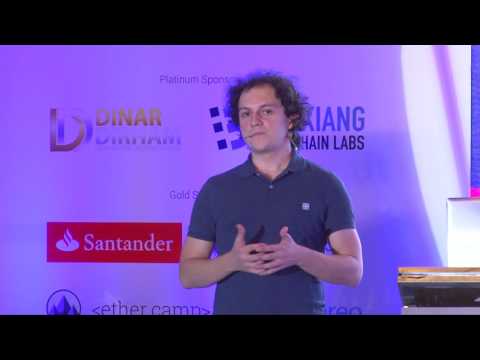](https://www.youtube.com/watch?v=R0Ia1U9Gxjg) |
||||
|
||||
## Library bundles |
||||
|
||||
The `go-ethereum` mobile library is distributed either as an Android `.aar` archive |
||||
(containing binaries for `arm-7`, `arm64`, `x86` and `x64`); or as an iOS XCode framework |
||||
(containing binaries for `arm-7`, `arm64` and `x86`). We do not provide library bundles |
||||
for Windows phone the moment. |
||||
|
||||
### Android archive |
||||
|
||||
The simplest way to use `go-ethereum` in your Android project is through a Maven |
||||
dependency. We provide bundles of all our stable releases (starting from v1.5.0) through |
||||
Maven Central, and also provide the latest develop bundle through the Sonatype OSS |
||||
repository. |
||||
|
||||
#### Stable dependency (Maven Central) |
||||
|
||||
To add an Android dependency to the **stable** library release of `go-ethereum`, you'll |
||||
need to ensure that the Maven Central repository is enabled in your Android project, and |
||||
that the `go-ethereum` code is listed as a required dependency of your application. You |
||||
can do both of these by editing the `build.gradle` script in your Android app's folder: |
||||
|
||||
```gradle |
||||
repositories { |
||||
mavenCentral() |
||||
} |
||||
|
||||
dependencies { |
||||
// All your previous dependencies |
||||
compile 'org.ethereum:geth:1.5.2' // Change the version to the latest release |
||||
} |
||||
``` |
||||
|
||||
#### Develop dependency (Sonatype) |
||||
|
||||
To add an Android dependency to the current version of `go-ethereum`, you'll need to |
||||
ensure that the Sonatype snapshot repository is enabled in your Android project, and that |
||||
the `go-ethereum` code is listed as a required `SNAPSHOT` dependency of your application. |
||||
You can do both of these by editing the `build.gradle` script in your Android app's |
||||
folder: |
||||
|
||||
```gradle |
||||
repositories { |
||||
maven { |
||||
url "https://oss.sonatype.org/content/groups/public" |
||||
} |
||||
} |
||||
|
||||
dependencies { |
||||
// All your previous dependencies |
||||
compile 'org.ethereum:geth:1.5.3-SNAPSHOT' // Change the version to the latest release |
||||
} |
||||
``` |
||||
|
||||
#### Custom dependency |
||||
|
||||
If you prefer not to depend on Maven Central or Sonatype; or would like to access an older |
||||
develop build not available any more as an online dependency, you can download any bundle |
||||
directly from [our website](https://geth.ethereum.org/downloads/) and insert it into your |
||||
project in Android Studio via `File -> New -> New module... -> Import .JAR/.AAR Package`. |
||||
|
||||
You will also need to configure `gradle` to link the mobile library bundle to your |
||||
application. This can be done by adding a new entry to the `dependencies` section of your |
||||
`build.gradle` script, pointing it to the module you just added (named `geth` by default). |
||||
|
||||
```gradle |
||||
dependencies { |
||||
// All your previous dependencies |
||||
compile project(':geth') |
||||
} |
||||
``` |
||||
|
||||
#### Manual builds |
||||
|
||||
Lastly, if you would like to make modifications to the `go-ethereum` mobile code and/or |
||||
build it yourself locally instead of downloading a pre-built bundle, you can do so using a |
||||
`make` command. This will create an Android archive called `geth.aar` in the `build/bin` |
||||
folder that you can import into your Android Studio as described above. |
||||
|
||||
```bash |
||||
$ make android |
||||
[...] |
||||
Done building. |
||||
Import "build/bin/geth.aar" to use the library. |
||||
``` |
||||
|
||||
### iOS framework |
||||
|
||||
The simplest way to use `go-ethereum` in your iOS project is through a |
||||
[CocoaPods](https://cocoapods.org/) dependency. We provide bundles of all our stable |
||||
releases (starting from v1.5.3) and also latest develop versions. |
||||
|
||||
#### Automatic dependency |
||||
|
||||
To add an iOS dependency to the current stable or latest develop version of `go-ethereum`, |
||||
you'll need to ensure that your iOS XCode project is configured to use CocoaPods. |
||||
Detailing that is out of scope in this document, but you can find a guide in the upstream |
||||
[Using CocoaPods](https://guides.cocoapods.org/using/using-cocoapods.html) page. |
||||
Afterwards you can edit your `Podfile` to list `go-ethereum` as a dependency: |
||||
|
||||
```ruby |
||||
target 'MyApp' do |
||||
# All your previous dependencies |
||||
pod 'Geth', '1.5.4' # Change the version to the latest release |
||||
end |
||||
``` |
||||
|
||||
Alternatively, if you'd like to use the latest develop version, replace the package |
||||
version `1.5.4` with `~> 1.5.5-unstable` to switch to pre-releases and to always pull in |
||||
the latest bundle from a particular release family. |
||||
|
||||
#### Custom dependency |
||||
|
||||
If you prefer not to depend on CocoaPods; or would like to access an older develop build |
||||
not available any more as an online dependency, you can download any bundle directly from |
||||
[our website](https://geth.ethereum.org/downloads/) and insert it into your project in |
||||
XCode via `Project Settings -> Build Phases -> Link Binary With Libraries`. |
||||
|
||||
Do not forget to extract the framework from the compressed `.tar.gz` archive. You can do |
||||
that either using a GUI tool or from the command line via (replace the archive with your |
||||
downloaded file): |
||||
|
||||
``` |
||||
tar -zxvf geth-ios-all-1.5.3-unstable-e05d35e6.tar.gz |
||||
``` |
||||
|
||||
#### Manual builds |
||||
|
||||
Lastly, if you would like to make modifications to the `go-ethereum` mobile code and/or |
||||
build it yourself locally instead of downloading a pre-built bundle, you can do so using a |
||||
`make` command. This will create an iOS XCode framework called `Geth.framework` in the |
||||
`build/bin` folder that you can import into XCode as described above. |
||||
|
||||
```bash |
||||
$ make ios |
||||
[...] |
||||
Done building. |
||||
Import "build/bin/Geth.framework" to use the library. |
||||
``` |
@ -0,0 +1,208 @@ |
||||
--- |
||||
title: Mining |
||||
sort_key: F |
||||
--- |
||||
|
||||
|
||||
The Ethereum blockchain grows when nodes add blocks and distribute them to their peers. Nodes |
||||
that add blocks are rewarded with ether payouts. This creates competition for the right to add |
||||
blocks to the blockchain. This means some mechanism must exist to select a single block for each |
||||
position in the blockchain that all nodes can agree upon. The mechanism that currently achieves |
||||
this for Ethereum is "proof-of-work" (PoW). This involved computing a certain value that can |
||||
only be calculated using repeated random guesses. Only if a node can demonstrate that they have |
||||
calculated this value, and therefore expended energy, will their block be accepted by other nodes. |
||||
This secures the network. This process of creating blocks and securing them using proof-of-work |
||||
is known as "mining". |
||||
|
||||
Much more information about mining, including details about the specific algorithm ("Ethash") used by |
||||
Ethereum nodes is available on |
||||
[ethereum.org](https://ethereum.org/en/developers/docs/consensus-mechanisms/pow/mining). |
||||
|
||||
|
||||
## Mining and the Merge |
||||
|
||||
[The Merge](https://ethereum.org/en/upgrades/merge) is an upcoming upgrade to Ethereum that |
||||
will swap the existing PoW for a [proof-of-stake (PoS)](https://ethereum.org/en/developers/docs/consensus-mechanisms/pos) consensus mechanism. This marks the end of mining on Ethereum. Instead, nodes can [stake ether](https://ethereum.org/en/staking/solo/#get-started-on-the-staking-launchpad) directly and earn ether rewards by running [validators](https://ethereum.org/en/developers/docs/consensus-mechanisms/pos/#validators). The merge is expected to happen in the second half of 2022. Until then, Ethereum will continue to be secured by PoW miners. It is no longer recommended to purchase new hardware to participate in Ethereum mining because the chances of returning a profit before The Merge are low. |
||||
|
||||
|
||||
## CPU vs GPU |
||||
|
||||
Participating in Ethereum's PoW mining requires running an algorithm called |
||||
["Ethash"](https://ethereum.org/en/developers/docs/consensus-mechanisms/pow/mining-algorithms/ethash). Geth includes |
||||
a CPU miner which runs Ethash within the Geth process. This might be useful for mining on some testnets. However, this is CPU mining is not viable on Ethereum Mainnet because CPU miners are easily out-competed by more efficient GPU miners. GPU mining is the recommended method for mining real ether on Ethereum Mainnet, but it is not part of the standard Geth installation. To mine using GPUs an additional piece of third-paty software is required. The recommended GPU mining software is [Ethminer](https://github.com/ethereum-mining/ethminer). |
||||
|
||||
Regardless of the mining method, the blockchain must be fully synced before mining is started, otherwise the miner will build on an incorrect chain, invalidating the block rewards. |
||||
|
||||
|
||||
## GPU Mining |
||||
|
||||
### Installing Ethminer |
||||
|
||||
The Ethminer software can be installed from a downloaded binary or built from source. The relevant downloads |
||||
and installation instructions are available from the [Ethminer Github](https://github.com/ethereum-mining/ethminer/#build). Standalone executables are available for Linux, macOS and Windows. |
||||
|
||||
### Using Ethminer with Geth |
||||
|
||||
|
||||
An account to receive block rewards must first be defined. The address of the account is all that is required to start mining - the mining rewards will be credited to that address. This can be an existing address or one that is newly created by Geth. More detailed instructions on creating and importing accounts are available on the [Account Management](/docs/interface/managing-your-accounts) page. |
||||
|
||||
The account address can be provided to `--mining.etherbase` when Geth is started. This instructs Geth to direct any block rewards to this address. Once started, Geth will sync the blockchain. If Geth has not connected to this network before, or if the data directory has been deleted, this can take several days. Also, enable HTTP traffic with the `--http` command. |
||||
|
||||
```shell |
||||
geth --http --miner.etherbase 0xC95767AC46EA2A9162F0734651d6cF17e5BfcF10 |
||||
``` |
||||
|
||||
The progress of the blockchain syncing can be monitored by attaching a JavaScript console in another terminal. More detailed information about the console can be found on the [Javascript Console](/docs/interface/javascript-console) page. To attach and open a console: |
||||
|
||||
```shell |
||||
geth attach http://127.0.0.1:8545 |
||||
``` |
||||
|
||||
Then in the console, to check the sync progress: |
||||
|
||||
```shell |
||||
eth.syncing |
||||
``` |
||||
|
||||
If the sync is progressing correctly the output will look similar to the following: |
||||
|
||||
```terminal |
||||
{ |
||||
currentBlock: 13891665, |
||||
healedBytecodeBytes: 0, |
||||
healedBytecodes: 0, |
||||
healedTrienodeBytes: 0, |
||||
healedTrienodes: 0, |
||||
healingBytecode: 0, |
||||
healingTrienodes: 0, |
||||
highestBlock: 14640000, |
||||
startingBlock: 13891665, |
||||
syncedAccountBytes: 0, |
||||
syncedAccounts: 0, |
||||
syncedBytecodeBytes: 0, |
||||
syncedBytecodes: 0, |
||||
syncedStorage: 0, |
||||
syncedStorageBytes: 0 |
||||
} |
||||
``` |
||||
|
||||
Once the blockchain is sync'd, mining can begin. In order to begin mining, Ethminer must be run and connected to Geth in a new terminal. OpenCL can be used for a wide range of GPUs, CUDA can be used specifically for Nvidia GPUs: |
||||
|
||||
```shell |
||||
#OpenCL |
||||
ethminer -v 9 -G -P http://127.0.0.1:8545 |
||||
``` |
||||
|
||||
```shell |
||||
#CUDA |
||||
ethminer -v -U -P http://127.0.0.1:8545 |
||||
``` |
||||
|
||||
Ethminer communicates with Geth on port 8545 (Geth's default RPC port) but this can be changed by providing a custom |
||||
port to the `http.port` command. The corresponding port must also be configured in Ethminer by providing |
||||
`-P http://127.0.0.1:<port-number>`. This is necessary when multiple instances of Geth/Ethminer will coexist on the same machine. |
||||
|
||||
If using OpenCL and the default for `ethminer` does not work, specifying the device using the `--opencl--device X` command is a common fix. `X` is an integer `1`, `2`, `3` etc. The Ethminer `-M` (benchmark) command should display something that looks like: |
||||
|
||||
```terminal |
||||
Benchmarking on platform: { "platform": "NVIDIA CUDA", "device": "GeForce GTX 750 Ti", "version": "OpenCL 1.1 CUDA" } |
||||
|
||||
Benchmarking on platform: { "platform": "Apple", "device": "Intel(R) Xeon(R) CPU E5-1620 v2 @ 3.70GHz", "version": "OpenCL 1.2 " } |
||||
``` |
||||
|
||||
Note that the Geth command `miner.hashrate` only works for CPU mining - it always reports zero for GPU mining. To check the GPU mining hashrate, check the logs `ethminer` displays to its terminal. More verbose logs can be configured using `-v` and a value between 0-9. |
||||
|
||||
The Ethash algorithm is [memory-hard](https://crypto.stackexchange.com/questions/84002/memory-hard-vs-memory-bound-functions) and requires a large dataset to be loaded into memory. Each GPU requires 4-5 GB of RAM. The error message `Error GPU mining. GPU memory fragmentation?` indicates that there is insufficient memory available. |
||||
|
||||
|
||||
## CPU Mining with Geth |
||||
|
||||
When Geth is started is is not mining by default. Unless it is specifically instructed to mine, it acts only as a node, not a miner. Geth starts as a (CPU) miner if the `--mine` flag is provided. The `--miner.threads` parameter can |
||||
be used to set the number parallel mining threads (defaulting to the total number of processor cores). |
||||
|
||||
```shell |
||||
geth --mine --miner.threads=4 |
||||
``` |
||||
|
||||
CPU mining can also be started and stopped at runtime using the [console](/docs/interface/javascript-console). The command `miner.start` takes an optional parameter for the number of miner threads. |
||||
|
||||
```js |
||||
miner.start(8) |
||||
true |
||||
miner.stop() |
||||
true |
||||
``` |
||||
|
||||
Note that mining for real ether only makes sense if you are in sync with the network (since you mine on top of the consensus block). Therefore the Ethereum blockchain downloader/synchroniser will delay mining until syncing is complete, and after that mining automatically starts unless you cancel your intention with `miner.stop()`. |
||||
|
||||
Like with GPU mining, an etherbase account must be set. This defaults to the primary account in the keystore but can be set to an alternative address using the `--miner.etherbase` command: |
||||
|
||||
```shell |
||||
geth --miner.etherbase '0xC95767AC46EA2A9162F0734651d6cF17e5BfcF10' --mine |
||||
``` |
||||
If there is no account available the miner will not start. The Javascript console can also be used to reset the etherbase account at runtime: |
||||
|
||||
```shell |
||||
miner.setEtherbase(eth.accounts[2]) |
||||
``` |
||||
|
||||
Note that your etherbase does not need to be an address of a local account, it just has to be set to an existing one. |
||||
|
||||
There is an option to add extra data (32 bytes only) to the mined blocks. By convention this is interpreted as a unicode string, so it can be used to add a short vanity tag using `miner.setExtra` in the Javascript console. |
||||
|
||||
```shell |
||||
miner.setExtra("ΞTHΞЯSPHΞЯΞ") |
||||
``` |
||||
|
||||
The console can also be used to check the current hashrate in units H/s (Hash operations per second): |
||||
|
||||
```shell |
||||
eth.hashrate |
||||
712000 |
||||
``` |
||||
|
||||
After some blocks have been mined, the etherbase account balance with be >0. Assuming the etherbase is a local account: |
||||
|
||||
```shell |
||||
eth.getBalance(eth.coinbase).toNumber(); |
||||
'34698870000000' |
||||
``` |
||||
|
||||
It is also possible to check which blocks were mined by a particular miner (address) using the following code snippet in the Javascript console: |
||||
|
||||
```js |
||||
function minedBlocks(lastn, addr) { |
||||
addrs = []; |
||||
if (!addr) { |
||||
addr = eth.coinbase |
||||
} |
||||
limit = eth.blockNumber - lastn |
||||
for (i = eth.blockNumber; i >= limit; i--) { |
||||
if (eth.getBlock(i).miner == addr) { |
||||
addrs.push(i) |
||||
} |
||||
} |
||||
return addrs |
||||
} |
||||
|
||||
// scans the last 1000 blocks and returns the blocknumbers of blocks mined by your coinbase |
||||
// (more precisely blocks the mining reward for which is sent to your coinbase). |
||||
minedBlocks(1000, eth.coinbase) |
||||
[352708, 352655, 352559] |
||||
|
||||
``` |
||||
|
||||
The etherbase balance will fluctuate because quite often a mined block may be re-org'd out |
||||
of the canonical chain. This means that when the local Geth node includes the mined block |
||||
in its own local blockchain the account balance appears higher because the block rewards are |
||||
applied. When the node switches to another version of the chain due to information received |
||||
from peers, that block may not be included and the block rewards are not applied. |
||||
|
||||
The logs show locally mined blocks confirmed after 5 blocks. |
||||
|
||||
|
||||
## Summary |
||||
|
||||
The page describes how to start Geth as a mining node. Mining can be done on CPUs - in which case Geth's built-in |
||||
miner can be used - or on GPUs which requires third party software. GPUs are required to mine real ether on Ethereum |
||||
Mainnet. It is important to note that Ethereum will swap its consensus mechanism from PoW to PoS in the second half of 2022. This swap, known as "The Merge" will end mining on Ethereum. |
@ -0,0 +1,597 @@ |
||||
--- |
||||
title: Geth and Clef |
||||
permalink: docs/getting-started/geth-and-clef |
||||
sort_key: B |
||||
--- |
||||
|
||||
This page explains how to set up Geth and execute some basic tasks using the command line tools. |
||||
In order to use Geth, the software must first be installed. There are several ways Geth can be |
||||
installed depending on the operating system and the user's choice of installation method, for example |
||||
using a package manager, container or building from source. Instructions for installing Geth can be |
||||
found on the ["Install and Build"](install-and-build/installing-geth) pages. The tutorial on this |
||||
page assumes Geth and the associated developer tools have been installed successfully. |
||||
|
||||
This page provides step-by-step instructions covering the fundamentals of using Geth. This |
||||
includes generating accounts, joining an Ethereum network, syncing the blockchain and sending ether |
||||
between accounts. This tutorial also uses [Clef](/docs/clef/tutorial). Clef is an account management tool |
||||
external to Geth itself that allows users to sign transactions. It is developed and maintained by |
||||
the Geth team and is intended to eventually replace the account management tool built in to Geth. |
||||
|
||||
## Prerequisites |
||||
|
||||
In order to get the most value from the tutorials on this page, the following skills are necessary: |
||||
|
||||
- Experience using the command line |
||||
- Basic knowledge about Ethereum and testnets |
||||
- Basic knowledge about HTTP and JavaScript |
||||
|
||||
Users that need to revisit these fundamentals can find helpful resources relating to the command line |
||||
[here][cli], |
||||
Ethereum and its testnets [here](https://ethereum.org/en/developers/tutorials/), http |
||||
[here](https://developer.mozilla.org/en-US/docs/Web/HTTP) and Javascript [here](https://www.javascript.com/learn). |
||||
|
||||
{% include note.html content="If Geth was installed from source on Linux, `make` saves the binaries for |
||||
Geth and the associated tools in `/build/bin`. To run these programs it is convenient to move them to |
||||
the top level project directory (e.g. running `mv ./build/bin/* ./`) from `/go-ethereum`. Then `./` must |
||||
be prepended to the commands in the code snippets in order to execute a particular program, e.g. `./geth` |
||||
instead of simply `geth`. If the executables are not moved then either navigate to the `bin` directory to |
||||
run them (e.g. `cd ./build/bin` and `./geth`) or provide their path (e.g. `./build/bin/geth`). These |
||||
instructions can be ignored for other installations." %} |
||||
|
||||
## Background |
||||
|
||||
Geth is an Ethereum client written in Go. This means running Geth turns a computer into an Ethereum node. |
||||
Ethereum is a peer-to-peer network where information is shared directly between nodes rather than being |
||||
managed by a central server. Nodes compete to generate new blocks of transactions to send to its peers |
||||
because they are rewarded for doing so in Ethereum's native token, ether (ETH). On receiving a new block, |
||||
each node checks that it is valid and adds it to their database. The sequence of discrete blocks is called |
||||
a "blockchain". The information provided in each block is used by Geth to update its "state" - the ether |
||||
balance of each account on Ethereum. There are two types of account: externally-owned accounts (EOAs) and |
||||
contract accounts. Contract accounts execute contract code when they receive transactions. EOAs are accounts |
||||
that users manage locally in order to sign and submit transactions. Each EOA is a public-private key pair, |
||||
where the public key is used to derive a unique address for the user and the private key is used to protect |
||||
the account and securely sign messages. Therefore, in order to use Ethereum, it is first necessary to generate |
||||
an EOA (hereafter, "account"). This tutorial will guide the user through creating an account, funding it with |
||||
ether and sending some to another address. |
||||
|
||||
Read more about Ethereum accounts [here](https://ethereum.org/en/developers/docs/accounts/). |
||||
|
||||
## Step 1: Generating accounts |
||||
|
||||
There are several methods for generating accounts in Geth. This tutorial demonstrates how to generate accounts |
||||
using Clef, as this is considered best practice, largely because it decouples the users' key management from Geth, |
||||
making it more modular and flexible. It can also be run from secure USB sticks or virtual machines, offering |
||||
security benefits. For convenience, this tutorial will execute Clef on the same computer that will also run Geth, |
||||
although more secure options are available (see [here](https://github.com/ethereum/go-ethereum/blob/master/cmd/clef/docs/setup.md)). |
||||
|
||||
An account is a pair of keys (public and private). Clef needs to know where to save these keys to so that they |
||||
can be retrieved later. This information is passed to Clef as an argument. This is achieved using the following command: |
||||
|
||||
```shell |
||||
clef newaccount --keystore geth-tutorial/keystore |
||||
``` |
||||
|
||||
The specific function from Clef that generates new accounts is `newaccount` and it accepts a |
||||
parameter, `--keystore`, that tells it where to store the newly generated keys. In this |
||||
example the keystore location is a new directory that will be created automatically: `geth-tutorial/keystore`. |
||||
Clef will return the following result in the terminal: |
||||
|
||||
```terminal |
||||
WARNING! |
||||
|
||||
Clef is an account management tool. It may, like any software, contain bugs. |
||||
|
||||
Please take care to |
||||
- backup your keystore files, |
||||
- verify that the keystore(s) can be opened with your password. |
||||
|
||||
Clef is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY |
||||
without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR |
||||
PURPOSE. See the GNU General Public License for more details. |
||||
|
||||
Enter 'ok' to proceed: |
||||
> |
||||
``` |
||||
|
||||
This is important information. The `geth-tutorial/keystore` directory will soon contain a secret |
||||
key that can be used to access any funds held in the new account. If it is compromised, the funds |
||||
can be stolen. If it is lost, there is no way to retrieve the funds. This tutorial will only use |
||||
dummy funds with no real world value, but when these steps are repeated on Ethereum mainnet is |
||||
critical that the keystore is kept secure and backed up. |
||||
|
||||
|
||||
Typing `ok` into the terminal and pressing `enter` causes Clef to prompt for a password. |
||||
Clef requires a password that is at least 10 characters long, and best practice would be |
||||
to use a combination of numbers, characters and special characters. Entering a suitable |
||||
password and pressing `enter` returns the following result to the terminal: |
||||
|
||||
```terminal |
||||
----------------------- |
||||
DEBUG[02-10|13:46:46.436] FS scan times list="92.081µs" set="12.629µs" diff="2.129µs" |
||||
INFO [02-10|13:46:46.592] Your new key was generated address=0xCe8dBA5e4157c2B284d8853afEEea259344C1653 |
||||
WARN [02-10|13:46:46.595] Please backup your key file! path=keystore:///.../geth-tutorial/keystore/UTC--2022-02-07T17-19-56.517538000Z--ca57f3b40b42fcce3c37b8d18adbca5260ca72ec |
||||
WARN [02-10|13:46:46.595] Please remember your password! |
||||
Generated account 0xCe8dBA5e4157c2B284d8853afEEea259344C1653 |
||||
``` |
||||
|
||||
It is important to save the account address and the password somewhere secure. |
||||
They will be used again later in this tutorial. Please note that the account address shown |
||||
in the code snippets above and later in this tutorials are examples - those generated by followers |
||||
of this tutorial will be different. The account generated above can be used as the main account |
||||
throughout the remainder of this tutorial. However in order to demonstrate transactions between |
||||
accounts it is also necessary to have a second account. A second account can be added to the |
||||
same keystore by precisely repeating the previous steps, providing the same password. |
||||
|
||||
## Step 2: Start Clef |
||||
|
||||
The previous commands used Clef's `newaccount` function to add new key pairs to the keystore. |
||||
Clef uses the private key(s) saved in the keystore is used to sign transactions. In order to do |
||||
this, Clef needs to be started and left running while Geth is running simultaneously, so that the |
||||
two programs can communicate between one another. |
||||
|
||||
To start Clef, run the Clef executable passing as arguments the keystore file location, |
||||
config directory location and a chain ID. The config directory was automatically created |
||||
inside the `geth-tutorial` directory during the previous step. The [chain ID](https://chainlist.org/) |
||||
is an integer that defines which Ethereum network to connect to. Ethereum mainnet has chain ID 1. |
||||
In this tutorial Chain ID 5 is used which is that of the Goerli testnet. It is very important that |
||||
this chain ID parameter is set to 5. The following command starts Clef on Goerli: |
||||
|
||||
```shell |
||||
|
||||
clef --keystore geth-tutorial/keystore --configdir geth-tutorial/clef --chainid 5 |
||||
|
||||
``` |
||||
|
||||
After running the command above, Clef requests the user to type “ok” to proceed. On typing "ok" and pressing enter, Clef returns the following to the terminal: |
||||
|
||||
```terminal |
||||
INFO [02-10|13:55:30.812] Using CLI as UI-channel |
||||
INFO [02-10|13:55:30.946] Loaded 4byte database embeds=146,841 locals=0 local=./4byte-custom.json |
||||
WARN [02-10|13:55:30.947] Failed to open master, rules disabled err="failed stat on geth-tutorial/clef/masterseed.json: stat geth-tutorial/clef/masterseed.json: no such file or directory" |
||||
INFO [02-10|13:55:30.947] Starting signer chainid=5 keystore=geth-tutorial/keystore light-kdf=false advanced=false |
||||
DEBUG[02-10|13:55:30.948] FS scan times list="133.35µs" set="5.692µs" diff="3.262µs" |
||||
DEBUG[02-10|13:55:30.970] Ledger support enabled |
||||
DEBUG[02-10|13:55:30.973] Trezor support enabled via HID |
||||
DEBUG[02-10|13:55:30.976] Trezor support enabled via WebUSB |
||||
INFO [02-10|13:55:30.978] Audit logs configured file=audit.log |
||||
DEBUG[02-10|13:55:30.981] IPCs registered namespaces=account |
||||
INFO [02-10|13:55:30.984] IPC endpoint opened url=geth-tutorial/clef/clef.ipc |
||||
------- Signer info ------- |
||||
* intapi_version : 7.0.1 |
||||
* extapi_version : 6.1.0 |
||||
* extapi_http : n/a |
||||
* extapi_ipc : geth-tutorial/clef/clef.ipc |
||||
``` |
||||
|
||||
This result indicates that Clef is running. This terminal should be left running for the duration of this |
||||
tutorial. If the tutorial is stopped and restarted later Clef must also be restarted by running the previous command. |
||||
|
||||
## Step 3: Start Geth |
||||
|
||||
Geth is the Ethereum client that will connect the computer to the Ethereum network. In this tutorial the |
||||
network is Goerli, an Ethereum testnet. Testnets are used to test Ethereum client software and smart |
||||
contracts in an environment where no real-world value is at risk. To start Geth, run the Geth executable |
||||
file passing argument that define the data directory (where Geth should save blockchain data), |
||||
signer (points Geth to Clef), the network ID and the sync mode. For this tutorial, snap sync is recommended |
||||
(see [here](https://blog.ethereum.org/2021/03/03/geth-v1-10-0/) for reasons why). The final argument |
||||
passed to Geth is the `--http` flag. This enables the http-rpc server that allows external programs to |
||||
interact with Geth by sending it http requests. By default the http server is only exposed locally using |
||||
port 8545: `localhost:8545`. |
||||
|
||||
The following command should be run in a new terminal, separate to the one running Clef: |
||||
|
||||
```shell |
||||
geth --datadir geth-tutorial --signer=geth-tutorial/clef/clef.ipc --goerli --syncmode snap --http |
||||
``` |
||||
|
||||
Running the above command starts Geth. The terminal should rapidly fill with status updates, starting with: |
||||
|
||||
```terminal |
||||
INFO [02-10|13:59:06.649] Starting Geth on goerli testnet... |
||||
INFO [02-10|13:59:06.649] Dropping default light client cache provided=1024 updated=128 |
||||
INFO [02-10|13:59:06.652] Maximum peer count ETH=50 LES=0 total=50 |
||||
INFO [02-10|13:59:06.655] Using external signer url=geth-tutorial/clef/clef.ipc |
||||
INFO [02-10|13:59:06.660] Set global gas cap cap=50,000,000 |
||||
INFO [02-10|13:59:06.661] Allocated cache and file handles database=/.../geth-tutorial/geth/chaindata cache=64.00MiB handles=5120 |
||||
INFO [02-10|13:59:06.855] Persisted trie from memory database nodes=361 size=51.17KiB time="643.54µs" gcnodes=0 gcsize=0.00B gctime=0s livenodes=1 livesize=0.00B |
||||
INFO [02-10|13:59:06.855] Initialised chain configuration config="{ChainID: 5 Homestead: 0 DAO: nil DAOSupport: true EIP150: 0 EIP155: 0 EIP158: 0 Byzantium: 0 Constantinople: 0 Petersburg: 0 Istanbul: 1561651, Muir Glacier: nil, Berlin: 4460644, London: 5062605, Arrow Glacier: nil, MergeFork: nil, Engine: clique}" |
||||
INFO [02-10|13:59:06.862] Added trusted checkpoint block=5,799,935 hash=2de018..c32427 |
||||
INFO [02-10|13:59:06.863] Loaded most recent local header number=6,340,934 hash=483cf5..858315 td=9,321,576 age=2d9h29m |
||||
INFO [02-10|13:59:06.867] Configured checkpoint oracle address=0x18CA0E045F0D772a851BC7e48357Bcaab0a0795D signers=5 threshold=2 |
||||
INFO [02-10|13:59:06.867] Gasprice oracle is ignoring threshold set threshold=2 |
||||
WARN [02-10|13:59:06.869] Unclean shutdown detected booted=2022-02-08T04:25:08+0100 age=2d9h33m |
||||
INFO [02-10|13:59:06.870] Starting peer-to-peer node instance=Geth/v1.10.15-stable/darwin-amd64/go1.17.5 |
||||
INFO [02-10|13:59:06.995] New local node record seq=1,644,272,735,880 id=d4ffcd252d322a89 ip=127.0.0.1 udp=30303 tcp=30303 |
||||
INFO [02-10|13:59:06.996] Started P2P networking self=enode://4b80ebd341b5308f7a6b61d91aa0ea31bd5fc9e0a6a5483e59fd4ea84e0646b13ecd289e31e00821ccedece0bf4b9189c474371af7393093138f546ac23ef93e@127.0.0.1:30303 |
||||
INFO [02-10|13:59:06.997] IPC endpoint opened url=/.../geth-tutorial/geth.ipc |
||||
INFO [02-10|13:59:06.998] HTTP server started endpoint=127.0.0.1:8545 prefix= cors= vhosts=localhost |
||||
WARN [02-10|13:59:06.998] Light client mode is an experimental feature |
||||
WARN [02-10|13:59:06.999] Failed to open wallet url=extapi://geth-tutorial/clef/cle.. err="operation not supported on external signers" |
||||
INFO [02-10|13:59:08.793] Block synchronisation started |
||||
``` |
||||
|
||||
This indicates that Geth has started up and is searching for peers to connect to. Once it finds peers it |
||||
can request block headers from them, starting at the genesis block for the Goerli blockchain. Geth continues |
||||
to download blocks sequentially, saving the data in files in `/go-ethereum/geth-tutorial/geth/chaindata/`. |
||||
This is confirmed by the logs printed to the terminal. There should be a rapidly-growing sequence of logs in |
||||
the terminal with the following syntax: |
||||
|
||||
```terminal |
||||
INFO [04-29][15:54:09.238] Looking for peers peercount=2 tried=0 static=0 |
||||
INFO [04-29][15:54:19.393] Imported new block headers count=2 elapsed=1.127ms number=996288 hash=09f1e3..718c47 age=13h9m5s |
||||
INFO [04-29][15:54:19:656] Imported new block receipts count=698 elapsed=4.464ms number=994566 hash=56dc44..007c93 age=13h9m9s |
||||
``` |
||||
|
||||
These logs indicate that Geth is running as expected. Sending an empty Curl request to the http server |
||||
provides a quick way to confirm that this too has been started without any issues. In a third terminal, |
||||
the following command can be run: |
||||
|
||||
```shell |
||||
curl http://localhost:8545 |
||||
``` |
||||
|
||||
If there is no error message reported to the terminal, everything is OK. Geth must be running in order for |
||||
a user to interact with the Ethereum network. If this terminal is closed down then Geth must be restarted |
||||
in a new terminal. Geth can be started and stopped easily, but it must be running for any interaction with |
||||
Ethereum to take place. To shut down Geth, simply press `CTRL+C` in the Geth terminal. To start it again, |
||||
run the previous command `geth --datadir ... ..`. |
||||
|
||||
{% include note.html content="Snap syncing Goerli will take some time and until the sync is finished you |
||||
can't use the node to transfer funds. You can also try doing a [light sync](interface/les) which will be |
||||
much quicker but depends on light servers being available to serve your node the data it needs." %} |
||||
|
||||
## Step 4: Get Testnet Ether |
||||
|
||||
In order to make some transactions, the user must fund their account with ether. On Ethereum mainnet, |
||||
ether can only be obtained in three ways: 1) by receiving it as a reward for mining/validating; |
||||
2) receiving it in a transfer from another Ethereum user or contract; 3) receiving it from an exchange, |
||||
3) having paid for it with fiat money. On Ethereum testnets, the ether has no real world value so it |
||||
4) can be made freely available via faucets. Faucets allow users to request a transfer of testnet ether to their account. |
||||
|
||||
The address generated by Clef in Step 1 can be pasted into the Paradigm Multifaucet faucet |
||||
[here](https://fauceth.komputing.org/?chain=1115511). This requires a Twitter login as proof of |
||||
personhood. The faucets adds ether to the given address on multiple testnets simultaneously, |
||||
including Goerli. In the next steps Geth will be used to check that the ether has been sent |
||||
to the given address and send some of it to the second address created earlier. |
||||
|
||||
|
||||
## Step 5: Interact with Geth |
||||
|
||||
For interacting with the blockchain, Geth provides JSON-RPC APIs. |
||||
[JSON-RPC](https://ethereum.org/en/developers/docs/apis/json-rpc/) is a way to execute specific |
||||
tasks by sending instructions to Geth in the form of [JSON](https://www.json.org/json-en.html) objects. |
||||
RPC stands for "Remote Procedure Call" and it refers to the ability to send these JSON-encoded |
||||
instructions from locations outside of those managed by Geth. It is possible to interact with Geth |
||||
by sending these JSON encoded instructions directly over Geth's exposed http port using tools like Curl. |
||||
However, this is somewhat user-unfriendly and error-prone, especially for more complex instructions. |
||||
For this reason, there are a set of libraries built on top of JSON-RPC that provide a more user-friendly |
||||
interface for interacting with Geth. One of the most widely used is Web3.js. |
||||
|
||||
Geth provides a Javascript console that exposes the Web3.js API. This means that with Geth running in one terminal, a Javascript environment can be opened in another allowing the user to interact with Geth using Web3.js. There are three transport protocols that can be used to connect the Javascript environment to Geth: |
||||
|
||||
- IPC (Inter-Process Communication): Provides unrestricted access to all APIs, but only works when the console is run on the same host as the geth node. |
||||
|
||||
- HTTP: By default provides access to the `eth`, `web3` and `net` method namespaces. |
||||
|
||||
- Websocket: By default provides access to the `eth`, `web3` and `net` method namespaces. |
||||
|
||||
This tutorial will use the HTTP option. Note that the terminals running Geth and Clef should both still be active. |
||||
In a new (third) terminal, the following command can be run to start the console and connect it to Geth |
||||
using the exposed http port: |
||||
|
||||
```shell |
||||
geth attach http://127.0.0.1:8545 |
||||
``` |
||||
|
||||
This command causes the terminal to hang because it is waiting for approval from Clef. Approving the request |
||||
in the terminal running Clef will lead to the following welcome message being displayed in the Javascript console: |
||||
|
||||
```terminal |
||||
Welcome to the Geth JavaScript console! |
||||
|
||||
instance: Geth/v1.10.15-stable/darwin-amd64/go1.17.5 |
||||
at block: 6354736 (Thu Feb 10 2022 14:01:46 GMT+0100 (WAT)) |
||||
modules: eth:1.0 net:1.0 rpc:1.0 web3:1.0 |
||||
|
||||
To exit, press ctrl-d or type exit |
||||
``` |
||||
|
||||
The console is now active and connected to Geth. It can now be used to interact with the Ethereum (Goerli) network. |
||||
|
||||
|
||||
### List of accounts |
||||
|
||||
In this tutorial, the accounts are managed using Clef. This means that requesting information about |
||||
the accounts requires explicit approval in Clef, which should still be running in its own terminal. |
||||
Earlier in this tutorial, two accounts were created using Clef. The following command will display the |
||||
addresses of those two accounts and any others that might have been added to the keystore before or since. |
||||
|
||||
```javascript |
||||
eth.accounts |
||||
``` |
||||
|
||||
The console will hang, because Clef is waiting for approval. The following message will be |
||||
displayed in the Clef terminal: |
||||
|
||||
```terminal |
||||
-------- List Account request-------------- |
||||
A request has been made to list all accounts. |
||||
You can select which accounts the caller can see |
||||
[x] 0xca57F3b40B42FCce3c37B8D18aDBca5260ca72EC |
||||
URL: keystore:///.../geth-tutorial/keystore/UTC--2022-02-07T17-19-56.517538000Z--ca57f3b40b42fcce3c37b8d18adbca5260ca72ec |
||||
[x] 0xCe8dBA5e4157c2B284d8853afEEea259344C1653 |
||||
URL: keystore:///.../geth-tutorial/keystore/UTC--2022-02-10T12-46-45.265592000Z--ce8dba5e4157c2b284d8853afeeea259344c1653 |
||||
------------------------------------------- |
||||
Request context: |
||||
NA - ipc - NA |
||||
|
||||
Additional HTTP header data, provided by the external caller: |
||||
User-Agent: "" |
||||
Origin: "" |
||||
Approve? [y/N]: |
||||
|
||||
``` |
||||
|
||||
Entering `y` approves the request from the console. In the terminal running the Javascript console, |
||||
the account addresses are now displayed: |
||||
|
||||
```terminal |
||||
["0xca57f3b40b42fcce3c37b8d18adbca5260ca72ec", "0xce8dba5e4157c2b284d8853afeeea259344c1653"] |
||||
``` |
||||
|
||||
It is also possible for this request to time out if the Clef approval took too long - |
||||
in this case simply repeat the request and approval. |
||||
|
||||
|
||||
### Checking account balance. |
||||
|
||||
Having confirmed that the two addresses created earlier are indeed in the keystore and accessible |
||||
through the Javascript console, it is possible to retrieve information about how much ether they own. |
||||
The Goerli faucet should have sent 1 ETH to the address provided, meaning that the balance of one of |
||||
the accounts should be 1 ether and the other should be 0. The following command displays the account |
||||
balance in the console: |
||||
|
||||
```javascript |
||||
web3.fromWei(eth.getBalance("0xca57F3b40B42FCce3c37B8D18aDBca5260ca72EC"), "ether") |
||||
``` |
||||
|
||||
There are actually two instructions sent in the above command. The inner one is the `getBalance` |
||||
function from the `eth` namespace. This takes the account address as its only argument. By default, |
||||
this returns the account balance in units of Wei. There are 10<sup>18</sup> Wei to one ether. |
||||
To present the result in units of ether, `getBalance` is wrapped in the `fromWei` function from |
||||
the `web3` namespace. Running this command should provide the following result |
||||
(for the account that received faucet funds): |
||||
|
||||
```terminal |
||||
1 |
||||
``` |
||||
|
||||
Repeating the command for the other account should yield: |
||||
|
||||
```terminal |
||||
0 |
||||
``` |
||||
|
||||
### Send ether to another account |
||||
|
||||
The command `eth.sendTransaction` can be used to send some ether from one address to another. |
||||
This command takes three arguments: `from`, `to` and `value`. These define the sender and |
||||
recipient addresses (as strings) and the amount of Wei to transfer. It is far less error |
||||
prone to enter the transaction value in units of ether rather than Wei, so the value field can |
||||
take the return value from the `toWei` function. The following command, run in the Javascript |
||||
console, sends 0.1 ether from one of the accounts in the Clef keystore to the other. Note that |
||||
the addresses here are examples - the user must replace the address in the `from` field with |
||||
the address currently owning 1 ether, and the address in the `to` field with the address |
||||
currently holding 0 ether. |
||||
|
||||
```javascript |
||||
eth.sendTransaction({ |
||||
from: "0xca57f3b40b42fcce3c37b8d18adbca5260ca72ec", |
||||
to: "0xce8dba5e4157c2b284d8853afeeea259344c1653", |
||||
value: web3.toWei(0.1, "ether") |
||||
}) |
||||
``` |
||||
|
||||
Note that submitting this transaction requires approval in Clef. In the Clef terminal, |
||||
Clef will prompt for approval and request the account password. If the password is correctly |
||||
entered, Geth proceeds with the transaction. The transaction request summary is presented by |
||||
Clef in the Clef terminal. This is an opportunity for the sender to review the details and |
||||
ensure they are correct. |
||||
|
||||
```terminal |
||||
--------- Transaction request------------- |
||||
to: 0xCe8dBA5e4157c2B284d8853afEEea259344C1653 |
||||
from: 0xca57F3b40B42FCce3c37B8D18aDBca5260ca72EC [chksum ok] |
||||
value: 10000000000000000 wei |
||||
gas: 0x5208 (21000) |
||||
maxFeePerGas: 2425000057 wei |
||||
maxPriorityFeePerGas: 2424999967 wei |
||||
nonce: 0x3 (3) |
||||
chainid: 0x5 |
||||
Accesslist |
||||
|
||||
Request context: |
||||
NA - ipc - NA |
||||
|
||||
Additional HTTP header data, provided by the external caller: |
||||
User-Agent: "" |
||||
Origin: "" |
||||
------------------------------------------- |
||||
Approve? [y/N]: |
||||
|
||||
Please enter the password for account 0xca57F3b40B42FCce3c37B8D18aDBca5260ca72EC |
||||
|
||||
``` |
||||
|
||||
After approving the transaction, the following confirmation screen in displayed in |
||||
the Clef terminal: |
||||
|
||||
```terminal |
||||
----------------------- |
||||
Transaction signed: |
||||
{ |
||||
"type": "0x2", |
||||
"nonce": "0x3", |
||||
"gasPrice": null, |
||||
"maxPriorityFeePerGas": "0x908a901f", |
||||
"maxFeePerGas": "0x908a9079", |
||||
"gas": "0x5208", |
||||
"value": "0x2386f26fc10000", |
||||
"input": "0x", |
||||
"v": "0x0", |
||||
"r": "0x66e5d23ad156e04363e68b986d3a09e879f7fe6c84993cef800bc3b7ba8af072", |
||||
"s": "0x647ff82be943ea4738600c831c4a19879f212eb77e32896c05055174045da1bc", |
||||
"to": "0xce8dba5e4157c2b284d8853afeeea259344c1653", |
||||
"chainId": "0x5", |
||||
"accessList": [], |
||||
"hash": "0x99d489d0bd984915fd370b307c2d39320860950666aac3f261921113ae4f95bb" |
||||
} |
||||
``` |
||||
|
||||
In the Javascript console, the transaction hash is displayed. This will be used in the |
||||
next section to retrieve the transaction details. |
||||
|
||||
```terminal |
||||
"0x99d489d0bd984915fd370b307c2d39320860950666aac3f261921113ae4f95bb" |
||||
``` |
||||
|
||||
It is also advised to check the account balances using Geth by repeating the instructions from |
||||
earlier. At this point in the tutorial, the two accounts in the Clef keystore should have balances |
||||
just below 0.9 ether (because 0.1 ether has been transferred out and some small amount paid in |
||||
transaction gas) and 0.1 ether. |
||||
|
||||
|
||||
### Checking the transaction hash |
||||
|
||||
The transaction hash is a unique identifier for this specific transaction that can be used |
||||
later to retrieve the transaction details. For example, the transaction details can be |
||||
viewed by pasting this hash into the [Goerli block explorer](https://goerli.etherscan.io/). |
||||
The same information can also be retrieved directly from the Geth node. The hash returned in |
||||
the previous step can be provided as an argument to `eth.getTransaction` to return the |
||||
transaction information: |
||||
|
||||
```javascript |
||||
eth.getTransaction("0x99d489d0bd984915fd370b307c2d39320860950666aac3f261921113ae4f95bb") |
||||
``` |
||||
|
||||
This returns the following response (although the actual values for each field will vary |
||||
because they are specific to each transaction): |
||||
|
||||
```terminal |
||||
{ |
||||
accessList: [], |
||||
blockHash: "0x1c5d3f8dd997b302935391b57dc3e4fffd1fa2088ef2836d51f844f993eb39c4", |
||||
blockNumber: 6355150, |
||||
chainId: "0x5", |
||||
from: "0xca57f3b40b42fcce3c37b8d18adbca5260ca72ec", |
||||
gas: 21000, |
||||
gasPrice: 2425000023, |
||||
hash: "0x99d489d0bd984915fd370b307c2d39320860950666aac3f261921113ae4f95bb", |
||||
input: "0x", |
||||
maxFeePerGas: 2425000057, |
||||
maxPriorityFeePerGas: 2424999967, |
||||
nonce: 3, |
||||
r: "0x66e5d23ad156e04363e68b986d3a09e879f7fe6c84993cef800bc3b7ba8af072", |
||||
s: "0x647ff82be943ea4738600c831c4a19879f212eb77e32896c05055174045da1bc", |
||||
to: "0xce8dba5e4157c2b284d8853afeeea259344c1653", |
||||
transactionIndex: 630, |
||||
type: "0x2", |
||||
v: "0x0", |
||||
value: 10000000000000000 |
||||
} |
||||
``` |
||||
|
||||
## Using Curl |
||||
|
||||
Up to this point this tutorial has interacted with Geth using the convenience library Web3.js. |
||||
This library enables the user to send instructions to Geth using a more user-friendly interface |
||||
compared to sending raw JSON objects. However, it is also possible for the user to send these |
||||
JSON objects directly to Geth's exposed HTTP port. Curl is a command line tool that sends HTTP |
||||
requests. This part of the tutorial demonstrates how to check account balances and send a |
||||
transaction using Curl. |
||||
|
||||
### Checking account balance |
||||
|
||||
The command below returns the balance of the given account. This is a HTTP POST request to the |
||||
local port 8545. The `-H` flag is for header information. It is used here to define the format |
||||
of the incoming payload, which is JSON. The `--data` flag defines the content of the payload, |
||||
which is a JSON object. That JSON object contains four fields: `jsonrpc` defines the spec version |
||||
for the JSON-RPC API, `method` is the specific function being invoked, `params` are the function |
||||
arguments, and `id` is used for ordering transactions. The two arguments passed to `eth_getBalance` |
||||
are the account address whose balance to check and the block to query (here `latest` is used to |
||||
check the balance in the most recently mined block). |
||||
|
||||
```shell |
||||
curl -X POST http://127.0.0.1:8545 \ |
||||
-H "Content-Type: application/json" \ |
||||
--data '{"jsonrpc":"2.0", "method":"eth_getBalance", "params":["0xca57f3b40b42fcce3c37b8d18adbca5260ca72ec","latest"], "id":1}' |
||||
``` |
||||
|
||||
A successful call will return a response like the one below: |
||||
|
||||
```terminal |
||||
{"jsonrpc":"2.0","id":1,"result":"0xc7d54951f87f7c0"} |
||||
``` |
||||
|
||||
The balance is in the `result` field in the returned JSON object. However, it is denominated in |
||||
Wei and presented as a hexadecimal string. There are many options for converting this value to a |
||||
decimal in units of ether, for example by opening a Python console and running: |
||||
|
||||
```python |
||||
0xc7d54951f87f7c0 / 1e18 |
||||
``` |
||||
This returns the balance in ether: |
||||
|
||||
```terminal |
||||
0.8999684999998321 |
||||
``` |
||||
|
||||
### Checking the account list |
||||
|
||||
The curl command below returns the list of all accounts. |
||||
|
||||
```shell |
||||
curl -X POST http://127.0.0.1:8545 \ |
||||
-H "Content-Type: application/json" \ |
||||
--data '{"jsonrpc":"2.0", "method":"eth_accounts","params":[], "id":1}' |
||||
``` |
||||
|
||||
This requires approval in Clef. Once approved, the following information is returned to the terminal: |
||||
|
||||
```terminal |
||||
{"jsonrpc":"2.0","id":1,"result":["0xca57f3b40b42fcce3c37b8d18adbca5260ca72ec"]} |
||||
``` |
||||
|
||||
### Sending Transactions |
||||
|
||||
Sending a transaction between accounts can also be achieved using Curl. Notice that the value of the |
||||
transaction is a hexadecimal string in units of Wei. To transfer 0.1 ether, it is first necessary to |
||||
convert this to Wei by multiplying by 10<sup>18</sup> then converting to hex. 0.1 ether is |
||||
`"0x16345785d8a0000"` in hex. As before, update the `to` and `from` fields with the addresses in |
||||
the Clef keystore. |
||||
|
||||
|
||||
```shell |
||||
curl -X POST http://127.0.0.1:8545 \ |
||||
-H "Content-Type: application/json" \ |
||||
--data '{"jsonrpc":"2.0", "method":"eth_sendTransaction", "params":[{"from": "0xca57f3b40b42fcce3c37b8d18adbca5260ca72ec","to": "0xce8dba5e4157c2b284d8853afeeea259344c1653","value": "0x16345785d8a0000"}], "id":1}' |
||||
``` |
||||
|
||||
This requires approval in Clef. Once the password for the sender account has been provided, |
||||
Clef will return a summary of the transaction details and the terminal that made the Curl |
||||
request will display a response containing the transaction hash. |
||||
|
||||
```terminal |
||||
{"jsonrpc":"2.0","id":5,"result":"0xac8b347d70a82805edb85fc136fc2c4e77d31677c2f9e4e7950e0342f0dc7e7c"} |
||||
``` |
||||
|
||||
## Summary |
||||
|
||||
This tutorial has demonstrated how to generate accounts using Clef, fund them with testnet ether and use |
||||
those accounts to interact with Ethereum (Goerli) through a Geth node. Checking account balances, sending |
||||
transactions and retrieving transaction details were explained using the web3.js library via the |
||||
Geth console and using the JSON-RPC directly using Curl. For more detailed information about Clef, please see |
||||
[the Clef docs](/docs/clef/tutorial). |
||||
|
||||
|
||||
[cli]: https://developer.mozilla.org/en-US/docs/Learn/Tools_and_testing/Understanding_client-side_tools/Command_line |
Loading…
Reference in new issue