mirror of https://github.com/go-gitea/gitea
Use binary version of revive linter (#15739)
Use the common `go get` method to install and run the revive linter, removing the useless build/lint.go and related vendor libraries.pull/15799/head^2
parent
a69fb523a7
commit
c3802dcc0f
@ -1,325 +0,0 @@ |
|||||||
// Copyright 2020 The Gitea Authors. All rights reserved.
|
|
||||||
// Copyright (c) 2018 Minko Gechev. All rights reserved.
|
|
||||||
// Use of this source code is governed by a MIT-style
|
|
||||||
// license that can be found in the LICENSE file.
|
|
||||||
|
|
||||||
// +build ignore
|
|
||||||
|
|
||||||
package main |
|
||||||
|
|
||||||
import ( |
|
||||||
"flag" |
|
||||||
"fmt" |
|
||||||
"io/ioutil" |
|
||||||
"os" |
|
||||||
"path/filepath" |
|
||||||
"strings" |
|
||||||
|
|
||||||
"github.com/mgechev/dots" |
|
||||||
"github.com/mgechev/revive/formatter" |
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
"github.com/mgechev/revive/rule" |
|
||||||
"github.com/mitchellh/go-homedir" |
|
||||||
"github.com/pelletier/go-toml" |
|
||||||
) |
|
||||||
|
|
||||||
func fail(err string) { |
|
||||||
fmt.Fprintln(os.Stderr, err) |
|
||||||
os.Exit(1) |
|
||||||
} |
|
||||||
|
|
||||||
var defaultRules = []lint.Rule{ |
|
||||||
&rule.VarDeclarationsRule{}, |
|
||||||
&rule.PackageCommentsRule{}, |
|
||||||
&rule.DotImportsRule{}, |
|
||||||
&rule.BlankImportsRule{}, |
|
||||||
&rule.ExportedRule{}, |
|
||||||
&rule.VarNamingRule{}, |
|
||||||
&rule.IndentErrorFlowRule{}, |
|
||||||
&rule.IfReturnRule{}, |
|
||||||
&rule.RangeRule{}, |
|
||||||
&rule.ErrorfRule{}, |
|
||||||
&rule.ErrorNamingRule{}, |
|
||||||
&rule.ErrorStringsRule{}, |
|
||||||
&rule.ReceiverNamingRule{}, |
|
||||||
&rule.IncrementDecrementRule{}, |
|
||||||
&rule.ErrorReturnRule{}, |
|
||||||
&rule.UnexportedReturnRule{}, |
|
||||||
&rule.TimeNamingRule{}, |
|
||||||
&rule.ContextKeysType{}, |
|
||||||
&rule.ContextAsArgumentRule{}, |
|
||||||
} |
|
||||||
|
|
||||||
var allRules = append([]lint.Rule{ |
|
||||||
&rule.ArgumentsLimitRule{}, |
|
||||||
&rule.CyclomaticRule{}, |
|
||||||
&rule.FileHeaderRule{}, |
|
||||||
&rule.EmptyBlockRule{}, |
|
||||||
&rule.SuperfluousElseRule{}, |
|
||||||
&rule.ConfusingNamingRule{}, |
|
||||||
&rule.GetReturnRule{}, |
|
||||||
&rule.ModifiesParamRule{}, |
|
||||||
&rule.ConfusingResultsRule{}, |
|
||||||
&rule.DeepExitRule{}, |
|
||||||
&rule.UnusedParamRule{}, |
|
||||||
&rule.UnreachableCodeRule{}, |
|
||||||
&rule.AddConstantRule{}, |
|
||||||
&rule.FlagParamRule{}, |
|
||||||
&rule.UnnecessaryStmtRule{}, |
|
||||||
&rule.StructTagRule{}, |
|
||||||
&rule.ModifiesValRecRule{}, |
|
||||||
&rule.ConstantLogicalExprRule{}, |
|
||||||
&rule.BoolLiteralRule{}, |
|
||||||
&rule.RedefinesBuiltinIDRule{}, |
|
||||||
&rule.ImportsBlacklistRule{}, |
|
||||||
&rule.FunctionResultsLimitRule{}, |
|
||||||
&rule.MaxPublicStructsRule{}, |
|
||||||
&rule.RangeValInClosureRule{}, |
|
||||||
&rule.RangeValAddress{}, |
|
||||||
&rule.WaitGroupByValueRule{}, |
|
||||||
&rule.AtomicRule{}, |
|
||||||
&rule.EmptyLinesRule{}, |
|
||||||
&rule.LineLengthLimitRule{}, |
|
||||||
&rule.CallToGCRule{}, |
|
||||||
&rule.DuplicatedImportsRule{}, |
|
||||||
&rule.ImportShadowingRule{}, |
|
||||||
&rule.BareReturnRule{}, |
|
||||||
&rule.UnusedReceiverRule{}, |
|
||||||
&rule.UnhandledErrorRule{}, |
|
||||||
&rule.CognitiveComplexityRule{}, |
|
||||||
&rule.StringOfIntRule{}, |
|
||||||
}, defaultRules...) |
|
||||||
|
|
||||||
var allFormatters = []lint.Formatter{ |
|
||||||
&formatter.Stylish{}, |
|
||||||
&formatter.Friendly{}, |
|
||||||
&formatter.JSON{}, |
|
||||||
&formatter.NDJSON{}, |
|
||||||
&formatter.Default{}, |
|
||||||
&formatter.Unix{}, |
|
||||||
&formatter.Checkstyle{}, |
|
||||||
&formatter.Plain{}, |
|
||||||
} |
|
||||||
|
|
||||||
func getFormatters() map[string]lint.Formatter { |
|
||||||
result := map[string]lint.Formatter{} |
|
||||||
for _, f := range allFormatters { |
|
||||||
result[f.Name()] = f |
|
||||||
} |
|
||||||
return result |
|
||||||
} |
|
||||||
|
|
||||||
func getLintingRules(config *lint.Config) []lint.Rule { |
|
||||||
rulesMap := map[string]lint.Rule{} |
|
||||||
for _, r := range allRules { |
|
||||||
rulesMap[r.Name()] = r |
|
||||||
} |
|
||||||
|
|
||||||
lintingRules := []lint.Rule{} |
|
||||||
for name := range config.Rules { |
|
||||||
rule, ok := rulesMap[name] |
|
||||||
if !ok { |
|
||||||
fail("cannot find rule: " + name) |
|
||||||
} |
|
||||||
lintingRules = append(lintingRules, rule) |
|
||||||
} |
|
||||||
|
|
||||||
return lintingRules |
|
||||||
} |
|
||||||
|
|
||||||
func parseConfig(path string) *lint.Config { |
|
||||||
config := &lint.Config{} |
|
||||||
file, err := ioutil.ReadFile(path) |
|
||||||
if err != nil { |
|
||||||
fail("cannot read the config file") |
|
||||||
} |
|
||||||
err = toml.Unmarshal(file, config) |
|
||||||
if err != nil { |
|
||||||
fail("cannot parse the config file: " + err.Error()) |
|
||||||
} |
|
||||||
return config |
|
||||||
} |
|
||||||
|
|
||||||
func normalizeConfig(config *lint.Config) { |
|
||||||
if config.Confidence == 0 { |
|
||||||
config.Confidence = 0.8 |
|
||||||
} |
|
||||||
severity := config.Severity |
|
||||||
if severity != "" { |
|
||||||
for k, v := range config.Rules { |
|
||||||
if v.Severity == "" { |
|
||||||
v.Severity = severity |
|
||||||
} |
|
||||||
config.Rules[k] = v |
|
||||||
} |
|
||||||
for k, v := range config.Directives { |
|
||||||
if v.Severity == "" { |
|
||||||
v.Severity = severity |
|
||||||
} |
|
||||||
config.Directives[k] = v |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
func getConfig() *lint.Config { |
|
||||||
config := defaultConfig() |
|
||||||
if configPath != "" { |
|
||||||
config = parseConfig(configPath) |
|
||||||
} |
|
||||||
normalizeConfig(config) |
|
||||||
return config |
|
||||||
} |
|
||||||
|
|
||||||
func getFormatter() lint.Formatter { |
|
||||||
formatters := getFormatters() |
|
||||||
formatter := formatters["default"] |
|
||||||
if formatterName != "" { |
|
||||||
f, ok := formatters[formatterName] |
|
||||||
if !ok { |
|
||||||
fail("unknown formatter " + formatterName) |
|
||||||
} |
|
||||||
formatter = f |
|
||||||
} |
|
||||||
return formatter |
|
||||||
} |
|
||||||
|
|
||||||
func buildDefaultConfigPath() string { |
|
||||||
var result string |
|
||||||
if homeDir, err := homedir.Dir(); err == nil { |
|
||||||
result = filepath.Join(homeDir, "revive.toml") |
|
||||||
if _, err := os.Stat(result); err != nil { |
|
||||||
result = "" |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return result |
|
||||||
} |
|
||||||
|
|
||||||
func defaultConfig() *lint.Config { |
|
||||||
defaultConfig := lint.Config{ |
|
||||||
Confidence: 0.0, |
|
||||||
Severity: lint.SeverityWarning, |
|
||||||
Rules: map[string]lint.RuleConfig{}, |
|
||||||
} |
|
||||||
for _, r := range defaultRules { |
|
||||||
defaultConfig.Rules[r.Name()] = lint.RuleConfig{} |
|
||||||
} |
|
||||||
return &defaultConfig |
|
||||||
} |
|
||||||
|
|
||||||
func normalizeSplit(strs []string) []string { |
|
||||||
res := []string{} |
|
||||||
for _, s := range strs { |
|
||||||
t := strings.Trim(s, " \t") |
|
||||||
if len(t) > 0 { |
|
||||||
res = append(res, t) |
|
||||||
} |
|
||||||
} |
|
||||||
return res |
|
||||||
} |
|
||||||
|
|
||||||
func getPackages() [][]string { |
|
||||||
globs := normalizeSplit(flag.Args()) |
|
||||||
if len(globs) == 0 { |
|
||||||
globs = append(globs, ".") |
|
||||||
} |
|
||||||
|
|
||||||
packages, err := dots.ResolvePackages(globs, normalizeSplit(excludePaths)) |
|
||||||
if err != nil { |
|
||||||
fail(err.Error()) |
|
||||||
} |
|
||||||
|
|
||||||
return packages |
|
||||||
} |
|
||||||
|
|
||||||
type arrayFlags []string |
|
||||||
|
|
||||||
func (i *arrayFlags) String() string { |
|
||||||
return strings.Join([]string(*i), " ") |
|
||||||
} |
|
||||||
|
|
||||||
func (i *arrayFlags) Set(value string) error { |
|
||||||
*i = append(*i, value) |
|
||||||
return nil |
|
||||||
} |
|
||||||
|
|
||||||
var configPath string |
|
||||||
var excludePaths arrayFlags |
|
||||||
var formatterName string |
|
||||||
var help bool |
|
||||||
|
|
||||||
var originalUsage = flag.Usage |
|
||||||
|
|
||||||
func init() { |
|
||||||
flag.Usage = func() { |
|
||||||
originalUsage() |
|
||||||
} |
|
||||||
// command line help strings
|
|
||||||
const ( |
|
||||||
configUsage = "path to the configuration TOML file, defaults to $HOME/revive.toml, if present (i.e. -config myconf.toml)" |
|
||||||
excludeUsage = "list of globs which specify files to be excluded (i.e. -exclude foo/...)" |
|
||||||
formatterUsage = "formatter to be used for the output (i.e. -formatter stylish)" |
|
||||||
) |
|
||||||
|
|
||||||
defaultConfigPath := buildDefaultConfigPath() |
|
||||||
|
|
||||||
flag.StringVar(&configPath, "config", defaultConfigPath, configUsage) |
|
||||||
flag.Var(&excludePaths, "exclude", excludeUsage) |
|
||||||
flag.StringVar(&formatterName, "formatter", "", formatterUsage) |
|
||||||
flag.Parse() |
|
||||||
} |
|
||||||
|
|
||||||
func main() { |
|
||||||
config := getConfig() |
|
||||||
formatter := getFormatter() |
|
||||||
packages := getPackages() |
|
||||||
|
|
||||||
revive := lint.New(func(file string) ([]byte, error) { |
|
||||||
return ioutil.ReadFile(file) |
|
||||||
}) |
|
||||||
|
|
||||||
lintingRules := getLintingRules(config) |
|
||||||
|
|
||||||
failures, err := revive.Lint(packages, lintingRules, *config) |
|
||||||
if err != nil { |
|
||||||
fail(err.Error()) |
|
||||||
} |
|
||||||
|
|
||||||
formatChan := make(chan lint.Failure) |
|
||||||
exitChan := make(chan bool) |
|
||||||
|
|
||||||
var output string |
|
||||||
go (func() { |
|
||||||
output, err = formatter.Format(formatChan, *config) |
|
||||||
if err != nil { |
|
||||||
fail(err.Error()) |
|
||||||
} |
|
||||||
exitChan <- true |
|
||||||
})() |
|
||||||
|
|
||||||
exitCode := 0 |
|
||||||
for f := range failures { |
|
||||||
if f.Confidence < config.Confidence { |
|
||||||
continue |
|
||||||
} |
|
||||||
if exitCode == 0 { |
|
||||||
exitCode = config.WarningCode |
|
||||||
} |
|
||||||
if c, ok := config.Rules[f.RuleName]; ok && c.Severity == lint.SeverityError { |
|
||||||
exitCode = config.ErrorCode |
|
||||||
} |
|
||||||
if c, ok := config.Directives[f.RuleName]; ok && c.Severity == lint.SeverityError { |
|
||||||
exitCode = config.ErrorCode |
|
||||||
} |
|
||||||
|
|
||||||
formatChan <- f |
|
||||||
} |
|
||||||
|
|
||||||
close(formatChan) |
|
||||||
<-exitChan |
|
||||||
if output != "" { |
|
||||||
fmt.Println(output) |
|
||||||
} |
|
||||||
|
|
||||||
os.Exit(exitCode) |
|
||||||
} |
|
@ -1,3 +0,0 @@ |
|||||||
*.test |
|
||||||
*.out |
|
||||||
.devcontainer/ |
|
@ -1,21 +0,0 @@ |
|||||||
MIT License |
|
||||||
|
|
||||||
Copyright (c) 2021 Salvador Cavadini |
|
||||||
|
|
||||||
Permission is hereby granted, free of charge, to any person obtaining a copy |
|
||||||
of this software and associated documentation files (the "Software"), to deal |
|
||||||
in the Software without restriction, including without limitation the rights |
|
||||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell |
|
||||||
copies of the Software, and to permit persons to whom the Software is |
|
||||||
furnished to do so, subject to the following conditions: |
|
||||||
|
|
||||||
The above copyright notice and this permission notice shall be included in all |
|
||||||
copies or substantial portions of the Software. |
|
||||||
|
|
||||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR |
|
||||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, |
|
||||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE |
|
||||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER |
|
||||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, |
|
||||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE |
|
||||||
SOFTWARE. |
|
@ -1,52 +0,0 @@ |
|||||||
# garif |
|
||||||
|
|
||||||
A GO package to create and manipulate SARIF logs. |
|
||||||
|
|
||||||
SARIF, from _Static Analysis Results Interchange Format_, is a standard JSON-based format for the output of static analysis tools defined and promoted by [OASIS](https://www.oasis-open.org/). |
|
||||||
|
|
||||||
Current supported version of the standard is [SARIF-v2.1.0](https://docs.oasis-open.org/sarif/sarif/v2.1.0/csprd01/sarif-v2.1.0-csprd01.html |
|
||||||
). |
|
||||||
|
|
||||||
## Usage |
|
||||||
|
|
||||||
The package provides access to every element of the SARIF model, therefore you are free to manipulate it at every detail. |
|
||||||
|
|
||||||
The package also provides constructors functions (`New...`) and decorators methods (`With...`) that simplify the creation of SARIF files for common use cases. |
|
||||||
|
|
||||||
Using these constructors and decorators we can easily create the example SARIF file of the [Microsoft SARIF pages](https://github.com/microsoft/sarif-tutorials/blob/master/docs/1-Introduction.md) |
|
||||||
|
|
||||||
|
|
||||||
```go |
|
||||||
import to `github.com/chavacava/garif` |
|
||||||
|
|
||||||
// ... |
|
||||||
|
|
||||||
rule := garif.NewRule("no-unused-vars"). |
|
||||||
WithHelpUri("https://eslint.org/docs/rules/no-unused-vars"). |
|
||||||
WithShortDescription("disallow unused variables"). |
|
||||||
WithProperties("category", "Variables") |
|
||||||
|
|
||||||
driver := garif.NewDriver("ESLint"). |
|
||||||
WithInformationUri("https://eslint.org"). |
|
||||||
WithRules(rule) |
|
||||||
|
|
||||||
run := garif.NewRun(NewTool(driver)). |
|
||||||
WithArtifactsURIs("file:///C:/dev/sarif/sarif-tutorials/samples/Introduction/simple-example.js") |
|
||||||
|
|
||||||
run.WithResult(rule.Id, "'x' is assigned a value but never used.", "file:///C:/dev/sarif/sarif-tutorials/samples/Introduction/simple-example.js", 1, 5) |
|
||||||
|
|
||||||
logFile := garif.NewLogFile([]*Run{run}, Version210) |
|
||||||
|
|
||||||
logFile.Write(os.Stdout) |
|
||||||
``` |
|
||||||
|
|
||||||
## Why this package? |
|
||||||
This package was initiated during my works on adding to [`revive`](https://github.com/mgechev/revive) a SARIF output formatter. |
|
||||||
I've tried to use [go-sarif](https://github.com/owenrumney/go-sarif) by [Owen Rumney](https://github.com/owenrumney) but it is too focused in the use case of the static analyzer [tfsec](https://tfsec.dev) so I've decided to create a package flexible enough to generate SARIF files in broader cases. |
|
||||||
|
|
||||||
## More information about SARIF |
|
||||||
For more information about SARIF, you can visit the [Oasis Open](https://www.oasis-open.org/committees/tc_home.php?wg_abbrev=sarif) site. |
|
||||||
|
|
||||||
|
|
||||||
## Contributing |
|
||||||
Of course, contributions are welcome! |
|
@ -1,338 +0,0 @@ |
|||||||
package garif |
|
||||||
|
|
||||||
// NewAddress creates a valid Address
|
|
||||||
func NewAddress() *Address { |
|
||||||
return &Address{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewArtifact creates a valid Artifact
|
|
||||||
func NewArtifact() *Artifact { |
|
||||||
return &Artifact{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewArtifactChange creates a valid ArtifactChange
|
|
||||||
func NewArtifactChange(location *ArtifactLocation, replacements ...*Replacement) *ArtifactChange { |
|
||||||
return &ArtifactChange{ |
|
||||||
ArtifactLocation: location, |
|
||||||
Replacements: replacements, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewArtifactContent creates a valid ArtifactContent
|
|
||||||
func NewArtifactContent() *ArtifactContent { |
|
||||||
return &ArtifactContent{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewArtifactLocation creates a valid ArtifactLocation
|
|
||||||
func NewArtifactLocation() *ArtifactLocation { |
|
||||||
return &ArtifactLocation{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewAttachment creates a valid Attachment
|
|
||||||
func NewAttachment(location *ArtifactLocation) *Attachment { |
|
||||||
return &Attachment{ArtifactLocation: location} |
|
||||||
} |
|
||||||
|
|
||||||
// NewCodeFlow creates a valid CodeFlow
|
|
||||||
func NewCodeFlow(threadFlows ...*ThreadFlow) *CodeFlow { |
|
||||||
return &CodeFlow{ThreadFlows: threadFlows} |
|
||||||
} |
|
||||||
|
|
||||||
// NewConfigurationOverride creates a valid ConfigurationOverride
|
|
||||||
func NewConfigurationOverride(configuration *ReportingConfiguration, descriptor *ReportingDescriptorReference) *ConfigurationOverride { |
|
||||||
return &ConfigurationOverride{ |
|
||||||
Configuration: configuration, |
|
||||||
Descriptor: descriptor, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewConversion creates a valid Conversion
|
|
||||||
func NewConversion(tool *Tool) *Conversion { |
|
||||||
return &Conversion{Tool: tool} |
|
||||||
} |
|
||||||
|
|
||||||
// NewEdge creates a valid Edge
|
|
||||||
func NewEdge(id, sourceNodeId, targetNodeId string) *Edge { |
|
||||||
return &Edge{ |
|
||||||
Id: id, |
|
||||||
SourceNodeId: sourceNodeId, |
|
||||||
TargetNodeId: targetNodeId, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewEdgeTraversal creates a valid EdgeTraversal
|
|
||||||
func NewEdgeTraversal(edgeId string) *EdgeTraversal { |
|
||||||
return &EdgeTraversal{ |
|
||||||
EdgeId: edgeId, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewException creates a valid Exception
|
|
||||||
func NewException() *Exception { |
|
||||||
return &Exception{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewExternalProperties creates a valid ExternalProperties
|
|
||||||
func NewExternalProperties() *ExternalProperties { |
|
||||||
return &ExternalProperties{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewExternalPropertyFileReference creates a valid ExternalPropertyFileReference
|
|
||||||
func NewExternalPropertyFileReference() *ExternalPropertyFileReference { |
|
||||||
return &ExternalPropertyFileReference{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewExternalPropertyFileReferences creates a valid ExternalPropertyFileReferences
|
|
||||||
func NewExternalPropertyFileReferences() *ExternalPropertyFileReferences { |
|
||||||
return &ExternalPropertyFileReferences{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewFix creates a valid Fix
|
|
||||||
func NewFix(artifactChanges ...*ArtifactChange) *Fix { |
|
||||||
return &Fix{ |
|
||||||
ArtifactChanges: artifactChanges, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewGraph creates a valid Graph
|
|
||||||
func NewGraph() *Graph { |
|
||||||
return &Graph{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewGraphTraversal creates a valid GraphTraversal
|
|
||||||
func NewGraphTraversal() *GraphTraversal { |
|
||||||
return &GraphTraversal{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewInvocation creates a valid Invocation
|
|
||||||
func NewInvocation(executionSuccessful bool) *Invocation { |
|
||||||
return &Invocation{ |
|
||||||
ExecutionSuccessful: executionSuccessful, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewLocation creates a valid Location
|
|
||||||
func NewLocation() *Location { |
|
||||||
return &Location{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewLocationRelationship creates a valid LocationRelationship
|
|
||||||
func NewLocationRelationship(target int) *LocationRelationship { |
|
||||||
return &LocationRelationship{ |
|
||||||
Target: target, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
type LogFileVersion string |
|
||||||
|
|
||||||
const Version210 LogFileVersion = "2.1.0" |
|
||||||
|
|
||||||
// NewLogFile creates a valid LogFile
|
|
||||||
func NewLogFile(runs []*Run, version LogFileVersion) *LogFile { |
|
||||||
return &LogFile{ |
|
||||||
Runs: runs, |
|
||||||
Version: version, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewLogicalLocation creates a valid LogicalLocation
|
|
||||||
func NewLogicalLocation() *LogicalLocation { |
|
||||||
return &LogicalLocation{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewMessage creates a valid Message
|
|
||||||
func NewMessage() *Message { |
|
||||||
return &Message{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewMessageFromText creates a valid Message with the given text
|
|
||||||
func NewMessageFromText(text string) *Message { |
|
||||||
return &Message{ |
|
||||||
Text: text, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewMultiformatMessageString creates a valid MultiformatMessageString
|
|
||||||
func NewMultiformatMessageString(text string) *MultiformatMessageString { |
|
||||||
return &MultiformatMessageString{ |
|
||||||
Text: text, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewNode creates a valid Node
|
|
||||||
func NewNode(id string) *Node { |
|
||||||
return &Node{ |
|
||||||
Id: id, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewNotification creates a valid Notification
|
|
||||||
func NewNotification(message *Message) *Notification { |
|
||||||
return &Notification{ |
|
||||||
Message: message, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewPhysicalLocation creates a valid PhysicalLocation
|
|
||||||
func NewPhysicalLocation() *PhysicalLocation { |
|
||||||
return &PhysicalLocation{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewPropertyBag creates a valid PropertyBag
|
|
||||||
func NewPropertyBag() *PropertyBag { |
|
||||||
return &PropertyBag{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewRectangle creates a valid Rectangle
|
|
||||||
func NewRectangle() *Rectangle { |
|
||||||
return &Rectangle{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewRegion creates a valid Region
|
|
||||||
func NewRegion() *Region { |
|
||||||
return &Region{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewReplacement creates a valid Replacement
|
|
||||||
func NewReplacement(deletedRegion *Region) *Replacement { |
|
||||||
return &Replacement{ |
|
||||||
DeletedRegion: deletedRegion, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewReportingConfiguration creates a valid ReportingConfiguration
|
|
||||||
func NewReportingConfiguration() *ReportingConfiguration { |
|
||||||
return &ReportingConfiguration{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewReportingDescriptor creates a valid ReportingDescriptor
|
|
||||||
func NewReportingDescriptor(id string) *ReportingDescriptor { |
|
||||||
return &ReportingDescriptor{ |
|
||||||
Id: id, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewRule is an alias for NewReportingDescriptor
|
|
||||||
func NewRule(id string) *ReportingDescriptor { |
|
||||||
return NewReportingDescriptor(id) |
|
||||||
} |
|
||||||
|
|
||||||
// NewReportingDescriptorReference creates a valid ReportingDescriptorReference
|
|
||||||
func NewReportingDescriptorReference() *ReportingDescriptorReference { |
|
||||||
return &ReportingDescriptorReference{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewReportingDescriptorRelationship creates a valid ReportingDescriptorRelationship
|
|
||||||
func NewReportingDescriptorRelationship(target *ReportingDescriptorReference) *ReportingDescriptorRelationship { |
|
||||||
return &ReportingDescriptorRelationship{ |
|
||||||
Target: target, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewResult creates a valid Result
|
|
||||||
func NewResult(message *Message) *Result { |
|
||||||
return &Result{ |
|
||||||
Message: message, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewResultProvenance creates a valid ResultProvenance
|
|
||||||
func NewResultProvenance() *ResultProvenance { |
|
||||||
return &ResultProvenance{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewRun creates a valid Run
|
|
||||||
func NewRun(tool *Tool) *Run { |
|
||||||
return &Run{ |
|
||||||
Tool: tool, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewRunAutomationDetails creates a valid RunAutomationDetails
|
|
||||||
func NewRunAutomationDetails() *RunAutomationDetails { |
|
||||||
return &RunAutomationDetails{} |
|
||||||
} |
|
||||||
|
|
||||||
// New creates a valid
|
|
||||||
func NewSpecialLocations() *SpecialLocations { |
|
||||||
return &SpecialLocations{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewStack creates a valid Stack
|
|
||||||
func NewStack(frames ...*StackFrame) *Stack { |
|
||||||
return &Stack{ |
|
||||||
Frames: frames, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewStackFrame creates a valid StackFrame
|
|
||||||
func NewStackFrame() *StackFrame { |
|
||||||
return &StackFrame{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewSuppression creates a valid Suppression
|
|
||||||
func NewSuppression(kind string) *Suppression { |
|
||||||
return &Suppression{ |
|
||||||
Kind: kind, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewThreadFlow creates a valid ThreadFlow
|
|
||||||
func NewThreadFlow(locations []*ThreadFlowLocation) *ThreadFlow { |
|
||||||
return &ThreadFlow{ |
|
||||||
Locations: locations, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewThreadFlowLocation creates a valid ThreadFlowLocation
|
|
||||||
func NewThreadFlowLocation() *ThreadFlowLocation { |
|
||||||
return &ThreadFlowLocation{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewTool creates a valid Tool
|
|
||||||
func NewTool(driver *ToolComponent) *Tool { |
|
||||||
return &Tool{ |
|
||||||
Driver: driver, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewToolComponent creates a valid ToolComponent
|
|
||||||
func NewToolComponent(name string) *ToolComponent { |
|
||||||
return &ToolComponent{ |
|
||||||
Name: name, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewDriver is an alias for NewToolComponent
|
|
||||||
func NewDriver(name string) *ToolComponent { |
|
||||||
return NewToolComponent(name) |
|
||||||
} |
|
||||||
|
|
||||||
// NewToolComponentReference creates a valid ToolComponentReference
|
|
||||||
func NewToolComponentReference() *ToolComponentReference { |
|
||||||
return &ToolComponentReference{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewTranslationMetadata creates a valid TranslationMetadata
|
|
||||||
func NewTranslationMetadata(name string) *TranslationMetadata { |
|
||||||
return &TranslationMetadata{ |
|
||||||
Name: name, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewVersionControlDetails creates a valid VersionControlDetails
|
|
||||||
func NewVersionControlDetails(repositoryUri string) *VersionControlDetails { |
|
||||||
return &VersionControlDetails{ |
|
||||||
RepositoryUri: repositoryUri, |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// NewWebRequest creates a valid WebRequest
|
|
||||||
func NewWebRequest() *WebRequest { |
|
||||||
return &WebRequest{} |
|
||||||
} |
|
||||||
|
|
||||||
// NewWebResponse creates a valid WebResponse
|
|
||||||
func NewWebResponse() *WebResponse { |
|
||||||
return &WebResponse{} |
|
||||||
} |
|
@ -1,94 +0,0 @@ |
|||||||
package garif |
|
||||||
|
|
||||||
// WithLineColumn sets a physical location with the given line and column
|
|
||||||
func (l *Location) WithLineColumn(line, column int) *Location { |
|
||||||
if l.PhysicalLocation == nil { |
|
||||||
l.PhysicalLocation = NewPhysicalLocation() |
|
||||||
} |
|
||||||
|
|
||||||
l.PhysicalLocation.Region = NewRegion() |
|
||||||
l.PhysicalLocation.Region.StartLine = line |
|
||||||
l.PhysicalLocation.Region.StartColumn = column |
|
||||||
|
|
||||||
return l |
|
||||||
} |
|
||||||
|
|
||||||
// WithURI sets a physical location with the given URI
|
|
||||||
func (l *Location) WithURI(uri string) *Location { |
|
||||||
if l.PhysicalLocation == nil { |
|
||||||
l.PhysicalLocation = NewPhysicalLocation() |
|
||||||
} |
|
||||||
|
|
||||||
l.PhysicalLocation.ArtifactLocation = NewArtifactLocation() |
|
||||||
l.PhysicalLocation.ArtifactLocation.Uri = uri |
|
||||||
|
|
||||||
return l |
|
||||||
} |
|
||||||
|
|
||||||
// WithKeyValue sets (overwrites) the value of the given key
|
|
||||||
func (b PropertyBag) WithKeyValue(key string, value interface{}) PropertyBag { |
|
||||||
b[key] = value |
|
||||||
return b |
|
||||||
} |
|
||||||
|
|
||||||
// WithHelpUri sets the help URI for this ReportingDescriptor
|
|
||||||
func (r *ReportingDescriptor) WithHelpUri(uri string) *ReportingDescriptor { |
|
||||||
r.HelpUri = uri |
|
||||||
return r |
|
||||||
} |
|
||||||
|
|
||||||
// WithProperties adds the key & value to the properties of this ReportingDescriptor
|
|
||||||
func (r *ReportingDescriptor) WithProperties(key string, value interface{}) *ReportingDescriptor { |
|
||||||
if r.Properties == nil { |
|
||||||
r.Properties = NewPropertyBag() |
|
||||||
} |
|
||||||
|
|
||||||
r.Properties.WithKeyValue(key, value) |
|
||||||
|
|
||||||
return r |
|
||||||
} |
|
||||||
|
|
||||||
// WithArtifactsURIs adds the given URI as artifacts of this Run
|
|
||||||
func (r *Run) WithArtifactsURIs(uris ...string) *Run { |
|
||||||
if r.Artifacts == nil { |
|
||||||
r.Artifacts = []*Artifact{} |
|
||||||
} |
|
||||||
|
|
||||||
for _, uri := range uris { |
|
||||||
a := NewArtifact() |
|
||||||
a.Location = NewArtifactLocation() |
|
||||||
a.Location.Uri = uri |
|
||||||
r.Artifacts = append(r.Artifacts, a) |
|
||||||
} |
|
||||||
|
|
||||||
return r |
|
||||||
} |
|
||||||
|
|
||||||
// WithResult adds a result to this Run
|
|
||||||
func (r *Run) WithResult(ruleId string, message string, uri string, line int, column int) *Run { |
|
||||||
if r.Results == nil { |
|
||||||
r.Results = []*Result{} |
|
||||||
} |
|
||||||
|
|
||||||
msg := NewMessage() |
|
||||||
msg.Text = message |
|
||||||
result := NewResult(msg) |
|
||||||
location := NewLocation().WithURI(uri).WithLineColumn(line, column) |
|
||||||
|
|
||||||
result.Locations = append(result.Locations, location) |
|
||||||
result.RuleId = ruleId |
|
||||||
r.Results = append(r.Results, result) |
|
||||||
return r |
|
||||||
} |
|
||||||
|
|
||||||
// WithInformationUri sets the information URI
|
|
||||||
func (t *ToolComponent) WithInformationUri(uri string) *ToolComponent { |
|
||||||
t.InformationUri = uri |
|
||||||
return t |
|
||||||
} |
|
||||||
|
|
||||||
// WithRules sets (overwrites) the rules
|
|
||||||
func (t *ToolComponent) WithRules(rules ...*ReportingDescriptor) *ToolComponent { |
|
||||||
t.Rules = rules |
|
||||||
return t |
|
||||||
} |
|
@ -1,11 +0,0 @@ |
|||||||
// Package garif defines all the GO structures required to model a SARIF log file.
|
|
||||||
// These structures were created using the JSON-schema sarif-schema-2.1.0.json of SARIF logfiles
|
|
||||||
// available at https://github.com/oasis-tcs/sarif-spec/tree/master/Schemata.
|
|
||||||
//
|
|
||||||
// The package provides constructors for all structures (see constructors.go) These constructors
|
|
||||||
// ensure that the returned structure instantiation is valid with respect to the JSON schema and
|
|
||||||
// should be used in place of plain structure instantiation.
|
|
||||||
// The root structure is LogFile.
|
|
||||||
//
|
|
||||||
// The package provides utility decorators for the most commonly used structures (see decorators.go)
|
|
||||||
package garif |
|
@ -1,5 +0,0 @@ |
|||||||
module github.com/chavacava/garif |
|
||||||
|
|
||||||
go 1.16 |
|
||||||
|
|
||||||
require github.com/stretchr/testify v1.7.0 |
|
@ -1,11 +0,0 @@ |
|||||||
github.com/davecgh/go-spew v1.1.0 h1:ZDRjVQ15GmhC3fiQ8ni8+OwkZQO4DARzQgrnXU1Liz8= |
|
||||||
github.com/davecgh/go-spew v1.1.0/go.mod h1:J7Y8YcW2NihsgmVo/mv3lAwl/skON4iLHjSsI+c5H38= |
|
||||||
github.com/pmezard/go-difflib v1.0.0 h1:4DBwDE0NGyQoBHbLQYPwSUPoCMWR5BEzIk/f1lZbAQM= |
|
||||||
github.com/pmezard/go-difflib v1.0.0/go.mod h1:iKH77koFhYxTK1pcRnkKkqfTogsbg7gZNVY4sRDYZ/4= |
|
||||||
github.com/stretchr/objx v0.1.0/go.mod h1:HFkY916IF+rwdDfMAkV7OtwuqBVzrE8GR6GFx+wExME= |
|
||||||
github.com/stretchr/testify v1.7.0 h1:nwc3DEeHmmLAfoZucVR881uASk0Mfjw8xYJ99tb5CcY= |
|
||||||
github.com/stretchr/testify v1.7.0/go.mod h1:6Fq8oRcR53rry900zMqJjRRixrwX3KX962/h/Wwjteg= |
|
||||||
gopkg.in/check.v1 v0.0.0-20161208181325-20d25e280405 h1:yhCVgyC4o1eVCa2tZl7eS0r+SDo693bJlVdllGtEeKM= |
|
||||||
gopkg.in/check.v1 v0.0.0-20161208181325-20d25e280405/go.mod h1:Co6ibVJAznAaIkqp8huTwlJQCZ016jof/cbN4VW5Yz0= |
|
||||||
gopkg.in/yaml.v3 v3.0.0-20200313102051-9f266ea9e77c h1:dUUwHk2QECo/6vqA44rthZ8ie2QXMNeKRTHCNY2nXvo= |
|
||||||
gopkg.in/yaml.v3 v3.0.0-20200313102051-9f266ea9e77c/go.mod h1:K4uyk7z7BCEPqu6E+C64Yfv1cQ7kz7rIZviUmN+EgEM= |
|
@ -1,26 +0,0 @@ |
|||||||
package garif |
|
||||||
|
|
||||||
import ( |
|
||||||
"encoding/json" |
|
||||||
"io" |
|
||||||
) |
|
||||||
|
|
||||||
// Write writes the JSON
|
|
||||||
func (l *LogFile) Write(w io.Writer) error { |
|
||||||
marshal, err := json.Marshal(l) |
|
||||||
if err != nil { |
|
||||||
return err |
|
||||||
} |
|
||||||
_, err = w.Write(marshal) |
|
||||||
return err |
|
||||||
} |
|
||||||
|
|
||||||
// PrettyWrite writes indented JSON
|
|
||||||
func (l *LogFile) PrettyWrite(w io.Writer) error { |
|
||||||
marshal, err := json.MarshalIndent(l, "", " ") |
|
||||||
if err != nil { |
|
||||||
return err |
|
||||||
} |
|
||||||
_, err = w.Write(marshal) |
|
||||||
return err |
|
||||||
} |
|
File diff suppressed because it is too large
Load Diff
@ -1,20 +0,0 @@ |
|||||||
The MIT License (MIT) |
|
||||||
|
|
||||||
Copyright (c) 2013 Fatih Arslan |
|
||||||
|
|
||||||
Permission is hereby granted, free of charge, to any person obtaining a copy of |
|
||||||
this software and associated documentation files (the "Software"), to deal in |
|
||||||
the Software without restriction, including without limitation the rights to |
|
||||||
use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of |
|
||||||
the Software, and to permit persons to whom the Software is furnished to do so, |
|
||||||
subject to the following conditions: |
|
||||||
|
|
||||||
The above copyright notice and this permission notice shall be included in all |
|
||||||
copies or substantial portions of the Software. |
|
||||||
|
|
||||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR |
|
||||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS |
|
||||||
FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR |
|
||||||
COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER |
|
||||||
IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN |
|
||||||
CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
|
@ -1,173 +0,0 @@ |
|||||||
# color [](https://github.com/fatih/color/actions) [](https://pkg.go.dev/github.com/fatih/color) |
|
||||||
|
|
||||||
Color lets you use colorized outputs in terms of [ANSI Escape |
|
||||||
Codes](http://en.wikipedia.org/wiki/ANSI_escape_code#Colors) in Go (Golang). It |
|
||||||
has support for Windows too! The API can be used in several ways, pick one that |
|
||||||
suits you. |
|
||||||
|
|
||||||
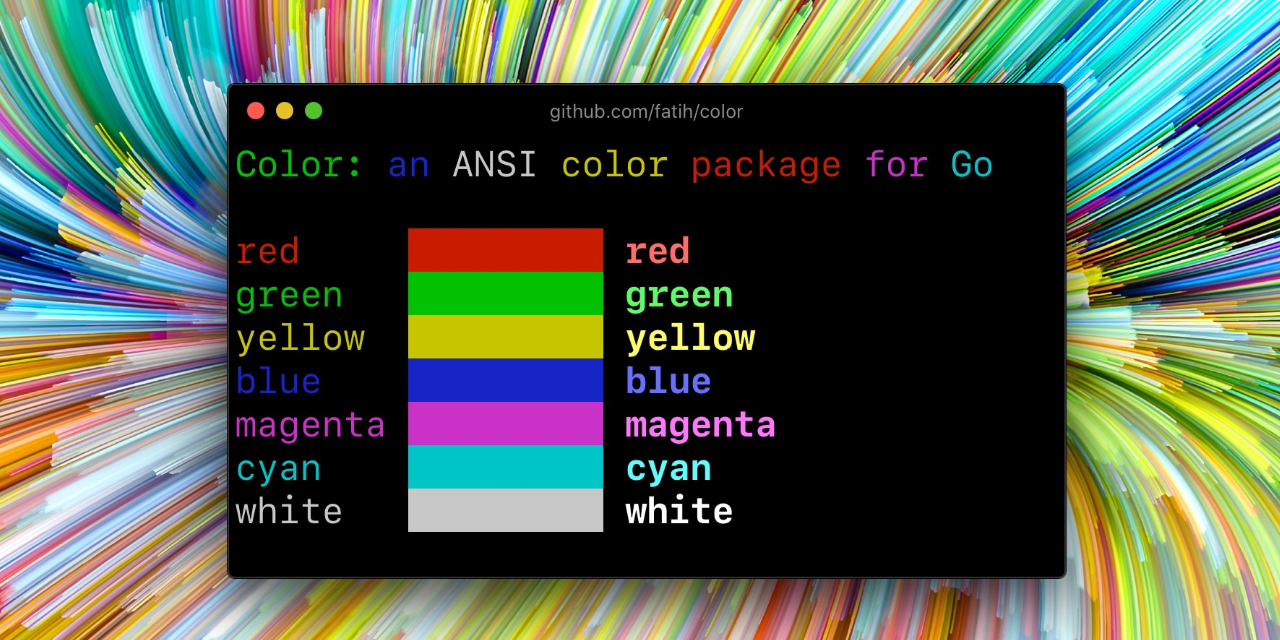 |
|
||||||
|
|
||||||
|
|
||||||
## Install |
|
||||||
|
|
||||||
```bash |
|
||||||
go get github.com/fatih/color |
|
||||||
``` |
|
||||||
|
|
||||||
## Examples |
|
||||||
|
|
||||||
### Standard colors |
|
||||||
|
|
||||||
```go |
|
||||||
// Print with default helper functions |
|
||||||
color.Cyan("Prints text in cyan.") |
|
||||||
|
|
||||||
// A newline will be appended automatically |
|
||||||
color.Blue("Prints %s in blue.", "text") |
|
||||||
|
|
||||||
// These are using the default foreground colors |
|
||||||
color.Red("We have red") |
|
||||||
color.Magenta("And many others ..") |
|
||||||
|
|
||||||
``` |
|
||||||
|
|
||||||
### Mix and reuse colors |
|
||||||
|
|
||||||
```go |
|
||||||
// Create a new color object |
|
||||||
c := color.New(color.FgCyan).Add(color.Underline) |
|
||||||
c.Println("Prints cyan text with an underline.") |
|
||||||
|
|
||||||
// Or just add them to New() |
|
||||||
d := color.New(color.FgCyan, color.Bold) |
|
||||||
d.Printf("This prints bold cyan %s\n", "too!.") |
|
||||||
|
|
||||||
// Mix up foreground and background colors, create new mixes! |
|
||||||
red := color.New(color.FgRed) |
|
||||||
|
|
||||||
boldRed := red.Add(color.Bold) |
|
||||||
boldRed.Println("This will print text in bold red.") |
|
||||||
|
|
||||||
whiteBackground := red.Add(color.BgWhite) |
|
||||||
whiteBackground.Println("Red text with white background.") |
|
||||||
``` |
|
||||||
|
|
||||||
### Use your own output (io.Writer) |
|
||||||
|
|
||||||
```go |
|
||||||
// Use your own io.Writer output |
|
||||||
color.New(color.FgBlue).Fprintln(myWriter, "blue color!") |
|
||||||
|
|
||||||
blue := color.New(color.FgBlue) |
|
||||||
blue.Fprint(writer, "This will print text in blue.") |
|
||||||
``` |
|
||||||
|
|
||||||
### Custom print functions (PrintFunc) |
|
||||||
|
|
||||||
```go |
|
||||||
// Create a custom print function for convenience |
|
||||||
red := color.New(color.FgRed).PrintfFunc() |
|
||||||
red("Warning") |
|
||||||
red("Error: %s", err) |
|
||||||
|
|
||||||
// Mix up multiple attributes |
|
||||||
notice := color.New(color.Bold, color.FgGreen).PrintlnFunc() |
|
||||||
notice("Don't forget this...") |
|
||||||
``` |
|
||||||
|
|
||||||
### Custom fprint functions (FprintFunc) |
|
||||||
|
|
||||||
```go |
|
||||||
blue := color.New(FgBlue).FprintfFunc() |
|
||||||
blue(myWriter, "important notice: %s", stars) |
|
||||||
|
|
||||||
// Mix up with multiple attributes |
|
||||||
success := color.New(color.Bold, color.FgGreen).FprintlnFunc() |
|
||||||
success(myWriter, "Don't forget this...") |
|
||||||
``` |
|
||||||
|
|
||||||
### Insert into noncolor strings (SprintFunc) |
|
||||||
|
|
||||||
```go |
|
||||||
// Create SprintXxx functions to mix strings with other non-colorized strings: |
|
||||||
yellow := color.New(color.FgYellow).SprintFunc() |
|
||||||
red := color.New(color.FgRed).SprintFunc() |
|
||||||
fmt.Printf("This is a %s and this is %s.\n", yellow("warning"), red("error")) |
|
||||||
|
|
||||||
info := color.New(color.FgWhite, color.BgGreen).SprintFunc() |
|
||||||
fmt.Printf("This %s rocks!\n", info("package")) |
|
||||||
|
|
||||||
// Use helper functions |
|
||||||
fmt.Println("This", color.RedString("warning"), "should be not neglected.") |
|
||||||
fmt.Printf("%v %v\n", color.GreenString("Info:"), "an important message.") |
|
||||||
|
|
||||||
// Windows supported too! Just don't forget to change the output to color.Output |
|
||||||
fmt.Fprintf(color.Output, "Windows support: %s", color.GreenString("PASS")) |
|
||||||
``` |
|
||||||
|
|
||||||
### Plug into existing code |
|
||||||
|
|
||||||
```go |
|
||||||
// Use handy standard colors |
|
||||||
color.Set(color.FgYellow) |
|
||||||
|
|
||||||
fmt.Println("Existing text will now be in yellow") |
|
||||||
fmt.Printf("This one %s\n", "too") |
|
||||||
|
|
||||||
color.Unset() // Don't forget to unset |
|
||||||
|
|
||||||
// You can mix up parameters |
|
||||||
color.Set(color.FgMagenta, color.Bold) |
|
||||||
defer color.Unset() // Use it in your function |
|
||||||
|
|
||||||
fmt.Println("All text will now be bold magenta.") |
|
||||||
``` |
|
||||||
|
|
||||||
### Disable/Enable color |
|
||||||
|
|
||||||
There might be a case where you want to explicitly disable/enable color output. the |
|
||||||
`go-isatty` package will automatically disable color output for non-tty output streams |
|
||||||
(for example if the output were piped directly to `less`) |
|
||||||
|
|
||||||
`Color` has support to disable/enable colors both globally and for single color |
|
||||||
definitions. For example suppose you have a CLI app and a `--no-color` bool flag. You |
|
||||||
can easily disable the color output with: |
|
||||||
|
|
||||||
```go |
|
||||||
|
|
||||||
var flagNoColor = flag.Bool("no-color", false, "Disable color output") |
|
||||||
|
|
||||||
if *flagNoColor { |
|
||||||
color.NoColor = true // disables colorized output |
|
||||||
} |
|
||||||
``` |
|
||||||
|
|
||||||
It also has support for single color definitions (local). You can |
|
||||||
disable/enable color output on the fly: |
|
||||||
|
|
||||||
```go |
|
||||||
c := color.New(color.FgCyan) |
|
||||||
c.Println("Prints cyan text") |
|
||||||
|
|
||||||
c.DisableColor() |
|
||||||
c.Println("This is printed without any color") |
|
||||||
|
|
||||||
c.EnableColor() |
|
||||||
c.Println("This prints again cyan...") |
|
||||||
``` |
|
||||||
|
|
||||||
## Todo |
|
||||||
|
|
||||||
* Save/Return previous values |
|
||||||
* Evaluate fmt.Formatter interface |
|
||||||
|
|
||||||
|
|
||||||
## Credits |
|
||||||
|
|
||||||
* [Fatih Arslan](https://github.com/fatih) |
|
||||||
* Windows support via @mattn: [colorable](https://github.com/mattn/go-colorable) |
|
||||||
|
|
||||||
## License |
|
||||||
|
|
||||||
The MIT License (MIT) - see [`LICENSE.md`](https://github.com/fatih/color/blob/master/LICENSE.md) for more details |
|
||||||
|
|
@ -1,603 +0,0 @@ |
|||||||
package color |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"io" |
|
||||||
"os" |
|
||||||
"strconv" |
|
||||||
"strings" |
|
||||||
"sync" |
|
||||||
|
|
||||||
"github.com/mattn/go-colorable" |
|
||||||
"github.com/mattn/go-isatty" |
|
||||||
) |
|
||||||
|
|
||||||
var ( |
|
||||||
// NoColor defines if the output is colorized or not. It's dynamically set to
|
|
||||||
// false or true based on the stdout's file descriptor referring to a terminal
|
|
||||||
// or not. This is a global option and affects all colors. For more control
|
|
||||||
// over each color block use the methods DisableColor() individually.
|
|
||||||
NoColor = os.Getenv("TERM") == "dumb" || |
|
||||||
(!isatty.IsTerminal(os.Stdout.Fd()) && !isatty.IsCygwinTerminal(os.Stdout.Fd())) |
|
||||||
|
|
||||||
// Output defines the standard output of the print functions. By default
|
|
||||||
// os.Stdout is used.
|
|
||||||
Output = colorable.NewColorableStdout() |
|
||||||
|
|
||||||
// Error defines a color supporting writer for os.Stderr.
|
|
||||||
Error = colorable.NewColorableStderr() |
|
||||||
|
|
||||||
// colorsCache is used to reduce the count of created Color objects and
|
|
||||||
// allows to reuse already created objects with required Attribute.
|
|
||||||
colorsCache = make(map[Attribute]*Color) |
|
||||||
colorsCacheMu sync.Mutex // protects colorsCache
|
|
||||||
) |
|
||||||
|
|
||||||
// Color defines a custom color object which is defined by SGR parameters.
|
|
||||||
type Color struct { |
|
||||||
params []Attribute |
|
||||||
noColor *bool |
|
||||||
} |
|
||||||
|
|
||||||
// Attribute defines a single SGR Code
|
|
||||||
type Attribute int |
|
||||||
|
|
||||||
const escape = "\x1b" |
|
||||||
|
|
||||||
// Base attributes
|
|
||||||
const ( |
|
||||||
Reset Attribute = iota |
|
||||||
Bold |
|
||||||
Faint |
|
||||||
Italic |
|
||||||
Underline |
|
||||||
BlinkSlow |
|
||||||
BlinkRapid |
|
||||||
ReverseVideo |
|
||||||
Concealed |
|
||||||
CrossedOut |
|
||||||
) |
|
||||||
|
|
||||||
// Foreground text colors
|
|
||||||
const ( |
|
||||||
FgBlack Attribute = iota + 30 |
|
||||||
FgRed |
|
||||||
FgGreen |
|
||||||
FgYellow |
|
||||||
FgBlue |
|
||||||
FgMagenta |
|
||||||
FgCyan |
|
||||||
FgWhite |
|
||||||
) |
|
||||||
|
|
||||||
// Foreground Hi-Intensity text colors
|
|
||||||
const ( |
|
||||||
FgHiBlack Attribute = iota + 90 |
|
||||||
FgHiRed |
|
||||||
FgHiGreen |
|
||||||
FgHiYellow |
|
||||||
FgHiBlue |
|
||||||
FgHiMagenta |
|
||||||
FgHiCyan |
|
||||||
FgHiWhite |
|
||||||
) |
|
||||||
|
|
||||||
// Background text colors
|
|
||||||
const ( |
|
||||||
BgBlack Attribute = iota + 40 |
|
||||||
BgRed |
|
||||||
BgGreen |
|
||||||
BgYellow |
|
||||||
BgBlue |
|
||||||
BgMagenta |
|
||||||
BgCyan |
|
||||||
BgWhite |
|
||||||
) |
|
||||||
|
|
||||||
// Background Hi-Intensity text colors
|
|
||||||
const ( |
|
||||||
BgHiBlack Attribute = iota + 100 |
|
||||||
BgHiRed |
|
||||||
BgHiGreen |
|
||||||
BgHiYellow |
|
||||||
BgHiBlue |
|
||||||
BgHiMagenta |
|
||||||
BgHiCyan |
|
||||||
BgHiWhite |
|
||||||
) |
|
||||||
|
|
||||||
// New returns a newly created color object.
|
|
||||||
func New(value ...Attribute) *Color { |
|
||||||
c := &Color{params: make([]Attribute, 0)} |
|
||||||
c.Add(value...) |
|
||||||
return c |
|
||||||
} |
|
||||||
|
|
||||||
// Set sets the given parameters immediately. It will change the color of
|
|
||||||
// output with the given SGR parameters until color.Unset() is called.
|
|
||||||
func Set(p ...Attribute) *Color { |
|
||||||
c := New(p...) |
|
||||||
c.Set() |
|
||||||
return c |
|
||||||
} |
|
||||||
|
|
||||||
// Unset resets all escape attributes and clears the output. Usually should
|
|
||||||
// be called after Set().
|
|
||||||
func Unset() { |
|
||||||
if NoColor { |
|
||||||
return |
|
||||||
} |
|
||||||
|
|
||||||
fmt.Fprintf(Output, "%s[%dm", escape, Reset) |
|
||||||
} |
|
||||||
|
|
||||||
// Set sets the SGR sequence.
|
|
||||||
func (c *Color) Set() *Color { |
|
||||||
if c.isNoColorSet() { |
|
||||||
return c |
|
||||||
} |
|
||||||
|
|
||||||
fmt.Fprintf(Output, c.format()) |
|
||||||
return c |
|
||||||
} |
|
||||||
|
|
||||||
func (c *Color) unset() { |
|
||||||
if c.isNoColorSet() { |
|
||||||
return |
|
||||||
} |
|
||||||
|
|
||||||
Unset() |
|
||||||
} |
|
||||||
|
|
||||||
func (c *Color) setWriter(w io.Writer) *Color { |
|
||||||
if c.isNoColorSet() { |
|
||||||
return c |
|
||||||
} |
|
||||||
|
|
||||||
fmt.Fprintf(w, c.format()) |
|
||||||
return c |
|
||||||
} |
|
||||||
|
|
||||||
func (c *Color) unsetWriter(w io.Writer) { |
|
||||||
if c.isNoColorSet() { |
|
||||||
return |
|
||||||
} |
|
||||||
|
|
||||||
if NoColor { |
|
||||||
return |
|
||||||
} |
|
||||||
|
|
||||||
fmt.Fprintf(w, "%s[%dm", escape, Reset) |
|
||||||
} |
|
||||||
|
|
||||||
// Add is used to chain SGR parameters. Use as many as parameters to combine
|
|
||||||
// and create custom color objects. Example: Add(color.FgRed, color.Underline).
|
|
||||||
func (c *Color) Add(value ...Attribute) *Color { |
|
||||||
c.params = append(c.params, value...) |
|
||||||
return c |
|
||||||
} |
|
||||||
|
|
||||||
func (c *Color) prepend(value Attribute) { |
|
||||||
c.params = append(c.params, 0) |
|
||||||
copy(c.params[1:], c.params[0:]) |
|
||||||
c.params[0] = value |
|
||||||
} |
|
||||||
|
|
||||||
// Fprint formats using the default formats for its operands and writes to w.
|
|
||||||
// Spaces are added between operands when neither is a string.
|
|
||||||
// It returns the number of bytes written and any write error encountered.
|
|
||||||
// On Windows, users should wrap w with colorable.NewColorable() if w is of
|
|
||||||
// type *os.File.
|
|
||||||
func (c *Color) Fprint(w io.Writer, a ...interface{}) (n int, err error) { |
|
||||||
c.setWriter(w) |
|
||||||
defer c.unsetWriter(w) |
|
||||||
|
|
||||||
return fmt.Fprint(w, a...) |
|
||||||
} |
|
||||||
|
|
||||||
// Print formats using the default formats for its operands and writes to
|
|
||||||
// standard output. Spaces are added between operands when neither is a
|
|
||||||
// string. It returns the number of bytes written and any write error
|
|
||||||
// encountered. This is the standard fmt.Print() method wrapped with the given
|
|
||||||
// color.
|
|
||||||
func (c *Color) Print(a ...interface{}) (n int, err error) { |
|
||||||
c.Set() |
|
||||||
defer c.unset() |
|
||||||
|
|
||||||
return fmt.Fprint(Output, a...) |
|
||||||
} |
|
||||||
|
|
||||||
// Fprintf formats according to a format specifier and writes to w.
|
|
||||||
// It returns the number of bytes written and any write error encountered.
|
|
||||||
// On Windows, users should wrap w with colorable.NewColorable() if w is of
|
|
||||||
// type *os.File.
|
|
||||||
func (c *Color) Fprintf(w io.Writer, format string, a ...interface{}) (n int, err error) { |
|
||||||
c.setWriter(w) |
|
||||||
defer c.unsetWriter(w) |
|
||||||
|
|
||||||
return fmt.Fprintf(w, format, a...) |
|
||||||
} |
|
||||||
|
|
||||||
// Printf formats according to a format specifier and writes to standard output.
|
|
||||||
// It returns the number of bytes written and any write error encountered.
|
|
||||||
// This is the standard fmt.Printf() method wrapped with the given color.
|
|
||||||
func (c *Color) Printf(format string, a ...interface{}) (n int, err error) { |
|
||||||
c.Set() |
|
||||||
defer c.unset() |
|
||||||
|
|
||||||
return fmt.Fprintf(Output, format, a...) |
|
||||||
} |
|
||||||
|
|
||||||
// Fprintln formats using the default formats for its operands and writes to w.
|
|
||||||
// Spaces are always added between operands and a newline is appended.
|
|
||||||
// On Windows, users should wrap w with colorable.NewColorable() if w is of
|
|
||||||
// type *os.File.
|
|
||||||
func (c *Color) Fprintln(w io.Writer, a ...interface{}) (n int, err error) { |
|
||||||
c.setWriter(w) |
|
||||||
defer c.unsetWriter(w) |
|
||||||
|
|
||||||
return fmt.Fprintln(w, a...) |
|
||||||
} |
|
||||||
|
|
||||||
// Println formats using the default formats for its operands and writes to
|
|
||||||
// standard output. Spaces are always added between operands and a newline is
|
|
||||||
// appended. It returns the number of bytes written and any write error
|
|
||||||
// encountered. This is the standard fmt.Print() method wrapped with the given
|
|
||||||
// color.
|
|
||||||
func (c *Color) Println(a ...interface{}) (n int, err error) { |
|
||||||
c.Set() |
|
||||||
defer c.unset() |
|
||||||
|
|
||||||
return fmt.Fprintln(Output, a...) |
|
||||||
} |
|
||||||
|
|
||||||
// Sprint is just like Print, but returns a string instead of printing it.
|
|
||||||
func (c *Color) Sprint(a ...interface{}) string { |
|
||||||
return c.wrap(fmt.Sprint(a...)) |
|
||||||
} |
|
||||||
|
|
||||||
// Sprintln is just like Println, but returns a string instead of printing it.
|
|
||||||
func (c *Color) Sprintln(a ...interface{}) string { |
|
||||||
return c.wrap(fmt.Sprintln(a...)) |
|
||||||
} |
|
||||||
|
|
||||||
// Sprintf is just like Printf, but returns a string instead of printing it.
|
|
||||||
func (c *Color) Sprintf(format string, a ...interface{}) string { |
|
||||||
return c.wrap(fmt.Sprintf(format, a...)) |
|
||||||
} |
|
||||||
|
|
||||||
// FprintFunc returns a new function that prints the passed arguments as
|
|
||||||
// colorized with color.Fprint().
|
|
||||||
func (c *Color) FprintFunc() func(w io.Writer, a ...interface{}) { |
|
||||||
return func(w io.Writer, a ...interface{}) { |
|
||||||
c.Fprint(w, a...) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// PrintFunc returns a new function that prints the passed arguments as
|
|
||||||
// colorized with color.Print().
|
|
||||||
func (c *Color) PrintFunc() func(a ...interface{}) { |
|
||||||
return func(a ...interface{}) { |
|
||||||
c.Print(a...) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// FprintfFunc returns a new function that prints the passed arguments as
|
|
||||||
// colorized with color.Fprintf().
|
|
||||||
func (c *Color) FprintfFunc() func(w io.Writer, format string, a ...interface{}) { |
|
||||||
return func(w io.Writer, format string, a ...interface{}) { |
|
||||||
c.Fprintf(w, format, a...) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// PrintfFunc returns a new function that prints the passed arguments as
|
|
||||||
// colorized with color.Printf().
|
|
||||||
func (c *Color) PrintfFunc() func(format string, a ...interface{}) { |
|
||||||
return func(format string, a ...interface{}) { |
|
||||||
c.Printf(format, a...) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// FprintlnFunc returns a new function that prints the passed arguments as
|
|
||||||
// colorized with color.Fprintln().
|
|
||||||
func (c *Color) FprintlnFunc() func(w io.Writer, a ...interface{}) { |
|
||||||
return func(w io.Writer, a ...interface{}) { |
|
||||||
c.Fprintln(w, a...) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// PrintlnFunc returns a new function that prints the passed arguments as
|
|
||||||
// colorized with color.Println().
|
|
||||||
func (c *Color) PrintlnFunc() func(a ...interface{}) { |
|
||||||
return func(a ...interface{}) { |
|
||||||
c.Println(a...) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// SprintFunc returns a new function that returns colorized strings for the
|
|
||||||
// given arguments with fmt.Sprint(). Useful to put into or mix into other
|
|
||||||
// string. Windows users should use this in conjunction with color.Output, example:
|
|
||||||
//
|
|
||||||
// put := New(FgYellow).SprintFunc()
|
|
||||||
// fmt.Fprintf(color.Output, "This is a %s", put("warning"))
|
|
||||||
func (c *Color) SprintFunc() func(a ...interface{}) string { |
|
||||||
return func(a ...interface{}) string { |
|
||||||
return c.wrap(fmt.Sprint(a...)) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// SprintfFunc returns a new function that returns colorized strings for the
|
|
||||||
// given arguments with fmt.Sprintf(). Useful to put into or mix into other
|
|
||||||
// string. Windows users should use this in conjunction with color.Output.
|
|
||||||
func (c *Color) SprintfFunc() func(format string, a ...interface{}) string { |
|
||||||
return func(format string, a ...interface{}) string { |
|
||||||
return c.wrap(fmt.Sprintf(format, a...)) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// SprintlnFunc returns a new function that returns colorized strings for the
|
|
||||||
// given arguments with fmt.Sprintln(). Useful to put into or mix into other
|
|
||||||
// string. Windows users should use this in conjunction with color.Output.
|
|
||||||
func (c *Color) SprintlnFunc() func(a ...interface{}) string { |
|
||||||
return func(a ...interface{}) string { |
|
||||||
return c.wrap(fmt.Sprintln(a...)) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// sequence returns a formatted SGR sequence to be plugged into a "\x1b[...m"
|
|
||||||
// an example output might be: "1;36" -> bold cyan
|
|
||||||
func (c *Color) sequence() string { |
|
||||||
format := make([]string, len(c.params)) |
|
||||||
for i, v := range c.params { |
|
||||||
format[i] = strconv.Itoa(int(v)) |
|
||||||
} |
|
||||||
|
|
||||||
return strings.Join(format, ";") |
|
||||||
} |
|
||||||
|
|
||||||
// wrap wraps the s string with the colors attributes. The string is ready to
|
|
||||||
// be printed.
|
|
||||||
func (c *Color) wrap(s string) string { |
|
||||||
if c.isNoColorSet() { |
|
||||||
return s |
|
||||||
} |
|
||||||
|
|
||||||
return c.format() + s + c.unformat() |
|
||||||
} |
|
||||||
|
|
||||||
func (c *Color) format() string { |
|
||||||
return fmt.Sprintf("%s[%sm", escape, c.sequence()) |
|
||||||
} |
|
||||||
|
|
||||||
func (c *Color) unformat() string { |
|
||||||
return fmt.Sprintf("%s[%dm", escape, Reset) |
|
||||||
} |
|
||||||
|
|
||||||
// DisableColor disables the color output. Useful to not change any existing
|
|
||||||
// code and still being able to output. Can be used for flags like
|
|
||||||
// "--no-color". To enable back use EnableColor() method.
|
|
||||||
func (c *Color) DisableColor() { |
|
||||||
c.noColor = boolPtr(true) |
|
||||||
} |
|
||||||
|
|
||||||
// EnableColor enables the color output. Use it in conjunction with
|
|
||||||
// DisableColor(). Otherwise this method has no side effects.
|
|
||||||
func (c *Color) EnableColor() { |
|
||||||
c.noColor = boolPtr(false) |
|
||||||
} |
|
||||||
|
|
||||||
func (c *Color) isNoColorSet() bool { |
|
||||||
// check first if we have user setted action
|
|
||||||
if c.noColor != nil { |
|
||||||
return *c.noColor |
|
||||||
} |
|
||||||
|
|
||||||
// if not return the global option, which is disabled by default
|
|
||||||
return NoColor |
|
||||||
} |
|
||||||
|
|
||||||
// Equals returns a boolean value indicating whether two colors are equal.
|
|
||||||
func (c *Color) Equals(c2 *Color) bool { |
|
||||||
if len(c.params) != len(c2.params) { |
|
||||||
return false |
|
||||||
} |
|
||||||
|
|
||||||
for _, attr := range c.params { |
|
||||||
if !c2.attrExists(attr) { |
|
||||||
return false |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return true |
|
||||||
} |
|
||||||
|
|
||||||
func (c *Color) attrExists(a Attribute) bool { |
|
||||||
for _, attr := range c.params { |
|
||||||
if attr == a { |
|
||||||
return true |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return false |
|
||||||
} |
|
||||||
|
|
||||||
func boolPtr(v bool) *bool { |
|
||||||
return &v |
|
||||||
} |
|
||||||
|
|
||||||
func getCachedColor(p Attribute) *Color { |
|
||||||
colorsCacheMu.Lock() |
|
||||||
defer colorsCacheMu.Unlock() |
|
||||||
|
|
||||||
c, ok := colorsCache[p] |
|
||||||
if !ok { |
|
||||||
c = New(p) |
|
||||||
colorsCache[p] = c |
|
||||||
} |
|
||||||
|
|
||||||
return c |
|
||||||
} |
|
||||||
|
|
||||||
func colorPrint(format string, p Attribute, a ...interface{}) { |
|
||||||
c := getCachedColor(p) |
|
||||||
|
|
||||||
if !strings.HasSuffix(format, "\n") { |
|
||||||
format += "\n" |
|
||||||
} |
|
||||||
|
|
||||||
if len(a) == 0 { |
|
||||||
c.Print(format) |
|
||||||
} else { |
|
||||||
c.Printf(format, a...) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
func colorString(format string, p Attribute, a ...interface{}) string { |
|
||||||
c := getCachedColor(p) |
|
||||||
|
|
||||||
if len(a) == 0 { |
|
||||||
return c.SprintFunc()(format) |
|
||||||
} |
|
||||||
|
|
||||||
return c.SprintfFunc()(format, a...) |
|
||||||
} |
|
||||||
|
|
||||||
// Black is a convenient helper function to print with black foreground. A
|
|
||||||
// newline is appended to format by default.
|
|
||||||
func Black(format string, a ...interface{}) { colorPrint(format, FgBlack, a...) } |
|
||||||
|
|
||||||
// Red is a convenient helper function to print with red foreground. A
|
|
||||||
// newline is appended to format by default.
|
|
||||||
func Red(format string, a ...interface{}) { colorPrint(format, FgRed, a...) } |
|
||||||
|
|
||||||
// Green is a convenient helper function to print with green foreground. A
|
|
||||||
// newline is appended to format by default.
|
|
||||||
func Green(format string, a ...interface{}) { colorPrint(format, FgGreen, a...) } |
|
||||||
|
|
||||||
// Yellow is a convenient helper function to print with yellow foreground.
|
|
||||||
// A newline is appended to format by default.
|
|
||||||
func Yellow(format string, a ...interface{}) { colorPrint(format, FgYellow, a...) } |
|
||||||
|
|
||||||
// Blue is a convenient helper function to print with blue foreground. A
|
|
||||||
// newline is appended to format by default.
|
|
||||||
func Blue(format string, a ...interface{}) { colorPrint(format, FgBlue, a...) } |
|
||||||
|
|
||||||
// Magenta is a convenient helper function to print with magenta foreground.
|
|
||||||
// A newline is appended to format by default.
|
|
||||||
func Magenta(format string, a ...interface{}) { colorPrint(format, FgMagenta, a...) } |
|
||||||
|
|
||||||
// Cyan is a convenient helper function to print with cyan foreground. A
|
|
||||||
// newline is appended to format by default.
|
|
||||||
func Cyan(format string, a ...interface{}) { colorPrint(format, FgCyan, a...) } |
|
||||||
|
|
||||||
// White is a convenient helper function to print with white foreground. A
|
|
||||||
// newline is appended to format by default.
|
|
||||||
func White(format string, a ...interface{}) { colorPrint(format, FgWhite, a...) } |
|
||||||
|
|
||||||
// BlackString is a convenient helper function to return a string with black
|
|
||||||
// foreground.
|
|
||||||
func BlackString(format string, a ...interface{}) string { return colorString(format, FgBlack, a...) } |
|
||||||
|
|
||||||
// RedString is a convenient helper function to return a string with red
|
|
||||||
// foreground.
|
|
||||||
func RedString(format string, a ...interface{}) string { return colorString(format, FgRed, a...) } |
|
||||||
|
|
||||||
// GreenString is a convenient helper function to return a string with green
|
|
||||||
// foreground.
|
|
||||||
func GreenString(format string, a ...interface{}) string { return colorString(format, FgGreen, a...) } |
|
||||||
|
|
||||||
// YellowString is a convenient helper function to return a string with yellow
|
|
||||||
// foreground.
|
|
||||||
func YellowString(format string, a ...interface{}) string { return colorString(format, FgYellow, a...) } |
|
||||||
|
|
||||||
// BlueString is a convenient helper function to return a string with blue
|
|
||||||
// foreground.
|
|
||||||
func BlueString(format string, a ...interface{}) string { return colorString(format, FgBlue, a...) } |
|
||||||
|
|
||||||
// MagentaString is a convenient helper function to return a string with magenta
|
|
||||||
// foreground.
|
|
||||||
func MagentaString(format string, a ...interface{}) string { |
|
||||||
return colorString(format, FgMagenta, a...) |
|
||||||
} |
|
||||||
|
|
||||||
// CyanString is a convenient helper function to return a string with cyan
|
|
||||||
// foreground.
|
|
||||||
func CyanString(format string, a ...interface{}) string { return colorString(format, FgCyan, a...) } |
|
||||||
|
|
||||||
// WhiteString is a convenient helper function to return a string with white
|
|
||||||
// foreground.
|
|
||||||
func WhiteString(format string, a ...interface{}) string { return colorString(format, FgWhite, a...) } |
|
||||||
|
|
||||||
// HiBlack is a convenient helper function to print with hi-intensity black foreground. A
|
|
||||||
// newline is appended to format by default.
|
|
||||||
func HiBlack(format string, a ...interface{}) { colorPrint(format, FgHiBlack, a...) } |
|
||||||
|
|
||||||
// HiRed is a convenient helper function to print with hi-intensity red foreground. A
|
|
||||||
// newline is appended to format by default.
|
|
||||||
func HiRed(format string, a ...interface{}) { colorPrint(format, FgHiRed, a...) } |
|
||||||
|
|
||||||
// HiGreen is a convenient helper function to print with hi-intensity green foreground. A
|
|
||||||
// newline is appended to format by default.
|
|
||||||
func HiGreen(format string, a ...interface{}) { colorPrint(format, FgHiGreen, a...) } |
|
||||||
|
|
||||||
// HiYellow is a convenient helper function to print with hi-intensity yellow foreground.
|
|
||||||
// A newline is appended to format by default.
|
|
||||||
func HiYellow(format string, a ...interface{}) { colorPrint(format, FgHiYellow, a...) } |
|
||||||
|
|
||||||
// HiBlue is a convenient helper function to print with hi-intensity blue foreground. A
|
|
||||||
// newline is appended to format by default.
|
|
||||||
func HiBlue(format string, a ...interface{}) { colorPrint(format, FgHiBlue, a...) } |
|
||||||
|
|
||||||
// HiMagenta is a convenient helper function to print with hi-intensity magenta foreground.
|
|
||||||
// A newline is appended to format by default.
|
|
||||||
func HiMagenta(format string, a ...interface{}) { colorPrint(format, FgHiMagenta, a...) } |
|
||||||
|
|
||||||
// HiCyan is a convenient helper function to print with hi-intensity cyan foreground. A
|
|
||||||
// newline is appended to format by default.
|
|
||||||
func HiCyan(format string, a ...interface{}) { colorPrint(format, FgHiCyan, a...) } |
|
||||||
|
|
||||||
// HiWhite is a convenient helper function to print with hi-intensity white foreground. A
|
|
||||||
// newline is appended to format by default.
|
|
||||||
func HiWhite(format string, a ...interface{}) { colorPrint(format, FgHiWhite, a...) } |
|
||||||
|
|
||||||
// HiBlackString is a convenient helper function to return a string with hi-intensity black
|
|
||||||
// foreground.
|
|
||||||
func HiBlackString(format string, a ...interface{}) string { |
|
||||||
return colorString(format, FgHiBlack, a...) |
|
||||||
} |
|
||||||
|
|
||||||
// HiRedString is a convenient helper function to return a string with hi-intensity red
|
|
||||||
// foreground.
|
|
||||||
func HiRedString(format string, a ...interface{}) string { return colorString(format, FgHiRed, a...) } |
|
||||||
|
|
||||||
// HiGreenString is a convenient helper function to return a string with hi-intensity green
|
|
||||||
// foreground.
|
|
||||||
func HiGreenString(format string, a ...interface{}) string { |
|
||||||
return colorString(format, FgHiGreen, a...) |
|
||||||
} |
|
||||||
|
|
||||||
// HiYellowString is a convenient helper function to return a string with hi-intensity yellow
|
|
||||||
// foreground.
|
|
||||||
func HiYellowString(format string, a ...interface{}) string { |
|
||||||
return colorString(format, FgHiYellow, a...) |
|
||||||
} |
|
||||||
|
|
||||||
// HiBlueString is a convenient helper function to return a string with hi-intensity blue
|
|
||||||
// foreground.
|
|
||||||
func HiBlueString(format string, a ...interface{}) string { return colorString(format, FgHiBlue, a...) } |
|
||||||
|
|
||||||
// HiMagentaString is a convenient helper function to return a string with hi-intensity magenta
|
|
||||||
// foreground.
|
|
||||||
func HiMagentaString(format string, a ...interface{}) string { |
|
||||||
return colorString(format, FgHiMagenta, a...) |
|
||||||
} |
|
||||||
|
|
||||||
// HiCyanString is a convenient helper function to return a string with hi-intensity cyan
|
|
||||||
// foreground.
|
|
||||||
func HiCyanString(format string, a ...interface{}) string { return colorString(format, FgHiCyan, a...) } |
|
||||||
|
|
||||||
// HiWhiteString is a convenient helper function to return a string with hi-intensity white
|
|
||||||
// foreground.
|
|
||||||
func HiWhiteString(format string, a ...interface{}) string { |
|
||||||
return colorString(format, FgHiWhite, a...) |
|
||||||
} |
|
@ -1,133 +0,0 @@ |
|||||||
/* |
|
||||||
Package color is an ANSI color package to output colorized or SGR defined |
|
||||||
output to the standard output. The API can be used in several way, pick one |
|
||||||
that suits you. |
|
||||||
|
|
||||||
Use simple and default helper functions with predefined foreground colors: |
|
||||||
|
|
||||||
color.Cyan("Prints text in cyan.") |
|
||||||
|
|
||||||
// a newline will be appended automatically
|
|
||||||
color.Blue("Prints %s in blue.", "text") |
|
||||||
|
|
||||||
// More default foreground colors..
|
|
||||||
color.Red("We have red") |
|
||||||
color.Yellow("Yellow color too!") |
|
||||||
color.Magenta("And many others ..") |
|
||||||
|
|
||||||
// Hi-intensity colors
|
|
||||||
color.HiGreen("Bright green color.") |
|
||||||
color.HiBlack("Bright black means gray..") |
|
||||||
color.HiWhite("Shiny white color!") |
|
||||||
|
|
||||||
However there are times where custom color mixes are required. Below are some |
|
||||||
examples to create custom color objects and use the print functions of each |
|
||||||
separate color object. |
|
||||||
|
|
||||||
// Create a new color object
|
|
||||||
c := color.New(color.FgCyan).Add(color.Underline) |
|
||||||
c.Println("Prints cyan text with an underline.") |
|
||||||
|
|
||||||
// Or just add them to New()
|
|
||||||
d := color.New(color.FgCyan, color.Bold) |
|
||||||
d.Printf("This prints bold cyan %s\n", "too!.") |
|
||||||
|
|
||||||
|
|
||||||
// Mix up foreground and background colors, create new mixes!
|
|
||||||
red := color.New(color.FgRed) |
|
||||||
|
|
||||||
boldRed := red.Add(color.Bold) |
|
||||||
boldRed.Println("This will print text in bold red.") |
|
||||||
|
|
||||||
whiteBackground := red.Add(color.BgWhite) |
|
||||||
whiteBackground.Println("Red text with White background.") |
|
||||||
|
|
||||||
// Use your own io.Writer output
|
|
||||||
color.New(color.FgBlue).Fprintln(myWriter, "blue color!") |
|
||||||
|
|
||||||
blue := color.New(color.FgBlue) |
|
||||||
blue.Fprint(myWriter, "This will print text in blue.") |
|
||||||
|
|
||||||
You can create PrintXxx functions to simplify even more: |
|
||||||
|
|
||||||
// Create a custom print function for convenient
|
|
||||||
red := color.New(color.FgRed).PrintfFunc() |
|
||||||
red("warning") |
|
||||||
red("error: %s", err) |
|
||||||
|
|
||||||
// Mix up multiple attributes
|
|
||||||
notice := color.New(color.Bold, color.FgGreen).PrintlnFunc() |
|
||||||
notice("don't forget this...") |
|
||||||
|
|
||||||
You can also FprintXxx functions to pass your own io.Writer: |
|
||||||
|
|
||||||
blue := color.New(FgBlue).FprintfFunc() |
|
||||||
blue(myWriter, "important notice: %s", stars) |
|
||||||
|
|
||||||
// Mix up with multiple attributes
|
|
||||||
success := color.New(color.Bold, color.FgGreen).FprintlnFunc() |
|
||||||
success(myWriter, don't forget this...") |
|
||||||
|
|
||||||
|
|
||||||
Or create SprintXxx functions to mix strings with other non-colorized strings: |
|
||||||
|
|
||||||
yellow := New(FgYellow).SprintFunc() |
|
||||||
red := New(FgRed).SprintFunc() |
|
||||||
|
|
||||||
fmt.Printf("this is a %s and this is %s.\n", yellow("warning"), red("error")) |
|
||||||
|
|
||||||
info := New(FgWhite, BgGreen).SprintFunc() |
|
||||||
fmt.Printf("this %s rocks!\n", info("package")) |
|
||||||
|
|
||||||
Windows support is enabled by default. All Print functions work as intended. |
|
||||||
However only for color.SprintXXX functions, user should use fmt.FprintXXX and |
|
||||||
set the output to color.Output: |
|
||||||
|
|
||||||
fmt.Fprintf(color.Output, "Windows support: %s", color.GreenString("PASS")) |
|
||||||
|
|
||||||
info := New(FgWhite, BgGreen).SprintFunc() |
|
||||||
fmt.Fprintf(color.Output, "this %s rocks!\n", info("package")) |
|
||||||
|
|
||||||
Using with existing code is possible. Just use the Set() method to set the |
|
||||||
standard output to the given parameters. That way a rewrite of an existing |
|
||||||
code is not required. |
|
||||||
|
|
||||||
// Use handy standard colors.
|
|
||||||
color.Set(color.FgYellow) |
|
||||||
|
|
||||||
fmt.Println("Existing text will be now in Yellow") |
|
||||||
fmt.Printf("This one %s\n", "too") |
|
||||||
|
|
||||||
color.Unset() // don't forget to unset
|
|
||||||
|
|
||||||
// You can mix up parameters
|
|
||||||
color.Set(color.FgMagenta, color.Bold) |
|
||||||
defer color.Unset() // use it in your function
|
|
||||||
|
|
||||||
fmt.Println("All text will be now bold magenta.") |
|
||||||
|
|
||||||
There might be a case where you want to disable color output (for example to |
|
||||||
pipe the standard output of your app to somewhere else). `Color` has support to |
|
||||||
disable colors both globally and for single color definition. For example |
|
||||||
suppose you have a CLI app and a `--no-color` bool flag. You can easily disable |
|
||||||
the color output with: |
|
||||||
|
|
||||||
var flagNoColor = flag.Bool("no-color", false, "Disable color output") |
|
||||||
|
|
||||||
if *flagNoColor { |
|
||||||
color.NoColor = true // disables colorized output
|
|
||||||
} |
|
||||||
|
|
||||||
It also has support for single color definitions (local). You can |
|
||||||
disable/enable color output on the fly: |
|
||||||
|
|
||||||
c := color.New(color.FgCyan) |
|
||||||
c.Println("Prints cyan text") |
|
||||||
|
|
||||||
c.DisableColor() |
|
||||||
c.Println("This is printed without any color") |
|
||||||
|
|
||||||
c.EnableColor() |
|
||||||
c.Println("This prints again cyan...") |
|
||||||
*/ |
|
||||||
package color |
|
@ -1,8 +0,0 @@ |
|||||||
module github.com/fatih/color |
|
||||||
|
|
||||||
go 1.13 |
|
||||||
|
|
||||||
require ( |
|
||||||
github.com/mattn/go-colorable v0.1.8 |
|
||||||
github.com/mattn/go-isatty v0.0.12 |
|
||||||
) |
|
@ -1,7 +0,0 @@ |
|||||||
github.com/mattn/go-colorable v0.1.8 h1:c1ghPdyEDarC70ftn0y+A/Ee++9zz8ljHG1b13eJ0s8= |
|
||||||
github.com/mattn/go-colorable v0.1.8/go.mod h1:u6P/XSegPjTcexA+o6vUJrdnUu04hMope9wVRipJSqc= |
|
||||||
github.com/mattn/go-isatty v0.0.12 h1:wuysRhFDzyxgEmMf5xjvJ2M9dZoWAXNNr5LSBS7uHXY= |
|
||||||
github.com/mattn/go-isatty v0.0.12/go.mod h1:cbi8OIDigv2wuxKPP5vlRcQ1OAZbq2CE4Kysco4FUpU= |
|
||||||
golang.org/x/sys v0.0.0-20200116001909-b77594299b42/go.mod h1:h1NjWce9XRLGQEsW7wpKNCjG9DtNlClVuFLEZdDNbEs= |
|
||||||
golang.org/x/sys v0.0.0-20200223170610-d5e6a3e2c0ae h1:/WDfKMnPU+m5M4xB+6x4kaepxRw6jWvR5iDRdvjHgy8= |
|
||||||
golang.org/x/sys v0.0.0-20200223170610-d5e6a3e2c0ae/go.mod h1:h1NjWce9XRLGQEsW7wpKNCjG9DtNlClVuFLEZdDNbEs= |
|
@ -1,60 +0,0 @@ |
|||||||
Copyright (c) 2017, Fatih Arslan |
|
||||||
All rights reserved. |
|
||||||
|
|
||||||
Redistribution and use in source and binary forms, with or without |
|
||||||
modification, are permitted provided that the following conditions are met: |
|
||||||
|
|
||||||
* Redistributions of source code must retain the above copyright notice, this |
|
||||||
list of conditions and the following disclaimer. |
|
||||||
|
|
||||||
* Redistributions in binary form must reproduce the above copyright notice, |
|
||||||
this list of conditions and the following disclaimer in the documentation |
|
||||||
and/or other materials provided with the distribution. |
|
||||||
|
|
||||||
* Neither the name of structtag nor the names of its |
|
||||||
contributors may be used to endorse or promote products derived from |
|
||||||
this software without specific prior written permission. |
|
||||||
|
|
||||||
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" |
|
||||||
AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE |
|
||||||
IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE |
|
||||||
DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE |
|
||||||
FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL |
|
||||||
DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR |
|
||||||
SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER |
|
||||||
CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, |
|
||||||
OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE |
|
||||||
OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. |
|
||||||
|
|
||||||
This software includes some portions from Go. Go is used under the terms of the |
|
||||||
BSD like license. |
|
||||||
|
|
||||||
Copyright (c) 2012 The Go Authors. All rights reserved. |
|
||||||
|
|
||||||
Redistribution and use in source and binary forms, with or without |
|
||||||
modification, are permitted provided that the following conditions are |
|
||||||
met: |
|
||||||
|
|
||||||
* Redistributions of source code must retain the above copyright |
|
||||||
notice, this list of conditions and the following disclaimer. |
|
||||||
* Redistributions in binary form must reproduce the above |
|
||||||
copyright notice, this list of conditions and the following disclaimer |
|
||||||
in the documentation and/or other materials provided with the |
|
||||||
distribution. |
|
||||||
* Neither the name of Google Inc. nor the names of its |
|
||||||
contributors may be used to endorse or promote products derived from |
|
||||||
this software without specific prior written permission. |
|
||||||
|
|
||||||
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS |
|
||||||
"AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT |
|
||||||
LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR |
|
||||||
A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT |
|
||||||
OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, |
|
||||||
SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT |
|
||||||
LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, |
|
||||||
DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY |
|
||||||
THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT |
|
||||||
(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE |
|
||||||
OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. |
|
||||||
|
|
||||||
The Go gopher was designed by Renee French. http://reneefrench.blogspot.com/ The design is licensed under the Creative Commons 3.0 Attributions license. Read this article for more details: https://blog.golang.org/gopher |
|
@ -1,73 +0,0 @@ |
|||||||
# structtag [](http://godoc.org/github.com/fatih/structtag) |
|
||||||
|
|
||||||
structtag provides an easy way of parsing and manipulating struct tag fields. |
|
||||||
Please vendor the library as it might change in future versions. |
|
||||||
|
|
||||||
# Install |
|
||||||
|
|
||||||
```bash |
|
||||||
go get github.com/fatih/structtag |
|
||||||
``` |
|
||||||
|
|
||||||
# Example |
|
||||||
|
|
||||||
```go |
|
||||||
package main |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"reflect" |
|
||||||
"sort" |
|
||||||
|
|
||||||
"github.com/fatih/structtag" |
|
||||||
) |
|
||||||
|
|
||||||
func main() { |
|
||||||
type t struct { |
|
||||||
t string `json:"foo,omitempty,string" xml:"foo"` |
|
||||||
} |
|
||||||
|
|
||||||
// get field tag |
|
||||||
tag := reflect.TypeOf(t{}).Field(0).Tag |
|
||||||
|
|
||||||
// ... and start using structtag by parsing the tag |
|
||||||
tags, err := structtag.Parse(string(tag)) |
|
||||||
if err != nil { |
|
||||||
panic(err) |
|
||||||
} |
|
||||||
|
|
||||||
// iterate over all tags |
|
||||||
for _, t := range tags.Tags() { |
|
||||||
fmt.Printf("tag: %+v\n", t) |
|
||||||
} |
|
||||||
|
|
||||||
// get a single tag |
|
||||||
jsonTag, err := tags.Get("json") |
|
||||||
if err != nil { |
|
||||||
panic(err) |
|
||||||
} |
|
||||||
fmt.Println(jsonTag) // Output: json:"foo,omitempty,string" |
|
||||||
fmt.Println(jsonTag.Key) // Output: json |
|
||||||
fmt.Println(jsonTag.Name) // Output: foo |
|
||||||
fmt.Println(jsonTag.Options) // Output: [omitempty string] |
|
||||||
|
|
||||||
// change existing tag |
|
||||||
jsonTag.Name = "foo_bar" |
|
||||||
jsonTag.Options = nil |
|
||||||
tags.Set(jsonTag) |
|
||||||
|
|
||||||
// add new tag |
|
||||||
tags.Set(&structtag.Tag{ |
|
||||||
Key: "hcl", |
|
||||||
Name: "foo", |
|
||||||
Options: []string{"squash"}, |
|
||||||
}) |
|
||||||
|
|
||||||
// print the tags |
|
||||||
fmt.Println(tags) // Output: json:"foo_bar" xml:"foo" hcl:"foo,squash" |
|
||||||
|
|
||||||
// sort tags according to keys |
|
||||||
sort.Sort(tags) |
|
||||||
fmt.Println(tags) // Output: hcl:"foo,squash" json:"foo_bar" xml:"foo" |
|
||||||
} |
|
||||||
``` |
|
@ -1,3 +0,0 @@ |
|||||||
module github.com/fatih/structtag |
|
||||||
|
|
||||||
go 1.12 |
|
@ -1,315 +0,0 @@ |
|||||||
package structtag |
|
||||||
|
|
||||||
import ( |
|
||||||
"bytes" |
|
||||||
"errors" |
|
||||||
"fmt" |
|
||||||
"strconv" |
|
||||||
"strings" |
|
||||||
) |
|
||||||
|
|
||||||
var ( |
|
||||||
errTagSyntax = errors.New("bad syntax for struct tag pair") |
|
||||||
errTagKeySyntax = errors.New("bad syntax for struct tag key") |
|
||||||
errTagValueSyntax = errors.New("bad syntax for struct tag value") |
|
||||||
|
|
||||||
errKeyNotSet = errors.New("tag key does not exist") |
|
||||||
errTagNotExist = errors.New("tag does not exist") |
|
||||||
errTagKeyMismatch = errors.New("mismatch between key and tag.key") |
|
||||||
) |
|
||||||
|
|
||||||
// Tags represent a set of tags from a single struct field
|
|
||||||
type Tags struct { |
|
||||||
tags []*Tag |
|
||||||
} |
|
||||||
|
|
||||||
// Tag defines a single struct's string literal tag
|
|
||||||
type Tag struct { |
|
||||||
// Key is the tag key, such as json, xml, etc..
|
|
||||||
// i.e: `json:"foo,omitempty". Here key is: "json"
|
|
||||||
Key string |
|
||||||
|
|
||||||
// Name is a part of the value
|
|
||||||
// i.e: `json:"foo,omitempty". Here name is: "foo"
|
|
||||||
Name string |
|
||||||
|
|
||||||
// Options is a part of the value. It contains a slice of tag options i.e:
|
|
||||||
// `json:"foo,omitempty". Here options is: ["omitempty"]
|
|
||||||
Options []string |
|
||||||
} |
|
||||||
|
|
||||||
// Parse parses a single struct field tag and returns the set of tags.
|
|
||||||
func Parse(tag string) (*Tags, error) { |
|
||||||
var tags []*Tag |
|
||||||
|
|
||||||
hasTag := tag != "" |
|
||||||
|
|
||||||
// NOTE(arslan) following code is from reflect and vet package with some
|
|
||||||
// modifications to collect all necessary information and extend it with
|
|
||||||
// usable methods
|
|
||||||
for tag != "" { |
|
||||||
// Skip leading space.
|
|
||||||
i := 0 |
|
||||||
for i < len(tag) && tag[i] == ' ' { |
|
||||||
i++ |
|
||||||
} |
|
||||||
tag = tag[i:] |
|
||||||
if tag == "" { |
|
||||||
break |
|
||||||
} |
|
||||||
|
|
||||||
// Scan to colon. A space, a quote or a control character is a syntax
|
|
||||||
// error. Strictly speaking, control chars include the range [0x7f,
|
|
||||||
// 0x9f], not just [0x00, 0x1f], but in practice, we ignore the
|
|
||||||
// multi-byte control characters as it is simpler to inspect the tag's
|
|
||||||
// bytes than the tag's runes.
|
|
||||||
i = 0 |
|
||||||
for i < len(tag) && tag[i] > ' ' && tag[i] != ':' && tag[i] != '"' && tag[i] != 0x7f { |
|
||||||
i++ |
|
||||||
} |
|
||||||
|
|
||||||
if i == 0 { |
|
||||||
return nil, errTagKeySyntax |
|
||||||
} |
|
||||||
if i+1 >= len(tag) || tag[i] != ':' { |
|
||||||
return nil, errTagSyntax |
|
||||||
} |
|
||||||
if tag[i+1] != '"' { |
|
||||||
return nil, errTagValueSyntax |
|
||||||
} |
|
||||||
|
|
||||||
key := string(tag[:i]) |
|
||||||
tag = tag[i+1:] |
|
||||||
|
|
||||||
// Scan quoted string to find value.
|
|
||||||
i = 1 |
|
||||||
for i < len(tag) && tag[i] != '"' { |
|
||||||
if tag[i] == '\\' { |
|
||||||
i++ |
|
||||||
} |
|
||||||
i++ |
|
||||||
} |
|
||||||
if i >= len(tag) { |
|
||||||
return nil, errTagValueSyntax |
|
||||||
} |
|
||||||
|
|
||||||
qvalue := string(tag[:i+1]) |
|
||||||
tag = tag[i+1:] |
|
||||||
|
|
||||||
value, err := strconv.Unquote(qvalue) |
|
||||||
if err != nil { |
|
||||||
return nil, errTagValueSyntax |
|
||||||
} |
|
||||||
|
|
||||||
res := strings.Split(value, ",") |
|
||||||
name := res[0] |
|
||||||
options := res[1:] |
|
||||||
if len(options) == 0 { |
|
||||||
options = nil |
|
||||||
} |
|
||||||
|
|
||||||
tags = append(tags, &Tag{ |
|
||||||
Key: key, |
|
||||||
Name: name, |
|
||||||
Options: options, |
|
||||||
}) |
|
||||||
} |
|
||||||
|
|
||||||
if hasTag && len(tags) == 0 { |
|
||||||
return nil, nil |
|
||||||
} |
|
||||||
|
|
||||||
return &Tags{ |
|
||||||
tags: tags, |
|
||||||
}, nil |
|
||||||
} |
|
||||||
|
|
||||||
// Get returns the tag associated with the given key. If the key is present
|
|
||||||
// in the tag the value (which may be empty) is returned. Otherwise the
|
|
||||||
// returned value will be the empty string. The ok return value reports whether
|
|
||||||
// the tag exists or not (which the return value is nil).
|
|
||||||
func (t *Tags) Get(key string) (*Tag, error) { |
|
||||||
for _, tag := range t.tags { |
|
||||||
if tag.Key == key { |
|
||||||
return tag, nil |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return nil, errTagNotExist |
|
||||||
} |
|
||||||
|
|
||||||
// Set sets the given tag. If the tag key already exists it'll override it
|
|
||||||
func (t *Tags) Set(tag *Tag) error { |
|
||||||
if tag.Key == "" { |
|
||||||
return errKeyNotSet |
|
||||||
} |
|
||||||
|
|
||||||
added := false |
|
||||||
for i, tg := range t.tags { |
|
||||||
if tg.Key == tag.Key { |
|
||||||
added = true |
|
||||||
t.tags[i] = tag |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
if !added { |
|
||||||
// this means this is a new tag, add it
|
|
||||||
t.tags = append(t.tags, tag) |
|
||||||
} |
|
||||||
|
|
||||||
return nil |
|
||||||
} |
|
||||||
|
|
||||||
// AddOptions adds the given option for the given key. If the option already
|
|
||||||
// exists it doesn't add it again.
|
|
||||||
func (t *Tags) AddOptions(key string, options ...string) { |
|
||||||
for i, tag := range t.tags { |
|
||||||
if tag.Key != key { |
|
||||||
continue |
|
||||||
} |
|
||||||
|
|
||||||
for _, opt := range options { |
|
||||||
if !tag.HasOption(opt) { |
|
||||||
tag.Options = append(tag.Options, opt) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
t.tags[i] = tag |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// DeleteOptions deletes the given options for the given key
|
|
||||||
func (t *Tags) DeleteOptions(key string, options ...string) { |
|
||||||
hasOption := func(option string) bool { |
|
||||||
for _, opt := range options { |
|
||||||
if opt == option { |
|
||||||
return true |
|
||||||
} |
|
||||||
} |
|
||||||
return false |
|
||||||
} |
|
||||||
|
|
||||||
for i, tag := range t.tags { |
|
||||||
if tag.Key != key { |
|
||||||
continue |
|
||||||
} |
|
||||||
|
|
||||||
var updated []string |
|
||||||
for _, opt := range tag.Options { |
|
||||||
if !hasOption(opt) { |
|
||||||
updated = append(updated, opt) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
tag.Options = updated |
|
||||||
t.tags[i] = tag |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// Delete deletes the tag for the given keys
|
|
||||||
func (t *Tags) Delete(keys ...string) { |
|
||||||
hasKey := func(key string) bool { |
|
||||||
for _, k := range keys { |
|
||||||
if k == key { |
|
||||||
return true |
|
||||||
} |
|
||||||
} |
|
||||||
return false |
|
||||||
} |
|
||||||
|
|
||||||
var updated []*Tag |
|
||||||
for _, tag := range t.tags { |
|
||||||
if !hasKey(tag.Key) { |
|
||||||
updated = append(updated, tag) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
t.tags = updated |
|
||||||
} |
|
||||||
|
|
||||||
// Tags returns a slice of tags. The order is the original tag order unless it
|
|
||||||
// was changed.
|
|
||||||
func (t *Tags) Tags() []*Tag { |
|
||||||
return t.tags |
|
||||||
} |
|
||||||
|
|
||||||
// Tags returns a slice of tags. The order is the original tag order unless it
|
|
||||||
// was changed.
|
|
||||||
func (t *Tags) Keys() []string { |
|
||||||
var keys []string |
|
||||||
for _, tag := range t.tags { |
|
||||||
keys = append(keys, tag.Key) |
|
||||||
} |
|
||||||
return keys |
|
||||||
} |
|
||||||
|
|
||||||
// String reassembles the tags into a valid literal tag field representation
|
|
||||||
func (t *Tags) String() string { |
|
||||||
tags := t.Tags() |
|
||||||
if len(tags) == 0 { |
|
||||||
return "" |
|
||||||
} |
|
||||||
|
|
||||||
var buf bytes.Buffer |
|
||||||
for i, tag := range t.Tags() { |
|
||||||
buf.WriteString(tag.String()) |
|
||||||
if i != len(tags)-1 { |
|
||||||
buf.WriteString(" ") |
|
||||||
} |
|
||||||
} |
|
||||||
return buf.String() |
|
||||||
} |
|
||||||
|
|
||||||
// HasOption returns true if the given option is available in options
|
|
||||||
func (t *Tag) HasOption(opt string) bool { |
|
||||||
for _, tagOpt := range t.Options { |
|
||||||
if tagOpt == opt { |
|
||||||
return true |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return false |
|
||||||
} |
|
||||||
|
|
||||||
// Value returns the raw value of the tag, i.e. if the tag is
|
|
||||||
// `json:"foo,omitempty", the Value is "foo,omitempty"
|
|
||||||
func (t *Tag) Value() string { |
|
||||||
options := strings.Join(t.Options, ",") |
|
||||||
if options != "" { |
|
||||||
return fmt.Sprintf(`%s,%s`, t.Name, options) |
|
||||||
} |
|
||||||
return t.Name |
|
||||||
} |
|
||||||
|
|
||||||
// String reassembles the tag into a valid tag field representation
|
|
||||||
func (t *Tag) String() string { |
|
||||||
return fmt.Sprintf(`%s:%q`, t.Key, t.Value()) |
|
||||||
} |
|
||||||
|
|
||||||
// GoString implements the fmt.GoStringer interface
|
|
||||||
func (t *Tag) GoString() string { |
|
||||||
template := `{ |
|
||||||
Key: '%s', |
|
||||||
Name: '%s', |
|
||||||
Option: '%s', |
|
||||||
}` |
|
||||||
|
|
||||||
if t.Options == nil { |
|
||||||
return fmt.Sprintf(template, t.Key, t.Name, "nil") |
|
||||||
} |
|
||||||
|
|
||||||
options := strings.Join(t.Options, ",") |
|
||||||
return fmt.Sprintf(template, t.Key, t.Name, options) |
|
||||||
} |
|
||||||
|
|
||||||
func (t *Tags) Len() int { |
|
||||||
return len(t.tags) |
|
||||||
} |
|
||||||
|
|
||||||
func (t *Tags) Less(i int, j int) bool { |
|
||||||
return t.tags[i].Key < t.tags[j].Key |
|
||||||
} |
|
||||||
|
|
||||||
func (t *Tags) Swap(i int, j int) { |
|
||||||
t.tags[i], t.tags[j] = t.tags[j], t.tags[i] |
|
||||||
} |
|
@ -1,15 +0,0 @@ |
|||||||
language: go |
|
||||||
sudo: false |
|
||||||
go: |
|
||||||
- 1.13.x |
|
||||||
- tip |
|
||||||
|
|
||||||
before_install: |
|
||||||
- go get -t -v ./... |
|
||||||
|
|
||||||
script: |
|
||||||
- ./go.test.sh |
|
||||||
|
|
||||||
after_success: |
|
||||||
- bash <(curl -s https://codecov.io/bash) |
|
||||||
|
|
@ -1,21 +0,0 @@ |
|||||||
The MIT License (MIT) |
|
||||||
|
|
||||||
Copyright (c) 2016 Yasuhiro Matsumoto |
|
||||||
|
|
||||||
Permission is hereby granted, free of charge, to any person obtaining a copy |
|
||||||
of this software and associated documentation files (the "Software"), to deal |
|
||||||
in the Software without restriction, including without limitation the rights |
|
||||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell |
|
||||||
copies of the Software, and to permit persons to whom the Software is |
|
||||||
furnished to do so, subject to the following conditions: |
|
||||||
|
|
||||||
The above copyright notice and this permission notice shall be included in all |
|
||||||
copies or substantial portions of the Software. |
|
||||||
|
|
||||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR |
|
||||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, |
|
||||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE |
|
||||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER |
|
||||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, |
|
||||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE |
|
||||||
SOFTWARE. |
|
@ -1,48 +0,0 @@ |
|||||||
# go-colorable |
|
||||||
|
|
||||||
[](https://travis-ci.org/mattn/go-colorable) |
|
||||||
[](https://codecov.io/gh/mattn/go-colorable) |
|
||||||
[](http://godoc.org/github.com/mattn/go-colorable) |
|
||||||
[](https://goreportcard.com/report/mattn/go-colorable) |
|
||||||
|
|
||||||
Colorable writer for windows. |
|
||||||
|
|
||||||
For example, most of logger packages doesn't show colors on windows. (I know we can do it with ansicon. But I don't want.) |
|
||||||
This package is possible to handle escape sequence for ansi color on windows. |
|
||||||
|
|
||||||
## Too Bad! |
|
||||||
|
|
||||||
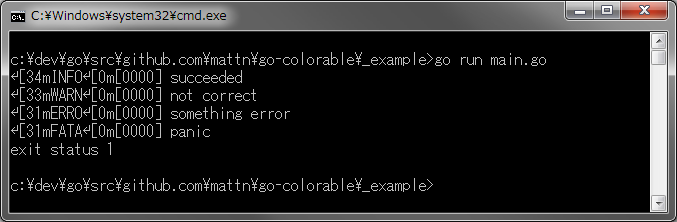 |
|
||||||
|
|
||||||
|
|
||||||
## So Good! |
|
||||||
|
|
||||||
 |
|
||||||
|
|
||||||
## Usage |
|
||||||
|
|
||||||
```go |
|
||||||
logrus.SetFormatter(&logrus.TextFormatter{ForceColors: true}) |
|
||||||
logrus.SetOutput(colorable.NewColorableStdout()) |
|
||||||
|
|
||||||
logrus.Info("succeeded") |
|
||||||
logrus.Warn("not correct") |
|
||||||
logrus.Error("something error") |
|
||||||
logrus.Fatal("panic") |
|
||||||
``` |
|
||||||
|
|
||||||
You can compile above code on non-windows OSs. |
|
||||||
|
|
||||||
## Installation |
|
||||||
|
|
||||||
``` |
|
||||||
$ go get github.com/mattn/go-colorable |
|
||||||
``` |
|
||||||
|
|
||||||
# License |
|
||||||
|
|
||||||
MIT |
|
||||||
|
|
||||||
# Author |
|
||||||
|
|
||||||
Yasuhiro Matsumoto (a.k.a mattn) |
|
@ -1,37 +0,0 @@ |
|||||||
// +build appengine
|
|
||||||
|
|
||||||
package colorable |
|
||||||
|
|
||||||
import ( |
|
||||||
"io" |
|
||||||
"os" |
|
||||||
|
|
||||||
_ "github.com/mattn/go-isatty" |
|
||||||
) |
|
||||||
|
|
||||||
// NewColorable returns new instance of Writer which handles escape sequence.
|
|
||||||
func NewColorable(file *os.File) io.Writer { |
|
||||||
if file == nil { |
|
||||||
panic("nil passed instead of *os.File to NewColorable()") |
|
||||||
} |
|
||||||
|
|
||||||
return file |
|
||||||
} |
|
||||||
|
|
||||||
// NewColorableStdout returns new instance of Writer which handles escape sequence for stdout.
|
|
||||||
func NewColorableStdout() io.Writer { |
|
||||||
return os.Stdout |
|
||||||
} |
|
||||||
|
|
||||||
// NewColorableStderr returns new instance of Writer which handles escape sequence for stderr.
|
|
||||||
func NewColorableStderr() io.Writer { |
|
||||||
return os.Stderr |
|
||||||
} |
|
||||||
|
|
||||||
// EnableColorsStdout enable colors if possible.
|
|
||||||
func EnableColorsStdout(enabled *bool) func() { |
|
||||||
if enabled != nil { |
|
||||||
*enabled = true |
|
||||||
} |
|
||||||
return func() {} |
|
||||||
} |
|
@ -1,38 +0,0 @@ |
|||||||
// +build !windows
|
|
||||||
// +build !appengine
|
|
||||||
|
|
||||||
package colorable |
|
||||||
|
|
||||||
import ( |
|
||||||
"io" |
|
||||||
"os" |
|
||||||
|
|
||||||
_ "github.com/mattn/go-isatty" |
|
||||||
) |
|
||||||
|
|
||||||
// NewColorable returns new instance of Writer which handles escape sequence.
|
|
||||||
func NewColorable(file *os.File) io.Writer { |
|
||||||
if file == nil { |
|
||||||
panic("nil passed instead of *os.File to NewColorable()") |
|
||||||
} |
|
||||||
|
|
||||||
return file |
|
||||||
} |
|
||||||
|
|
||||||
// NewColorableStdout returns new instance of Writer which handles escape sequence for stdout.
|
|
||||||
func NewColorableStdout() io.Writer { |
|
||||||
return os.Stdout |
|
||||||
} |
|
||||||
|
|
||||||
// NewColorableStderr returns new instance of Writer which handles escape sequence for stderr.
|
|
||||||
func NewColorableStderr() io.Writer { |
|
||||||
return os.Stderr |
|
||||||
} |
|
||||||
|
|
||||||
// EnableColorsStdout enable colors if possible.
|
|
||||||
func EnableColorsStdout(enabled *bool) func() { |
|
||||||
if enabled != nil { |
|
||||||
*enabled = true |
|
||||||
} |
|
||||||
return func() {} |
|
||||||
} |
|
File diff suppressed because it is too large
Load Diff
@ -1,8 +0,0 @@ |
|||||||
module github.com/mattn/go-colorable |
|
||||||
|
|
||||||
require ( |
|
||||||
github.com/mattn/go-isatty v0.0.12 |
|
||||||
golang.org/x/sys v0.0.0-20200223170610-d5e6a3e2c0ae // indirect |
|
||||||
) |
|
||||||
|
|
||||||
go 1.13 |
|
@ -1,5 +0,0 @@ |
|||||||
github.com/mattn/go-isatty v0.0.12 h1:wuysRhFDzyxgEmMf5xjvJ2M9dZoWAXNNr5LSBS7uHXY= |
|
||||||
github.com/mattn/go-isatty v0.0.12/go.mod h1:cbi8OIDigv2wuxKPP5vlRcQ1OAZbq2CE4Kysco4FUpU= |
|
||||||
golang.org/x/sys v0.0.0-20200116001909-b77594299b42/go.mod h1:h1NjWce9XRLGQEsW7wpKNCjG9DtNlClVuFLEZdDNbEs= |
|
||||||
golang.org/x/sys v0.0.0-20200223170610-d5e6a3e2c0ae h1:/WDfKMnPU+m5M4xB+6x4kaepxRw6jWvR5iDRdvjHgy8= |
|
||||||
golang.org/x/sys v0.0.0-20200223170610-d5e6a3e2c0ae/go.mod h1:h1NjWce9XRLGQEsW7wpKNCjG9DtNlClVuFLEZdDNbEs= |
|
@ -1,12 +0,0 @@ |
|||||||
#!/usr/bin/env bash |
|
||||||
|
|
||||||
set -e |
|
||||||
echo "" > coverage.txt |
|
||||||
|
|
||||||
for d in $(go list ./... | grep -v vendor); do |
|
||||||
go test -race -coverprofile=profile.out -covermode=atomic "$d" |
|
||||||
if [ -f profile.out ]; then |
|
||||||
cat profile.out >> coverage.txt |
|
||||||
rm profile.out |
|
||||||
fi |
|
||||||
done |
|
@ -1,55 +0,0 @@ |
|||||||
package colorable |
|
||||||
|
|
||||||
import ( |
|
||||||
"bytes" |
|
||||||
"io" |
|
||||||
) |
|
||||||
|
|
||||||
// NonColorable holds writer but removes escape sequence.
|
|
||||||
type NonColorable struct { |
|
||||||
out io.Writer |
|
||||||
} |
|
||||||
|
|
||||||
// NewNonColorable returns new instance of Writer which removes escape sequence from Writer.
|
|
||||||
func NewNonColorable(w io.Writer) io.Writer { |
|
||||||
return &NonColorable{out: w} |
|
||||||
} |
|
||||||
|
|
||||||
// Write writes data on console
|
|
||||||
func (w *NonColorable) Write(data []byte) (n int, err error) { |
|
||||||
er := bytes.NewReader(data) |
|
||||||
var bw [1]byte |
|
||||||
loop: |
|
||||||
for { |
|
||||||
c1, err := er.ReadByte() |
|
||||||
if err != nil { |
|
||||||
break loop |
|
||||||
} |
|
||||||
if c1 != 0x1b { |
|
||||||
bw[0] = c1 |
|
||||||
w.out.Write(bw[:]) |
|
||||||
continue |
|
||||||
} |
|
||||||
c2, err := er.ReadByte() |
|
||||||
if err != nil { |
|
||||||
break loop |
|
||||||
} |
|
||||||
if c2 != 0x5b { |
|
||||||
continue |
|
||||||
} |
|
||||||
|
|
||||||
var buf bytes.Buffer |
|
||||||
for { |
|
||||||
c, err := er.ReadByte() |
|
||||||
if err != nil { |
|
||||||
break loop |
|
||||||
} |
|
||||||
if ('a' <= c && c <= 'z') || ('A' <= c && c <= 'Z') || c == '@' { |
|
||||||
break |
|
||||||
} |
|
||||||
buf.Write([]byte(string(c))) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return len(data), nil |
|
||||||
} |
|
@ -1,2 +0,0 @@ |
|||||||
language: go |
|
||||||
go: master |
|
@ -1,21 +0,0 @@ |
|||||||
MIT License |
|
||||||
|
|
||||||
Copyright (c) 2018 Minko Gechev |
|
||||||
|
|
||||||
Permission is hereby granted, free of charge, to any person obtaining a copy |
|
||||||
of this software and associated documentation files (the "Software"), to deal |
|
||||||
in the Software without restriction, including without limitation the rights |
|
||||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell |
|
||||||
copies of the Software, and to permit persons to whom the Software is |
|
||||||
furnished to do so, subject to the following conditions: |
|
||||||
|
|
||||||
The above copyright notice and this permission notice shall be included in all |
|
||||||
copies or substantial portions of the Software. |
|
||||||
|
|
||||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR |
|
||||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, |
|
||||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE |
|
||||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER |
|
||||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, |
|
||||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE |
|
||||||
SOFTWARE. |
|
@ -1,456 +0,0 @@ |
|||||||
package dots |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/build" |
|
||||||
"log" |
|
||||||
"os" |
|
||||||
"path" |
|
||||||
"path/filepath" |
|
||||||
"regexp" |
|
||||||
"runtime" |
|
||||||
"strings" |
|
||||||
) |
|
||||||
|
|
||||||
var ( |
|
||||||
buildContext = build.Default |
|
||||||
goroot = filepath.Clean(runtime.GOROOT()) |
|
||||||
gorootSrc = filepath.Join(goroot, "src") |
|
||||||
) |
|
||||||
|
|
||||||
func flatten(arr [][]string) []string { |
|
||||||
var res []string |
|
||||||
for _, e := range arr { |
|
||||||
res = append(res, e...) |
|
||||||
} |
|
||||||
return res |
|
||||||
} |
|
||||||
|
|
||||||
// Resolve accepts a slice of paths with optional "..." placeholder and a slice with paths to be skipped.
|
|
||||||
// The final result is the set of all files from the selected directories subtracted with
|
|
||||||
// the files in the skip slice.
|
|
||||||
func Resolve(includePatterns, skipPatterns []string) ([]string, error) { |
|
||||||
skip, err := resolvePatterns(skipPatterns) |
|
||||||
filter := newPathFilter(flatten(skip)) |
|
||||||
if err != nil { |
|
||||||
return nil, err |
|
||||||
} |
|
||||||
|
|
||||||
pathSet := map[string]bool{} |
|
||||||
includePackages, err := resolvePatterns(includePatterns) |
|
||||||
include := flatten(includePackages) |
|
||||||
if err != nil { |
|
||||||
return nil, err |
|
||||||
} |
|
||||||
|
|
||||||
var result []string |
|
||||||
for _, i := range include { |
|
||||||
if _, ok := pathSet[i]; !ok && !filter(i) { |
|
||||||
pathSet[i] = true |
|
||||||
result = append(result, i) |
|
||||||
} |
|
||||||
} |
|
||||||
return result, err |
|
||||||
} |
|
||||||
|
|
||||||
// ResolvePackages accepts a slice of paths with optional "..." placeholder and a slice with paths to be skipped.
|
|
||||||
// The final result is the set of all files from the selected directories subtracted with
|
|
||||||
// the files in the skip slice. The difference between `Resolve` and `ResolvePackages`
|
|
||||||
// is that `ResolvePackages` preserves the package structure in the nested slices.
|
|
||||||
func ResolvePackages(includePatterns, skipPatterns []string) ([][]string, error) { |
|
||||||
skip, err := resolvePatterns(skipPatterns) |
|
||||||
filter := newPathFilter(flatten(skip)) |
|
||||||
if err != nil { |
|
||||||
return nil, err |
|
||||||
} |
|
||||||
|
|
||||||
pathSet := map[string]bool{} |
|
||||||
include, err := resolvePatterns(includePatterns) |
|
||||||
if err != nil { |
|
||||||
return nil, err |
|
||||||
} |
|
||||||
|
|
||||||
var result [][]string |
|
||||||
for _, p := range include { |
|
||||||
var packageFiles []string |
|
||||||
for _, f := range p { |
|
||||||
if _, ok := pathSet[f]; !ok && !filter(f) { |
|
||||||
pathSet[f] = true |
|
||||||
packageFiles = append(packageFiles, f) |
|
||||||
} |
|
||||||
} |
|
||||||
result = append(result, packageFiles) |
|
||||||
} |
|
||||||
return result, err |
|
||||||
} |
|
||||||
|
|
||||||
func isDir(filename string) bool { |
|
||||||
fi, err := os.Stat(filename) |
|
||||||
return err == nil && fi.IsDir() |
|
||||||
} |
|
||||||
|
|
||||||
func exists(filename string) bool { |
|
||||||
_, err := os.Stat(filename) |
|
||||||
return err == nil |
|
||||||
} |
|
||||||
|
|
||||||
func resolveDir(dirname string) ([]string, error) { |
|
||||||
pkg, err := build.ImportDir(dirname, 0) |
|
||||||
return resolveImportedPackage(pkg, err) |
|
||||||
} |
|
||||||
|
|
||||||
func resolvePackage(pkgname string) ([]string, error) { |
|
||||||
pkg, err := build.Import(pkgname, ".", 0) |
|
||||||
return resolveImportedPackage(pkg, err) |
|
||||||
} |
|
||||||
|
|
||||||
func resolveImportedPackage(pkg *build.Package, err error) ([]string, error) { |
|
||||||
if err != nil { |
|
||||||
if _, nogo := err.(*build.NoGoError); nogo { |
|
||||||
// Don't complain if the failure is due to no Go source files.
|
|
||||||
return nil, nil |
|
||||||
} |
|
||||||
return nil, err |
|
||||||
} |
|
||||||
|
|
||||||
var files []string |
|
||||||
files = append(files, pkg.GoFiles...) |
|
||||||
files = append(files, pkg.CgoFiles...) |
|
||||||
files = append(files, pkg.TestGoFiles...) |
|
||||||
if pkg.Dir != "." { |
|
||||||
for i, f := range files { |
|
||||||
files[i] = filepath.Join(pkg.Dir, f) |
|
||||||
} |
|
||||||
} |
|
||||||
return files, nil |
|
||||||
} |
|
||||||
|
|
||||||
func resolvePatterns(patterns []string) ([][]string, error) { |
|
||||||
var files [][]string |
|
||||||
for _, pattern := range patterns { |
|
||||||
f, err := resolvePattern(pattern) |
|
||||||
if err != nil { |
|
||||||
return nil, err |
|
||||||
} |
|
||||||
files = append(files, f...) |
|
||||||
} |
|
||||||
return files, nil |
|
||||||
} |
|
||||||
|
|
||||||
func resolvePattern(pattern string) ([][]string, error) { |
|
||||||
// dirsRun, filesRun, and pkgsRun indicate whether golint is applied to
|
|
||||||
// directory, file or package targets. The distinction affects which
|
|
||||||
// checks are run. It is no valid to mix target types.
|
|
||||||
var dirsRun, filesRun, pkgsRun int |
|
||||||
var matches []string |
|
||||||
|
|
||||||
if strings.HasSuffix(pattern, "/...") && isDir(pattern[:len(pattern)-len("/...")]) { |
|
||||||
dirsRun = 1 |
|
||||||
for _, dirname := range matchPackagesInFS(pattern) { |
|
||||||
matches = append(matches, dirname) |
|
||||||
} |
|
||||||
} else if isDir(pattern) { |
|
||||||
dirsRun = 1 |
|
||||||
matches = append(matches, pattern) |
|
||||||
} else if exists(pattern) { |
|
||||||
filesRun = 1 |
|
||||||
matches = append(matches, pattern) |
|
||||||
} else { |
|
||||||
pkgsRun = 1 |
|
||||||
matches = append(matches, pattern) |
|
||||||
} |
|
||||||
|
|
||||||
result := [][]string{} |
|
||||||
switch { |
|
||||||
case dirsRun == 1: |
|
||||||
for _, dir := range matches { |
|
||||||
res, err := resolveDir(dir) |
|
||||||
if err != nil { |
|
||||||
return nil, err |
|
||||||
} |
|
||||||
result = append(result, res) |
|
||||||
} |
|
||||||
case filesRun == 1: |
|
||||||
return [][]string{matches}, nil |
|
||||||
case pkgsRun == 1: |
|
||||||
for _, pkg := range importPaths(matches) { |
|
||||||
res, err := resolvePackage(pkg) |
|
||||||
if err != nil { |
|
||||||
return nil, err |
|
||||||
} |
|
||||||
result = append(result, res) |
|
||||||
} |
|
||||||
} |
|
||||||
return result, nil |
|
||||||
} |
|
||||||
|
|
||||||
func newPathFilter(skip []string) func(string) bool { |
|
||||||
filter := map[string]bool{} |
|
||||||
for _, name := range skip { |
|
||||||
filter[name] = true |
|
||||||
} |
|
||||||
|
|
||||||
return func(path string) bool { |
|
||||||
base := filepath.Base(path) |
|
||||||
if filter[base] || filter[path] { |
|
||||||
return true |
|
||||||
} |
|
||||||
return base != "." && base != ".." && strings.ContainsAny(base[0:1], "_.") |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// importPathsNoDotExpansion returns the import paths to use for the given
|
|
||||||
// command line, but it does no ... expansion.
|
|
||||||
func importPathsNoDotExpansion(args []string) []string { |
|
||||||
if len(args) == 0 { |
|
||||||
return []string{"."} |
|
||||||
} |
|
||||||
var out []string |
|
||||||
for _, a := range args { |
|
||||||
// Arguments are supposed to be import paths, but
|
|
||||||
// as a courtesy to Windows developers, rewrite \ to /
|
|
||||||
// in command-line arguments. Handles .\... and so on.
|
|
||||||
if filepath.Separator == '\\' { |
|
||||||
a = strings.Replace(a, `\`, `/`, -1) |
|
||||||
} |
|
||||||
|
|
||||||
// Put argument in canonical form, but preserve leading ./.
|
|
||||||
if strings.HasPrefix(a, "./") { |
|
||||||
a = "./" + path.Clean(a) |
|
||||||
if a == "./." { |
|
||||||
a = "." |
|
||||||
} |
|
||||||
} else { |
|
||||||
a = path.Clean(a) |
|
||||||
} |
|
||||||
if a == "all" || a == "std" { |
|
||||||
out = append(out, matchPackages(a)...) |
|
||||||
continue |
|
||||||
} |
|
||||||
out = append(out, a) |
|
||||||
} |
|
||||||
return out |
|
||||||
} |
|
||||||
|
|
||||||
// importPaths returns the import paths to use for the given command line.
|
|
||||||
func importPaths(args []string) []string { |
|
||||||
args = importPathsNoDotExpansion(args) |
|
||||||
var out []string |
|
||||||
for _, a := range args { |
|
||||||
if strings.Contains(a, "...") { |
|
||||||
if build.IsLocalImport(a) { |
|
||||||
out = append(out, matchPackagesInFS(a)...) |
|
||||||
} else { |
|
||||||
out = append(out, matchPackages(a)...) |
|
||||||
} |
|
||||||
continue |
|
||||||
} |
|
||||||
out = append(out, a) |
|
||||||
} |
|
||||||
return out |
|
||||||
} |
|
||||||
|
|
||||||
// matchPattern(pattern)(name) reports whether
|
|
||||||
// name matches pattern. Pattern is a limited glob
|
|
||||||
// pattern in which '...' means 'any string' and there
|
|
||||||
// is no other special syntax.
|
|
||||||
func matchPattern(pattern string) func(name string) bool { |
|
||||||
re := regexp.QuoteMeta(pattern) |
|
||||||
re = strings.Replace(re, `\.\.\.`, `.*`, -1) |
|
||||||
// Special case: foo/... matches foo too.
|
|
||||||
if strings.HasSuffix(re, `/.*`) { |
|
||||||
re = re[:len(re)-len(`/.*`)] + `(/.*)?` |
|
||||||
} |
|
||||||
reg := regexp.MustCompile(`^` + re + `$`) |
|
||||||
return func(name string) bool { |
|
||||||
return reg.MatchString(name) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// hasPathPrefix reports whether the path s begins with the
|
|
||||||
// elements in prefix.
|
|
||||||
func hasPathPrefix(s, prefix string) bool { |
|
||||||
switch { |
|
||||||
default: |
|
||||||
return false |
|
||||||
case len(s) == len(prefix): |
|
||||||
return s == prefix |
|
||||||
case len(s) > len(prefix): |
|
||||||
if prefix != "" && prefix[len(prefix)-1] == '/' { |
|
||||||
return strings.HasPrefix(s, prefix) |
|
||||||
} |
|
||||||
return s[len(prefix)] == '/' && s[:len(prefix)] == prefix |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// treeCanMatchPattern(pattern)(name) reports whether
|
|
||||||
// name or children of name can possibly match pattern.
|
|
||||||
// Pattern is the same limited glob accepted by matchPattern.
|
|
||||||
func treeCanMatchPattern(pattern string) func(name string) bool { |
|
||||||
wildCard := false |
|
||||||
if i := strings.Index(pattern, "..."); i >= 0 { |
|
||||||
wildCard = true |
|
||||||
pattern = pattern[:i] |
|
||||||
} |
|
||||||
return func(name string) bool { |
|
||||||
return len(name) <= len(pattern) && hasPathPrefix(pattern, name) || |
|
||||||
wildCard && strings.HasPrefix(name, pattern) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
func matchPackages(pattern string) []string { |
|
||||||
match := func(string) bool { return true } |
|
||||||
treeCanMatch := func(string) bool { return true } |
|
||||||
if pattern != "all" && pattern != "std" { |
|
||||||
match = matchPattern(pattern) |
|
||||||
treeCanMatch = treeCanMatchPattern(pattern) |
|
||||||
} |
|
||||||
|
|
||||||
have := map[string]bool{ |
|
||||||
"builtin": true, // ignore pseudo-package that exists only for documentation
|
|
||||||
} |
|
||||||
if !buildContext.CgoEnabled { |
|
||||||
have["runtime/cgo"] = true // ignore during walk
|
|
||||||
} |
|
||||||
var pkgs []string |
|
||||||
|
|
||||||
// Commands
|
|
||||||
cmd := filepath.Join(goroot, "src/cmd") + string(filepath.Separator) |
|
||||||
filepath.Walk(cmd, func(path string, fi os.FileInfo, err error) error { |
|
||||||
if err != nil || !fi.IsDir() || path == cmd { |
|
||||||
return nil |
|
||||||
} |
|
||||||
name := path[len(cmd):] |
|
||||||
if !treeCanMatch(name) { |
|
||||||
return filepath.SkipDir |
|
||||||
} |
|
||||||
// Commands are all in cmd/, not in subdirectories.
|
|
||||||
if strings.Contains(name, string(filepath.Separator)) { |
|
||||||
return filepath.SkipDir |
|
||||||
} |
|
||||||
|
|
||||||
// We use, e.g., cmd/gofmt as the pseudo import path for gofmt.
|
|
||||||
name = "cmd/" + name |
|
||||||
if have[name] { |
|
||||||
return nil |
|
||||||
} |
|
||||||
have[name] = true |
|
||||||
if !match(name) { |
|
||||||
return nil |
|
||||||
} |
|
||||||
_, err = buildContext.ImportDir(path, 0) |
|
||||||
if err != nil { |
|
||||||
if _, noGo := err.(*build.NoGoError); !noGo { |
|
||||||
log.Print(err) |
|
||||||
} |
|
||||||
return nil |
|
||||||
} |
|
||||||
pkgs = append(pkgs, name) |
|
||||||
return nil |
|
||||||
}) |
|
||||||
|
|
||||||
for _, src := range buildContext.SrcDirs() { |
|
||||||
if (pattern == "std" || pattern == "cmd") && src != gorootSrc { |
|
||||||
continue |
|
||||||
} |
|
||||||
src = filepath.Clean(src) + string(filepath.Separator) |
|
||||||
root := src |
|
||||||
if pattern == "cmd" { |
|
||||||
root += "cmd" + string(filepath.Separator) |
|
||||||
} |
|
||||||
filepath.Walk(root, func(path string, fi os.FileInfo, err error) error { |
|
||||||
if err != nil || !fi.IsDir() || path == src { |
|
||||||
return nil |
|
||||||
} |
|
||||||
|
|
||||||
// Avoid .foo, _foo, and testdata directory trees.
|
|
||||||
_, elem := filepath.Split(path) |
|
||||||
if strings.HasPrefix(elem, ".") || strings.HasPrefix(elem, "_") || elem == "testdata" { |
|
||||||
return filepath.SkipDir |
|
||||||
} |
|
||||||
|
|
||||||
name := filepath.ToSlash(path[len(src):]) |
|
||||||
if pattern == "std" && (strings.Contains(name, ".") || name == "cmd") { |
|
||||||
// The name "std" is only the standard library.
|
|
||||||
// If the name is cmd, it's the root of the command tree.
|
|
||||||
return filepath.SkipDir |
|
||||||
} |
|
||||||
if !treeCanMatch(name) { |
|
||||||
return filepath.SkipDir |
|
||||||
} |
|
||||||
if have[name] { |
|
||||||
return nil |
|
||||||
} |
|
||||||
have[name] = true |
|
||||||
if !match(name) { |
|
||||||
return nil |
|
||||||
} |
|
||||||
_, err = buildContext.ImportDir(path, 0) |
|
||||||
if err != nil { |
|
||||||
if _, noGo := err.(*build.NoGoError); noGo { |
|
||||||
return nil |
|
||||||
} |
|
||||||
} |
|
||||||
pkgs = append(pkgs, name) |
|
||||||
return nil |
|
||||||
}) |
|
||||||
} |
|
||||||
return pkgs |
|
||||||
} |
|
||||||
|
|
||||||
func matchPackagesInFS(pattern string) []string { |
|
||||||
// Find directory to begin the scan.
|
|
||||||
// Could be smarter but this one optimization
|
|
||||||
// is enough for now, since ... is usually at the
|
|
||||||
// end of a path.
|
|
||||||
i := strings.Index(pattern, "...") |
|
||||||
dir, _ := path.Split(pattern[:i]) |
|
||||||
|
|
||||||
// pattern begins with ./ or ../.
|
|
||||||
// path.Clean will discard the ./ but not the ../.
|
|
||||||
// We need to preserve the ./ for pattern matching
|
|
||||||
// and in the returned import paths.
|
|
||||||
prefix := "" |
|
||||||
if strings.HasPrefix(pattern, "./") { |
|
||||||
prefix = "./" |
|
||||||
} |
|
||||||
match := matchPattern(pattern) |
|
||||||
|
|
||||||
var pkgs []string |
|
||||||
filepath.Walk(dir, func(path string, fi os.FileInfo, err error) error { |
|
||||||
if err != nil || !fi.IsDir() { |
|
||||||
return nil |
|
||||||
} |
|
||||||
if path == dir { |
|
||||||
// filepath.Walk starts at dir and recurses. For the recursive case,
|
|
||||||
// the path is the result of filepath.Join, which calls filepath.Clean.
|
|
||||||
// The initial case is not Cleaned, though, so we do this explicitly.
|
|
||||||
//
|
|
||||||
// This converts a path like "./io/" to "io". Without this step, running
|
|
||||||
// "cd $GOROOT/src/pkg; go list ./io/..." would incorrectly skip the io
|
|
||||||
// package, because prepending the prefix "./" to the unclean path would
|
|
||||||
// result in "././io", and match("././io") returns false.
|
|
||||||
path = filepath.Clean(path) |
|
||||||
} |
|
||||||
|
|
||||||
// Avoid .foo, _foo, and testdata directory trees, but do not avoid "." or "..".
|
|
||||||
_, elem := filepath.Split(path) |
|
||||||
dot := strings.HasPrefix(elem, ".") && elem != "." && elem != ".." |
|
||||||
if dot || strings.HasPrefix(elem, "_") || elem == "testdata" { |
|
||||||
return filepath.SkipDir |
|
||||||
} |
|
||||||
|
|
||||||
name := prefix + filepath.ToSlash(path) |
|
||||||
if !match(name) { |
|
||||||
return nil |
|
||||||
} |
|
||||||
if _, err = build.ImportDir(path, 0); err != nil { |
|
||||||
if _, noGo := err.(*build.NoGoError); !noGo { |
|
||||||
log.Print(err) |
|
||||||
} |
|
||||||
return nil |
|
||||||
} |
|
||||||
pkgs = append(pkgs, name) |
|
||||||
return nil |
|
||||||
}) |
|
||||||
return pkgs |
|
||||||
} |
|
@ -1,21 +0,0 @@ |
|||||||
MIT License |
|
||||||
|
|
||||||
Copyright (c) 2018 Minko Gechev |
|
||||||
|
|
||||||
Permission is hereby granted, free of charge, to any person obtaining a copy |
|
||||||
of this software and associated documentation files (the "Software"), to deal |
|
||||||
in the Software without restriction, including without limitation the rights |
|
||||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell |
|
||||||
copies of the Software, and to permit persons to whom the Software is |
|
||||||
furnished to do so, subject to the following conditions: |
|
||||||
|
|
||||||
The above copyright notice and this permission notice shall be included in all |
|
||||||
copies or substantial portions of the Software. |
|
||||||
|
|
||||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR |
|
||||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, |
|
||||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE |
|
||||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER |
|
||||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, |
|
||||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE |
|
||||||
SOFTWARE. |
|
@ -1,76 +0,0 @@ |
|||||||
package formatter |
|
||||||
|
|
||||||
import ( |
|
||||||
"bytes" |
|
||||||
"encoding/xml" |
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
plainTemplate "text/template" |
|
||||||
) |
|
||||||
|
|
||||||
// Checkstyle is an implementation of the Formatter interface
|
|
||||||
// which formats the errors to Checkstyle-like format.
|
|
||||||
type Checkstyle struct { |
|
||||||
Metadata lint.FormatterMetadata |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the name of the formatter
|
|
||||||
func (f *Checkstyle) Name() string { |
|
||||||
return "checkstyle" |
|
||||||
} |
|
||||||
|
|
||||||
type issue struct { |
|
||||||
Line int |
|
||||||
Col int |
|
||||||
What string |
|
||||||
Confidence float64 |
|
||||||
Severity lint.Severity |
|
||||||
RuleName string |
|
||||||
} |
|
||||||
|
|
||||||
// Format formats the failures gotten from the lint.
|
|
||||||
func (f *Checkstyle) Format(failures <-chan lint.Failure, config lint.Config) (string, error) { |
|
||||||
var issues = map[string][]issue{} |
|
||||||
for failure := range failures { |
|
||||||
buf := new(bytes.Buffer) |
|
||||||
xml.Escape(buf, []byte(failure.Failure)) |
|
||||||
what := buf.String() |
|
||||||
iss := issue{ |
|
||||||
Line: failure.Position.Start.Line, |
|
||||||
Col: failure.Position.Start.Column, |
|
||||||
What: what, |
|
||||||
Confidence: failure.Confidence, |
|
||||||
Severity: severity(config, failure), |
|
||||||
RuleName: failure.RuleName, |
|
||||||
} |
|
||||||
fn := failure.GetFilename() |
|
||||||
if issues[fn] == nil { |
|
||||||
issues[fn] = make([]issue, 0) |
|
||||||
} |
|
||||||
issues[fn] = append(issues[fn], iss) |
|
||||||
} |
|
||||||
|
|
||||||
t, err := plainTemplate.New("revive").Parse(checkstyleTemplate) |
|
||||||
if err != nil { |
|
||||||
return "", err |
|
||||||
} |
|
||||||
|
|
||||||
buf := new(bytes.Buffer) |
|
||||||
|
|
||||||
err = t.Execute(buf, issues) |
|
||||||
if err != nil { |
|
||||||
return "", err |
|
||||||
} |
|
||||||
|
|
||||||
return buf.String(), nil |
|
||||||
} |
|
||||||
|
|
||||||
const checkstyleTemplate = `<?xml version='1.0' encoding='UTF-8'?> |
|
||||||
<checkstyle version="5.0"> |
|
||||||
{{- range $k, $v := . }} |
|
||||||
<file name="{{ $k }}"> |
|
||||||
{{- range $i, $issue := $v }} |
|
||||||
<error line="{{ $issue.Line }}" column="{{ $issue.Col }}" message="{{ $issue.What }} (confidence {{ $issue.Confidence}})" severity="{{ $issue.Severity }}" source="revive/{{ $issue.RuleName }}"/> |
|
||||||
{{- end }} |
|
||||||
</file> |
|
||||||
{{- end }} |
|
||||||
</checkstyle>` |
|
@ -1,26 +0,0 @@ |
|||||||
package formatter |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// Default is an implementation of the Formatter interface
|
|
||||||
// which formats the errors to text.
|
|
||||||
type Default struct { |
|
||||||
Metadata lint.FormatterMetadata |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the name of the formatter
|
|
||||||
func (f *Default) Name() string { |
|
||||||
return "default" |
|
||||||
} |
|
||||||
|
|
||||||
// Format formats the failures gotten from the lint.
|
|
||||||
func (f *Default) Format(failures <-chan lint.Failure, _ lint.Config) (string, error) { |
|
||||||
for failure := range failures { |
|
||||||
fmt.Printf("%v: %s\n", failure.Position.Start, failure.Failure) |
|
||||||
} |
|
||||||
return "", nil |
|
||||||
} |
|
@ -1,149 +0,0 @@ |
|||||||
package formatter |
|
||||||
|
|
||||||
import ( |
|
||||||
"bytes" |
|
||||||
"fmt" |
|
||||||
"sort" |
|
||||||
|
|
||||||
"github.com/fatih/color" |
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
"github.com/olekukonko/tablewriter" |
|
||||||
) |
|
||||||
|
|
||||||
var newLines = map[rune]bool{ |
|
||||||
0x000A: true, |
|
||||||
0x000B: true, |
|
||||||
0x000C: true, |
|
||||||
0x000D: true, |
|
||||||
0x0085: true, |
|
||||||
0x2028: true, |
|
||||||
0x2029: true, |
|
||||||
} |
|
||||||
|
|
||||||
func getErrorEmoji() string { |
|
||||||
return color.RedString("✘") |
|
||||||
} |
|
||||||
|
|
||||||
func getWarningEmoji() string { |
|
||||||
return color.YellowString("⚠") |
|
||||||
} |
|
||||||
|
|
||||||
// Friendly is an implementation of the Formatter interface
|
|
||||||
// which formats the errors to JSON.
|
|
||||||
type Friendly struct { |
|
||||||
Metadata lint.FormatterMetadata |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the name of the formatter
|
|
||||||
func (f *Friendly) Name() string { |
|
||||||
return "friendly" |
|
||||||
} |
|
||||||
|
|
||||||
// Format formats the failures gotten from the lint.
|
|
||||||
func (f *Friendly) Format(failures <-chan lint.Failure, config lint.Config) (string, error) { |
|
||||||
errorMap := map[string]int{} |
|
||||||
warningMap := map[string]int{} |
|
||||||
totalErrors := 0 |
|
||||||
totalWarnings := 0 |
|
||||||
for failure := range failures { |
|
||||||
sev := severity(config, failure) |
|
||||||
f.printFriendlyFailure(failure, sev) |
|
||||||
if sev == lint.SeverityWarning { |
|
||||||
warningMap[failure.RuleName] = warningMap[failure.RuleName] + 1 |
|
||||||
totalWarnings++ |
|
||||||
} |
|
||||||
if sev == lint.SeverityError { |
|
||||||
errorMap[failure.RuleName] = errorMap[failure.RuleName] + 1 |
|
||||||
totalErrors++ |
|
||||||
} |
|
||||||
} |
|
||||||
f.printSummary(totalErrors, totalWarnings) |
|
||||||
f.printStatistics(color.RedString("Errors:"), errorMap) |
|
||||||
f.printStatistics(color.YellowString("Warnings:"), warningMap) |
|
||||||
return "", nil |
|
||||||
} |
|
||||||
|
|
||||||
func (f *Friendly) printFriendlyFailure(failure lint.Failure, severity lint.Severity) { |
|
||||||
f.printHeaderRow(failure, severity) |
|
||||||
f.printFilePosition(failure) |
|
||||||
fmt.Println() |
|
||||||
fmt.Println() |
|
||||||
} |
|
||||||
|
|
||||||
func (f *Friendly) printHeaderRow(failure lint.Failure, severity lint.Severity) { |
|
||||||
emoji := getWarningEmoji() |
|
||||||
if severity == lint.SeverityError { |
|
||||||
emoji = getErrorEmoji() |
|
||||||
} |
|
||||||
fmt.Print(f.table([][]string{{emoji, "https://revive.run/r#" + failure.RuleName, color.GreenString(failure.Failure)}})) |
|
||||||
} |
|
||||||
|
|
||||||
func (f *Friendly) printFilePosition(failure lint.Failure) { |
|
||||||
fmt.Printf(" %s:%d:%d", failure.GetFilename(), failure.Position.Start.Line, failure.Position.Start.Column) |
|
||||||
} |
|
||||||
|
|
||||||
type statEntry struct { |
|
||||||
name string |
|
||||||
failures int |
|
||||||
} |
|
||||||
|
|
||||||
func (f *Friendly) printSummary(errors, warnings int) { |
|
||||||
emoji := getWarningEmoji() |
|
||||||
if errors > 0 { |
|
||||||
emoji = getErrorEmoji() |
|
||||||
} |
|
||||||
problemsLabel := "problems" |
|
||||||
if errors+warnings == 1 { |
|
||||||
problemsLabel = "problem" |
|
||||||
} |
|
||||||
warningsLabel := "warnings" |
|
||||||
if warnings == 1 { |
|
||||||
warningsLabel = "warning" |
|
||||||
} |
|
||||||
errorsLabel := "errors" |
|
||||||
if errors == 1 { |
|
||||||
errorsLabel = "error" |
|
||||||
} |
|
||||||
str := fmt.Sprintf("%d %s (%d %s, %d %s)", errors+warnings, problemsLabel, errors, errorsLabel, warnings, warningsLabel) |
|
||||||
if errors > 0 { |
|
||||||
fmt.Printf("%s %s\n", emoji, color.RedString(str)) |
|
||||||
fmt.Println() |
|
||||||
return |
|
||||||
} |
|
||||||
if warnings > 0 { |
|
||||||
fmt.Printf("%s %s\n", emoji, color.YellowString(str)) |
|
||||||
fmt.Println() |
|
||||||
return |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
func (f *Friendly) printStatistics(header string, stats map[string]int) { |
|
||||||
if len(stats) == 0 { |
|
||||||
return |
|
||||||
} |
|
||||||
var data []statEntry |
|
||||||
for name, total := range stats { |
|
||||||
data = append(data, statEntry{name, total}) |
|
||||||
} |
|
||||||
sort.Slice(data, func(i, j int) bool { |
|
||||||
return data[i].failures > data[j].failures |
|
||||||
}) |
|
||||||
formatted := [][]string{} |
|
||||||
for _, entry := range data { |
|
||||||
formatted = append(formatted, []string{color.GreenString(fmt.Sprintf("%d", entry.failures)), entry.name}) |
|
||||||
} |
|
||||||
fmt.Println(header) |
|
||||||
fmt.Println(f.table(formatted)) |
|
||||||
} |
|
||||||
|
|
||||||
func (f *Friendly) table(rows [][]string) string { |
|
||||||
buf := new(bytes.Buffer) |
|
||||||
table := tablewriter.NewWriter(buf) |
|
||||||
table.SetBorder(false) |
|
||||||
table.SetColumnSeparator("") |
|
||||||
table.SetRowSeparator("") |
|
||||||
table.SetAutoWrapText(false) |
|
||||||
table.AppendBulk(rows) |
|
||||||
table.Render() |
|
||||||
return buf.String() |
|
||||||
} |
|
@ -1,40 +0,0 @@ |
|||||||
package formatter |
|
||||||
|
|
||||||
import ( |
|
||||||
"encoding/json" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// JSON is an implementation of the Formatter interface
|
|
||||||
// which formats the errors to JSON.
|
|
||||||
type JSON struct { |
|
||||||
Metadata lint.FormatterMetadata |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the name of the formatter
|
|
||||||
func (f *JSON) Name() string { |
|
||||||
return "json" |
|
||||||
} |
|
||||||
|
|
||||||
// jsonObject defines a JSON object of an failure
|
|
||||||
type jsonObject struct { |
|
||||||
Severity lint.Severity |
|
||||||
lint.Failure `json:",inline"` |
|
||||||
} |
|
||||||
|
|
||||||
// Format formats the failures gotten from the lint.
|
|
||||||
func (f *JSON) Format(failures <-chan lint.Failure, config lint.Config) (string, error) { |
|
||||||
var slice []jsonObject |
|
||||||
for failure := range failures { |
|
||||||
obj := jsonObject{} |
|
||||||
obj.Severity = severity(config, failure) |
|
||||||
obj.Failure = failure |
|
||||||
slice = append(slice, obj) |
|
||||||
} |
|
||||||
result, err := json.Marshal(slice) |
|
||||||
if err != nil { |
|
||||||
return "", err |
|
||||||
} |
|
||||||
return string(result), err |
|
||||||
} |
|
@ -1,34 +0,0 @@ |
|||||||
package formatter |
|
||||||
|
|
||||||
import ( |
|
||||||
"encoding/json" |
|
||||||
"os" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// NDJSON is an implementation of the Formatter interface
|
|
||||||
// which formats the errors to NDJSON stream.
|
|
||||||
type NDJSON struct { |
|
||||||
Metadata lint.FormatterMetadata |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the name of the formatter
|
|
||||||
func (f *NDJSON) Name() string { |
|
||||||
return "ndjson" |
|
||||||
} |
|
||||||
|
|
||||||
// Format formats the failures gotten from the lint.
|
|
||||||
func (f *NDJSON) Format(failures <-chan lint.Failure, config lint.Config) (string, error) { |
|
||||||
enc := json.NewEncoder(os.Stdout) |
|
||||||
for failure := range failures { |
|
||||||
obj := jsonObject{} |
|
||||||
obj.Severity = severity(config, failure) |
|
||||||
obj.Failure = failure |
|
||||||
err := enc.Encode(obj) |
|
||||||
if err != nil { |
|
||||||
return "", err |
|
||||||
} |
|
||||||
} |
|
||||||
return "", nil |
|
||||||
} |
|
@ -1,26 +0,0 @@ |
|||||||
package formatter |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// Plain is an implementation of the Formatter interface
|
|
||||||
// which formats the errors to JSON.
|
|
||||||
type Plain struct { |
|
||||||
Metadata lint.FormatterMetadata |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the name of the formatter
|
|
||||||
func (f *Plain) Name() string { |
|
||||||
return "plain" |
|
||||||
} |
|
||||||
|
|
||||||
// Format formats the failures gotten from the lint.
|
|
||||||
func (f *Plain) Format(failures <-chan lint.Failure, _ lint.Config) (string, error) { |
|
||||||
for failure := range failures { |
|
||||||
fmt.Printf("%v: %s %s\n", failure.Position.Start, failure.Failure, "https://revive.run/r#"+failure.RuleName) |
|
||||||
} |
|
||||||
return "", nil |
|
||||||
} |
|
@ -1,107 +0,0 @@ |
|||||||
package formatter |
|
||||||
|
|
||||||
import ( |
|
||||||
"bytes" |
|
||||||
"fmt" |
|
||||||
"strings" |
|
||||||
|
|
||||||
"github.com/chavacava/garif" |
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// Sarif is an implementation of the Formatter interface
|
|
||||||
// which formats revive failures into SARIF format.
|
|
||||||
type Sarif struct { |
|
||||||
Metadata lint.FormatterMetadata |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the name of the formatter
|
|
||||||
func (f *Sarif) Name() string { |
|
||||||
return "sarif" |
|
||||||
} |
|
||||||
|
|
||||||
const reviveSite = "https://revive.run" |
|
||||||
|
|
||||||
// Format formats the failures gotten from the lint.
|
|
||||||
func (f *Sarif) Format(failures <-chan lint.Failure, cfg lint.Config) (string, error) { |
|
||||||
sarifLog := newReviveRunLog(cfg) |
|
||||||
|
|
||||||
for failure := range failures { |
|
||||||
sarifLog.AddResult(failure) |
|
||||||
} |
|
||||||
|
|
||||||
buf := new(bytes.Buffer) |
|
||||||
sarifLog.PrettyWrite(buf) |
|
||||||
|
|
||||||
return buf.String(), nil |
|
||||||
} |
|
||||||
|
|
||||||
type reviveRunLog struct { |
|
||||||
*garif.LogFile |
|
||||||
run *garif.Run |
|
||||||
rules map[string]lint.RuleConfig |
|
||||||
} |
|
||||||
|
|
||||||
func newReviveRunLog(cfg lint.Config) *reviveRunLog { |
|
||||||
run := garif.NewRun(garif.NewTool(garif.NewDriver("revive").WithInformationUri(reviveSite))) |
|
||||||
log := garif.NewLogFile([]*garif.Run{run}, garif.Version210) |
|
||||||
|
|
||||||
reviveLog := &reviveRunLog{ |
|
||||||
log, |
|
||||||
run, |
|
||||||
cfg.Rules, |
|
||||||
} |
|
||||||
|
|
||||||
reviveLog.addRules(cfg.Rules) |
|
||||||
|
|
||||||
return reviveLog |
|
||||||
} |
|
||||||
|
|
||||||
func (l *reviveRunLog) addRules(cfg map[string]lint.RuleConfig) { |
|
||||||
for name, ruleCfg := range cfg { |
|
||||||
rule := garif.NewRule(name).WithHelpUri(reviveSite + "/r#" + name) |
|
||||||
setRuleProperties(rule, ruleCfg) |
|
||||||
driver := l.run.Tool.Driver |
|
||||||
|
|
||||||
if driver.Rules == nil { |
|
||||||
driver.Rules = []*garif.ReportingDescriptor{rule} |
|
||||||
return |
|
||||||
} |
|
||||||
|
|
||||||
driver.Rules = append(driver.Rules, rule) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
func (l *reviveRunLog) AddResult(failure lint.Failure) { |
|
||||||
positiveOrZero := func(x int) int { |
|
||||||
if x > 0 { |
|
||||||
return x |
|
||||||
} |
|
||||||
return 0 |
|
||||||
} |
|
||||||
position := failure.Position |
|
||||||
filename := position.Start.Filename |
|
||||||
line := positiveOrZero(position.Start.Line - 1) // https://docs.oasis-open.org/sarif/sarif/v2.1.0/csprd01/sarif-v2.1.0-csprd01.html#def_line
|
|
||||||
column := positiveOrZero(position.Start.Column - 1) // https://docs.oasis-open.org/sarif/sarif/v2.1.0/csprd01/sarif-v2.1.0-csprd01.html#def_column
|
|
||||||
|
|
||||||
result := garif.NewResult(garif.NewMessageFromText(failure.Failure)) |
|
||||||
location := garif.NewLocation().WithURI(filename).WithLineColumn(line, column) |
|
||||||
result.Locations = append(result.Locations, location) |
|
||||||
result.RuleId = failure.RuleName |
|
||||||
result.Level = l.rules[failure.RuleName].Severity |
|
||||||
|
|
||||||
l.run.Results = append(l.run.Results, result) |
|
||||||
} |
|
||||||
|
|
||||||
func setRuleProperties(sarifRule *garif.ReportingDescriptor, lintRule lint.RuleConfig) { |
|
||||||
arguments := make([]string, len(lintRule.Arguments)) |
|
||||||
for i, arg := range lintRule.Arguments { |
|
||||||
arguments[i] = fmt.Sprintf("%+v", arg) |
|
||||||
} |
|
||||||
|
|
||||||
if len(arguments) > 0 { |
|
||||||
sarifRule.WithProperties("arguments", strings.Join(arguments, ",")) |
|
||||||
} |
|
||||||
|
|
||||||
sarifRule.WithProperties("severity", string(lintRule.Severity)) |
|
||||||
} |
|
@ -1,13 +0,0 @@ |
|||||||
package formatter |
|
||||||
|
|
||||||
import "github.com/mgechev/revive/lint" |
|
||||||
|
|
||||||
func severity(config lint.Config, failure lint.Failure) lint.Severity { |
|
||||||
if config, ok := config.Rules[failure.RuleName]; ok && config.Severity == lint.SeverityError { |
|
||||||
return lint.SeverityError |
|
||||||
} |
|
||||||
if config, ok := config.Directives[failure.RuleName]; ok && config.Severity == lint.SeverityError { |
|
||||||
return lint.SeverityError |
|
||||||
} |
|
||||||
return lint.SeverityWarning |
|
||||||
} |
|
@ -1,89 +0,0 @@ |
|||||||
package formatter |
|
||||||
|
|
||||||
import ( |
|
||||||
"bytes" |
|
||||||
"fmt" |
|
||||||
|
|
||||||
"github.com/fatih/color" |
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
"github.com/olekukonko/tablewriter" |
|
||||||
) |
|
||||||
|
|
||||||
// Stylish is an implementation of the Formatter interface
|
|
||||||
// which formats the errors to JSON.
|
|
||||||
type Stylish struct { |
|
||||||
Metadata lint.FormatterMetadata |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the name of the formatter
|
|
||||||
func (f *Stylish) Name() string { |
|
||||||
return "stylish" |
|
||||||
} |
|
||||||
|
|
||||||
func formatFailure(failure lint.Failure, severity lint.Severity) []string { |
|
||||||
fString := color.CyanString(failure.Failure) |
|
||||||
fName := color.RedString("https://revive.run/r#" + failure.RuleName) |
|
||||||
lineColumn := failure.Position |
|
||||||
pos := fmt.Sprintf("(%d, %d)", lineColumn.Start.Line, lineColumn.Start.Column) |
|
||||||
if severity == lint.SeverityWarning { |
|
||||||
fName = color.YellowString("https://revive.run/r#" + failure.RuleName) |
|
||||||
} |
|
||||||
return []string{failure.GetFilename(), pos, fName, fString} |
|
||||||
} |
|
||||||
|
|
||||||
// Format formats the failures gotten from the lint.
|
|
||||||
func (f *Stylish) Format(failures <-chan lint.Failure, config lint.Config) (string, error) { |
|
||||||
var result [][]string |
|
||||||
var totalErrors = 0 |
|
||||||
var total = 0 |
|
||||||
|
|
||||||
for f := range failures { |
|
||||||
total++ |
|
||||||
currentType := severity(config, f) |
|
||||||
if currentType == lint.SeverityError { |
|
||||||
totalErrors++ |
|
||||||
} |
|
||||||
result = append(result, formatFailure(f, lint.Severity(currentType))) |
|
||||||
} |
|
||||||
ps := "problems" |
|
||||||
if total == 1 { |
|
||||||
ps = "problem" |
|
||||||
} |
|
||||||
|
|
||||||
fileReport := make(map[string][][]string) |
|
||||||
|
|
||||||
for _, row := range result { |
|
||||||
if _, ok := fileReport[row[0]]; !ok { |
|
||||||
fileReport[row[0]] = [][]string{} |
|
||||||
} |
|
||||||
|
|
||||||
fileReport[row[0]] = append(fileReport[row[0]], []string{row[1], row[2], row[3]}) |
|
||||||
} |
|
||||||
|
|
||||||
output := "" |
|
||||||
for filename, val := range fileReport { |
|
||||||
buf := new(bytes.Buffer) |
|
||||||
table := tablewriter.NewWriter(buf) |
|
||||||
table.SetBorder(false) |
|
||||||
table.SetColumnSeparator("") |
|
||||||
table.SetRowSeparator("") |
|
||||||
table.SetAutoWrapText(false) |
|
||||||
table.AppendBulk(val) |
|
||||||
table.Render() |
|
||||||
c := color.New(color.Underline) |
|
||||||
output += c.SprintfFunc()(filename + "\n") |
|
||||||
output += buf.String() + "\n" |
|
||||||
} |
|
||||||
|
|
||||||
suffix := fmt.Sprintf(" %d %s (%d errors) (%d warnings)", total, ps, totalErrors, total-totalErrors) |
|
||||||
|
|
||||||
if total > 0 && totalErrors > 0 { |
|
||||||
suffix = color.RedString("\n ✖" + suffix) |
|
||||||
} else if total > 0 && totalErrors == 0 { |
|
||||||
suffix = color.YellowString("\n ✖" + suffix) |
|
||||||
} else { |
|
||||||
suffix, output = "", "" |
|
||||||
} |
|
||||||
|
|
||||||
return output + suffix, nil |
|
||||||
} |
|
@ -1,27 +0,0 @@ |
|||||||
package formatter |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// Unix is an implementation of the Formatter interface
|
|
||||||
// which formats the errors to a simple line based error format
|
|
||||||
// main.go:24:9: [errorf] should replace errors.New(fmt.Sprintf(...)) with fmt.Errorf(...)
|
|
||||||
type Unix struct { |
|
||||||
Metadata lint.FormatterMetadata |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the name of the formatter
|
|
||||||
func (f *Unix) Name() string { |
|
||||||
return "unix" |
|
||||||
} |
|
||||||
|
|
||||||
// Format formats the failures gotten from the lint.
|
|
||||||
func (f *Unix) Format(failures <-chan lint.Failure, _ lint.Config) (string, error) { |
|
||||||
for failure := range failures { |
|
||||||
fmt.Printf("%v: [%s] %s\n", failure.Position.Start, failure.RuleName, failure.Failure) |
|
||||||
} |
|
||||||
return "", nil |
|
||||||
} |
|
@ -1,33 +0,0 @@ |
|||||||
package lint |
|
||||||
|
|
||||||
// Arguments is type used for the arguments of a rule.
|
|
||||||
type Arguments = []interface{} |
|
||||||
|
|
||||||
// RuleConfig is type used for the rule configuration.
|
|
||||||
type RuleConfig struct { |
|
||||||
Arguments Arguments |
|
||||||
Severity Severity |
|
||||||
} |
|
||||||
|
|
||||||
// RulesConfig defines the config for all rules.
|
|
||||||
type RulesConfig = map[string]RuleConfig |
|
||||||
|
|
||||||
// DirectiveConfig is type used for the linter directive configuration.
|
|
||||||
type DirectiveConfig struct { |
|
||||||
Severity Severity |
|
||||||
} |
|
||||||
|
|
||||||
// DirectivesConfig defines the config for all directives.
|
|
||||||
type DirectivesConfig = map[string]DirectiveConfig |
|
||||||
|
|
||||||
// Config defines the config of the linter.
|
|
||||||
type Config struct { |
|
||||||
IgnoreGeneratedHeader bool `toml:"ignoreGeneratedHeader"` |
|
||||||
Confidence float64 |
|
||||||
Severity Severity |
|
||||||
Rules RulesConfig `toml:"rule"` |
|
||||||
ErrorCode int `toml:"errorCode"` |
|
||||||
WarningCode int `toml:"warningCode"` |
|
||||||
Directives DirectivesConfig `toml:"directive"` |
|
||||||
Exclude []string `toml:"exclude"` |
|
||||||
} |
|
@ -1,39 +0,0 @@ |
|||||||
package lint |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
"go/token" |
|
||||||
) |
|
||||||
|
|
||||||
const ( |
|
||||||
// SeverityWarning declares failures of type warning
|
|
||||||
SeverityWarning = "warning" |
|
||||||
// SeverityError declares failures of type error.
|
|
||||||
SeverityError = "error" |
|
||||||
) |
|
||||||
|
|
||||||
// Severity is the type for the failure types.
|
|
||||||
type Severity string |
|
||||||
|
|
||||||
// FailurePosition returns the failure position
|
|
||||||
type FailurePosition struct { |
|
||||||
Start token.Position |
|
||||||
End token.Position |
|
||||||
} |
|
||||||
|
|
||||||
// Failure defines a struct for a linting failure.
|
|
||||||
type Failure struct { |
|
||||||
Failure string |
|
||||||
RuleName string |
|
||||||
Category string |
|
||||||
Position FailurePosition |
|
||||||
Node ast.Node `json:"-"` |
|
||||||
Confidence float64 |
|
||||||
// For future use
|
|
||||||
ReplacementLine string |
|
||||||
} |
|
||||||
|
|
||||||
// GetFilename returns the filename.
|
|
||||||
func (f *Failure) GetFilename() string { |
|
||||||
return f.Position.Start.Filename |
|
||||||
} |
|
@ -1,278 +0,0 @@ |
|||||||
package lint |
|
||||||
|
|
||||||
import ( |
|
||||||
"bytes" |
|
||||||
"go/ast" |
|
||||||
"go/parser" |
|
||||||
"go/printer" |
|
||||||
"go/token" |
|
||||||
"go/types" |
|
||||||
"math" |
|
||||||
"regexp" |
|
||||||
"strings" |
|
||||||
) |
|
||||||
|
|
||||||
// File abstraction used for representing files.
|
|
||||||
type File struct { |
|
||||||
Name string |
|
||||||
Pkg *Package |
|
||||||
content []byte |
|
||||||
AST *ast.File |
|
||||||
} |
|
||||||
|
|
||||||
// IsTest returns if the file contains tests.
|
|
||||||
func (f *File) IsTest() bool { return strings.HasSuffix(f.Name, "_test.go") } |
|
||||||
|
|
||||||
// Content returns the file's content.
|
|
||||||
func (f *File) Content() []byte { |
|
||||||
return f.content |
|
||||||
} |
|
||||||
|
|
||||||
// NewFile creates a new file
|
|
||||||
func NewFile(name string, content []byte, pkg *Package) (*File, error) { |
|
||||||
f, err := parser.ParseFile(pkg.fset, name, content, parser.ParseComments) |
|
||||||
if err != nil { |
|
||||||
return nil, err |
|
||||||
} |
|
||||||
return &File{ |
|
||||||
Name: name, |
|
||||||
content: content, |
|
||||||
Pkg: pkg, |
|
||||||
AST: f, |
|
||||||
}, nil |
|
||||||
} |
|
||||||
|
|
||||||
// ToPosition returns line and column for given position.
|
|
||||||
func (f *File) ToPosition(pos token.Pos) token.Position { |
|
||||||
return f.Pkg.fset.Position(pos) |
|
||||||
} |
|
||||||
|
|
||||||
// Render renters a node.
|
|
||||||
func (f *File) Render(x interface{}) string { |
|
||||||
var buf bytes.Buffer |
|
||||||
if err := printer.Fprint(&buf, f.Pkg.fset, x); err != nil { |
|
||||||
panic(err) |
|
||||||
} |
|
||||||
return buf.String() |
|
||||||
} |
|
||||||
|
|
||||||
// CommentMap builds a comment map for the file.
|
|
||||||
func (f *File) CommentMap() ast.CommentMap { |
|
||||||
return ast.NewCommentMap(f.Pkg.fset, f.AST, f.AST.Comments) |
|
||||||
} |
|
||||||
|
|
||||||
var basicTypeKinds = map[types.BasicKind]string{ |
|
||||||
types.UntypedBool: "bool", |
|
||||||
types.UntypedInt: "int", |
|
||||||
types.UntypedRune: "rune", |
|
||||||
types.UntypedFloat: "float64", |
|
||||||
types.UntypedComplex: "complex128", |
|
||||||
types.UntypedString: "string", |
|
||||||
} |
|
||||||
|
|
||||||
// IsUntypedConst reports whether expr is an untyped constant,
|
|
||||||
// and indicates what its default type is.
|
|
||||||
// scope may be nil.
|
|
||||||
func (f *File) IsUntypedConst(expr ast.Expr) (defType string, ok bool) { |
|
||||||
// Re-evaluate expr outside of its context to see if it's untyped.
|
|
||||||
// (An expr evaluated within, for example, an assignment context will get the type of the LHS.)
|
|
||||||
exprStr := f.Render(expr) |
|
||||||
tv, err := types.Eval(f.Pkg.fset, f.Pkg.TypesPkg, expr.Pos(), exprStr) |
|
||||||
if err != nil { |
|
||||||
return "", false |
|
||||||
} |
|
||||||
if b, ok := tv.Type.(*types.Basic); ok { |
|
||||||
if dt, ok := basicTypeKinds[b.Kind()]; ok { |
|
||||||
return dt, true |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return "", false |
|
||||||
} |
|
||||||
|
|
||||||
func (f *File) isMain() bool { |
|
||||||
if f.AST.Name.Name == "main" { |
|
||||||
return true |
|
||||||
} |
|
||||||
return false |
|
||||||
} |
|
||||||
|
|
||||||
const directiveSpecifyDisableReason = "specify-disable-reason" |
|
||||||
|
|
||||||
func (f *File) lint(rules []Rule, config Config, failures chan Failure) { |
|
||||||
rulesConfig := config.Rules |
|
||||||
_, mustSpecifyDisableReason := config.Directives[directiveSpecifyDisableReason] |
|
||||||
disabledIntervals := f.disabledIntervals(rules, mustSpecifyDisableReason, failures) |
|
||||||
for _, currentRule := range rules { |
|
||||||
ruleConfig := rulesConfig[currentRule.Name()] |
|
||||||
currentFailures := currentRule.Apply(f, ruleConfig.Arguments) |
|
||||||
for idx, failure := range currentFailures { |
|
||||||
if failure.RuleName == "" { |
|
||||||
failure.RuleName = currentRule.Name() |
|
||||||
} |
|
||||||
if failure.Node != nil { |
|
||||||
failure.Position = ToFailurePosition(failure.Node.Pos(), failure.Node.End(), f) |
|
||||||
} |
|
||||||
currentFailures[idx] = failure |
|
||||||
} |
|
||||||
currentFailures = f.filterFailures(currentFailures, disabledIntervals) |
|
||||||
for _, failure := range currentFailures { |
|
||||||
if failure.Confidence >= config.Confidence { |
|
||||||
failures <- failure |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
type enableDisableConfig struct { |
|
||||||
enabled bool |
|
||||||
position int |
|
||||||
} |
|
||||||
|
|
||||||
const directiveRE = `^//[\s]*revive:(enable|disable)(?:-(line|next-line))?(?::([^\s]+))?[\s]*(?: (.+))?$` |
|
||||||
const directivePos = 1 |
|
||||||
const modifierPos = 2 |
|
||||||
const rulesPos = 3 |
|
||||||
const reasonPos = 4 |
|
||||||
|
|
||||||
var re = regexp.MustCompile(directiveRE) |
|
||||||
|
|
||||||
func (f *File) disabledIntervals(rules []Rule, mustSpecifyDisableReason bool, failures chan Failure) disabledIntervalsMap { |
|
||||||
enabledDisabledRulesMap := make(map[string][]enableDisableConfig) |
|
||||||
|
|
||||||
getEnabledDisabledIntervals := func() disabledIntervalsMap { |
|
||||||
result := make(disabledIntervalsMap) |
|
||||||
|
|
||||||
for ruleName, disabledArr := range enabledDisabledRulesMap { |
|
||||||
ruleResult := []DisabledInterval{} |
|
||||||
for i := 0; i < len(disabledArr); i++ { |
|
||||||
interval := DisabledInterval{ |
|
||||||
RuleName: ruleName, |
|
||||||
From: token.Position{ |
|
||||||
Filename: f.Name, |
|
||||||
Line: disabledArr[i].position, |
|
||||||
}, |
|
||||||
To: token.Position{ |
|
||||||
Filename: f.Name, |
|
||||||
Line: math.MaxInt32, |
|
||||||
}, |
|
||||||
} |
|
||||||
if i%2 == 0 { |
|
||||||
ruleResult = append(ruleResult, interval) |
|
||||||
} else { |
|
||||||
ruleResult[len(ruleResult)-1].To.Line = disabledArr[i].position |
|
||||||
} |
|
||||||
} |
|
||||||
result[ruleName] = ruleResult |
|
||||||
} |
|
||||||
|
|
||||||
return result |
|
||||||
} |
|
||||||
|
|
||||||
handleConfig := func(isEnabled bool, line int, name string) { |
|
||||||
existing, ok := enabledDisabledRulesMap[name] |
|
||||||
if !ok { |
|
||||||
existing = []enableDisableConfig{} |
|
||||||
enabledDisabledRulesMap[name] = existing |
|
||||||
} |
|
||||||
if (len(existing) > 1 && existing[len(existing)-1].enabled == isEnabled) || |
|
||||||
(len(existing) == 0 && isEnabled) { |
|
||||||
return |
|
||||||
} |
|
||||||
existing = append(existing, enableDisableConfig{ |
|
||||||
enabled: isEnabled, |
|
||||||
position: line, |
|
||||||
}) |
|
||||||
enabledDisabledRulesMap[name] = existing |
|
||||||
} |
|
||||||
|
|
||||||
handleRules := func(filename, modifier string, isEnabled bool, line int, ruleNames []string) []DisabledInterval { |
|
||||||
var result []DisabledInterval |
|
||||||
for _, name := range ruleNames { |
|
||||||
if modifier == "line" { |
|
||||||
handleConfig(isEnabled, line, name) |
|
||||||
handleConfig(!isEnabled, line, name) |
|
||||||
} else if modifier == "next-line" { |
|
||||||
handleConfig(isEnabled, line+1, name) |
|
||||||
handleConfig(!isEnabled, line+1, name) |
|
||||||
} else { |
|
||||||
handleConfig(isEnabled, line, name) |
|
||||||
} |
|
||||||
} |
|
||||||
return result |
|
||||||
} |
|
||||||
|
|
||||||
handleComment := func(filename string, c *ast.CommentGroup, line int) { |
|
||||||
comments := c.List |
|
||||||
for _, c := range comments { |
|
||||||
match := re.FindStringSubmatch(c.Text) |
|
||||||
if len(match) == 0 { |
|
||||||
return |
|
||||||
} |
|
||||||
|
|
||||||
ruleNames := []string{} |
|
||||||
tempNames := strings.Split(match[rulesPos], ",") |
|
||||||
for _, name := range tempNames { |
|
||||||
name = strings.Trim(name, "\n") |
|
||||||
if len(name) > 0 { |
|
||||||
ruleNames = append(ruleNames, name) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
mustCheckDisablingReason := mustSpecifyDisableReason && match[directivePos] == "disable" |
|
||||||
if mustCheckDisablingReason && strings.Trim(match[reasonPos], " ") == "" { |
|
||||||
failures <- Failure{ |
|
||||||
Confidence: 1, |
|
||||||
RuleName: directiveSpecifyDisableReason, |
|
||||||
Failure: "reason of lint disabling not found", |
|
||||||
Position: ToFailurePosition(c.Pos(), c.End(), f), |
|
||||||
Node: c, |
|
||||||
} |
|
||||||
continue // skip this linter disabling directive
|
|
||||||
} |
|
||||||
|
|
||||||
// TODO: optimize
|
|
||||||
if len(ruleNames) == 0 { |
|
||||||
for _, rule := range rules { |
|
||||||
ruleNames = append(ruleNames, rule.Name()) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
handleRules(filename, match[modifierPos], match[directivePos] == "enable", line, ruleNames) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
comments := f.AST.Comments |
|
||||||
for _, c := range comments { |
|
||||||
handleComment(f.Name, c, f.ToPosition(c.End()).Line) |
|
||||||
} |
|
||||||
|
|
||||||
return getEnabledDisabledIntervals() |
|
||||||
} |
|
||||||
|
|
||||||
func (f *File) filterFailures(failures []Failure, disabledIntervals disabledIntervalsMap) []Failure { |
|
||||||
result := []Failure{} |
|
||||||
for _, failure := range failures { |
|
||||||
fStart := failure.Position.Start.Line |
|
||||||
fEnd := failure.Position.End.Line |
|
||||||
intervals, ok := disabledIntervals[failure.RuleName] |
|
||||||
if !ok { |
|
||||||
result = append(result, failure) |
|
||||||
} else { |
|
||||||
include := true |
|
||||||
for _, interval := range intervals { |
|
||||||
intStart := interval.From.Line |
|
||||||
intEnd := interval.To.Line |
|
||||||
if (fStart >= intStart && fStart <= intEnd) || |
|
||||||
(fEnd >= intStart && fEnd <= intEnd) { |
|
||||||
include = false |
|
||||||
break |
|
||||||
} |
|
||||||
} |
|
||||||
if include { |
|
||||||
result = append(result, failure) |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
return result |
|
||||||
} |
|
@ -1,14 +0,0 @@ |
|||||||
package lint |
|
||||||
|
|
||||||
// FormatterMetadata configuration of a formatter
|
|
||||||
type FormatterMetadata struct { |
|
||||||
Name string |
|
||||||
Description string |
|
||||||
Sample string |
|
||||||
} |
|
||||||
|
|
||||||
// Formatter defines an interface for failure formatters
|
|
||||||
type Formatter interface { |
|
||||||
Format(<-chan Failure, Config) (string, error) |
|
||||||
Name() string |
|
||||||
} |
|
@ -1,99 +0,0 @@ |
|||||||
package lint |
|
||||||
|
|
||||||
import ( |
|
||||||
"bufio" |
|
||||||
"bytes" |
|
||||||
"fmt" |
|
||||||
"go/token" |
|
||||||
"os" |
|
||||||
"sync" |
|
||||||
) |
|
||||||
|
|
||||||
// ReadFile defines an abstraction for reading files.
|
|
||||||
type ReadFile func(path string) (result []byte, err error) |
|
||||||
|
|
||||||
type disabledIntervalsMap = map[string][]DisabledInterval |
|
||||||
|
|
||||||
// Linter is used for linting set of files.
|
|
||||||
type Linter struct { |
|
||||||
reader ReadFile |
|
||||||
} |
|
||||||
|
|
||||||
// New creates a new Linter
|
|
||||||
func New(reader ReadFile) Linter { |
|
||||||
return Linter{reader: reader} |
|
||||||
} |
|
||||||
|
|
||||||
var ( |
|
||||||
genHdr = []byte("// Code generated ") |
|
||||||
genFtr = []byte(" DO NOT EDIT.") |
|
||||||
) |
|
||||||
|
|
||||||
// Lint lints a set of files with the specified rule.
|
|
||||||
func (l *Linter) Lint(packages [][]string, ruleSet []Rule, config Config) (<-chan Failure, error) { |
|
||||||
failures := make(chan Failure) |
|
||||||
|
|
||||||
var wg sync.WaitGroup |
|
||||||
for _, pkg := range packages { |
|
||||||
wg.Add(1) |
|
||||||
go func(pkg []string) { |
|
||||||
if err := l.lintPackage(pkg, ruleSet, config, failures); err != nil { |
|
||||||
fmt.Fprintln(os.Stderr, err) |
|
||||||
os.Exit(1) |
|
||||||
} |
|
||||||
defer wg.Done() |
|
||||||
}(pkg) |
|
||||||
} |
|
||||||
|
|
||||||
go func() { |
|
||||||
wg.Wait() |
|
||||||
close(failures) |
|
||||||
}() |
|
||||||
|
|
||||||
return failures, nil |
|
||||||
} |
|
||||||
|
|
||||||
func (l *Linter) lintPackage(filenames []string, ruleSet []Rule, config Config, failures chan Failure) error { |
|
||||||
pkg := &Package{ |
|
||||||
fset: token.NewFileSet(), |
|
||||||
files: map[string]*File{}, |
|
||||||
mu: sync.Mutex{}, |
|
||||||
} |
|
||||||
for _, filename := range filenames { |
|
||||||
content, err := l.reader(filename) |
|
||||||
if err != nil { |
|
||||||
return err |
|
||||||
} |
|
||||||
if isGenerated(content) && !config.IgnoreGeneratedHeader { |
|
||||||
continue |
|
||||||
} |
|
||||||
|
|
||||||
file, err := NewFile(filename, content, pkg) |
|
||||||
if err != nil { |
|
||||||
return err |
|
||||||
} |
|
||||||
pkg.files[filename] = file |
|
||||||
} |
|
||||||
|
|
||||||
if len(pkg.files) == 0 { |
|
||||||
return nil |
|
||||||
} |
|
||||||
|
|
||||||
pkg.lint(ruleSet, config, failures) |
|
||||||
|
|
||||||
return nil |
|
||||||
} |
|
||||||
|
|
||||||
// isGenerated reports whether the source file is generated code
|
|
||||||
// according the rules from https://golang.org/s/generatedcode.
|
|
||||||
// This is inherited from the original go lint.
|
|
||||||
func isGenerated(src []byte) bool { |
|
||||||
sc := bufio.NewScanner(bytes.NewReader(src)) |
|
||||||
for sc.Scan() { |
|
||||||
b := sc.Bytes() |
|
||||||
if bytes.HasPrefix(b, genHdr) && bytes.HasSuffix(b, genFtr) && len(b) >= len(genHdr)+len(genFtr) { |
|
||||||
return true |
|
||||||
} |
|
||||||
} |
|
||||||
return false |
|
||||||
} |
|
@ -1,178 +0,0 @@ |
|||||||
package lint |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
"go/token" |
|
||||||
"go/types" |
|
||||||
"sync" |
|
||||||
|
|
||||||
"golang.org/x/tools/go/gcexportdata" |
|
||||||
) |
|
||||||
|
|
||||||
// Package represents a package in the project.
|
|
||||||
type Package struct { |
|
||||||
fset *token.FileSet |
|
||||||
files map[string]*File |
|
||||||
|
|
||||||
TypesPkg *types.Package |
|
||||||
TypesInfo *types.Info |
|
||||||
|
|
||||||
// sortable is the set of types in the package that implement sort.Interface.
|
|
||||||
Sortable map[string]bool |
|
||||||
// main is whether this is a "main" package.
|
|
||||||
main int |
|
||||||
mu sync.Mutex |
|
||||||
} |
|
||||||
|
|
||||||
var newImporter = func(fset *token.FileSet) types.ImporterFrom { |
|
||||||
return gcexportdata.NewImporter(fset, make(map[string]*types.Package)) |
|
||||||
} |
|
||||||
|
|
||||||
var ( |
|
||||||
trueValue = 1 |
|
||||||
falseValue = 2 |
|
||||||
notSet = 3 |
|
||||||
) |
|
||||||
|
|
||||||
// IsMain returns if that's the main package.
|
|
||||||
func (p *Package) IsMain() bool { |
|
||||||
if p.main == trueValue { |
|
||||||
return true |
|
||||||
} else if p.main == falseValue { |
|
||||||
return false |
|
||||||
} |
|
||||||
for _, f := range p.files { |
|
||||||
if f.isMain() { |
|
||||||
p.main = trueValue |
|
||||||
return true |
|
||||||
} |
|
||||||
} |
|
||||||
p.main = falseValue |
|
||||||
return false |
|
||||||
} |
|
||||||
|
|
||||||
// TypeCheck performs type checking for given package.
|
|
||||||
func (p *Package) TypeCheck() error { |
|
||||||
p.mu.Lock() |
|
||||||
// If type checking has already been performed
|
|
||||||
// skip it.
|
|
||||||
if p.TypesInfo != nil || p.TypesPkg != nil { |
|
||||||
p.mu.Unlock() |
|
||||||
return nil |
|
||||||
} |
|
||||||
config := &types.Config{ |
|
||||||
// By setting a no-op error reporter, the type checker does as much work as possible.
|
|
||||||
Error: func(error) {}, |
|
||||||
Importer: newImporter(p.fset), |
|
||||||
} |
|
||||||
info := &types.Info{ |
|
||||||
Types: make(map[ast.Expr]types.TypeAndValue), |
|
||||||
Defs: make(map[*ast.Ident]types.Object), |
|
||||||
Uses: make(map[*ast.Ident]types.Object), |
|
||||||
Scopes: make(map[ast.Node]*types.Scope), |
|
||||||
} |
|
||||||
var anyFile *File |
|
||||||
var astFiles []*ast.File |
|
||||||
for _, f := range p.files { |
|
||||||
anyFile = f |
|
||||||
astFiles = append(astFiles, f.AST) |
|
||||||
} |
|
||||||
|
|
||||||
typesPkg, err := check(config, anyFile.AST.Name.Name, p.fset, astFiles, info) |
|
||||||
|
|
||||||
// Remember the typechecking info, even if config.Check failed,
|
|
||||||
// since we will get partial information.
|
|
||||||
p.TypesPkg = typesPkg |
|
||||||
p.TypesInfo = info |
|
||||||
p.mu.Unlock() |
|
||||||
return err |
|
||||||
} |
|
||||||
|
|
||||||
// check function encapsulates the call to go/types.Config.Check method and
|
|
||||||
// recovers if the called method panics (see issue #59)
|
|
||||||
func check(config *types.Config, n string, fset *token.FileSet, astFiles []*ast.File, info *types.Info) (p *types.Package, err error) { |
|
||||||
defer func() { |
|
||||||
if r := recover(); r != nil { |
|
||||||
err, _ = r.(error) |
|
||||||
p = nil |
|
||||||
return |
|
||||||
} |
|
||||||
}() |
|
||||||
|
|
||||||
return config.Check(n, fset, astFiles, info) |
|
||||||
} |
|
||||||
|
|
||||||
// TypeOf returns the type of an expression.
|
|
||||||
func (p *Package) TypeOf(expr ast.Expr) types.Type { |
|
||||||
if p.TypesInfo == nil { |
|
||||||
return nil |
|
||||||
} |
|
||||||
return p.TypesInfo.TypeOf(expr) |
|
||||||
} |
|
||||||
|
|
||||||
type walker struct { |
|
||||||
nmap map[string]int |
|
||||||
has map[string]int |
|
||||||
} |
|
||||||
|
|
||||||
func (w *walker) Visit(n ast.Node) ast.Visitor { |
|
||||||
fn, ok := n.(*ast.FuncDecl) |
|
||||||
if !ok || fn.Recv == nil || len(fn.Recv.List) == 0 { |
|
||||||
return w |
|
||||||
} |
|
||||||
// TODO(dsymonds): We could check the signature to be more precise.
|
|
||||||
recv := receiverType(fn) |
|
||||||
if i, ok := w.nmap[fn.Name.Name]; ok { |
|
||||||
w.has[recv] |= i |
|
||||||
} |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
func (p *Package) scanSortable() { |
|
||||||
p.Sortable = make(map[string]bool) |
|
||||||
|
|
||||||
// bitfield for which methods exist on each type.
|
|
||||||
const ( |
|
||||||
Len = 1 << iota |
|
||||||
Less |
|
||||||
Swap |
|
||||||
) |
|
||||||
nmap := map[string]int{"Len": Len, "Less": Less, "Swap": Swap} |
|
||||||
has := make(map[string]int) |
|
||||||
for _, f := range p.files { |
|
||||||
ast.Walk(&walker{nmap, has}, f.AST) |
|
||||||
} |
|
||||||
for typ, ms := range has { |
|
||||||
if ms == Len|Less|Swap { |
|
||||||
p.Sortable[typ] = true |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// receiverType returns the named type of the method receiver, sans "*",
|
|
||||||
// or "invalid-type" if fn.Recv is ill formed.
|
|
||||||
func receiverType(fn *ast.FuncDecl) string { |
|
||||||
switch e := fn.Recv.List[0].Type.(type) { |
|
||||||
case *ast.Ident: |
|
||||||
return e.Name |
|
||||||
case *ast.StarExpr: |
|
||||||
if id, ok := e.X.(*ast.Ident); ok { |
|
||||||
return id.Name |
|
||||||
} |
|
||||||
} |
|
||||||
// The parser accepts much more than just the legal forms.
|
|
||||||
return "invalid-type" |
|
||||||
} |
|
||||||
|
|
||||||
func (p *Package) lint(rules []Rule, config Config, failures chan Failure) { |
|
||||||
p.scanSortable() |
|
||||||
var wg sync.WaitGroup |
|
||||||
for _, file := range p.files { |
|
||||||
wg.Add(1) |
|
||||||
go (func(file *File) { |
|
||||||
file.lint(rules, config, failures) |
|
||||||
defer wg.Done() |
|
||||||
})(file) |
|
||||||
} |
|
||||||
wg.Wait() |
|
||||||
} |
|
@ -1,31 +0,0 @@ |
|||||||
package lint |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/token" |
|
||||||
) |
|
||||||
|
|
||||||
// DisabledInterval contains a single disabled interval and the associated rule name.
|
|
||||||
type DisabledInterval struct { |
|
||||||
From token.Position |
|
||||||
To token.Position |
|
||||||
RuleName string |
|
||||||
} |
|
||||||
|
|
||||||
// Rule defines an abstract rule interaface
|
|
||||||
type Rule interface { |
|
||||||
Name() string |
|
||||||
Apply(*File, Arguments) []Failure |
|
||||||
} |
|
||||||
|
|
||||||
// AbstractRule defines an abstract rule.
|
|
||||||
type AbstractRule struct { |
|
||||||
Failures []Failure |
|
||||||
} |
|
||||||
|
|
||||||
// ToFailurePosition returns the failure position.
|
|
||||||
func ToFailurePosition(start token.Pos, end token.Pos, file *File) FailurePosition { |
|
||||||
return FailurePosition{ |
|
||||||
Start: file.ToPosition(start), |
|
||||||
End: file.ToPosition(end), |
|
||||||
} |
|
||||||
} |
|
@ -1,128 +0,0 @@ |
|||||||
package lint |
|
||||||
|
|
||||||
import ( |
|
||||||
"strings" |
|
||||||
"unicode" |
|
||||||
) |
|
||||||
|
|
||||||
// Name returns a different name if it should be different.
|
|
||||||
func Name(name string, whitelist, blacklist []string) (should string) { |
|
||||||
// Fast path for simple cases: "_" and all lowercase.
|
|
||||||
if name == "_" { |
|
||||||
return name |
|
||||||
} |
|
||||||
allLower := true |
|
||||||
for _, r := range name { |
|
||||||
if !unicode.IsLower(r) { |
|
||||||
allLower = false |
|
||||||
break |
|
||||||
} |
|
||||||
} |
|
||||||
if allLower { |
|
||||||
return name |
|
||||||
} |
|
||||||
|
|
||||||
// Split camelCase at any lower->upper transition, and split on underscores.
|
|
||||||
// Check each word for common initialisms.
|
|
||||||
runes := []rune(name) |
|
||||||
w, i := 0, 0 // index of start of word, scan
|
|
||||||
for i+1 <= len(runes) { |
|
||||||
eow := false // whether we hit the end of a word
|
|
||||||
if i+1 == len(runes) { |
|
||||||
eow = true |
|
||||||
} else if runes[i+1] == '_' { |
|
||||||
// underscore; shift the remainder forward over any run of underscores
|
|
||||||
eow = true |
|
||||||
n := 1 |
|
||||||
for i+n+1 < len(runes) && runes[i+n+1] == '_' { |
|
||||||
n++ |
|
||||||
} |
|
||||||
|
|
||||||
// Leave at most one underscore if the underscore is between two digits
|
|
||||||
if i+n+1 < len(runes) && unicode.IsDigit(runes[i]) && unicode.IsDigit(runes[i+n+1]) { |
|
||||||
n-- |
|
||||||
} |
|
||||||
|
|
||||||
copy(runes[i+1:], runes[i+n+1:]) |
|
||||||
runes = runes[:len(runes)-n] |
|
||||||
} else if unicode.IsLower(runes[i]) && !unicode.IsLower(runes[i+1]) { |
|
||||||
// lower->non-lower
|
|
||||||
eow = true |
|
||||||
} |
|
||||||
i++ |
|
||||||
if !eow { |
|
||||||
continue |
|
||||||
} |
|
||||||
|
|
||||||
// [w,i) is a word.
|
|
||||||
word := string(runes[w:i]) |
|
||||||
ignoreInitWarnings := map[string]bool{} |
|
||||||
for _, i := range whitelist { |
|
||||||
ignoreInitWarnings[i] = true |
|
||||||
} |
|
||||||
|
|
||||||
extraInits := map[string]bool{} |
|
||||||
for _, i := range blacklist { |
|
||||||
extraInits[i] = true |
|
||||||
} |
|
||||||
|
|
||||||
if u := strings.ToUpper(word); (commonInitialisms[u] || extraInits[u]) && !ignoreInitWarnings[u] { |
|
||||||
// Keep consistent case, which is lowercase only at the start.
|
|
||||||
if w == 0 && unicode.IsLower(runes[w]) { |
|
||||||
u = strings.ToLower(u) |
|
||||||
} |
|
||||||
// All the common initialisms are ASCII,
|
|
||||||
// so we can replace the bytes exactly.
|
|
||||||
copy(runes[w:], []rune(u)) |
|
||||||
} else if w > 0 && strings.ToLower(word) == word { |
|
||||||
// already all lowercase, and not the first word, so uppercase the first character.
|
|
||||||
runes[w] = unicode.ToUpper(runes[w]) |
|
||||||
} |
|
||||||
w = i |
|
||||||
} |
|
||||||
return string(runes) |
|
||||||
} |
|
||||||
|
|
||||||
// commonInitialisms is a set of common initialisms.
|
|
||||||
// Only add entries that are highly unlikely to be non-initialisms.
|
|
||||||
// For instance, "ID" is fine (Freudian code is rare), but "AND" is not.
|
|
||||||
var commonInitialisms = map[string]bool{ |
|
||||||
"ACL": true, |
|
||||||
"API": true, |
|
||||||
"ASCII": true, |
|
||||||
"CPU": true, |
|
||||||
"CSS": true, |
|
||||||
"DNS": true, |
|
||||||
"EOF": true, |
|
||||||
"GUID": true, |
|
||||||
"HTML": true, |
|
||||||
"HTTP": true, |
|
||||||
"HTTPS": true, |
|
||||||
"ID": true, |
|
||||||
"IP": true, |
|
||||||
"JSON": true, |
|
||||||
"LHS": true, |
|
||||||
"QPS": true, |
|
||||||
"RAM": true, |
|
||||||
"RHS": true, |
|
||||||
"RPC": true, |
|
||||||
"SLA": true, |
|
||||||
"SMTP": true, |
|
||||||
"SQL": true, |
|
||||||
"SSH": true, |
|
||||||
"TCP": true, |
|
||||||
"TLS": true, |
|
||||||
"TTL": true, |
|
||||||
"UDP": true, |
|
||||||
"UI": true, |
|
||||||
"UID": true, |
|
||||||
"UUID": true, |
|
||||||
"URI": true, |
|
||||||
"URL": true, |
|
||||||
"UTF8": true, |
|
||||||
"VM": true, |
|
||||||
"XML": true, |
|
||||||
"XMPP": true, |
|
||||||
"XSRF": true, |
|
||||||
"XSS": true, |
|
||||||
} |
|
@ -1,151 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
"go/ast" |
|
||||||
"strconv" |
|
||||||
"strings" |
|
||||||
) |
|
||||||
|
|
||||||
const ( |
|
||||||
defaultStrLitLimit = 2 |
|
||||||
kindFLOAT = "FLOAT" |
|
||||||
kindINT = "INT" |
|
||||||
kindSTRING = "STRING" |
|
||||||
) |
|
||||||
|
|
||||||
type whiteList map[string]map[string]bool |
|
||||||
|
|
||||||
func newWhiteList() whiteList { |
|
||||||
return map[string]map[string]bool{kindINT: map[string]bool{}, kindFLOAT: map[string]bool{}, kindSTRING: map[string]bool{}} |
|
||||||
} |
|
||||||
|
|
||||||
func (wl whiteList) add(kind string, list string) { |
|
||||||
elems := strings.Split(list, ",") |
|
||||||
for _, e := range elems { |
|
||||||
wl[kind][e] = true |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// AddConstantRule lints unused params in functions.
|
|
||||||
type AddConstantRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *AddConstantRule) Apply(file *lint.File, arguments lint.Arguments) []lint.Failure { |
|
||||||
strLitLimit := defaultStrLitLimit |
|
||||||
var whiteList = newWhiteList() |
|
||||||
if len(arguments) > 0 { |
|
||||||
args, ok := arguments[0].(map[string]interface{}) |
|
||||||
if !ok { |
|
||||||
panic(fmt.Sprintf("Invalid argument to the add-constant rule. Expecting a k,v map, got %T", arguments[0])) |
|
||||||
} |
|
||||||
for k, v := range args { |
|
||||||
kind := "" |
|
||||||
switch k { |
|
||||||
case "allowFloats": |
|
||||||
kind = kindFLOAT |
|
||||||
fallthrough |
|
||||||
case "allowInts": |
|
||||||
if kind == "" { |
|
||||||
kind = kindINT |
|
||||||
} |
|
||||||
fallthrough |
|
||||||
case "allowStrs": |
|
||||||
if kind == "" { |
|
||||||
kind = kindSTRING |
|
||||||
} |
|
||||||
list, ok := v.(string) |
|
||||||
if !ok { |
|
||||||
panic(fmt.Sprintf("Invalid argument to the add-constant rule, string expected. Got '%v' (%T)", v, v)) |
|
||||||
} |
|
||||||
whiteList.add(kind, list) |
|
||||||
case "maxLitCount": |
|
||||||
sl, ok := v.(string) |
|
||||||
if !ok { |
|
||||||
panic(fmt.Sprintf("Invalid argument to the add-constant rule, expecting string representation of an integer. Got '%v' (%T)", v, v)) |
|
||||||
} |
|
||||||
|
|
||||||
limit, err := strconv.Atoi(sl) |
|
||||||
if err != nil { |
|
||||||
panic(fmt.Sprintf("Invalid argument to the add-constant rule, expecting string representation of an integer. Got '%v'", v)) |
|
||||||
} |
|
||||||
strLitLimit = limit |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
onFailure := func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
} |
|
||||||
|
|
||||||
w := lintAddConstantRule{onFailure: onFailure, strLits: make(map[string]int, 0), strLitLimit: strLitLimit, whiteLst: whiteList} |
|
||||||
|
|
||||||
ast.Walk(w, file.AST) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *AddConstantRule) Name() string { |
|
||||||
return "add-constant" |
|
||||||
} |
|
||||||
|
|
||||||
type lintAddConstantRule struct { |
|
||||||
onFailure func(lint.Failure) |
|
||||||
strLits map[string]int |
|
||||||
strLitLimit int |
|
||||||
whiteLst whiteList |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintAddConstantRule) Visit(node ast.Node) ast.Visitor { |
|
||||||
switch n := node.(type) { |
|
||||||
case *ast.GenDecl: |
|
||||||
return nil // skip declarations
|
|
||||||
case *ast.BasicLit: |
|
||||||
switch kind := n.Kind.String(); kind { |
|
||||||
case kindFLOAT, kindINT: |
|
||||||
w.checkNumLit(kind, n) |
|
||||||
case kindSTRING: |
|
||||||
w.checkStrLit(n) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return w |
|
||||||
|
|
||||||
} |
|
||||||
|
|
||||||
func (w lintAddConstantRule) checkStrLit(n *ast.BasicLit) { |
|
||||||
if w.whiteLst[kindSTRING][n.Value] { |
|
||||||
return |
|
||||||
} |
|
||||||
|
|
||||||
count := w.strLits[n.Value] |
|
||||||
if count >= 0 { |
|
||||||
w.strLits[n.Value] = count + 1 |
|
||||||
if w.strLits[n.Value] > w.strLitLimit { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Node: n, |
|
||||||
Category: "style", |
|
||||||
Failure: fmt.Sprintf("string literal %s appears, at least, %d times, create a named constant for it", n.Value, w.strLits[n.Value]), |
|
||||||
}) |
|
||||||
w.strLits[n.Value] = -1 // mark it to avoid failing again on the same literal
|
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintAddConstantRule) checkNumLit(kind string, n *ast.BasicLit) { |
|
||||||
if w.whiteLst[kind][n.Value] { |
|
||||||
return |
|
||||||
} |
|
||||||
|
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Node: n, |
|
||||||
Category: "style", |
|
||||||
Failure: fmt.Sprintf("avoid magic numbers like '%s', create a named constant for it", n.Value), |
|
||||||
}) |
|
||||||
} |
|
@ -1,67 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"go/ast" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// ArgumentsLimitRule lints given else constructs.
|
|
||||||
type ArgumentsLimitRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *ArgumentsLimitRule) Apply(file *lint.File, arguments lint.Arguments) []lint.Failure { |
|
||||||
if len(arguments) != 1 { |
|
||||||
panic(`invalid configuration for "argument-limit"`) |
|
||||||
} |
|
||||||
|
|
||||||
total, ok := arguments[0].(int64) // Alt. non panicking version
|
|
||||||
if !ok { |
|
||||||
panic(`invalid value passed as argument number to the "argument-list" rule`) |
|
||||||
} |
|
||||||
|
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
walker := lintArgsNum{ |
|
||||||
total: int(total), |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
ast.Walk(walker, file.AST) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *ArgumentsLimitRule) Name() string { |
|
||||||
return "argument-limit" |
|
||||||
} |
|
||||||
|
|
||||||
type lintArgsNum struct { |
|
||||||
total int |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintArgsNum) Visit(n ast.Node) ast.Visitor { |
|
||||||
node, ok := n.(*ast.FuncDecl) |
|
||||||
if ok { |
|
||||||
num := 0 |
|
||||||
for _, l := range node.Type.Params.List { |
|
||||||
for range l.Names { |
|
||||||
num++ |
|
||||||
} |
|
||||||
} |
|
||||||
if num > w.total { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Failure: fmt.Sprintf("maximum number of arguments per function exceeded; max %d but got %d", w.total, num), |
|
||||||
Node: node.Type, |
|
||||||
}) |
|
||||||
return w |
|
||||||
} |
|
||||||
} |
|
||||||
return w |
|
||||||
} |
|
@ -1,94 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
"go/token" |
|
||||||
"go/types" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// AtomicRule lints given else constructs.
|
|
||||||
type AtomicRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *AtomicRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
walker := atomic{ |
|
||||||
pkgTypesInfo: file.Pkg.TypesInfo, |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
ast.Walk(walker, file.AST) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *AtomicRule) Name() string { |
|
||||||
return "atomic" |
|
||||||
} |
|
||||||
|
|
||||||
type atomic struct { |
|
||||||
pkgTypesInfo *types.Info |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w atomic) Visit(node ast.Node) ast.Visitor { |
|
||||||
n, ok := node.(*ast.AssignStmt) |
|
||||||
if !ok { |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
if len(n.Lhs) != len(n.Rhs) { |
|
||||||
return nil // skip assignment sub-tree
|
|
||||||
} |
|
||||||
if len(n.Lhs) == 1 && n.Tok == token.DEFINE { |
|
||||||
return nil // skip assignment sub-tree
|
|
||||||
} |
|
||||||
|
|
||||||
for i, right := range n.Rhs { |
|
||||||
call, ok := right.(*ast.CallExpr) |
|
||||||
if !ok { |
|
||||||
continue |
|
||||||
} |
|
||||||
sel, ok := call.Fun.(*ast.SelectorExpr) |
|
||||||
if !ok { |
|
||||||
continue |
|
||||||
} |
|
||||||
pkgIdent, _ := sel.X.(*ast.Ident) |
|
||||||
if w.pkgTypesInfo != nil { |
|
||||||
pkgName, ok := w.pkgTypesInfo.Uses[pkgIdent].(*types.PkgName) |
|
||||||
if !ok || pkgName.Imported().Path() != "sync/atomic" { |
|
||||||
continue |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
switch sel.Sel.Name { |
|
||||||
case "AddInt32", "AddInt64", "AddUint32", "AddUint64", "AddUintptr": |
|
||||||
left := n.Lhs[i] |
|
||||||
if len(call.Args) != 2 { |
|
||||||
continue |
|
||||||
} |
|
||||||
arg := call.Args[0] |
|
||||||
broken := false |
|
||||||
|
|
||||||
if uarg, ok := arg.(*ast.UnaryExpr); ok && uarg.Op == token.AND { |
|
||||||
broken = gofmt(left) == gofmt(uarg.X) |
|
||||||
} else if star, ok := left.(*ast.StarExpr); ok { |
|
||||||
broken = gofmt(star.X) == gofmt(arg) |
|
||||||
} |
|
||||||
|
|
||||||
if broken { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Failure: "direct assignment to atomic value", |
|
||||||
Node: n, |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
return w |
|
||||||
} |
|
@ -1,84 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// BareReturnRule lints given else constructs.
|
|
||||||
type BareReturnRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *BareReturnRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
onFailure := func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
} |
|
||||||
|
|
||||||
w := lintBareReturnRule{onFailure: onFailure} |
|
||||||
ast.Walk(w, file.AST) |
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *BareReturnRule) Name() string { |
|
||||||
return "bare-return" |
|
||||||
} |
|
||||||
|
|
||||||
type lintBareReturnRule struct { |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintBareReturnRule) Visit(node ast.Node) ast.Visitor { |
|
||||||
switch n := node.(type) { |
|
||||||
case *ast.FuncDecl: |
|
||||||
w.checkFunc(n.Type.Results, n.Body) |
|
||||||
case *ast.FuncLit: // to cope with deferred functions and go-routines
|
|
||||||
w.checkFunc(n.Type.Results, n.Body) |
|
||||||
} |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
// checkFunc will verify if the given function has named result and bare returns
|
|
||||||
func (w lintBareReturnRule) checkFunc(results *ast.FieldList, body *ast.BlockStmt) { |
|
||||||
hasNamedResults := results != nil && len(results.List) > 0 && results.List[0].Names != nil |
|
||||||
if !hasNamedResults || body == nil { |
|
||||||
return // nothing to do
|
|
||||||
} |
|
||||||
|
|
||||||
brf := bareReturnFinder{w.onFailure} |
|
||||||
ast.Walk(brf, body) |
|
||||||
} |
|
||||||
|
|
||||||
type bareReturnFinder struct { |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w bareReturnFinder) Visit(node ast.Node) ast.Visitor { |
|
||||||
_, ok := node.(*ast.FuncLit) |
|
||||||
if ok { |
|
||||||
// skip analysing function literals
|
|
||||||
// they will analyzed by the lintBareReturnRule.Visit method
|
|
||||||
return nil |
|
||||||
} |
|
||||||
|
|
||||||
rs, ok := node.(*ast.ReturnStmt) |
|
||||||
if !ok { |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
if len(rs.Results) > 0 { |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Node: rs, |
|
||||||
Failure: "avoid using bare returns, please add return expressions", |
|
||||||
}) |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
@ -1,75 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
"strings" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// BlankImportsRule lints given else constructs.
|
|
||||||
type BlankImportsRule struct{} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *BlankImportsRule) Name() string { |
|
||||||
return "blank-imports" |
|
||||||
} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *BlankImportsRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
if file.Pkg.IsMain() || file.IsTest() { |
|
||||||
return nil |
|
||||||
} |
|
||||||
|
|
||||||
const ( |
|
||||||
message = "a blank import should be only in a main or test package, or have a comment justifying it" |
|
||||||
category = "imports" |
|
||||||
|
|
||||||
embedImportPath = `"embed"` |
|
||||||
) |
|
||||||
|
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
// The first element of each contiguous group of blank imports should have
|
|
||||||
// an explanatory comment of some kind.
|
|
||||||
for i, imp := range file.AST.Imports { |
|
||||||
pos := file.ToPosition(imp.Pos()) |
|
||||||
|
|
||||||
if !isBlank(imp.Name) { |
|
||||||
continue // Ignore non-blank imports.
|
|
||||||
} |
|
||||||
|
|
||||||
if i > 0 { |
|
||||||
prev := file.AST.Imports[i-1] |
|
||||||
prevPos := file.ToPosition(prev.Pos()) |
|
||||||
|
|
||||||
isSubsequentBlancInAGroup := isBlank(prev.Name) && prevPos.Line+1 == pos.Line && prev.Path.Value != embedImportPath |
|
||||||
if isSubsequentBlancInAGroup { |
|
||||||
continue |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
if imp.Path.Value == embedImportPath && r.fileHasValidEmbedComment(file.AST) { |
|
||||||
continue |
|
||||||
} |
|
||||||
|
|
||||||
// This is the first blank import of a group.
|
|
||||||
if imp.Doc == nil && imp.Comment == nil { |
|
||||||
failures = append(failures, lint.Failure{Failure: message, Category: category, Node: imp, Confidence: 1}) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
func (r *BlankImportsRule) fileHasValidEmbedComment(fileAst *ast.File) bool { |
|
||||||
for _, commentGroup := range fileAst.Comments { |
|
||||||
for _, comment := range commentGroup.List { |
|
||||||
if strings.HasPrefix(comment.Text, "//go:embed ") { |
|
||||||
return true |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return false |
|
||||||
} |
|
@ -1,73 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
"go/token" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// BoolLiteralRule warns when logic expressions contains Boolean literals.
|
|
||||||
type BoolLiteralRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *BoolLiteralRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
onFailure := func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
} |
|
||||||
|
|
||||||
astFile := file.AST |
|
||||||
w := &lintBoolLiteral{astFile, onFailure} |
|
||||||
ast.Walk(w, astFile) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *BoolLiteralRule) Name() string { |
|
||||||
return "bool-literal-in-expr" |
|
||||||
} |
|
||||||
|
|
||||||
type lintBoolLiteral struct { |
|
||||||
file *ast.File |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w *lintBoolLiteral) Visit(node ast.Node) ast.Visitor { |
|
||||||
switch n := node.(type) { |
|
||||||
case *ast.BinaryExpr: |
|
||||||
if !isBoolOp(n.Op) { |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
lexeme, ok := isExprABooleanLit(n.X) |
|
||||||
if !ok { |
|
||||||
lexeme, ok = isExprABooleanLit(n.Y) |
|
||||||
|
|
||||||
if !ok { |
|
||||||
return w |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
isConstant := (n.Op == token.LAND && lexeme == "false") || (n.Op == token.LOR && lexeme == "true") |
|
||||||
|
|
||||||
if isConstant { |
|
||||||
w.addFailure(n, "Boolean expression seems to always evaluate to "+lexeme, "logic") |
|
||||||
} else { |
|
||||||
w.addFailure(n, "omit Boolean literal in expression", "style") |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintBoolLiteral) addFailure(node ast.Node, msg string, cat string) { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Node: node, |
|
||||||
Category: cat, |
|
||||||
Failure: msg, |
|
||||||
}) |
|
||||||
} |
|
@ -1,70 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// CallToGCRule lints calls to the garbage collector.
|
|
||||||
type CallToGCRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *CallToGCRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
onFailure := func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
} |
|
||||||
|
|
||||||
var gcTriggeringFunctions = map[string]map[string]bool{ |
|
||||||
"runtime": map[string]bool{"GC": true}, |
|
||||||
} |
|
||||||
|
|
||||||
w := lintCallToGC{onFailure, gcTriggeringFunctions} |
|
||||||
ast.Walk(w, file.AST) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *CallToGCRule) Name() string { |
|
||||||
return "call-to-gc" |
|
||||||
} |
|
||||||
|
|
||||||
type lintCallToGC struct { |
|
||||||
onFailure func(lint.Failure) |
|
||||||
gcTriggeringFunctions map[string]map[string]bool |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintCallToGC) Visit(node ast.Node) ast.Visitor { |
|
||||||
ce, ok := node.(*ast.CallExpr) |
|
||||||
if !ok { |
|
||||||
return w // nothing to do, the node is not a call
|
|
||||||
} |
|
||||||
|
|
||||||
fc, ok := ce.Fun.(*ast.SelectorExpr) |
|
||||||
if !ok { |
|
||||||
return nil // nothing to do, the call is not of the form pkg.func(...)
|
|
||||||
} |
|
||||||
|
|
||||||
id, ok := fc.X.(*ast.Ident) |
|
||||||
|
|
||||||
if !ok { |
|
||||||
return nil // in case X is not an id (it should be!)
|
|
||||||
} |
|
||||||
|
|
||||||
fn := fc.Sel.Name |
|
||||||
pkg := id.Name |
|
||||||
if !w.gcTriggeringFunctions[pkg][fn] { |
|
||||||
return nil // it isn't a call to a GC triggering function
|
|
||||||
} |
|
||||||
|
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Node: node, |
|
||||||
Category: "bad practice", |
|
||||||
Failure: "explicit call to the garbage collector", |
|
||||||
}) |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
@ -1,195 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"go/ast" |
|
||||||
"go/token" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
"golang.org/x/tools/go/ast/astutil" |
|
||||||
) |
|
||||||
|
|
||||||
// CognitiveComplexityRule lints given else constructs.
|
|
||||||
type CognitiveComplexityRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *CognitiveComplexityRule) Apply(file *lint.File, arguments lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
const expectedArgumentsCount = 1 |
|
||||||
if len(arguments) < expectedArgumentsCount { |
|
||||||
panic(fmt.Sprintf("not enough arguments for cognitive-complexity, expected %d, got %d", expectedArgumentsCount, len(arguments))) |
|
||||||
} |
|
||||||
complexity, ok := arguments[0].(int64) |
|
||||||
if !ok { |
|
||||||
panic(fmt.Sprintf("invalid argument type for cognitive-complexity, expected int64, got %T", arguments[0])) |
|
||||||
} |
|
||||||
|
|
||||||
linter := cognitiveComplexityLinter{ |
|
||||||
file: file, |
|
||||||
maxComplexity: int(complexity), |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
linter.lint() |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *CognitiveComplexityRule) Name() string { |
|
||||||
return "cognitive-complexity" |
|
||||||
} |
|
||||||
|
|
||||||
type cognitiveComplexityLinter struct { |
|
||||||
file *lint.File |
|
||||||
maxComplexity int |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w cognitiveComplexityLinter) lint() { |
|
||||||
f := w.file |
|
||||||
for _, decl := range f.AST.Decls { |
|
||||||
if fn, ok := decl.(*ast.FuncDecl); ok && fn.Body != nil { |
|
||||||
v := cognitiveComplexityVisitor{} |
|
||||||
c := v.subTreeComplexity(fn.Body) |
|
||||||
if c > w.maxComplexity { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Category: "maintenance", |
|
||||||
Failure: fmt.Sprintf("function %s has cognitive complexity %d (> max enabled %d)", funcName(fn), c, w.maxComplexity), |
|
||||||
Node: fn, |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
type cognitiveComplexityVisitor struct { |
|
||||||
complexity int |
|
||||||
nestingLevel int |
|
||||||
} |
|
||||||
|
|
||||||
// subTreeComplexity calculates the cognitive complexity of an AST-subtree.
|
|
||||||
func (v cognitiveComplexityVisitor) subTreeComplexity(n ast.Node) int { |
|
||||||
ast.Walk(&v, n) |
|
||||||
return v.complexity |
|
||||||
} |
|
||||||
|
|
||||||
// Visit implements the ast.Visitor interface.
|
|
||||||
func (v *cognitiveComplexityVisitor) Visit(n ast.Node) ast.Visitor { |
|
||||||
switch n := n.(type) { |
|
||||||
case *ast.IfStmt: |
|
||||||
targets := []ast.Node{n.Cond, n.Body, n.Else} |
|
||||||
v.walk(1, targets...) |
|
||||||
return nil |
|
||||||
case *ast.ForStmt: |
|
||||||
targets := []ast.Node{n.Cond, n.Body} |
|
||||||
v.walk(1, targets...) |
|
||||||
return nil |
|
||||||
case *ast.RangeStmt: |
|
||||||
v.walk(1, n.Body) |
|
||||||
return nil |
|
||||||
case *ast.SelectStmt: |
|
||||||
v.walk(1, n.Body) |
|
||||||
return nil |
|
||||||
case *ast.SwitchStmt: |
|
||||||
v.walk(1, n.Body) |
|
||||||
return nil |
|
||||||
case *ast.TypeSwitchStmt: |
|
||||||
v.walk(1, n.Body) |
|
||||||
return nil |
|
||||||
case *ast.FuncLit: |
|
||||||
v.walk(0, n.Body) // do not increment the complexity, just do the nesting
|
|
||||||
return nil |
|
||||||
case *ast.BinaryExpr: |
|
||||||
v.complexity += v.binExpComplexity(n) |
|
||||||
return nil // skip visiting binexp sub-tree (already visited by binExpComplexity)
|
|
||||||
case *ast.BranchStmt: |
|
||||||
if n.Label != nil { |
|
||||||
v.complexity++ |
|
||||||
} |
|
||||||
} |
|
||||||
// TODO handle (at least) direct recursion
|
|
||||||
|
|
||||||
return v |
|
||||||
} |
|
||||||
|
|
||||||
func (v *cognitiveComplexityVisitor) walk(complexityIncrement int, targets ...ast.Node) { |
|
||||||
v.complexity += complexityIncrement + v.nestingLevel |
|
||||||
nesting := v.nestingLevel |
|
||||||
v.nestingLevel++ |
|
||||||
|
|
||||||
for _, t := range targets { |
|
||||||
if t == nil { |
|
||||||
continue |
|
||||||
} |
|
||||||
|
|
||||||
ast.Walk(v, t) |
|
||||||
} |
|
||||||
|
|
||||||
v.nestingLevel = nesting |
|
||||||
} |
|
||||||
|
|
||||||
func (cognitiveComplexityVisitor) binExpComplexity(n *ast.BinaryExpr) int { |
|
||||||
calculator := binExprComplexityCalculator{opsStack: []token.Token{}} |
|
||||||
|
|
||||||
astutil.Apply(n, calculator.pre, calculator.post) |
|
||||||
|
|
||||||
return calculator.complexity |
|
||||||
} |
|
||||||
|
|
||||||
type binExprComplexityCalculator struct { |
|
||||||
complexity int |
|
||||||
opsStack []token.Token // stack of bool operators
|
|
||||||
subexpStarted bool |
|
||||||
} |
|
||||||
|
|
||||||
func (becc *binExprComplexityCalculator) pre(c *astutil.Cursor) bool { |
|
||||||
switch n := c.Node().(type) { |
|
||||||
case *ast.BinaryExpr: |
|
||||||
isBoolOp := n.Op == token.LAND || n.Op == token.LOR |
|
||||||
if !isBoolOp { |
|
||||||
break |
|
||||||
} |
|
||||||
|
|
||||||
ops := len(becc.opsStack) |
|
||||||
// if
|
|
||||||
// is the first boolop in the expression OR
|
|
||||||
// is the first boolop inside a subexpression (...) OR
|
|
||||||
// is not the same to the previous one
|
|
||||||
// then
|
|
||||||
// increment complexity
|
|
||||||
if ops == 0 || becc.subexpStarted || n.Op != becc.opsStack[ops-1] { |
|
||||||
becc.complexity++ |
|
||||||
becc.subexpStarted = false |
|
||||||
} |
|
||||||
|
|
||||||
becc.opsStack = append(becc.opsStack, n.Op) |
|
||||||
case *ast.ParenExpr: |
|
||||||
becc.subexpStarted = true |
|
||||||
} |
|
||||||
|
|
||||||
return true |
|
||||||
} |
|
||||||
|
|
||||||
func (becc *binExprComplexityCalculator) post(c *astutil.Cursor) bool { |
|
||||||
switch n := c.Node().(type) { |
|
||||||
case *ast.BinaryExpr: |
|
||||||
isBoolOp := n.Op == token.LAND || n.Op == token.LOR |
|
||||||
if !isBoolOp { |
|
||||||
break |
|
||||||
} |
|
||||||
|
|
||||||
ops := len(becc.opsStack) |
|
||||||
if ops > 0 { |
|
||||||
becc.opsStack = becc.opsStack[:ops-1] |
|
||||||
} |
|
||||||
case *ast.ParenExpr: |
|
||||||
becc.subexpStarted = false |
|
||||||
} |
|
||||||
|
|
||||||
return true |
|
||||||
} |
|
@ -1,190 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"go/ast" |
|
||||||
|
|
||||||
"strings" |
|
||||||
"sync" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
type referenceMethod struct { |
|
||||||
fileName string |
|
||||||
id *ast.Ident |
|
||||||
} |
|
||||||
|
|
||||||
type pkgMethods struct { |
|
||||||
pkg *lint.Package |
|
||||||
methods map[string]map[string]*referenceMethod |
|
||||||
mu *sync.Mutex |
|
||||||
} |
|
||||||
|
|
||||||
type packages struct { |
|
||||||
pkgs []pkgMethods |
|
||||||
mu sync.Mutex |
|
||||||
} |
|
||||||
|
|
||||||
func (ps *packages) methodNames(lp *lint.Package) pkgMethods { |
|
||||||
ps.mu.Lock() |
|
||||||
|
|
||||||
for _, pkg := range ps.pkgs { |
|
||||||
if pkg.pkg == lp { |
|
||||||
ps.mu.Unlock() |
|
||||||
return pkg |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
pkgm := pkgMethods{pkg: lp, methods: make(map[string]map[string]*referenceMethod), mu: &sync.Mutex{}} |
|
||||||
ps.pkgs = append(ps.pkgs, pkgm) |
|
||||||
|
|
||||||
ps.mu.Unlock() |
|
||||||
return pkgm |
|
||||||
} |
|
||||||
|
|
||||||
var allPkgs = packages{pkgs: make([]pkgMethods, 1)} |
|
||||||
|
|
||||||
// ConfusingNamingRule lints method names that differ only by capitalization
|
|
||||||
type ConfusingNamingRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *ConfusingNamingRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
fileAst := file.AST |
|
||||||
pkgm := allPkgs.methodNames(file.Pkg) |
|
||||||
walker := lintConfusingNames{ |
|
||||||
fileName: file.Name, |
|
||||||
pkgm: pkgm, |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
ast.Walk(&walker, fileAst) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *ConfusingNamingRule) Name() string { |
|
||||||
return "confusing-naming" |
|
||||||
} |
|
||||||
|
|
||||||
//checkMethodName checks if a given method/function name is similar (just case differences) to other method/function of the same struct/file.
|
|
||||||
func checkMethodName(holder string, id *ast.Ident, w *lintConfusingNames) { |
|
||||||
if id.Name == "init" && holder == defaultStructName { |
|
||||||
// ignore init functions
|
|
||||||
return |
|
||||||
} |
|
||||||
|
|
||||||
pkgm := w.pkgm |
|
||||||
name := strings.ToUpper(id.Name) |
|
||||||
|
|
||||||
pkgm.mu.Lock() |
|
||||||
defer pkgm.mu.Unlock() |
|
||||||
|
|
||||||
if pkgm.methods[holder] != nil { |
|
||||||
if pkgm.methods[holder][name] != nil { |
|
||||||
refMethod := pkgm.methods[holder][name] |
|
||||||
// confusing names
|
|
||||||
var kind string |
|
||||||
if holder == defaultStructName { |
|
||||||
kind = "function" |
|
||||||
} else { |
|
||||||
kind = "method" |
|
||||||
} |
|
||||||
var fileName string |
|
||||||
if w.fileName == refMethod.fileName { |
|
||||||
fileName = "the same source file" |
|
||||||
} else { |
|
||||||
fileName = refMethod.fileName |
|
||||||
} |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Failure: fmt.Sprintf("Method '%s' differs only by capitalization to %s '%s' in %s", id.Name, kind, refMethod.id.Name, fileName), |
|
||||||
Confidence: 1, |
|
||||||
Node: id, |
|
||||||
Category: "naming", |
|
||||||
}) |
|
||||||
|
|
||||||
return |
|
||||||
} |
|
||||||
} else { |
|
||||||
pkgm.methods[holder] = make(map[string]*referenceMethod, 1) |
|
||||||
} |
|
||||||
|
|
||||||
// update the black list
|
|
||||||
if pkgm.methods[holder] == nil { |
|
||||||
println("no entry for '", holder, "'") |
|
||||||
} |
|
||||||
pkgm.methods[holder][name] = &referenceMethod{fileName: w.fileName, id: id} |
|
||||||
} |
|
||||||
|
|
||||||
type lintConfusingNames struct { |
|
||||||
fileName string |
|
||||||
pkgm pkgMethods |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
const defaultStructName = "_" // used to map functions
|
|
||||||
|
|
||||||
//getStructName of a function receiver. Defaults to defaultStructName
|
|
||||||
func getStructName(r *ast.FieldList) string { |
|
||||||
result := defaultStructName |
|
||||||
|
|
||||||
if r == nil || len(r.List) < 1 { |
|
||||||
return result |
|
||||||
} |
|
||||||
|
|
||||||
t := r.List[0].Type |
|
||||||
|
|
||||||
if p, _ := t.(*ast.StarExpr); p != nil { // if a pointer receiver => dereference pointer receiver types
|
|
||||||
t = p.X |
|
||||||
} |
|
||||||
|
|
||||||
if p, _ := t.(*ast.Ident); p != nil { |
|
||||||
result = p.Name |
|
||||||
} |
|
||||||
|
|
||||||
return result |
|
||||||
} |
|
||||||
|
|
||||||
func checkStructFields(fields *ast.FieldList, structName string, w *lintConfusingNames) { |
|
||||||
bl := make(map[string]bool, len(fields.List)) |
|
||||||
for _, f := range fields.List { |
|
||||||
for _, id := range f.Names { |
|
||||||
normName := strings.ToUpper(id.Name) |
|
||||||
if bl[normName] { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Failure: fmt.Sprintf("Field '%s' differs only by capitalization to other field in the struct type %s", id.Name, structName), |
|
||||||
Confidence: 1, |
|
||||||
Node: id, |
|
||||||
Category: "naming", |
|
||||||
}) |
|
||||||
} else { |
|
||||||
bl[normName] = true |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
func (w *lintConfusingNames) Visit(n ast.Node) ast.Visitor { |
|
||||||
switch v := n.(type) { |
|
||||||
case *ast.FuncDecl: |
|
||||||
// Exclude naming warnings for functions that are exported to C but
|
|
||||||
// not exported in the Go API.
|
|
||||||
// See https://github.com/golang/lint/issues/144.
|
|
||||||
if ast.IsExported(v.Name.Name) || !isCgoExported(v) { |
|
||||||
checkMethodName(getStructName(v.Recv), v.Name, w) |
|
||||||
} |
|
||||||
case *ast.TypeSpec: |
|
||||||
if s, ok := v.Type.(*ast.StructType); ok { |
|
||||||
checkStructFields(s.Fields, v.Name.Name, w) |
|
||||||
} |
|
||||||
|
|
||||||
default: |
|
||||||
// will add other checks like field names, struct names, etc.
|
|
||||||
} |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
@ -1,67 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// ConfusingResultsRule lints given function declarations
|
|
||||||
type ConfusingResultsRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *ConfusingResultsRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
fileAst := file.AST |
|
||||||
walker := lintConfusingResults{ |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
ast.Walk(walker, fileAst) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *ConfusingResultsRule) Name() string { |
|
||||||
return "confusing-results" |
|
||||||
} |
|
||||||
|
|
||||||
type lintConfusingResults struct { |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintConfusingResults) Visit(n ast.Node) ast.Visitor { |
|
||||||
fn, ok := n.(*ast.FuncDecl) |
|
||||||
if !ok || fn.Type.Results == nil || len(fn.Type.Results.List) < 2 { |
|
||||||
return w |
|
||||||
} |
|
||||||
lastType := "" |
|
||||||
for _, result := range fn.Type.Results.List { |
|
||||||
if len(result.Names) > 0 { |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
t, ok := result.Type.(*ast.Ident) |
|
||||||
if !ok { |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
if t.Name == lastType { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Node: n, |
|
||||||
Confidence: 1, |
|
||||||
Category: "naming", |
|
||||||
Failure: "unnamed results of the same type may be confusing, consider using named results", |
|
||||||
}) |
|
||||||
break |
|
||||||
} |
|
||||||
lastType = t.Name |
|
||||||
|
|
||||||
} |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
@ -1,88 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
"go/ast" |
|
||||||
"go/token" |
|
||||||
) |
|
||||||
|
|
||||||
// ConstantLogicalExprRule warns on constant logical expressions.
|
|
||||||
type ConstantLogicalExprRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *ConstantLogicalExprRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
onFailure := func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
} |
|
||||||
|
|
||||||
astFile := file.AST |
|
||||||
w := &lintConstantLogicalExpr{astFile, onFailure} |
|
||||||
ast.Walk(w, astFile) |
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *ConstantLogicalExprRule) Name() string { |
|
||||||
return "constant-logical-expr" |
|
||||||
} |
|
||||||
|
|
||||||
type lintConstantLogicalExpr struct { |
|
||||||
file *ast.File |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w *lintConstantLogicalExpr) Visit(node ast.Node) ast.Visitor { |
|
||||||
switch n := node.(type) { |
|
||||||
case *ast.BinaryExpr: |
|
||||||
if !w.isOperatorWithLogicalResult(n.Op) { |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
if gofmt(n.X) != gofmt(n.Y) { // check if subexpressions are the same
|
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
if n.Op == token.EQL { |
|
||||||
w.newFailure(n, "expression always evaluates to true") |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
if w.isInequalityOperator(n.Op) { |
|
||||||
w.newFailure(n, "expression always evaluates to false") |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
w.newFailure(n, "left and right hand-side sub-expressions are the same") |
|
||||||
} |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
func (w *lintConstantLogicalExpr) isOperatorWithLogicalResult(t token.Token) bool { |
|
||||||
switch t { |
|
||||||
case token.LAND, token.LOR, token.EQL, token.LSS, token.GTR, token.NEQ, token.LEQ, token.GEQ: |
|
||||||
return true |
|
||||||
} |
|
||||||
|
|
||||||
return false |
|
||||||
} |
|
||||||
|
|
||||||
func (w *lintConstantLogicalExpr) isInequalityOperator(t token.Token) bool { |
|
||||||
switch t { |
|
||||||
case token.LSS, token.GTR, token.NEQ, token.LEQ, token.GEQ: |
|
||||||
return true |
|
||||||
} |
|
||||||
|
|
||||||
return false |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintConstantLogicalExpr) newFailure(node ast.Node, msg string) { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Node: node, |
|
||||||
Category: "logic", |
|
||||||
Failure: msg, |
|
||||||
}) |
|
||||||
} |
|
@ -1,60 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// ContextAsArgumentRule lints given else constructs.
|
|
||||||
type ContextAsArgumentRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *ContextAsArgumentRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
fileAst := file.AST |
|
||||||
walker := lintContextArguments{ |
|
||||||
file: file, |
|
||||||
fileAst: fileAst, |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
ast.Walk(walker, fileAst) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *ContextAsArgumentRule) Name() string { |
|
||||||
return "context-as-argument" |
|
||||||
} |
|
||||||
|
|
||||||
type lintContextArguments struct { |
|
||||||
file *lint.File |
|
||||||
fileAst *ast.File |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintContextArguments) Visit(n ast.Node) ast.Visitor { |
|
||||||
fn, ok := n.(*ast.FuncDecl) |
|
||||||
if !ok || len(fn.Type.Params.List) <= 1 { |
|
||||||
return w |
|
||||||
} |
|
||||||
// A context.Context should be the first parameter of a function.
|
|
||||||
// Flag any that show up after the first.
|
|
||||||
for _, arg := range fn.Type.Params.List[1:] { |
|
||||||
if isPkgDot(arg.Type, "context", "Context") { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Node: fn, |
|
||||||
Category: "arg-order", |
|
||||||
Failure: "context.Context should be the first parameter of a function", |
|
||||||
Confidence: 0.9, |
|
||||||
}) |
|
||||||
break // only flag one
|
|
||||||
} |
|
||||||
} |
|
||||||
return w |
|
||||||
} |
|
@ -1,81 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"go/ast" |
|
||||||
"go/types" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// ContextKeysType lints given else constructs.
|
|
||||||
type ContextKeysType struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *ContextKeysType) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
fileAst := file.AST |
|
||||||
walker := lintContextKeyTypes{ |
|
||||||
file: file, |
|
||||||
fileAst: fileAst, |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
file.Pkg.TypeCheck() |
|
||||||
ast.Walk(walker, fileAst) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *ContextKeysType) Name() string { |
|
||||||
return "context-keys-type" |
|
||||||
} |
|
||||||
|
|
||||||
type lintContextKeyTypes struct { |
|
||||||
file *lint.File |
|
||||||
fileAst *ast.File |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintContextKeyTypes) Visit(n ast.Node) ast.Visitor { |
|
||||||
switch n := n.(type) { |
|
||||||
case *ast.CallExpr: |
|
||||||
checkContextKeyType(w, n) |
|
||||||
} |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
func checkContextKeyType(w lintContextKeyTypes, x *ast.CallExpr) { |
|
||||||
f := w.file |
|
||||||
sel, ok := x.Fun.(*ast.SelectorExpr) |
|
||||||
if !ok { |
|
||||||
return |
|
||||||
} |
|
||||||
pkg, ok := sel.X.(*ast.Ident) |
|
||||||
if !ok || pkg.Name != "context" { |
|
||||||
return |
|
||||||
} |
|
||||||
if sel.Sel.Name != "WithValue" { |
|
||||||
return |
|
||||||
} |
|
||||||
|
|
||||||
// key is second argument to context.WithValue
|
|
||||||
if len(x.Args) != 3 { |
|
||||||
return |
|
||||||
} |
|
||||||
key := f.Pkg.TypesInfo.Types[x.Args[1]] |
|
||||||
|
|
||||||
if ktyp, ok := key.Type.(*types.Basic); ok && ktyp.Kind() != types.Invalid { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Node: x, |
|
||||||
Category: "content", |
|
||||||
Failure: fmt.Sprintf("should not use basic type %s as key in context.WithValue", key.Type), |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
@ -1,115 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"go/ast" |
|
||||||
"go/token" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// Based on https://github.com/fzipp/gocyclo
|
|
||||||
|
|
||||||
// CyclomaticRule lints given else constructs.
|
|
||||||
type CyclomaticRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *CyclomaticRule) Apply(file *lint.File, arguments lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
complexity, ok := arguments[0].(int64) // Alt. non panicking version
|
|
||||||
if !ok { |
|
||||||
panic("invalid argument for cyclomatic complexity") |
|
||||||
} |
|
||||||
|
|
||||||
fileAst := file.AST |
|
||||||
walker := lintCyclomatic{ |
|
||||||
file: file, |
|
||||||
complexity: int(complexity), |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
ast.Walk(walker, fileAst) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *CyclomaticRule) Name() string { |
|
||||||
return "cyclomatic" |
|
||||||
} |
|
||||||
|
|
||||||
type lintCyclomatic struct { |
|
||||||
file *lint.File |
|
||||||
complexity int |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintCyclomatic) Visit(_ ast.Node) ast.Visitor { |
|
||||||
f := w.file |
|
||||||
for _, decl := range f.AST.Decls { |
|
||||||
if fn, ok := decl.(*ast.FuncDecl); ok { |
|
||||||
c := complexity(fn) |
|
||||||
if c > w.complexity { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Category: "maintenance", |
|
||||||
Failure: fmt.Sprintf("function %s has cyclomatic complexity %d", funcName(fn), c), |
|
||||||
Node: fn, |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
return nil |
|
||||||
} |
|
||||||
|
|
||||||
// funcName returns the name representation of a function or method:
|
|
||||||
// "(Type).Name" for methods or simply "Name" for functions.
|
|
||||||
func funcName(fn *ast.FuncDecl) string { |
|
||||||
if fn.Recv != nil { |
|
||||||
if fn.Recv.NumFields() > 0 { |
|
||||||
typ := fn.Recv.List[0].Type |
|
||||||
return fmt.Sprintf("(%s).%s", recvString(typ), fn.Name) |
|
||||||
} |
|
||||||
} |
|
||||||
return fn.Name.Name |
|
||||||
} |
|
||||||
|
|
||||||
// recvString returns a string representation of recv of the
|
|
||||||
// form "T", "*T", or "BADRECV" (if not a proper receiver type).
|
|
||||||
func recvString(recv ast.Expr) string { |
|
||||||
switch t := recv.(type) { |
|
||||||
case *ast.Ident: |
|
||||||
return t.Name |
|
||||||
case *ast.StarExpr: |
|
||||||
return "*" + recvString(t.X) |
|
||||||
} |
|
||||||
return "BADRECV" |
|
||||||
} |
|
||||||
|
|
||||||
// complexity calculates the cyclomatic complexity of a function.
|
|
||||||
func complexity(fn *ast.FuncDecl) int { |
|
||||||
v := complexityVisitor{} |
|
||||||
ast.Walk(&v, fn) |
|
||||||
return v.Complexity |
|
||||||
} |
|
||||||
|
|
||||||
type complexityVisitor struct { |
|
||||||
// Complexity is the cyclomatic complexity
|
|
||||||
Complexity int |
|
||||||
} |
|
||||||
|
|
||||||
// Visit implements the ast.Visitor interface.
|
|
||||||
func (v *complexityVisitor) Visit(n ast.Node) ast.Visitor { |
|
||||||
switch n := n.(type) { |
|
||||||
case *ast.FuncDecl, *ast.IfStmt, *ast.ForStmt, *ast.RangeStmt, *ast.CaseClause, *ast.CommClause: |
|
||||||
v.Complexity++ |
|
||||||
case *ast.BinaryExpr: |
|
||||||
if n.Op == token.LAND || n.Op == token.LOR { |
|
||||||
v.Complexity++ |
|
||||||
} |
|
||||||
} |
|
||||||
return v |
|
||||||
} |
|
@ -1,94 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"go/ast" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// DeepExitRule lints program exit at functions other than main or init.
|
|
||||||
type DeepExitRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *DeepExitRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
onFailure := func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
} |
|
||||||
|
|
||||||
var exitFunctions = map[string]map[string]bool{ |
|
||||||
"os": map[string]bool{"Exit": true}, |
|
||||||
"syscall": map[string]bool{"Exit": true}, |
|
||||||
"log": map[string]bool{ |
|
||||||
"Fatal": true, |
|
||||||
"Fatalf": true, |
|
||||||
"Fatalln": true, |
|
||||||
"Panic": true, |
|
||||||
"Panicf": true, |
|
||||||
"Panicln": true, |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
w := lintDeepExit{onFailure, exitFunctions, file.IsTest()} |
|
||||||
ast.Walk(w, file.AST) |
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *DeepExitRule) Name() string { |
|
||||||
return "deep-exit" |
|
||||||
} |
|
||||||
|
|
||||||
type lintDeepExit struct { |
|
||||||
onFailure func(lint.Failure) |
|
||||||
exitFunctions map[string]map[string]bool |
|
||||||
isTestFile bool |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintDeepExit) Visit(node ast.Node) ast.Visitor { |
|
||||||
if fd, ok := node.(*ast.FuncDecl); ok { |
|
||||||
if w.mustIgnore(fd) { |
|
||||||
return nil // skip analysis of this function
|
|
||||||
} |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
se, ok := node.(*ast.ExprStmt) |
|
||||||
if !ok { |
|
||||||
return w |
|
||||||
} |
|
||||||
ce, ok := se.X.(*ast.CallExpr) |
|
||||||
if !ok { |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
fc, ok := ce.Fun.(*ast.SelectorExpr) |
|
||||||
if !ok { |
|
||||||
return w |
|
||||||
} |
|
||||||
id, ok := fc.X.(*ast.Ident) |
|
||||||
if !ok { |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
fn := fc.Sel.Name |
|
||||||
pkg := id.Name |
|
||||||
if w.exitFunctions[pkg] != nil && w.exitFunctions[pkg][fn] { // it's a call to an exit function
|
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Node: ce, |
|
||||||
Category: "bad practice", |
|
||||||
Failure: fmt.Sprintf("calls to %s.%s only in main() or init() functions", pkg, fn), |
|
||||||
}) |
|
||||||
} |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
func (w *lintDeepExit) mustIgnore(fd *ast.FuncDecl) bool { |
|
||||||
fn := fd.Name.Name |
|
||||||
|
|
||||||
return fn == "init" || fn == "main" || (w.isTestFile && fn == "TestMain") |
|
||||||
} |
|
@ -1,137 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"go/ast" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// DeferRule lints unused params in functions.
|
|
||||||
type DeferRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *DeferRule) Apply(file *lint.File, arguments lint.Arguments) []lint.Failure { |
|
||||||
allow := r.allowFromArgs(arguments) |
|
||||||
|
|
||||||
var failures []lint.Failure |
|
||||||
onFailure := func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
} |
|
||||||
|
|
||||||
w := lintDeferRule{onFailure: onFailure, allow: allow} |
|
||||||
|
|
||||||
ast.Walk(w, file.AST) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *DeferRule) Name() string { |
|
||||||
return "defer" |
|
||||||
} |
|
||||||
|
|
||||||
func (r *DeferRule) allowFromArgs(args lint.Arguments) map[string]bool { |
|
||||||
if len(args) < 1 { |
|
||||||
allow := map[string]bool{ |
|
||||||
"loop": true, |
|
||||||
"call-chain": true, |
|
||||||
"method-call": true, |
|
||||||
"return": true, |
|
||||||
"recover": true, |
|
||||||
} |
|
||||||
|
|
||||||
return allow |
|
||||||
} |
|
||||||
|
|
||||||
aa, ok := args[0].([]interface{}) |
|
||||||
if !ok { |
|
||||||
panic(fmt.Sprintf("Invalid argument '%v' for 'defer' rule. Expecting []string, got %T", args[0], args[0])) |
|
||||||
} |
|
||||||
|
|
||||||
allow := make(map[string]bool, len(aa)) |
|
||||||
for _, subcase := range aa { |
|
||||||
sc, ok := subcase.(string) |
|
||||||
if !ok { |
|
||||||
panic(fmt.Sprintf("Invalid argument '%v' for 'defer' rule. Expecting string, got %T", subcase, subcase)) |
|
||||||
} |
|
||||||
allow[sc] = true |
|
||||||
} |
|
||||||
|
|
||||||
return allow |
|
||||||
} |
|
||||||
|
|
||||||
type lintDeferRule struct { |
|
||||||
onFailure func(lint.Failure) |
|
||||||
inALoop bool |
|
||||||
inADefer bool |
|
||||||
inAFuncLit bool |
|
||||||
allow map[string]bool |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintDeferRule) Visit(node ast.Node) ast.Visitor { |
|
||||||
switch n := node.(type) { |
|
||||||
case *ast.ForStmt: |
|
||||||
w.visitSubtree(n.Body, w.inADefer, true, w.inAFuncLit) |
|
||||||
return nil |
|
||||||
case *ast.RangeStmt: |
|
||||||
w.visitSubtree(n.Body, w.inADefer, true, w.inAFuncLit) |
|
||||||
return nil |
|
||||||
case *ast.FuncLit: |
|
||||||
w.visitSubtree(n.Body, w.inADefer, false, true) |
|
||||||
return nil |
|
||||||
case *ast.ReturnStmt: |
|
||||||
if len(n.Results) != 0 && w.inADefer && w.inAFuncLit { |
|
||||||
w.newFailure("return in a defer function has no effect", n, 1.0, "logic", "return") |
|
||||||
} |
|
||||||
case *ast.CallExpr: |
|
||||||
if isIdent(n.Fun, "recover") && !w.inADefer { |
|
||||||
// confidence is not 1 because recover can be in a function that is deferred elsewhere
|
|
||||||
w.newFailure("recover must be called inside a deferred function", n, 0.8, "logic", "recover") |
|
||||||
} |
|
||||||
case *ast.DeferStmt: |
|
||||||
w.visitSubtree(n.Call.Fun, true, false, false) |
|
||||||
|
|
||||||
if w.inALoop { |
|
||||||
w.newFailure("prefer not to defer inside loops", n, 1.0, "bad practice", "loop") |
|
||||||
} |
|
||||||
|
|
||||||
switch fn := n.Call.Fun.(type) { |
|
||||||
case *ast.CallExpr: |
|
||||||
w.newFailure("prefer not to defer chains of function calls", fn, 1.0, "bad practice", "call-chain") |
|
||||||
case *ast.SelectorExpr: |
|
||||||
if id, ok := fn.X.(*ast.Ident); ok { |
|
||||||
isMethodCall := id != nil && id.Obj != nil && id.Obj.Kind == ast.Typ |
|
||||||
if isMethodCall { |
|
||||||
w.newFailure("be careful when deferring calls to methods without pointer receiver", fn, 0.8, "bad practice", "method-call") |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
return nil |
|
||||||
} |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintDeferRule) visitSubtree(n ast.Node, inADefer, inALoop, inAFuncLit bool) { |
|
||||||
nw := &lintDeferRule{ |
|
||||||
onFailure: w.onFailure, |
|
||||||
inADefer: inADefer, |
|
||||||
inALoop: inALoop, |
|
||||||
inAFuncLit: inAFuncLit, |
|
||||||
allow: w.allow} |
|
||||||
ast.Walk(nw, n) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintDeferRule) newFailure(msg string, node ast.Node, confidence float64, cat string, subcase string) { |
|
||||||
if !w.allow[subcase] { |
|
||||||
return |
|
||||||
} |
|
||||||
|
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: confidence, |
|
||||||
Node: node, |
|
||||||
Category: cat, |
|
||||||
Failure: msg, |
|
||||||
}) |
|
||||||
} |
|
@ -1,54 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// DotImportsRule lints given else constructs.
|
|
||||||
type DotImportsRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *DotImportsRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
fileAst := file.AST |
|
||||||
walker := lintImports{ |
|
||||||
file: file, |
|
||||||
fileAst: fileAst, |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
ast.Walk(walker, fileAst) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *DotImportsRule) Name() string { |
|
||||||
return "dot-imports" |
|
||||||
} |
|
||||||
|
|
||||||
type lintImports struct { |
|
||||||
file *lint.File |
|
||||||
fileAst *ast.File |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintImports) Visit(_ ast.Node) ast.Visitor { |
|
||||||
for i, is := range w.fileAst.Imports { |
|
||||||
_ = i |
|
||||||
if is.Name != nil && is.Name.Name == "." && !w.file.IsTest() { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Failure: "should not use dot imports", |
|
||||||
Node: is, |
|
||||||
Category: "imports", |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
||||||
return nil |
|
||||||
} |
|
@ -1,39 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// DuplicatedImportsRule lints given else constructs.
|
|
||||||
type DuplicatedImportsRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *DuplicatedImportsRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
impPaths := map[string]struct{}{} |
|
||||||
for _, imp := range file.AST.Imports { |
|
||||||
path := imp.Path.Value |
|
||||||
_, ok := impPaths[path] |
|
||||||
if ok { |
|
||||||
failures = append(failures, lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Failure: fmt.Sprintf("Package %s already imported", path), |
|
||||||
Node: imp, |
|
||||||
Category: "imports", |
|
||||||
}) |
|
||||||
continue |
|
||||||
} |
|
||||||
|
|
||||||
impPaths[path] = struct{}{} |
|
||||||
} |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *DuplicatedImportsRule) Name() string { |
|
||||||
return "duplicated-imports" |
|
||||||
} |
|
@ -1,78 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// EarlyReturnRule lints given else constructs.
|
|
||||||
type EarlyReturnRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *EarlyReturnRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
onFailure := func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
} |
|
||||||
|
|
||||||
w := lintEarlyReturnRule{onFailure: onFailure} |
|
||||||
ast.Walk(w, file.AST) |
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *EarlyReturnRule) Name() string { |
|
||||||
return "early-return" |
|
||||||
} |
|
||||||
|
|
||||||
type lintEarlyReturnRule struct { |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintEarlyReturnRule) Visit(node ast.Node) ast.Visitor { |
|
||||||
switch n := node.(type) { |
|
||||||
case *ast.IfStmt: |
|
||||||
if n.Else == nil { |
|
||||||
// no else branch
|
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
elseBlock, ok := n.Else.(*ast.BlockStmt) |
|
||||||
if !ok { |
|
||||||
// is if-else-if
|
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
lenElseBlock := len(elseBlock.List) |
|
||||||
if lenElseBlock < 1 { |
|
||||||
// empty else block, continue (there is another rule that warns on empty blocks)
|
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
lenThenBlock := len(n.Body.List) |
|
||||||
if lenThenBlock < 1 { |
|
||||||
// then block is empty thus the stmt can be simplified
|
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Node: n, |
|
||||||
Failure: "if c { } else {... return} can be simplified to if !c { ... return }", |
|
||||||
}) |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
_, lastThenStmtIsReturn := n.Body.List[lenThenBlock-1].(*ast.ReturnStmt) |
|
||||||
_, lastElseStmtIsReturn := elseBlock.List[lenElseBlock-1].(*ast.ReturnStmt) |
|
||||||
if lastElseStmtIsReturn && !lastThenStmtIsReturn { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Node: n, |
|
||||||
Failure: "if c {...} else {... return } can be simplified to if !c { ... return } ...", |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
@ -1,65 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// EmptyBlockRule lints given else constructs.
|
|
||||||
type EmptyBlockRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *EmptyBlockRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
onFailure := func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
} |
|
||||||
|
|
||||||
w := lintEmptyBlock{make(map[*ast.BlockStmt]bool, 0), onFailure} |
|
||||||
ast.Walk(w, file.AST) |
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *EmptyBlockRule) Name() string { |
|
||||||
return "empty-block" |
|
||||||
} |
|
||||||
|
|
||||||
type lintEmptyBlock struct { |
|
||||||
ignore map[*ast.BlockStmt]bool |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintEmptyBlock) Visit(node ast.Node) ast.Visitor { |
|
||||||
switch n := node.(type) { |
|
||||||
case *ast.FuncDecl: |
|
||||||
w.ignore[n.Body] = true |
|
||||||
return w |
|
||||||
case *ast.FuncLit: |
|
||||||
w.ignore[n.Body] = true |
|
||||||
return w |
|
||||||
case *ast.RangeStmt: |
|
||||||
if len(n.Body.List) == 0 { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 0.9, |
|
||||||
Node: n, |
|
||||||
Category: "logic", |
|
||||||
Failure: "this block is empty, you can remove it", |
|
||||||
}) |
|
||||||
return nil // skip visiting the range subtree (it will produce a duplicated failure)
|
|
||||||
} |
|
||||||
case *ast.BlockStmt: |
|
||||||
if !w.ignore[n] && len(n.List) == 0 { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Node: n, |
|
||||||
Category: "logic", |
|
||||||
Failure: "this block is empty, you can remove it", |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
@ -1,113 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
"go/token" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// EmptyLinesRule lints empty lines in blocks.
|
|
||||||
type EmptyLinesRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *EmptyLinesRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
onFailure := func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
} |
|
||||||
|
|
||||||
w := lintEmptyLines{file, file.CommentMap(), onFailure} |
|
||||||
ast.Walk(w, file.AST) |
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *EmptyLinesRule) Name() string { |
|
||||||
return "empty-lines" |
|
||||||
} |
|
||||||
|
|
||||||
type lintEmptyLines struct { |
|
||||||
file *lint.File |
|
||||||
cmap ast.CommentMap |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintEmptyLines) Visit(node ast.Node) ast.Visitor { |
|
||||||
block, ok := node.(*ast.BlockStmt) |
|
||||||
if !ok { |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
w.checkStart(block) |
|
||||||
w.checkEnd(block) |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintEmptyLines) checkStart(block *ast.BlockStmt) { |
|
||||||
if len(block.List) == 0 { |
|
||||||
return |
|
||||||
} |
|
||||||
|
|
||||||
start := w.position(block.Lbrace) |
|
||||||
firstNode := block.List[0] |
|
||||||
|
|
||||||
if w.commentBetween(start, firstNode) { |
|
||||||
return |
|
||||||
} |
|
||||||
|
|
||||||
first := w.position(firstNode.Pos()) |
|
||||||
if first.Line-start.Line > 1 { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Node: block, |
|
||||||
Category: "style", |
|
||||||
Failure: "extra empty line at the start of a block", |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintEmptyLines) checkEnd(block *ast.BlockStmt) { |
|
||||||
if len(block.List) < 1 { |
|
||||||
return |
|
||||||
} |
|
||||||
|
|
||||||
end := w.position(block.Rbrace) |
|
||||||
lastNode := block.List[len(block.List)-1] |
|
||||||
|
|
||||||
if w.commentBetween(end, lastNode) { |
|
||||||
return |
|
||||||
} |
|
||||||
|
|
||||||
last := w.position(lastNode.End()) |
|
||||||
if end.Line-last.Line > 1 { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Node: lastNode, |
|
||||||
Category: "style", |
|
||||||
Failure: "extra empty line at the end of a block", |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintEmptyLines) commentBetween(position token.Position, node ast.Node) bool { |
|
||||||
comments := w.cmap.Filter(node).Comments() |
|
||||||
if len(comments) == 0 { |
|
||||||
return false |
|
||||||
} |
|
||||||
|
|
||||||
for _, comment := range comments { |
|
||||||
start, end := w.position(comment.Pos()), w.position(comment.End()) |
|
||||||
if start.Line-position.Line == 1 || position.Line-end.Line == 1 { |
|
||||||
return true |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return false |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintEmptyLines) position(pos token.Pos) token.Position { |
|
||||||
return w.file.ToPosition(pos) |
|
||||||
} |
|
@ -1,79 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"go/ast" |
|
||||||
"go/token" |
|
||||||
"strings" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// ErrorNamingRule lints given else constructs.
|
|
||||||
type ErrorNamingRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *ErrorNamingRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
fileAst := file.AST |
|
||||||
walker := lintErrors{ |
|
||||||
file: file, |
|
||||||
fileAst: fileAst, |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
ast.Walk(walker, fileAst) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *ErrorNamingRule) Name() string { |
|
||||||
return "error-naming" |
|
||||||
} |
|
||||||
|
|
||||||
type lintErrors struct { |
|
||||||
file *lint.File |
|
||||||
fileAst *ast.File |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintErrors) Visit(_ ast.Node) ast.Visitor { |
|
||||||
for _, decl := range w.fileAst.Decls { |
|
||||||
gd, ok := decl.(*ast.GenDecl) |
|
||||||
if !ok || gd.Tok != token.VAR { |
|
||||||
continue |
|
||||||
} |
|
||||||
for _, spec := range gd.Specs { |
|
||||||
spec := spec.(*ast.ValueSpec) |
|
||||||
if len(spec.Names) != 1 || len(spec.Values) != 1 { |
|
||||||
continue |
|
||||||
} |
|
||||||
ce, ok := spec.Values[0].(*ast.CallExpr) |
|
||||||
if !ok { |
|
||||||
continue |
|
||||||
} |
|
||||||
if !isPkgDot(ce.Fun, "errors", "New") && !isPkgDot(ce.Fun, "fmt", "Errorf") { |
|
||||||
continue |
|
||||||
} |
|
||||||
|
|
||||||
id := spec.Names[0] |
|
||||||
prefix := "err" |
|
||||||
if id.IsExported() { |
|
||||||
prefix = "Err" |
|
||||||
} |
|
||||||
if !strings.HasPrefix(id.Name, prefix) { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Node: id, |
|
||||||
Confidence: 0.9, |
|
||||||
Category: "naming", |
|
||||||
Failure: fmt.Sprintf("error var %s should have name of the form %sFoo", id.Name, prefix), |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
return nil |
|
||||||
} |
|
@ -1,67 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// ErrorReturnRule lints given else constructs.
|
|
||||||
type ErrorReturnRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *ErrorReturnRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
fileAst := file.AST |
|
||||||
walker := lintErrorReturn{ |
|
||||||
file: file, |
|
||||||
fileAst: fileAst, |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
ast.Walk(walker, fileAst) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *ErrorReturnRule) Name() string { |
|
||||||
return "error-return" |
|
||||||
} |
|
||||||
|
|
||||||
type lintErrorReturn struct { |
|
||||||
file *lint.File |
|
||||||
fileAst *ast.File |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintErrorReturn) Visit(n ast.Node) ast.Visitor { |
|
||||||
fn, ok := n.(*ast.FuncDecl) |
|
||||||
if !ok || fn.Type.Results == nil { |
|
||||||
return w |
|
||||||
} |
|
||||||
ret := fn.Type.Results.List |
|
||||||
if len(ret) <= 1 { |
|
||||||
return w |
|
||||||
} |
|
||||||
if isIdent(ret[len(ret)-1].Type, "error") { |
|
||||||
return nil |
|
||||||
} |
|
||||||
// An error return parameter should be the last parameter.
|
|
||||||
// Flag any error parameters found before the last.
|
|
||||||
for _, r := range ret[:len(ret)-1] { |
|
||||||
if isIdent(r.Type, "error") { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Category: "arg-order", |
|
||||||
Confidence: 0.9, |
|
||||||
Node: fn, |
|
||||||
Failure: "error should be the last type when returning multiple items", |
|
||||||
}) |
|
||||||
break // only flag one
|
|
||||||
} |
|
||||||
} |
|
||||||
return w |
|
||||||
} |
|
@ -1,98 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
"go/token" |
|
||||||
"strconv" |
|
||||||
"unicode" |
|
||||||
"unicode/utf8" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// ErrorStringsRule lints given else constructs.
|
|
||||||
type ErrorStringsRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *ErrorStringsRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
fileAst := file.AST |
|
||||||
walker := lintErrorStrings{ |
|
||||||
file: file, |
|
||||||
fileAst: fileAst, |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
ast.Walk(walker, fileAst) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *ErrorStringsRule) Name() string { |
|
||||||
return "error-strings" |
|
||||||
} |
|
||||||
|
|
||||||
type lintErrorStrings struct { |
|
||||||
file *lint.File |
|
||||||
fileAst *ast.File |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintErrorStrings) Visit(n ast.Node) ast.Visitor { |
|
||||||
ce, ok := n.(*ast.CallExpr) |
|
||||||
if !ok { |
|
||||||
return w |
|
||||||
} |
|
||||||
if !isPkgDot(ce.Fun, "errors", "New") && !isPkgDot(ce.Fun, "fmt", "Errorf") { |
|
||||||
return w |
|
||||||
} |
|
||||||
if len(ce.Args) < 1 { |
|
||||||
return w |
|
||||||
} |
|
||||||
str, ok := ce.Args[0].(*ast.BasicLit) |
|
||||||
if !ok || str.Kind != token.STRING { |
|
||||||
return w |
|
||||||
} |
|
||||||
s, _ := strconv.Unquote(str.Value) // can assume well-formed Go
|
|
||||||
if s == "" { |
|
||||||
return w |
|
||||||
} |
|
||||||
clean, conf := lintErrorString(s) |
|
||||||
if clean { |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Node: str, |
|
||||||
Confidence: conf, |
|
||||||
Category: "errors", |
|
||||||
Failure: "error strings should not be capitalized or end with punctuation or a newline", |
|
||||||
}) |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
func lintErrorString(s string) (isClean bool, conf float64) { |
|
||||||
const basicConfidence = 0.8 |
|
||||||
const capConfidence = basicConfidence - 0.2 |
|
||||||
first, firstN := utf8.DecodeRuneInString(s) |
|
||||||
last, _ := utf8.DecodeLastRuneInString(s) |
|
||||||
if last == '.' || last == ':' || last == '!' || last == '\n' { |
|
||||||
return false, basicConfidence |
|
||||||
} |
|
||||||
if unicode.IsUpper(first) { |
|
||||||
// People use proper nouns and exported Go identifiers in error strings,
|
|
||||||
// so decrease the confidence of warnings for capitalization.
|
|
||||||
if len(s) <= firstN { |
|
||||||
return false, capConfidence |
|
||||||
} |
|
||||||
// Flag strings starting with something that doesn't look like an initialism.
|
|
||||||
if second, _ := utf8.DecodeRuneInString(s[firstN:]); !unicode.IsUpper(second) { |
|
||||||
return false, capConfidence |
|
||||||
} |
|
||||||
} |
|
||||||
return true, 0 |
|
||||||
} |
|
@ -1,93 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"go/ast" |
|
||||||
"regexp" |
|
||||||
"strings" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// ErrorfRule lints given else constructs.
|
|
||||||
type ErrorfRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *ErrorfRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
fileAst := file.AST |
|
||||||
walker := lintErrorf{ |
|
||||||
file: file, |
|
||||||
fileAst: fileAst, |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
file.Pkg.TypeCheck() |
|
||||||
ast.Walk(walker, fileAst) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *ErrorfRule) Name() string { |
|
||||||
return "errorf" |
|
||||||
} |
|
||||||
|
|
||||||
type lintErrorf struct { |
|
||||||
file *lint.File |
|
||||||
fileAst *ast.File |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintErrorf) Visit(n ast.Node) ast.Visitor { |
|
||||||
ce, ok := n.(*ast.CallExpr) |
|
||||||
if !ok || len(ce.Args) != 1 { |
|
||||||
return w |
|
||||||
} |
|
||||||
isErrorsNew := isPkgDot(ce.Fun, "errors", "New") |
|
||||||
var isTestingError bool |
|
||||||
se, ok := ce.Fun.(*ast.SelectorExpr) |
|
||||||
if ok && se.Sel.Name == "Error" { |
|
||||||
if typ := w.file.Pkg.TypeOf(se.X); typ != nil { |
|
||||||
isTestingError = typ.String() == "*testing.T" |
|
||||||
} |
|
||||||
} |
|
||||||
if !isErrorsNew && !isTestingError { |
|
||||||
return w |
|
||||||
} |
|
||||||
arg := ce.Args[0] |
|
||||||
ce, ok = arg.(*ast.CallExpr) |
|
||||||
if !ok || !isPkgDot(ce.Fun, "fmt", "Sprintf") { |
|
||||||
return w |
|
||||||
} |
|
||||||
errorfPrefix := "fmt" |
|
||||||
if isTestingError { |
|
||||||
errorfPrefix = w.file.Render(se.X) |
|
||||||
} |
|
||||||
|
|
||||||
failure := lint.Failure{ |
|
||||||
Category: "errors", |
|
||||||
Node: n, |
|
||||||
Confidence: 1, |
|
||||||
Failure: fmt.Sprintf("should replace %s(fmt.Sprintf(...)) with %s.Errorf(...)", w.file.Render(se), errorfPrefix), |
|
||||||
} |
|
||||||
|
|
||||||
m := srcLineWithMatch(w.file, ce, `^(.*)`+w.file.Render(se)+`\(fmt\.Sprintf\((.*)\)\)(.*)$`) |
|
||||||
if m != nil { |
|
||||||
failure.ReplacementLine = m[1] + errorfPrefix + ".Errorf(" + m[2] + ")" + m[3] |
|
||||||
} |
|
||||||
|
|
||||||
w.onFailure(failure) |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
func srcLineWithMatch(file *lint.File, node ast.Node, pattern string) (m []string) { |
|
||||||
line := srcLine(file.Content(), file.ToPosition(node.Pos())) |
|
||||||
line = strings.TrimSuffix(line, "\n") |
|
||||||
rx := regexp.MustCompile(pattern) |
|
||||||
return rx.FindStringSubmatch(line) |
|
||||||
} |
|
@ -1,272 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"go/ast" |
|
||||||
"go/token" |
|
||||||
"strings" |
|
||||||
"unicode" |
|
||||||
"unicode/utf8" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// ExportedRule lints given else constructs.
|
|
||||||
type ExportedRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *ExportedRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
if isTest(file) { |
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
fileAst := file.AST |
|
||||||
walker := lintExported{ |
|
||||||
file: file, |
|
||||||
fileAst: fileAst, |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
genDeclMissingComments: make(map[*ast.GenDecl]bool), |
|
||||||
} |
|
||||||
|
|
||||||
ast.Walk(&walker, fileAst) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *ExportedRule) Name() string { |
|
||||||
return "exported" |
|
||||||
} |
|
||||||
|
|
||||||
type lintExported struct { |
|
||||||
file *lint.File |
|
||||||
fileAst *ast.File |
|
||||||
lastGen *ast.GenDecl |
|
||||||
genDeclMissingComments map[*ast.GenDecl]bool |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w *lintExported) lintFuncDoc(fn *ast.FuncDecl) { |
|
||||||
if !ast.IsExported(fn.Name.Name) { |
|
||||||
// func is unexported
|
|
||||||
return |
|
||||||
} |
|
||||||
kind := "function" |
|
||||||
name := fn.Name.Name |
|
||||||
if fn.Recv != nil && len(fn.Recv.List) > 0 { |
|
||||||
// method
|
|
||||||
kind = "method" |
|
||||||
recv := receiverType(fn) |
|
||||||
if !ast.IsExported(recv) { |
|
||||||
// receiver is unexported
|
|
||||||
return |
|
||||||
} |
|
||||||
if commonMethods[name] { |
|
||||||
return |
|
||||||
} |
|
||||||
switch name { |
|
||||||
case "Len", "Less", "Swap": |
|
||||||
if w.file.Pkg.Sortable[recv] { |
|
||||||
return |
|
||||||
} |
|
||||||
} |
|
||||||
name = recv + "." + name |
|
||||||
} |
|
||||||
if fn.Doc == nil { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Node: fn, |
|
||||||
Confidence: 1, |
|
||||||
Category: "comments", |
|
||||||
Failure: fmt.Sprintf("exported %s %s should have comment or be unexported", kind, name), |
|
||||||
}) |
|
||||||
return |
|
||||||
} |
|
||||||
s := normalizeText(fn.Doc.Text()) |
|
||||||
prefix := fn.Name.Name + " " |
|
||||||
if !strings.HasPrefix(s, prefix) { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Node: fn.Doc, |
|
||||||
Confidence: 0.8, |
|
||||||
Category: "comments", |
|
||||||
Failure: fmt.Sprintf(`comment on exported %s %s should be of the form "%s..."`, kind, name, prefix), |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
func (w *lintExported) checkStutter(id *ast.Ident, thing string) { |
|
||||||
pkg, name := w.fileAst.Name.Name, id.Name |
|
||||||
if !ast.IsExported(name) { |
|
||||||
// unexported name
|
|
||||||
return |
|
||||||
} |
|
||||||
// A name stutters if the package name is a strict prefix
|
|
||||||
// and the next character of the name starts a new word.
|
|
||||||
if len(name) <= len(pkg) { |
|
||||||
// name is too short to stutter.
|
|
||||||
// This permits the name to be the same as the package name.
|
|
||||||
return |
|
||||||
} |
|
||||||
if !strings.EqualFold(pkg, name[:len(pkg)]) { |
|
||||||
return |
|
||||||
} |
|
||||||
// We can assume the name is well-formed UTF-8.
|
|
||||||
// If the next rune after the package name is uppercase or an underscore
|
|
||||||
// the it's starting a new word and thus this name stutters.
|
|
||||||
rem := name[len(pkg):] |
|
||||||
if next, _ := utf8.DecodeRuneInString(rem); next == '_' || unicode.IsUpper(next) { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Node: id, |
|
||||||
Confidence: 0.8, |
|
||||||
Category: "naming", |
|
||||||
Failure: fmt.Sprintf("%s name will be used as %s.%s by other packages, and that stutters; consider calling this %s", thing, pkg, name, rem), |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
func (w *lintExported) lintTypeDoc(t *ast.TypeSpec, doc *ast.CommentGroup) { |
|
||||||
if !ast.IsExported(t.Name.Name) { |
|
||||||
return |
|
||||||
} |
|
||||||
if doc == nil { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Node: t, |
|
||||||
Confidence: 1, |
|
||||||
Category: "comments", |
|
||||||
Failure: fmt.Sprintf("exported type %v should have comment or be unexported", t.Name), |
|
||||||
}) |
|
||||||
return |
|
||||||
} |
|
||||||
|
|
||||||
s := normalizeText(doc.Text()) |
|
||||||
articles := [...]string{"A", "An", "The", "This"} |
|
||||||
for _, a := range articles { |
|
||||||
if t.Name.Name == a { |
|
||||||
continue |
|
||||||
} |
|
||||||
if strings.HasPrefix(s, a+" ") { |
|
||||||
s = s[len(a)+1:] |
|
||||||
break |
|
||||||
} |
|
||||||
} |
|
||||||
if !strings.HasPrefix(s, t.Name.Name+" ") { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Node: doc, |
|
||||||
Confidence: 1, |
|
||||||
Category: "comments", |
|
||||||
Failure: fmt.Sprintf(`comment on exported type %v should be of the form "%v ..." (with optional leading article)`, t.Name, t.Name), |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
func (w *lintExported) lintValueSpecDoc(vs *ast.ValueSpec, gd *ast.GenDecl, genDeclMissingComments map[*ast.GenDecl]bool) { |
|
||||||
kind := "var" |
|
||||||
if gd.Tok == token.CONST { |
|
||||||
kind = "const" |
|
||||||
} |
|
||||||
|
|
||||||
if len(vs.Names) > 1 { |
|
||||||
// Check that none are exported except for the first.
|
|
||||||
for _, n := range vs.Names[1:] { |
|
||||||
if ast.IsExported(n.Name) { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Category: "comments", |
|
||||||
Confidence: 1, |
|
||||||
Failure: fmt.Sprintf("exported %s %s should have its own declaration", kind, n.Name), |
|
||||||
Node: vs, |
|
||||||
}) |
|
||||||
return |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// Only one name.
|
|
||||||
name := vs.Names[0].Name |
|
||||||
if !ast.IsExported(name) { |
|
||||||
return |
|
||||||
} |
|
||||||
|
|
||||||
if vs.Doc == nil && gd.Doc == nil { |
|
||||||
if genDeclMissingComments[gd] { |
|
||||||
return |
|
||||||
} |
|
||||||
block := "" |
|
||||||
if kind == "const" && gd.Lparen.IsValid() { |
|
||||||
block = " (or a comment on this block)" |
|
||||||
} |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Node: vs, |
|
||||||
Category: "comments", |
|
||||||
Failure: fmt.Sprintf("exported %s %s should have comment%s or be unexported", kind, name, block), |
|
||||||
}) |
|
||||||
genDeclMissingComments[gd] = true |
|
||||||
return |
|
||||||
} |
|
||||||
// If this GenDecl has parens and a comment, we don't check its comment form.
|
|
||||||
if gd.Lparen.IsValid() && gd.Doc != nil { |
|
||||||
return |
|
||||||
} |
|
||||||
// The relevant text to check will be on either vs.Doc or gd.Doc.
|
|
||||||
// Use vs.Doc preferentially.
|
|
||||||
doc := vs.Doc |
|
||||||
if doc == nil { |
|
||||||
doc = gd.Doc |
|
||||||
} |
|
||||||
prefix := name + " " |
|
||||||
s := normalizeText(doc.Text()) |
|
||||||
if !strings.HasPrefix(s, prefix) { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Node: doc, |
|
||||||
Category: "comments", |
|
||||||
Failure: fmt.Sprintf(`comment on exported %s %s should be of the form "%s..."`, kind, name, prefix), |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// normalizeText is a helper function that normalizes comment strings by:
|
|
||||||
// * removing one leading space
|
|
||||||
//
|
|
||||||
// This function is needed because ast.CommentGroup.Text() does not handle //-style and /*-style comments uniformly
|
|
||||||
func normalizeText(t string) string { |
|
||||||
return strings.TrimPrefix(t, " ") |
|
||||||
} |
|
||||||
|
|
||||||
func (w *lintExported) Visit(n ast.Node) ast.Visitor { |
|
||||||
switch v := n.(type) { |
|
||||||
case *ast.GenDecl: |
|
||||||
if v.Tok == token.IMPORT { |
|
||||||
return nil |
|
||||||
} |
|
||||||
// token.CONST, token.TYPE or token.VAR
|
|
||||||
w.lastGen = v |
|
||||||
return w |
|
||||||
case *ast.FuncDecl: |
|
||||||
w.lintFuncDoc(v) |
|
||||||
if v.Recv == nil { |
|
||||||
// Only check for stutter on functions, not methods.
|
|
||||||
// Method names are not used package-qualified.
|
|
||||||
w.checkStutter(v.Name, "func") |
|
||||||
} |
|
||||||
// Don't proceed inside funcs.
|
|
||||||
return nil |
|
||||||
case *ast.TypeSpec: |
|
||||||
// inside a GenDecl, which usually has the doc
|
|
||||||
doc := v.Doc |
|
||||||
if doc == nil { |
|
||||||
doc = w.lastGen.Doc |
|
||||||
} |
|
||||||
w.lintTypeDoc(v, doc) |
|
||||||
w.checkStutter(v.Name, "type") |
|
||||||
// Don't proceed inside types.
|
|
||||||
return nil |
|
||||||
case *ast.ValueSpec: |
|
||||||
w.lintValueSpecDoc(v, w.lastGen, w.genDeclMissingComments) |
|
||||||
return nil |
|
||||||
} |
|
||||||
return w |
|
||||||
} |
|
@ -1,69 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"regexp" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// FileHeaderRule lints given else constructs.
|
|
||||||
type FileHeaderRule struct{} |
|
||||||
|
|
||||||
var ( |
|
||||||
multiRegexp = regexp.MustCompile("^/\\*") |
|
||||||
singleRegexp = regexp.MustCompile("^//") |
|
||||||
) |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *FileHeaderRule) Apply(file *lint.File, arguments lint.Arguments) []lint.Failure { |
|
||||||
if len(arguments) != 1 { |
|
||||||
panic(`invalid configuration for "file-header" rule`) |
|
||||||
} |
|
||||||
|
|
||||||
header, ok := arguments[0].(string) |
|
||||||
if !ok { |
|
||||||
panic(`invalid argument for "file-header" rule: first argument should be a string`) |
|
||||||
} |
|
||||||
|
|
||||||
failure := []lint.Failure{ |
|
||||||
{ |
|
||||||
Node: file.AST, |
|
||||||
Confidence: 1, |
|
||||||
Failure: "the file doesn't have an appropriate header", |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
if len(file.AST.Comments) == 0 { |
|
||||||
return failure |
|
||||||
} |
|
||||||
|
|
||||||
g := file.AST.Comments[0] |
|
||||||
if g == nil { |
|
||||||
return failure |
|
||||||
} |
|
||||||
comment := "" |
|
||||||
for _, c := range g.List { |
|
||||||
text := c.Text |
|
||||||
if multiRegexp.Match([]byte(text)) { |
|
||||||
text = text[2 : len(text)-2] |
|
||||||
} else if singleRegexp.Match([]byte(text)) { |
|
||||||
text = text[2:] |
|
||||||
} |
|
||||||
comment += text |
|
||||||
} |
|
||||||
|
|
||||||
regex, err := regexp.Compile(header) |
|
||||||
if err != nil { |
|
||||||
panic(err.Error()) |
|
||||||
} |
|
||||||
|
|
||||||
if !regex.Match([]byte(comment)) { |
|
||||||
return failure |
|
||||||
} |
|
||||||
return nil |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *FileHeaderRule) Name() string { |
|
||||||
return "file-header" |
|
||||||
} |
|
@ -1,104 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
"go/ast" |
|
||||||
) |
|
||||||
|
|
||||||
// FlagParamRule lints given else constructs.
|
|
||||||
type FlagParamRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *FlagParamRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
onFailure := func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
} |
|
||||||
|
|
||||||
w := lintFlagParamRule{onFailure: onFailure} |
|
||||||
ast.Walk(w, file.AST) |
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *FlagParamRule) Name() string { |
|
||||||
return "flag-parameter" |
|
||||||
} |
|
||||||
|
|
||||||
type lintFlagParamRule struct { |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintFlagParamRule) Visit(node ast.Node) ast.Visitor { |
|
||||||
fd, ok := node.(*ast.FuncDecl) |
|
||||||
if !ok { |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
if fd.Body == nil { |
|
||||||
return nil // skip whole function declaration
|
|
||||||
} |
|
||||||
|
|
||||||
for _, p := range fd.Type.Params.List { |
|
||||||
t := p.Type |
|
||||||
|
|
||||||
id, ok := t.(*ast.Ident) |
|
||||||
if !ok { |
|
||||||
continue |
|
||||||
} |
|
||||||
|
|
||||||
if id.Name != "bool" { |
|
||||||
continue |
|
||||||
} |
|
||||||
|
|
||||||
cv := conditionVisitor{p.Names, fd, w} |
|
||||||
ast.Walk(cv, fd.Body) |
|
||||||
} |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
type conditionVisitor struct { |
|
||||||
ids []*ast.Ident |
|
||||||
fd *ast.FuncDecl |
|
||||||
linter lintFlagParamRule |
|
||||||
} |
|
||||||
|
|
||||||
func (w conditionVisitor) Visit(node ast.Node) ast.Visitor { |
|
||||||
ifStmt, ok := node.(*ast.IfStmt) |
|
||||||
if !ok { |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
fselect := func(n ast.Node) bool { |
|
||||||
ident, ok := n.(*ast.Ident) |
|
||||||
if !ok { |
|
||||||
return false |
|
||||||
} |
|
||||||
|
|
||||||
for _, id := range w.ids { |
|
||||||
if ident.Name == id.Name { |
|
||||||
return true |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return false |
|
||||||
} |
|
||||||
|
|
||||||
uses := pick(ifStmt.Cond, fselect, nil) |
|
||||||
|
|
||||||
if len(uses) < 1 { |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
w.linter.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Node: w.fd.Type.Params, |
|
||||||
Category: "bad practice", |
|
||||||
Failure: fmt.Sprintf("parameter '%s' seems to be a control flag, avoid control coupling", uses[0]), |
|
||||||
}) |
|
||||||
|
|
||||||
return nil |
|
||||||
} |
|
@ -1,153 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"go/ast" |
|
||||||
"reflect" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// FunctionLength lint.
|
|
||||||
type FunctionLength struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *FunctionLength) Apply(file *lint.File, arguments lint.Arguments) []lint.Failure { |
|
||||||
maxStmt, maxLines := r.parseArguments(arguments) |
|
||||||
|
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
walker := lintFuncLength{ |
|
||||||
file: file, |
|
||||||
maxStmt: int(maxStmt), |
|
||||||
maxLines: int(maxLines), |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
ast.Walk(walker, file.AST) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *FunctionLength) Name() string { |
|
||||||
return "function-length" |
|
||||||
} |
|
||||||
|
|
||||||
func (r *FunctionLength) parseArguments(arguments lint.Arguments) (maxStmt int64, maxLines int64) { |
|
||||||
if len(arguments) != 2 { |
|
||||||
panic(fmt.Sprintf(`invalid configuration for "function-length" rule, expected 2 arguments but got %d`, len(arguments))) |
|
||||||
} |
|
||||||
|
|
||||||
maxStmt, maxStmtOk := arguments[0].(int64) |
|
||||||
if !maxStmtOk { |
|
||||||
panic(fmt.Sprintf(`invalid configuration value for max statements in "function-length" rule; need int64 but got %T`, arguments[0])) |
|
||||||
} |
|
||||||
if maxStmt < 0 { |
|
||||||
panic(fmt.Sprintf(`the configuration value for max statements in "function-length" rule cannot be negative, got %d`, maxStmt)) |
|
||||||
} |
|
||||||
|
|
||||||
maxLines, maxLinesOk := arguments[1].(int64) |
|
||||||
if !maxLinesOk { |
|
||||||
panic(fmt.Sprintf(`invalid configuration value for max lines in "function-length" rule; need int64 but got %T`, arguments[1])) |
|
||||||
} |
|
||||||
if maxLines < 0 { |
|
||||||
panic(fmt.Sprintf(`the configuration value for max statements in "function-length" rule cannot be negative, got %d`, maxLines)) |
|
||||||
} |
|
||||||
|
|
||||||
return |
|
||||||
} |
|
||||||
|
|
||||||
type lintFuncLength struct { |
|
||||||
file *lint.File |
|
||||||
maxStmt int |
|
||||||
maxLines int |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintFuncLength) Visit(n ast.Node) ast.Visitor { |
|
||||||
node, ok := n.(*ast.FuncDecl) |
|
||||||
if !ok { |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
body := node.Body |
|
||||||
if body == nil || len(node.Body.List) == 0 { |
|
||||||
return nil |
|
||||||
} |
|
||||||
|
|
||||||
if w.maxStmt > 0 { |
|
||||||
stmtCount := w.countStmts(node.Body.List) |
|
||||||
if stmtCount > w.maxStmt { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Failure: fmt.Sprintf("maximum number of statements per function exceeded; max %d but got %d", w.maxStmt, stmtCount), |
|
||||||
Node: node, |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
if w.maxLines > 0 { |
|
||||||
lineCount := w.countLines(node.Body) |
|
||||||
if lineCount > w.maxLines { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Failure: fmt.Sprintf("maximum number of lines per function exceeded; max %d but got %d", w.maxLines, lineCount), |
|
||||||
Node: node, |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return nil |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintFuncLength) countLines(b *ast.BlockStmt) int { |
|
||||||
return w.file.ToPosition(b.End()).Line - w.file.ToPosition(b.Pos()).Line - 1 |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintFuncLength) countStmts(b []ast.Stmt) int { |
|
||||||
count := 0 |
|
||||||
for _, s := range b { |
|
||||||
switch stmt := s.(type) { |
|
||||||
case *ast.BlockStmt: |
|
||||||
count += w.countStmts(stmt.List) |
|
||||||
case *ast.IfStmt: |
|
||||||
count += 1 + w.countBodyListStmts(stmt) |
|
||||||
if stmt.Else != nil { |
|
||||||
elseBody, ok := stmt.Else.(*ast.BlockStmt) |
|
||||||
if ok { |
|
||||||
count += w.countStmts(elseBody.List) |
|
||||||
} |
|
||||||
} |
|
||||||
case *ast.ForStmt, *ast.RangeStmt, |
|
||||||
*ast.SwitchStmt, *ast.TypeSwitchStmt, *ast.SelectStmt: |
|
||||||
count += 1 + w.countBodyListStmts(stmt) |
|
||||||
case *ast.CaseClause: |
|
||||||
count += w.countStmts(stmt.Body) |
|
||||||
case *ast.AssignStmt: |
|
||||||
count += 1 + w.countFuncLitStmts(stmt.Rhs[0]) |
|
||||||
case *ast.GoStmt: |
|
||||||
count += 1 + w.countFuncLitStmts(stmt.Call.Fun) |
|
||||||
case *ast.DeferStmt: |
|
||||||
count += 1 + w.countFuncLitStmts(stmt.Call.Fun) |
|
||||||
default: |
|
||||||
count++ |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return count |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintFuncLength) countFuncLitStmts(stmt ast.Expr) int { |
|
||||||
if block, ok := stmt.(*ast.FuncLit); ok { |
|
||||||
return w.countStmts(block.Body.List) |
|
||||||
} |
|
||||||
return 0 |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintFuncLength) countBodyListStmts(t interface{}) int { |
|
||||||
i := reflect.ValueOf(t).Elem().FieldByName(`Body`).Elem().FieldByName(`List`).Interface() |
|
||||||
return w.countStmts(i.([]ast.Stmt)) |
|
||||||
} |
|
@ -1,68 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"go/ast" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// FunctionResultsLimitRule lints given else constructs.
|
|
||||||
type FunctionResultsLimitRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *FunctionResultsLimitRule) Apply(file *lint.File, arguments lint.Arguments) []lint.Failure { |
|
||||||
if len(arguments) != 1 { |
|
||||||
panic(`invalid configuration for "function-result-limit"`) |
|
||||||
} |
|
||||||
|
|
||||||
max, ok := arguments[0].(int64) // Alt. non panicking version
|
|
||||||
if !ok { |
|
||||||
panic(fmt.Sprintf(`invalid value passed as return results number to the "function-result-limit" rule; need int64 but got %T`, arguments[0])) |
|
||||||
} |
|
||||||
if max < 0 { |
|
||||||
panic(`the value passed as return results number to the "function-result-limit" rule cannot be negative`) |
|
||||||
} |
|
||||||
|
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
walker := lintFunctionResultsNum{ |
|
||||||
max: int(max), |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
ast.Walk(walker, file.AST) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *FunctionResultsLimitRule) Name() string { |
|
||||||
return "function-result-limit" |
|
||||||
} |
|
||||||
|
|
||||||
type lintFunctionResultsNum struct { |
|
||||||
max int |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintFunctionResultsNum) Visit(n ast.Node) ast.Visitor { |
|
||||||
node, ok := n.(*ast.FuncDecl) |
|
||||||
if ok { |
|
||||||
num := 0 |
|
||||||
if node.Type.Results != nil { |
|
||||||
num = node.Type.Results.NumFields() |
|
||||||
} |
|
||||||
if num > w.max { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Failure: fmt.Sprintf("maximum number of return results per function exceeded; max %d but got %d", w.max, num), |
|
||||||
Node: node.Type, |
|
||||||
}) |
|
||||||
return w |
|
||||||
} |
|
||||||
} |
|
||||||
return w |
|
||||||
} |
|
@ -1,70 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"go/ast" |
|
||||||
"strings" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// GetReturnRule lints given else constructs.
|
|
||||||
type GetReturnRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *GetReturnRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
onFailure := func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
} |
|
||||||
|
|
||||||
w := lintReturnRule{onFailure} |
|
||||||
ast.Walk(w, file.AST) |
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *GetReturnRule) Name() string { |
|
||||||
return "get-return" |
|
||||||
} |
|
||||||
|
|
||||||
type lintReturnRule struct { |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func isGetter(name string) bool { |
|
||||||
if strings.HasPrefix(strings.ToUpper(name), "GET") { |
|
||||||
if len(name) > 3 { |
|
||||||
c := name[3] |
|
||||||
return !(c >= 'a' && c <= 'z') |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return false |
|
||||||
} |
|
||||||
|
|
||||||
func hasResults(rs *ast.FieldList) bool { |
|
||||||
return rs != nil && len(rs.List) > 0 |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintReturnRule) Visit(node ast.Node) ast.Visitor { |
|
||||||
fd, ok := node.(*ast.FuncDecl) |
|
||||||
if !ok { |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
if !isGetter(fd.Name.Name) { |
|
||||||
return w |
|
||||||
} |
|
||||||
if !hasResults(fd.Type.Results) { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 0.8, |
|
||||||
Node: fd, |
|
||||||
Category: "logic", |
|
||||||
Failure: fmt.Sprintf("function '%s' seems to be a getter but it does not return any result", fd.Name.Name), |
|
||||||
}) |
|
||||||
} |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
@ -1,82 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// IdenticalBranchesRule warns on constant logical expressions.
|
|
||||||
type IdenticalBranchesRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *IdenticalBranchesRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
onFailure := func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
} |
|
||||||
|
|
||||||
astFile := file.AST |
|
||||||
w := &lintIdenticalBranches{astFile, onFailure} |
|
||||||
ast.Walk(w, astFile) |
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *IdenticalBranchesRule) Name() string { |
|
||||||
return "identical-branches" |
|
||||||
} |
|
||||||
|
|
||||||
type lintIdenticalBranches struct { |
|
||||||
file *ast.File |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w *lintIdenticalBranches) Visit(node ast.Node) ast.Visitor { |
|
||||||
n, ok := node.(*ast.IfStmt) |
|
||||||
if !ok { |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
if n.Else == nil { |
|
||||||
return w |
|
||||||
} |
|
||||||
branches := []*ast.BlockStmt{n.Body} |
|
||||||
|
|
||||||
elseBranch, ok := n.Else.(*ast.BlockStmt) |
|
||||||
if !ok { // if-else-if construction
|
|
||||||
return w |
|
||||||
} |
|
||||||
branches = append(branches, elseBranch) |
|
||||||
|
|
||||||
if w.identicalBranches(branches) { |
|
||||||
w.newFailure(n, "both branches of the if are identical") |
|
||||||
} |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
func (w *lintIdenticalBranches) identicalBranches(branches []*ast.BlockStmt) bool { |
|
||||||
if len(branches) < 2 { |
|
||||||
return false |
|
||||||
} |
|
||||||
|
|
||||||
ref := gofmt(branches[0]) |
|
||||||
for i := 1; i < len(branches); i++ { |
|
||||||
if gofmt(branches[i]) != ref { |
|
||||||
return false |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return true |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintIdenticalBranches) newFailure(node ast.Node, msg string) { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Node: node, |
|
||||||
Category: "logic", |
|
||||||
Failure: msg, |
|
||||||
}) |
|
||||||
} |
|
@ -1,115 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
"go/token" |
|
||||||
"strings" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// IfReturnRule lints given else constructs.
|
|
||||||
type IfReturnRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *IfReturnRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
onFailure := func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
} |
|
||||||
|
|
||||||
astFile := file.AST |
|
||||||
w := &lintElseError{astFile, onFailure} |
|
||||||
ast.Walk(w, astFile) |
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *IfReturnRule) Name() string { |
|
||||||
return "if-return" |
|
||||||
} |
|
||||||
|
|
||||||
type lintElseError struct { |
|
||||||
file *ast.File |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w *lintElseError) Visit(node ast.Node) ast.Visitor { |
|
||||||
switch v := node.(type) { |
|
||||||
case *ast.BlockStmt: |
|
||||||
for i := 0; i < len(v.List)-1; i++ { |
|
||||||
// if var := whatever; var != nil { return var }
|
|
||||||
s, ok := v.List[i].(*ast.IfStmt) |
|
||||||
if !ok || s.Body == nil || len(s.Body.List) != 1 || s.Else != nil { |
|
||||||
continue |
|
||||||
} |
|
||||||
assign, ok := s.Init.(*ast.AssignStmt) |
|
||||||
if !ok || len(assign.Lhs) != 1 || !(assign.Tok == token.DEFINE || assign.Tok == token.ASSIGN) { |
|
||||||
continue |
|
||||||
} |
|
||||||
id, ok := assign.Lhs[0].(*ast.Ident) |
|
||||||
if !ok { |
|
||||||
continue |
|
||||||
} |
|
||||||
expr, ok := s.Cond.(*ast.BinaryExpr) |
|
||||||
if !ok || expr.Op != token.NEQ { |
|
||||||
continue |
|
||||||
} |
|
||||||
if lhs, ok := expr.X.(*ast.Ident); !ok || lhs.Name != id.Name { |
|
||||||
continue |
|
||||||
} |
|
||||||
if rhs, ok := expr.Y.(*ast.Ident); !ok || rhs.Name != "nil" { |
|
||||||
continue |
|
||||||
} |
|
||||||
r, ok := s.Body.List[0].(*ast.ReturnStmt) |
|
||||||
if !ok || len(r.Results) != 1 { |
|
||||||
continue |
|
||||||
} |
|
||||||
if r, ok := r.Results[0].(*ast.Ident); !ok || r.Name != id.Name { |
|
||||||
continue |
|
||||||
} |
|
||||||
|
|
||||||
// return nil
|
|
||||||
r, ok = v.List[i+1].(*ast.ReturnStmt) |
|
||||||
if !ok || len(r.Results) != 1 { |
|
||||||
continue |
|
||||||
} |
|
||||||
if r, ok := r.Results[0].(*ast.Ident); !ok || r.Name != "nil" { |
|
||||||
continue |
|
||||||
} |
|
||||||
|
|
||||||
// check if there are any comments explaining the construct, don't emit an error if there are some.
|
|
||||||
if containsComments(s.Pos(), r.Pos(), w.file) { |
|
||||||
continue |
|
||||||
} |
|
||||||
|
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: .9, |
|
||||||
Node: v.List[i], |
|
||||||
Failure: "redundant if ...; err != nil check, just return error instead.", |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
func containsComments(start, end token.Pos, f *ast.File) bool { |
|
||||||
for _, cgroup := range f.Comments { |
|
||||||
comments := cgroup.List |
|
||||||
if comments[0].Slash >= end { |
|
||||||
// All comments starting with this group are after end pos.
|
|
||||||
return false |
|
||||||
} |
|
||||||
if comments[len(comments)-1].Slash < start { |
|
||||||
// Comments group ends before start pos.
|
|
||||||
continue |
|
||||||
} |
|
||||||
for _, c := range comments { |
|
||||||
if start <= c.Slash && c.Slash < end && !strings.HasPrefix(c.Text, "// MATCH ") { |
|
||||||
return true |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
return false |
|
||||||
} |
|
@ -1,102 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"go/ast" |
|
||||||
"go/token" |
|
||||||
"strings" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// ImportShadowingRule lints given else constructs.
|
|
||||||
type ImportShadowingRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *ImportShadowingRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
importNames := map[string]struct{}{} |
|
||||||
for _, imp := range file.AST.Imports { |
|
||||||
importNames[getName(imp)] = struct{}{} |
|
||||||
} |
|
||||||
|
|
||||||
fileAst := file.AST |
|
||||||
walker := importShadowing{ |
|
||||||
importNames: importNames, |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
alreadySeen: map[*ast.Object]struct{}{}, |
|
||||||
} |
|
||||||
|
|
||||||
ast.Walk(walker, fileAst) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *ImportShadowingRule) Name() string { |
|
||||||
return "import-shadowing" |
|
||||||
} |
|
||||||
|
|
||||||
func getName(imp *ast.ImportSpec) string { |
|
||||||
const pathSep = "/" |
|
||||||
const strDelim = `"` |
|
||||||
if imp.Name != nil { |
|
||||||
return imp.Name.Name |
|
||||||
} |
|
||||||
|
|
||||||
path := imp.Path.Value |
|
||||||
i := strings.LastIndex(path, pathSep) |
|
||||||
if i == -1 { |
|
||||||
return strings.Trim(path, strDelim) |
|
||||||
} |
|
||||||
|
|
||||||
return strings.Trim(path[i+1:], strDelim) |
|
||||||
} |
|
||||||
|
|
||||||
type importShadowing struct { |
|
||||||
importNames map[string]struct{} |
|
||||||
onFailure func(lint.Failure) |
|
||||||
alreadySeen map[*ast.Object]struct{} |
|
||||||
} |
|
||||||
|
|
||||||
// Visit visits AST nodes and checks if id nodes (ast.Ident) shadow an import name
|
|
||||||
func (w importShadowing) Visit(n ast.Node) ast.Visitor { |
|
||||||
switch n := n.(type) { |
|
||||||
case *ast.AssignStmt: |
|
||||||
if n.Tok == token.DEFINE { |
|
||||||
return w // analyze variable declarations of the form id := expr
|
|
||||||
} |
|
||||||
|
|
||||||
return nil // skip assigns of the form id = expr (not an id declaration)
|
|
||||||
case *ast.CallExpr, // skip call expressions (not an id declaration)
|
|
||||||
*ast.ImportSpec, // skip import section subtree because we already have the list of imports
|
|
||||||
*ast.KeyValueExpr, // skip analysis of key-val expressions ({key:value}): ids of such expressions, even the same of an import name, do not shadow the import name
|
|
||||||
*ast.ReturnStmt, // skip skipping analysis of returns, ids in expression were already analyzed
|
|
||||||
*ast.SelectorExpr, // skip analysis of selector expressions (anId.otherId): because if anId shadows an import name, it was already detected, and otherId does not shadows the import name
|
|
||||||
*ast.StructType: // skip analysis of struct type because struct fields can not shadow an import name
|
|
||||||
return nil |
|
||||||
case *ast.Ident: |
|
||||||
id := n.Name |
|
||||||
if id == "_" { |
|
||||||
return w // skip _ id
|
|
||||||
} |
|
||||||
|
|
||||||
_, isImportName := w.importNames[id] |
|
||||||
_, alreadySeen := w.alreadySeen[n.Obj] |
|
||||||
if isImportName && !alreadySeen { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Node: n, |
|
||||||
Category: "namming", |
|
||||||
Failure: fmt.Sprintf("The name '%s' shadows an import name", id), |
|
||||||
}) |
|
||||||
|
|
||||||
w.alreadySeen[n.Obj] = struct{}{} |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
@ -1,52 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// ImportsBlacklistRule lints given else constructs.
|
|
||||||
type ImportsBlacklistRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *ImportsBlacklistRule) Apply(file *lint.File, arguments lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
if file.IsTest() { |
|
||||||
return failures // skip, test file
|
|
||||||
} |
|
||||||
|
|
||||||
blacklist := make(map[string]bool, len(arguments)) |
|
||||||
|
|
||||||
for _, arg := range arguments { |
|
||||||
argStr, ok := arg.(string) |
|
||||||
if !ok { |
|
||||||
panic(fmt.Sprintf("Invalid argument to the imports-blacklist rule. Expecting a string, got %T", arg)) |
|
||||||
} |
|
||||||
// we add quotes if not present, because when parsed, the value of the AST node, will be quoted
|
|
||||||
if len(argStr) > 2 && argStr[0] != '"' && argStr[len(argStr)-1] != '"' { |
|
||||||
argStr = fmt.Sprintf(`"%s"`, argStr) |
|
||||||
} |
|
||||||
blacklist[argStr] = true |
|
||||||
} |
|
||||||
|
|
||||||
for _, is := range file.AST.Imports { |
|
||||||
path := is.Path |
|
||||||
if path != nil && blacklist[path.Value] { |
|
||||||
failures = append(failures, lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Failure: "should not use the following blacklisted import: " + path.Value, |
|
||||||
Node: is, |
|
||||||
Category: "imports", |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *ImportsBlacklistRule) Name() string { |
|
||||||
return "imports-blacklist" |
|
||||||
} |
|
@ -1,74 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"go/ast" |
|
||||||
"go/token" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// IncrementDecrementRule lints given else constructs.
|
|
||||||
type IncrementDecrementRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *IncrementDecrementRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
fileAst := file.AST |
|
||||||
walker := lintIncrementDecrement{ |
|
||||||
file: file, |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
ast.Walk(walker, fileAst) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *IncrementDecrementRule) Name() string { |
|
||||||
return "increment-decrement" |
|
||||||
} |
|
||||||
|
|
||||||
type lintIncrementDecrement struct { |
|
||||||
file *lint.File |
|
||||||
fileAst *ast.File |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintIncrementDecrement) Visit(n ast.Node) ast.Visitor { |
|
||||||
as, ok := n.(*ast.AssignStmt) |
|
||||||
if !ok { |
|
||||||
return w |
|
||||||
} |
|
||||||
if len(as.Lhs) != 1 { |
|
||||||
return w |
|
||||||
} |
|
||||||
if !isOne(as.Rhs[0]) { |
|
||||||
return w |
|
||||||
} |
|
||||||
var suffix string |
|
||||||
switch as.Tok { |
|
||||||
case token.ADD_ASSIGN: |
|
||||||
suffix = "++" |
|
||||||
case token.SUB_ASSIGN: |
|
||||||
suffix = "--" |
|
||||||
default: |
|
||||||
return w |
|
||||||
} |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 0.8, |
|
||||||
Node: as, |
|
||||||
Category: "unary-op", |
|
||||||
Failure: fmt.Sprintf("should replace %s with %s%s", w.file.Render(as), w.file.Render(as.Lhs[0]), suffix), |
|
||||||
}) |
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
func isOne(expr ast.Expr) bool { |
|
||||||
lit, ok := expr.(*ast.BasicLit) |
|
||||||
return ok && lit.Kind == token.INT && lit.Value == "1" |
|
||||||
} |
|
@ -1,78 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
"go/token" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// IndentErrorFlowRule lints given else constructs.
|
|
||||||
type IndentErrorFlowRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *IndentErrorFlowRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
onFailure := func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
} |
|
||||||
|
|
||||||
w := lintElse{make(map[*ast.IfStmt]bool), onFailure} |
|
||||||
ast.Walk(w, file.AST) |
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *IndentErrorFlowRule) Name() string { |
|
||||||
return "indent-error-flow" |
|
||||||
} |
|
||||||
|
|
||||||
type lintElse struct { |
|
||||||
ignore map[*ast.IfStmt]bool |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintElse) Visit(node ast.Node) ast.Visitor { |
|
||||||
ifStmt, ok := node.(*ast.IfStmt) |
|
||||||
if !ok || ifStmt.Else == nil { |
|
||||||
return w |
|
||||||
} |
|
||||||
if w.ignore[ifStmt] { |
|
||||||
if elseif, ok := ifStmt.Else.(*ast.IfStmt); ok { |
|
||||||
w.ignore[elseif] = true |
|
||||||
} |
|
||||||
return w |
|
||||||
} |
|
||||||
if elseif, ok := ifStmt.Else.(*ast.IfStmt); ok { |
|
||||||
w.ignore[elseif] = true |
|
||||||
return w |
|
||||||
} |
|
||||||
if _, ok := ifStmt.Else.(*ast.BlockStmt); !ok { |
|
||||||
// only care about elses without conditions
|
|
||||||
return w |
|
||||||
} |
|
||||||
if len(ifStmt.Body.List) == 0 { |
|
||||||
return w |
|
||||||
} |
|
||||||
shortDecl := false // does the if statement have a ":=" initialization statement?
|
|
||||||
if ifStmt.Init != nil { |
|
||||||
if as, ok := ifStmt.Init.(*ast.AssignStmt); ok && as.Tok == token.DEFINE { |
|
||||||
shortDecl = true |
|
||||||
} |
|
||||||
} |
|
||||||
lastStmt := ifStmt.Body.List[len(ifStmt.Body.List)-1] |
|
||||||
if _, ok := lastStmt.(*ast.ReturnStmt); ok { |
|
||||||
extra := "" |
|
||||||
if shortDecl { |
|
||||||
extra = " (move short variable declaration to its own line if necessary)" |
|
||||||
} |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 1, |
|
||||||
Node: ifStmt.Else, |
|
||||||
Category: "indent", |
|
||||||
Failure: "if block ends with a return statement, so drop this else and outdent its block" + extra, |
|
||||||
}) |
|
||||||
} |
|
||||||
return w |
|
||||||
} |
|
@ -1,84 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"bufio" |
|
||||||
"bytes" |
|
||||||
"fmt" |
|
||||||
"go/token" |
|
||||||
"strings" |
|
||||||
"unicode/utf8" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// LineLengthLimitRule lints given else constructs.
|
|
||||||
type LineLengthLimitRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *LineLengthLimitRule) Apply(file *lint.File, arguments lint.Arguments) []lint.Failure { |
|
||||||
if len(arguments) != 1 { |
|
||||||
panic(`invalid configuration for "line-length-limit"`) |
|
||||||
} |
|
||||||
|
|
||||||
max, ok := arguments[0].(int64) // Alt. non panicking version
|
|
||||||
if !ok || max < 0 { |
|
||||||
panic(`invalid value passed as argument number to the "line-length-limit" rule`) |
|
||||||
} |
|
||||||
|
|
||||||
var failures []lint.Failure |
|
||||||
checker := lintLineLengthNum{ |
|
||||||
max: int(max), |
|
||||||
file: file, |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
checker.check() |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *LineLengthLimitRule) Name() string { |
|
||||||
return "line-length-limit" |
|
||||||
} |
|
||||||
|
|
||||||
type lintLineLengthNum struct { |
|
||||||
max int |
|
||||||
file *lint.File |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (r lintLineLengthNum) check() { |
|
||||||
f := bytes.NewReader(r.file.Content()) |
|
||||||
spaces := strings.Repeat(" ", 4) // tab width = 4
|
|
||||||
l := 1 |
|
||||||
s := bufio.NewScanner(f) |
|
||||||
for s.Scan() { |
|
||||||
t := s.Text() |
|
||||||
t = strings.Replace(t, "\t", spaces, -1) |
|
||||||
c := utf8.RuneCountInString(t) |
|
||||||
if c > r.max { |
|
||||||
r.onFailure(lint.Failure{ |
|
||||||
Category: "code-style", |
|
||||||
Position: lint.FailurePosition{ |
|
||||||
// Offset not set; it is non-trivial, and doesn't appear to be needed.
|
|
||||||
Start: token.Position{ |
|
||||||
Filename: r.file.Name, |
|
||||||
Line: l, |
|
||||||
Column: 0, |
|
||||||
}, |
|
||||||
End: token.Position{ |
|
||||||
Filename: r.file.Name, |
|
||||||
Line: l, |
|
||||||
Column: c, |
|
||||||
}, |
|
||||||
}, |
|
||||||
Confidence: 1, |
|
||||||
Failure: fmt.Sprintf("line is %d characters, out of limit %d", c, r.max), |
|
||||||
}) |
|
||||||
} |
|
||||||
l++ |
|
||||||
} |
|
||||||
} |
|
@ -1,67 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
|
|
||||||
"strings" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// MaxPublicStructsRule lints given else constructs.
|
|
||||||
type MaxPublicStructsRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *MaxPublicStructsRule) Apply(file *lint.File, arguments lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
fileAst := file.AST |
|
||||||
walker := &lintMaxPublicStructs{ |
|
||||||
fileAst: fileAst, |
|
||||||
onFailure: func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
}, |
|
||||||
} |
|
||||||
|
|
||||||
ast.Walk(walker, fileAst) |
|
||||||
|
|
||||||
max, ok := arguments[0].(int64) // Alt. non panicking version
|
|
||||||
if !ok { |
|
||||||
panic(`invalid value passed as argument number to the "max-public-structs" rule`) |
|
||||||
} |
|
||||||
|
|
||||||
if walker.current > max { |
|
||||||
walker.onFailure(lint.Failure{ |
|
||||||
Failure: "you have exceeded the maximum number of public struct declarations", |
|
||||||
Confidence: 1, |
|
||||||
Node: fileAst, |
|
||||||
Category: "style", |
|
||||||
}) |
|
||||||
} |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *MaxPublicStructsRule) Name() string { |
|
||||||
return "max-public-structs" |
|
||||||
} |
|
||||||
|
|
||||||
type lintMaxPublicStructs struct { |
|
||||||
current int64 |
|
||||||
fileAst *ast.File |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w *lintMaxPublicStructs) Visit(n ast.Node) ast.Visitor { |
|
||||||
switch v := n.(type) { |
|
||||||
case *ast.TypeSpec: |
|
||||||
name := v.Name.Name |
|
||||||
first := string(name[0]) |
|
||||||
if strings.ToUpper(first) == first { |
|
||||||
w.current++ |
|
||||||
} |
|
||||||
break |
|
||||||
} |
|
||||||
return w |
|
||||||
} |
|
@ -1,80 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"go/ast" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// ModifiesParamRule lints given else constructs.
|
|
||||||
type ModifiesParamRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *ModifiesParamRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
onFailure := func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
} |
|
||||||
|
|
||||||
w := lintModifiesParamRule{onFailure: onFailure} |
|
||||||
ast.Walk(w, file.AST) |
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *ModifiesParamRule) Name() string { |
|
||||||
return "modifies-parameter" |
|
||||||
} |
|
||||||
|
|
||||||
type lintModifiesParamRule struct { |
|
||||||
params map[string]bool |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func retrieveParamNames(pl []*ast.Field) map[string]bool { |
|
||||||
result := make(map[string]bool, len(pl)) |
|
||||||
for _, p := range pl { |
|
||||||
for _, n := range p.Names { |
|
||||||
if n.Name == "_" { |
|
||||||
continue |
|
||||||
} |
|
||||||
|
|
||||||
result[n.Name] = true |
|
||||||
} |
|
||||||
} |
|
||||||
return result |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintModifiesParamRule) Visit(node ast.Node) ast.Visitor { |
|
||||||
switch v := node.(type) { |
|
||||||
case *ast.FuncDecl: |
|
||||||
w.params = retrieveParamNames(v.Type.Params.List) |
|
||||||
case *ast.IncDecStmt: |
|
||||||
if id, ok := v.X.(*ast.Ident); ok { |
|
||||||
checkParam(id, &w) |
|
||||||
} |
|
||||||
case *ast.AssignStmt: |
|
||||||
lhs := v.Lhs |
|
||||||
for _, e := range lhs { |
|
||||||
id, ok := e.(*ast.Ident) |
|
||||||
if ok { |
|
||||||
checkParam(id, &w) |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
func checkParam(id *ast.Ident, w *lintModifiesParamRule) { |
|
||||||
if w.params[id.Name] { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Confidence: 0.5, // confidence is low because of shadow variables
|
|
||||||
Node: id, |
|
||||||
Category: "bad practice", |
|
||||||
Failure: fmt.Sprintf("parameter '%s' seems to be modified", id), |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
@ -1,134 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"go/ast" |
|
||||||
"strings" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// ModifiesValRecRule lints assignments to value method-receivers.
|
|
||||||
type ModifiesValRecRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *ModifiesValRecRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
onFailure := func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
} |
|
||||||
|
|
||||||
w := lintModifiesValRecRule{file: file, onFailure: onFailure} |
|
||||||
file.Pkg.TypeCheck() |
|
||||||
ast.Walk(w, file.AST) |
|
||||||
|
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *ModifiesValRecRule) Name() string { |
|
||||||
return "modifies-value-receiver" |
|
||||||
} |
|
||||||
|
|
||||||
type lintModifiesValRecRule struct { |
|
||||||
file *lint.File |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintModifiesValRecRule) Visit(node ast.Node) ast.Visitor { |
|
||||||
switch n := node.(type) { |
|
||||||
case *ast.FuncDecl: |
|
||||||
if n.Recv == nil { |
|
||||||
return nil // skip, not a method
|
|
||||||
} |
|
||||||
|
|
||||||
receiver := n.Recv.List[0] |
|
||||||
if _, ok := receiver.Type.(*ast.StarExpr); ok { |
|
||||||
return nil // skip, method with pointer receiver
|
|
||||||
} |
|
||||||
|
|
||||||
if w.skipType(receiver.Type) { |
|
||||||
return nil // skip, receiver is a map or array
|
|
||||||
} |
|
||||||
|
|
||||||
if len(receiver.Names) < 1 { |
|
||||||
return nil // skip, anonymous receiver
|
|
||||||
} |
|
||||||
|
|
||||||
receiverName := receiver.Names[0].Name |
|
||||||
if receiverName == "_" { |
|
||||||
return nil // skip, anonymous receiver
|
|
||||||
} |
|
||||||
|
|
||||||
fselect := func(n ast.Node) bool { |
|
||||||
// look for assignments with the receiver in the right hand
|
|
||||||
asgmt, ok := n.(*ast.AssignStmt) |
|
||||||
if !ok { |
|
||||||
return false |
|
||||||
} |
|
||||||
|
|
||||||
for _, exp := range asgmt.Lhs { |
|
||||||
switch e := exp.(type) { |
|
||||||
case *ast.IndexExpr: // receiver...[] = ...
|
|
||||||
continue |
|
||||||
case *ast.StarExpr: // *receiver = ...
|
|
||||||
continue |
|
||||||
case *ast.SelectorExpr: // receiver.field = ...
|
|
||||||
name := w.getNameFromExpr(e.X) |
|
||||||
if name == "" || name != receiverName { |
|
||||||
continue |
|
||||||
} |
|
||||||
|
|
||||||
if w.skipType(ast.Expr(e.Sel)) { |
|
||||||
continue |
|
||||||
} |
|
||||||
|
|
||||||
case *ast.Ident: // receiver := ...
|
|
||||||
if e.Name != receiverName { |
|
||||||
continue |
|
||||||
} |
|
||||||
default: |
|
||||||
continue |
|
||||||
} |
|
||||||
|
|
||||||
return true |
|
||||||
} |
|
||||||
|
|
||||||
return false |
|
||||||
} |
|
||||||
|
|
||||||
assignmentsToReceiver := pick(n.Body, fselect, nil) |
|
||||||
|
|
||||||
for _, assignment := range assignmentsToReceiver { |
|
||||||
w.onFailure(lint.Failure{ |
|
||||||
Node: assignment, |
|
||||||
Confidence: 1, |
|
||||||
Failure: "suspicious assignment to a by-value method receiver", |
|
||||||
}) |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
return w |
|
||||||
} |
|
||||||
|
|
||||||
func (w lintModifiesValRecRule) skipType(t ast.Expr) bool { |
|
||||||
rt := w.file.Pkg.TypeOf(t) |
|
||||||
if rt == nil { |
|
||||||
return false |
|
||||||
} |
|
||||||
|
|
||||||
rt = rt.Underlying() |
|
||||||
rtName := rt.String() |
|
||||||
|
|
||||||
// skip when receiver is a map or array
|
|
||||||
return strings.HasPrefix(rtName, "[]") || strings.HasPrefix(rtName, "map[") |
|
||||||
} |
|
||||||
|
|
||||||
func (lintModifiesValRecRule) getNameFromExpr(ie ast.Expr) string { |
|
||||||
ident, ok := ie.(*ast.Ident) |
|
||||||
if !ok { |
|
||||||
return "" |
|
||||||
} |
|
||||||
|
|
||||||
return ident.Name |
|
||||||
} |
|
@ -1,121 +0,0 @@ |
|||||||
package rule |
|
||||||
|
|
||||||
import ( |
|
||||||
"fmt" |
|
||||||
"go/ast" |
|
||||||
"go/token" |
|
||||||
"strings" |
|
||||||
|
|
||||||
"github.com/mgechev/revive/lint" |
|
||||||
) |
|
||||||
|
|
||||||
// PackageCommentsRule lints the package comments. It complains if
|
|
||||||
// there is no package comment, or if it is not of the right form.
|
|
||||||
// This has a notable false positive in that a package comment
|
|
||||||
// could rightfully appear in a different file of the same package,
|
|
||||||
// but that's not easy to fix since this linter is file-oriented.
|
|
||||||
type PackageCommentsRule struct{} |
|
||||||
|
|
||||||
// Apply applies the rule to given file.
|
|
||||||
func (r *PackageCommentsRule) Apply(file *lint.File, _ lint.Arguments) []lint.Failure { |
|
||||||
var failures []lint.Failure |
|
||||||
|
|
||||||
if isTest(file) { |
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
onFailure := func(failure lint.Failure) { |
|
||||||
failures = append(failures, failure) |
|
||||||
} |
|
||||||
|
|
||||||
fileAst := file.AST |
|
||||||
w := &lintPackageComments{fileAst, file, onFailure} |
|
||||||
ast.Walk(w, fileAst) |
|
||||||
return failures |
|
||||||
} |
|
||||||
|
|
||||||
// Name returns the rule name.
|
|
||||||
func (r *PackageCommentsRule) Name() string { |
|
||||||
return "package-comments" |
|
||||||
} |
|
||||||
|
|
||||||
type lintPackageComments struct { |
|
||||||
fileAst *ast.File |
|
||||||
file *lint.File |
|
||||||
onFailure func(lint.Failure) |
|
||||||
} |
|
||||||
|
|
||||||
func (l *lintPackageComments) Visit(_ ast.Node) ast.Visitor { |
|
||||||
if l.file.IsTest() { |
|
||||||
return nil |
|
||||||
} |
|
||||||
|
|
||||||
const ref = styleGuideBase + "#package-comments" |
|
||||||
prefix := "Package " + l.fileAst.Name.Name + " " |
|
||||||
|
|
||||||
// Look for a detached package comment.
|
|
||||||
// First, scan for the last comment that occurs before the "package" keyword.
|
|
||||||
var lastCG *ast.CommentGroup |
|
||||||
for _, cg := range l.fileAst.Comments { |
|
||||||
if cg.Pos() > l.fileAst.Package { |
|
||||||
// Gone past "package" keyword.
|
|
||||||
break |
|
||||||
} |
|
||||||
lastCG = cg |
|
||||||
} |
|
||||||
if lastCG != nil && strings.HasPrefix(lastCG.Text(), prefix) { |
|
||||||
endPos := l.file.ToPosition(lastCG.End()) |
|
||||||
pkgPos := l.file.ToPosition(l.fileAst.Package) |
|
||||||
if endPos.Line+1 < pkgPos.Line { |
|
||||||
// There isn't a great place to anchor this error;
|
|
||||||
// the start of the blank lines between the doc and the package statement
|
|
||||||
// is at least pointing at the location of the problem.
|
|
||||||
pos := token.Position{ |
|
||||||
Filename: endPos.Filename, |
|
||||||
// Offset not set; it is non-trivial, and doesn't appear to be needed.
|
|
||||||
Line: endPos.Line + 1, |
|
||||||
Column: 1, |
|
||||||
} |
|
||||||
l.onFailure(lint.Failure{ |
|
||||||
Category: "comments", |
|
||||||
Position: lint.FailurePosition{ |
|
||||||
Start: pos, |
|
||||||
End: pos, |
|
||||||
}, |
|
||||||
Confidence: 0.9, |
|
||||||
Failure: "package comment is detached; there should be no blank lines between it and the package statement", |
|
||||||
}) |
|
||||||
return nil |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
if l.fileAst.Doc == nil { |
|
||||||
l.onFailure(lint.Failure{ |
|
||||||
Category: "comments", |
|
||||||
Node: l.fileAst, |
|
||||||
Confidence: 0.2, |
|
||||||
Failure: "should have a package comment, unless it's in another file for this package", |
|
||||||
}) |
|
||||||
return nil |
|
||||||
} |
|
||||||
s := l.fileAst.Doc.Text() |
|
||||||
if ts := strings.TrimLeft(s, " \t"); ts != s { |
|
||||||
l.onFailure(lint.Failure{ |
|
||||||
Category: "comments", |
|
||||||
Node: l.fileAst.Doc, |
|
||||||
Confidence: 1, |
|
||||||
Failure: "package comment should not have leading space", |
|
||||||
}) |
|
||||||
s = ts |
|
||||||
} |
|
||||||
// Only non-main packages need to keep to this form.
|
|
||||||
if !l.file.Pkg.IsMain() && !strings.HasPrefix(s, prefix) { |
|
||||||
l.onFailure(lint.Failure{ |
|
||||||
Category: "comments", |
|
||||||
Node: l.fileAst.Doc, |
|
||||||
Confidence: 1, |
|
||||||
Failure: fmt.Sprintf(`package comment should be of the form "%s..."`, prefix), |
|
||||||
}) |
|
||||||
} |
|
||||||
return nil |
|
||||||
} |
|
Some files were not shown because too many files have changed in this diff Show More
Loading…
Reference in new issue